Upload to S3 bucket with Python
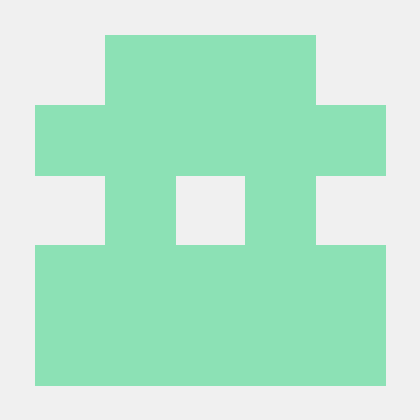
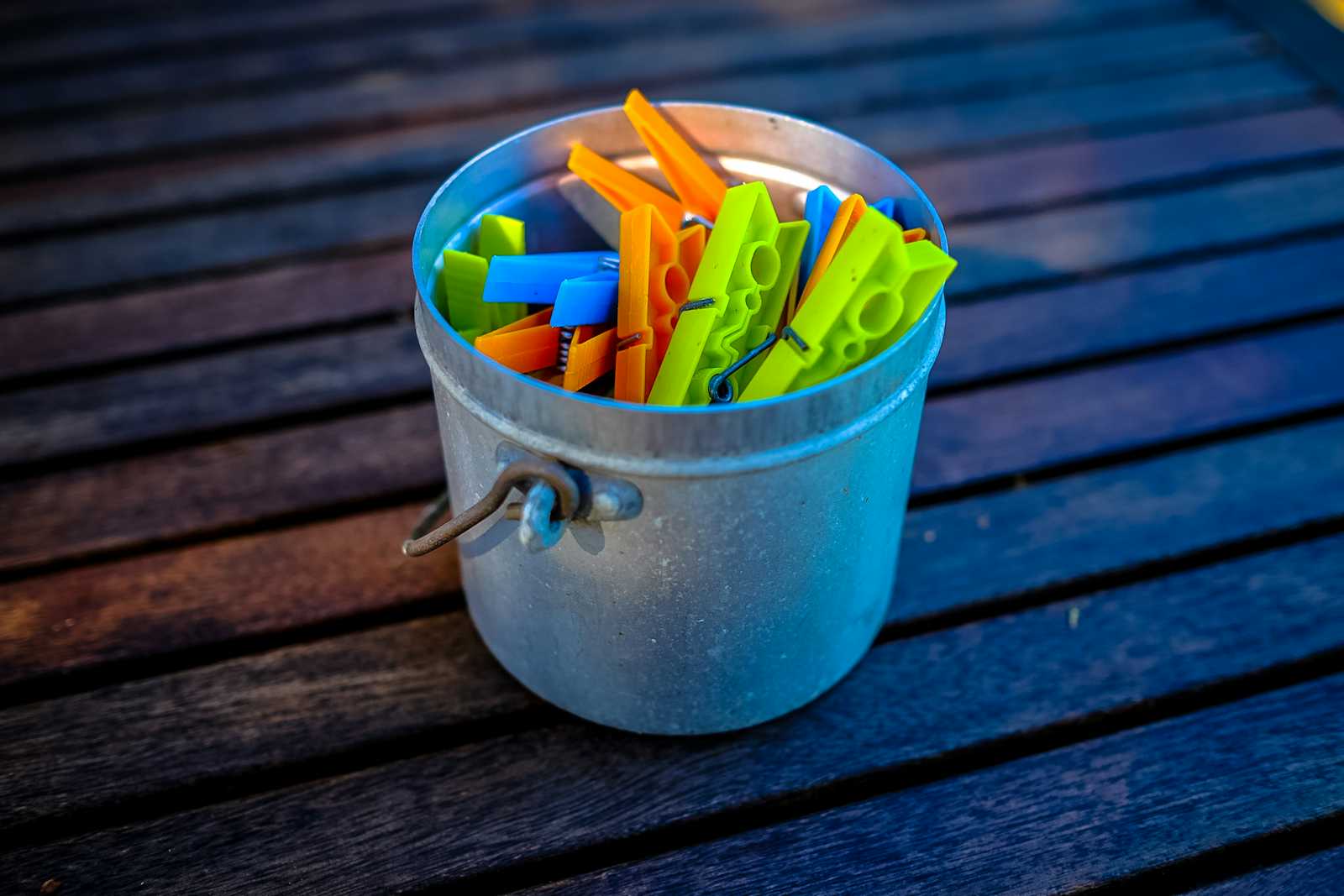
Today, I implemented a simple Python script to upload a file to an S3 bucket.
You can find it here.
https://github.com/jrsokolow/budget_planner/tree/main/s3
What have I learned from this exercise?
The official AWS Python library is called boto3. It is used in the uploader.py script.
The Python library Click (Command Line Interface Creation Kit) allows you to build command-line interfaces. It is used in the cli.py script.
The implemented script assumes that the S3 bucket provided in the cli.py script already exists. However, it is possible to modify the uploader.py script to create the bucket if it is missing. Below, you can find example code to create a missing bucket.
import boto3 from botocore.exceptions import ClientError, NoCredentialsError import os def ensure_bucket_exists(bucket_name, region=None): """Checks if bucket exists; creates it if it doesn't.""" s3 = boto3.client('s3', region_name=region) try: s3.head_bucket(Bucket=bucket_name) print(f"✅ Bucket '{bucket_name}' already exists.") except ClientError as e: error_code = int(e.response['Error']['Code']) if error_code == 404: print(f"ℹ️ Bucket '{bucket_name}' not found. Creating...") create_bucket(bucket_name, region) else: print(f"❌ Could not check bucket: {e}") raise def create_bucket(bucket_name, region=None): """Creates a new S3 bucket in the specified region.""" s3 = boto3.client('s3', region_name=region) try: if region is None or region == 'us-east-1': s3.create_bucket(Bucket=bucket_name) else: s3.create_bucket( Bucket=bucket_name, CreateBucketConfiguration={'LocationConstraint': region} ) print(f"✅ Bucket '{bucket_name}' created.") except ClientError as e: print(f"❌ Failed to create bucket: {e}") raise def upload_file_to_s3(file_name, bucket, object_name=None, region=None): if object_name is None: object_name = file_name # Ensure bucket exists or create it ensure_bucket_exists(bucket, region) s3_client = boto3.client('s3', region_name=region) try: s3_client.upload_file(file_name, bucket, object_name) print(f"✅ File '{file_name}' uploaded to 's3://{bucket}/{object_name}'") except FileNotFoundError: print("❌ File not found.") except NoCredentialsError: print("❌ Missing AWS credentials.") except Exception as e: print(f"❌ Error: {e}")
For me, this is an interesting line.
s3.head_bucket(Bucket=bucket_name)
The
head_bucket
method checks if an S3 bucket exists and if you can access it. If the bucket exists and you have permission, the call succeeds quietly (returns HTTP 200). If something is wrong, the method will return a 404 error if the bucket does not exist. It might also return a 403 error if the user defined in the AWS configuration does not have access to the bucket. Additionally, the method could return a 400 error if there is another issue, like a network problem.As I am not a Python developer, I started to wonder why, when calling the
head_bucket()
method, we pass the bucket name in this form:Bucket=bucket_name
. This is because thehead_bucket
method does not accept positional arguments; instead, it uses named keyword arguments. Specifically,head_bucket()
expects a parameter dictionary like:{ 'Bucket': 'my-bucket-name' }
Uploading a file to an S3 bucket turned out to be a simple task, but at the same time, I discovered a few things I didn't know. So, it was a beneficial exercise.
Subscribe to my newsletter
Read articles from Jakub Sokolowski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
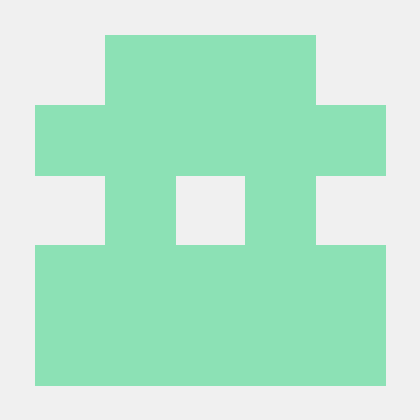