Time Without Surprises: Monotonic Clocks in GO

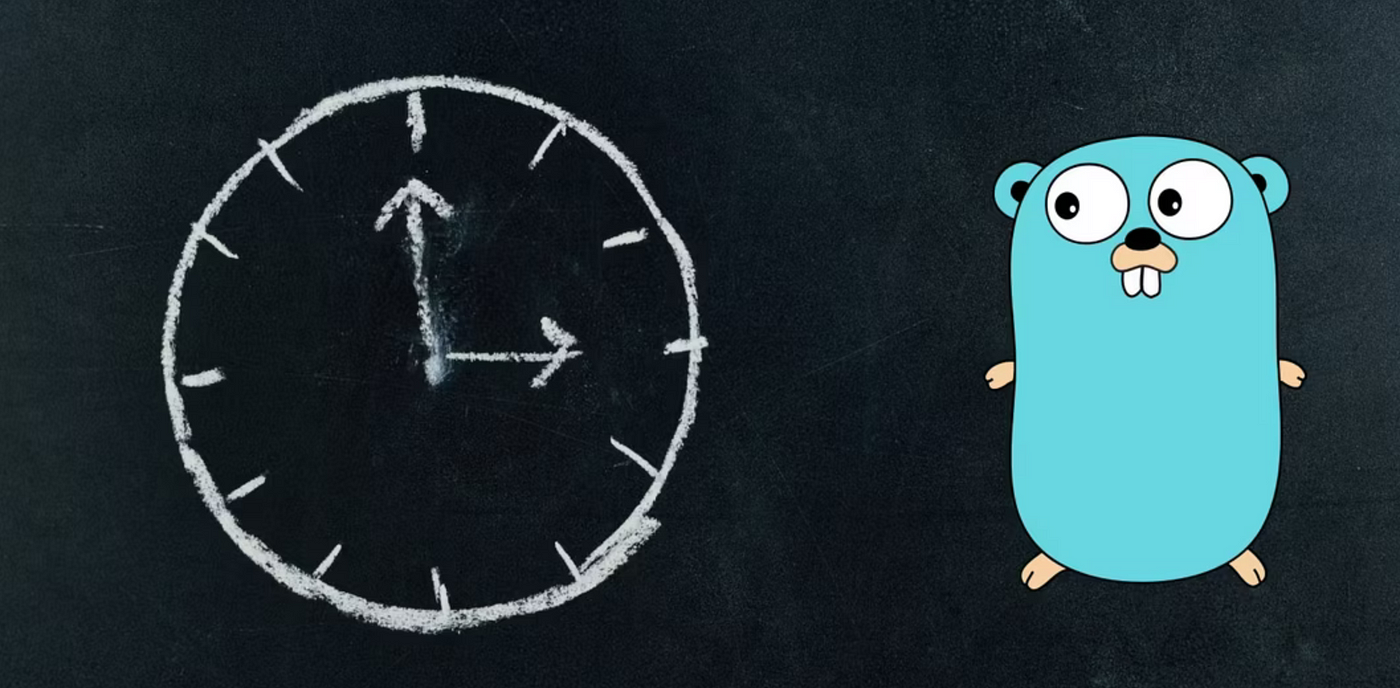
Operating systems keep track of two kinds of time:
Wall clock time: This reflects the current date and time, like what you'd see on a wristwatch.
Monotonic clock time: This increases continuously and steadily from the moment the system boots.
The general rule is:
Wall clocks are used to tell time, and monotonic clocks are used to measure time.
Let’s understand why that distinction is important.
Suppose we want to measure the execution time of a process:
--- t1 ---
... p - takes x minutes ...
--- t2 ---
(t1
and t2
are wall clock time instances, and p
is the process.)
To measure how long p
took, we can do:
elapsed := t2.Sub(t1)
Seems simple, right? But here's the problem:
Wall clocks are subject to external changes — they can jump forward or backward unexpectedly.
This can happen due to:
Daylight Saving Time
Leap Seconds
Network Time Protocol (NTP) syncs
If such a change happens during the execution of p
, the difference t2 - t1
may be inaccurate, resulting in incorrect elapsed time.
How Go Solves This: Monotonic Time
To solve this, Go uses monotonic time under the hood. This support is invisible — you don’t have to worry about it. You get monotonic safety without any extra effort unless you're doing something advanced.
Go reads the system’s monotonic clock and stores it alongside the wall time in every time.Time
value returned by time.Now()
. Based on the use case, it uses the appropriate clock reading when needed.
When you measure time differences (like time.Since(start)
or t2.Sub(t1)
), Go uses the monotonic part if both times have it else these operations fall back to using the wall clock readings.
start := time.Now() // contains both wall and monotonic time
doSomething()
elapsed := time.Since(start) // uses monotonic clock
This avoids issues caused by wall clock jumps.
Why is Monotonic Time "Invisible" ?
Go keeps this support hidden as an internal implementation detail and you cannot directly access the monotonic time field, as the monotonic time by itself has no meaning and is useless for absolute timestamps. Monotonic times also have no scope in marshalling and unmarshalling. That's why Go hides it to avoid confusion.
However, if you want to see the monotonic offset, you can print it:
time.Sleep(1 * time.Second)
fmt.Println(time.Now().String())
Output:
2025-03-29 14:38:37.879037 +0530 IST m=+1.005962418
// The m=+... part is the monotonic time offset.
That said we cannot completely ignore the monotonic time completely when comparing two time.Time
values. Go’s ==
operator compares not just wall time and location, but also the monotonic clock reading (if present).
This means:
t1 := time.Now()
t2 := t1.Round(0) // strips monotonic part
fmt.Println(t1 == t2) // false
fmt.Println(t1.Equal(t2)) // true
Always use
.Equal()
to compare wall times safely — it ignores monotonic differences.
Operations That Strip Monotonic Time
Some operations are pure wall time manipulations and remove the monotonic clock reading. These include:
t.AddDate()
t.Round()
t.Truncate()
t.In()
t.Local()
t.UTC()
If you perform any of these, the resulting time.Time
will no longer have a monotonic reading.
The idiomatic way to strip monotonic time is:
t = t.Round(0)
Subscribe to my newsletter
Read articles from Likhith R Krishna directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
