JavaScript : Everything You Need to Know to Get Started

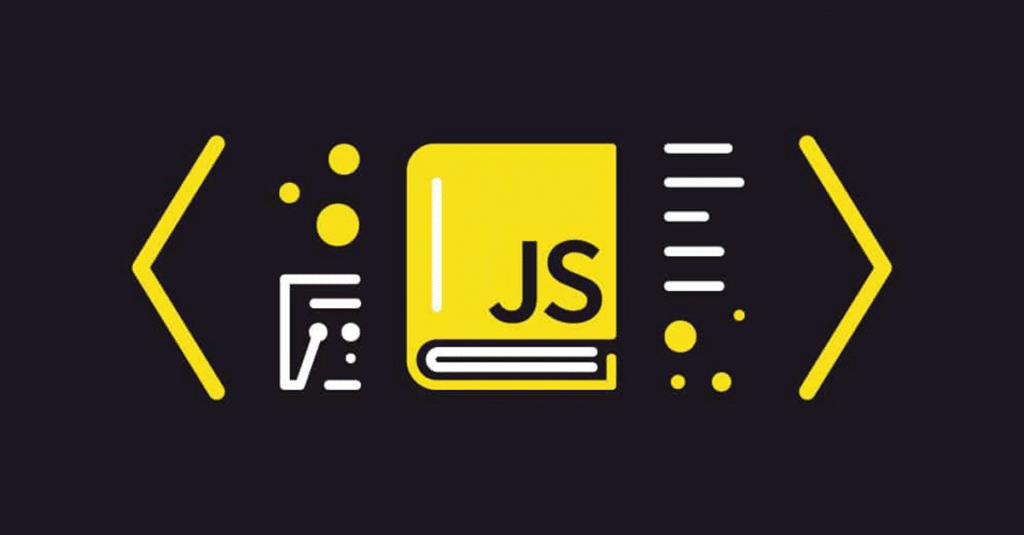
Introduction
JavaScript is a versatile and powerful programming language used to create interactive web pages and applications. Understanding its fundamentals is essential for anyone looking to build dynamic and efficient web solutions. This blog covers the core concepts of JavaScript, helping you establish a strong foundation.
1. Why Use JavaScript?
JavaScript is one of the most widely used programming languages due to its flexibility and vast ecosystem. Here’s why you should use JavaScript:
Client-Side Interactivity: JavaScript enables interactive web pages by handling user interactions like clicks, form submissions, and animations.
Server-Side Development: With Node.js, JavaScript can be used on the backend, making it a full-stack development language.
Cross-Platform Compatibility: JavaScript works on all modern web browsers without needing additional software.
Rich Ecosystem & Community: JavaScript has an extensive library ecosystem, including React, Angular, Vue, and Express.js, supporting rapid development.
Asynchronous Processing: JavaScript supports asynchronous programming (using callbacks, promises, and async/await) to handle API requests efficiently.
2. Where to Use JavaScript?
JavaScript is used in various domains, including:
Web Development: Enhancing the interactivity of websites.
Mobile App Development: Frameworks like React Native allow building mobile applications using JavaScript.
Server-Side Applications: Using Node.js, JavaScript powers scalable backend systems.
Game Development: JavaScript-based engines like Phaser.js help in game creation.
Data Visualization: Libraries like D3.js and Chart.js allow complex data representation.
Artificial Intelligence & Machine Learning: Libraries like TensorFlow.js bring AI capabilities to web applications.
3. Understanding JavaScript Basics
a. Variables and Data Types
JavaScript provides three ways to declare variables:
var
(function-scoped, outdated)let
(block-scoped, preferred for mutable values)const
(block-scoped, used for constants)
let name = "Alice"; // String
const age = 30; // Number
var isActive = true; // Boolean
let user = { id: 1, name: "Alice" }; // Object
Data Types
JavaScript has two main categories of data types:
Primitive:
string
,number
,boolean
,null
,undefined
,symbol
,bigint
Reference:
object
,array
,function
b. Operators
JavaScript supports various operators:
Arithmetic:
+
,-
,*
,/
,%
,++
,--
Comparison:
==
,===
,!=
,!==
,>
,<
,>=
,<=
Logical:
&&
,||
,!
Assignment:
=
,+=
,-=
,*=
,/=
Example:
console.log(5 + "5"); // "55" (string concatenation)
console.log(5 == "5"); // true (loose comparison)
console.log(5 === "5"); // false (strict comparison)
4. Functions and Scope
a. Function Types
- Function Declaration
function greet() {
return "Hello!";
}
- Function Expression
const greet = function() {
return "Hello!";
};
- Arrow Functions (ES6+)
const greet = () => "Hello!";
b. Scope and Hoisting
Scope defines where variables can be accessed.
Global Scope: Accessible anywhere.
Function Scope: Defined inside a function.
Block Scope: Limited to
{}
blocks (let
,const
).
Example:
function example() {
let localVar = "I'm inside the function";
console.log(localVar); // Accessible
}
console.log(localVar); // Error: localVar is not defined
Hoisting allows function and variable declarations to be moved to the top of their scope before execution.
console.log(x); // undefined (hoisted)
var x = 10;
5. Control Flow & Loops
a. Conditional Statements
- if-else
let num = 10;
if (num > 5) {
console.log("Greater than 5");
} else {
console.log("Less or equal to 5");
}
b. Loops
Loops help iterate over data.
- for loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
- while loop
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
6. Asynchronous JavaScript
a. Callbacks
function fetchData(callback) {
setTimeout(() => {
callback("Data received");
}, 2000);
}
fetchData(console.log);
b. Promises
const fetchData = new Promise((resolve, reject) => {
setTimeout(() => resolve("Data received"), 2000);
});
fetchData.then(data => console.log(data));
c. Async/Await
async function getData() {
let response = await fetch("https://api.example.com/data");
let data = await response.json();
console.log(data);
}
7. Best Practices for JavaScript
Always use
const
andlet
overvar
.Use strict equality (
===
) to avoid type coercion issues.Write modular code with reusable functions.
Optimize performance by reducing DOM manipulations.
Use
try...catch
for error handling in async functions.
try {
let response = await fetch("https://api.example.com");
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data", error);
}
Conclusion
Mastering JavaScript fundamentals is the first step toward becoming a proficient developer. By understanding variables, functions, loops, objects, and asynchronous programming, you can build efficient and dynamic applications. JavaScript's broad usability across web, mobile, backend, and AI makes it a valuable skill for any developer. Keep practicing, explore modern frameworks, and stay updated with the latest ECMAScript features!
Happy Coding!
Subscribe to my newsletter
Read articles from Raj Goldar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
