When to use '@Observable' Macro
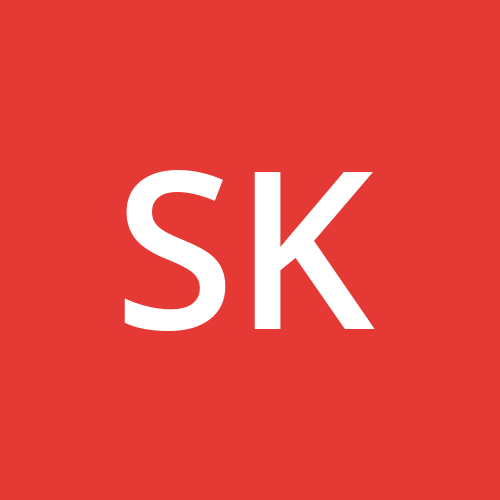
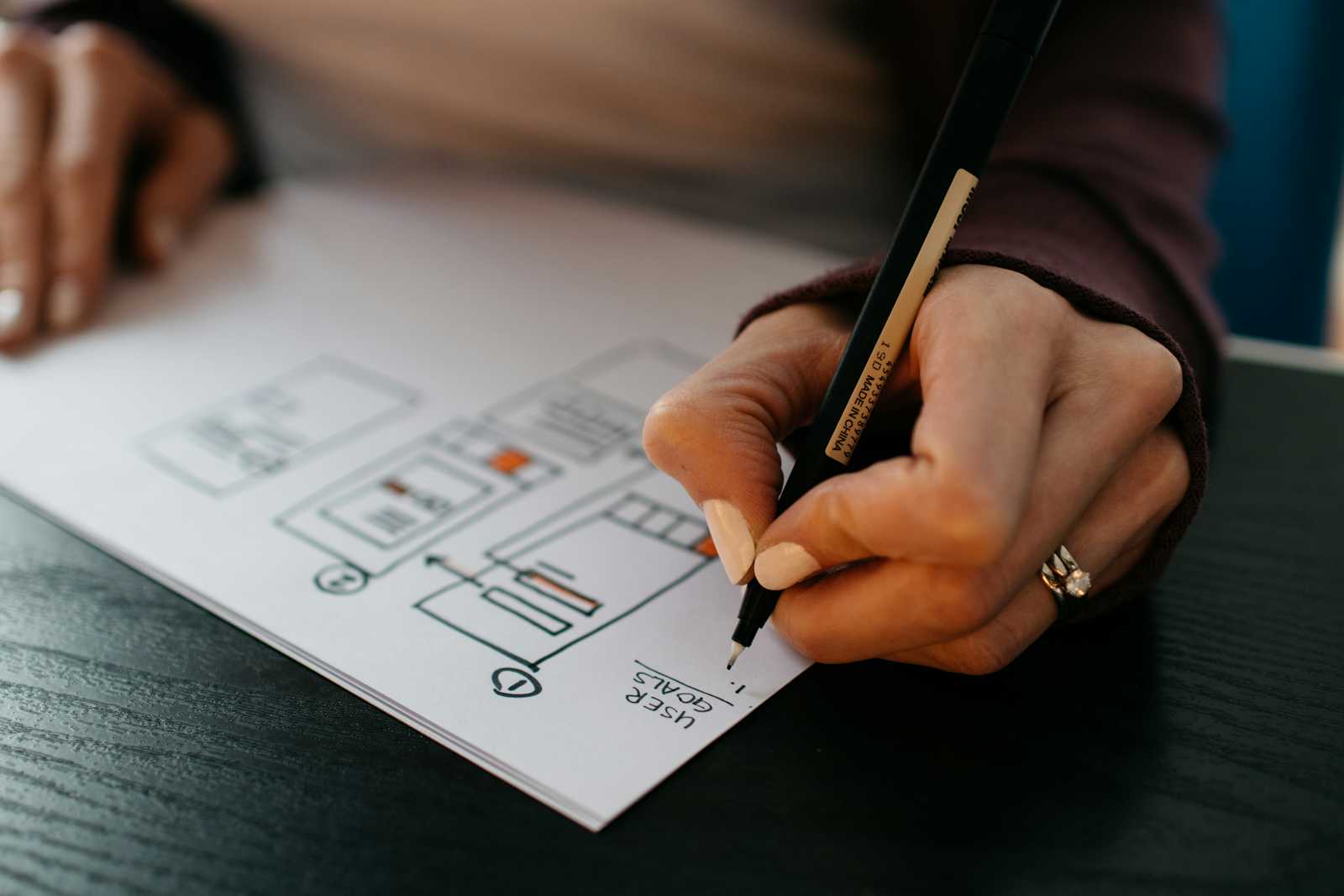
Introduction
In this article, we will discuss how state management works in SwiftUI. We then will discuss macros and how the @Observable
macro comes into play. It will assume some basic Swift and SwiftUI knowledge and is geared towards users who are familiar with the base concepts and are looking for a primer on @Observable
macro.
Prerequisites: Basic working knowledge of Swift, SwiftUI and ObservableObject
After that introduction, we will discuss several aspects of using @Observable
especially how it differs from the ObservableObject
that was the recommended way before @Observable
was introduced. We will discuss the performance benefits, how to migrate existing codebases and how to use this macro properly with protocols and mocking.
State Management in SwiftUI
If you are familiar with state management in modern javascript frameworks or SwiftUI, you can skip this part. If you’re coming from a game dev background like I am, this will be really helpful to understand.
When using SwiftUI, we do not update our screen every frame like we do with game engines. We keep track of all the UI elements and only re-render the UI elements if some aspect of them change.
Let’s say you have a Text
element that displays 0
. And you want to increment it by tapping on a button, you can use a variable to do it. Something similar to the code below. But if you try to compile the code, you get an error.
import SwiftUI
struct MyView : View {
private var counter: Int = 0
var body: some View {
VStack {
Text("Counter: \(counter)")
Button("Increment") {
counter += 1
}
}
}
}
You may have noticed that the variable text
is being changed from within the body
variable of this struct. But structs in Swift are immutable and in the computed body property, you are changing the variable — which is not allowed. And the only way to get this to compile ( and to get the intended results ) is to add the @State
macro to the variable and change that line to
@State private var counter: Int = 0
The @State
macro is very important and powerful because it will track the changes to this variable and will update the UI when it detects any changes to this variable.
But this macro is also limited to value types and is typically only used with simpler types like Int
, String
and Float
etc.
If you want to track changes in a larger object like a ViewModel
in MVVC architecture or a controller
in an MVC architecture, you want to use the @Observable
macro on the class definition.
In the past, it was recommended to conform the class to ObservableObject
for the class definition and use @StateObject
or @ObservedObject
macro at the time of usage ( like the @State
macro ). However this has since been simplified to just one macro and much less boiler plate code to get it up and running.
This macro helps us with reducing boiler plate code, improving performance and writing more clean code.
Understanding Macros in Swift
What are Macros?
Macros are introduced in Swift 5.9 and they enable us to generate code at compile time. They are essentially shortcuts to writing boilerplate code.
If you used SwiftUI in the recent past, you might have noticed something like this
#Preview {
MyView()
}
Here, the #Preview
is a macro that hides the actual implementation. In the past we used to create a struct with a static variable that will show us the preview.
struct MyViewPreview: PreviewProvider {
static var previews: some View {
MyView()
}
}
As you can see the new #Preview
macro is much nicer to program and most of the time, it is all you need. However, it always helps to understand what’s going on with a macro. To understand that, you can right click on the macro and select the Expand Macro
option in Xcode.
The @Observable
macro is one such macro that simplifies a lot of boilerplate code. It is an attached macro, which means that instead of creating new code, they will modify the existing declarations. Lets look at what this macro does.
What does @Observable Macro do?
In the example we used above, you were able to use @State
macro to keep track of the number of times an object was clicked. However, let’s say you want to move that counter to a separate class. Let’s create a ViewModel for that and call it MyViewModel
and lets add a more complicated property in there.
class MyViewModel {
public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter < 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
}
The gist of this class is that for every counter increment, you compute the counterText
property to display in the view.
If you want to use this class to track the counter, you can try using it similar to what you had in the past.
struct MyView : View {
var vm = MyViewModel()
var body: some View {
VStack {
Text("Counter: \(vm.counterText)")
Button("Increment") {
vm.counter += 1
}
}
}
}
This compiles but is not behaving as intended. This is because you do not track the changes in the vm
variable ( MyViewModel
class )
In the past, you had to make a few changes.
Make the
MyViewModel
conform toObservableObject
.Mark the properties you want to track as
@Published
Mark the
vm
definition as eitherStateObject
orObservedObject
This will result in the following code.
import SwiftUI
class MyViewModel : ObservableObject {
@Published public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter <= 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
}
struct MyView : View {
@StateObject var vm = MyViewModel()
var body: some View {
VStack {
Text("Counter: \(vm.counterText) and \(vm.counter) times")
Button("Increment") {
vm.counter += 1
}
}
}
}
As you can see, we added three different stubs for this to work. The computed property will not accept the @Published
tag, but will update when the downstream variables update.
In WWDC 2023, apple unveiled the @Observable
macro that makes this much simpler. We just add the @Observable
tag to the MyViewModel
and let it handle the rest.
So the change then becomes much simpler. Instead of doing the three things ( three is the minimum number of changes since usually you would have more than 1 variable in a class that you want to watch ), we can achieve our intended functionality with one macro.
import SwiftUI
@Observable
class MyViewModel {
public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter <= 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
}
struct MyView : View {
var vm = MyViewModel()
var body: some View {
VStack {
Text("Counter: \(vm.counterText) and \(vm.counter) times")
Button("Increment") {
vm.counter += 1
}
}
}
}
As you can see, this is much simpler and cleaner compared to the old way of doing things. Lets go over the benefits of using @Observable
over ObservedObject
.
Benefits over Observable Object
Simplified Code
This is the really obvious one. But it cannot be overstated how much simpler and cleaner the codebase feels to write and read with these changes.
We no longer have to add @Published
for all our variables that we want to observe ( which can get out of hand )
We do not have to worry about @StateObject
vs @ObservedObject
specifiers for the instance of the object we’re using. It just feels more natural and Swift-y
Performance Gains
A really big advantage of using @Observable
instead of ObservableObject
that sometimes goes under the radar is huge amount of performance gains you get from this simple change alone.
When a @Published
variable changes in a ObservableObject
, the views that depend on this object will get a signal to redraw the view — even if the view does not depend specifically on the @Published
variable that got changed. The @Observable
macro does not do that.
The example below will not redraw the view if you use @Observable
because the unrelatedCount
is not being used in the CounterView
, whereas if you switch to using ObservableObject
, you will get an update. You can read more about it in this really good post @Observable Macro performance increase over ObservableObject
import SwiftUI
@Observable
final class CounterViewModel {
private(set) var count: Int = 0
private(set) var unrelatedCount: Int = 0
func increaseUnrelatedCount() {
unrelatedCount = 1
}
}
struct CounterView: View {
var viewModel = CounterViewModel()
var body: some View {
return VStack {
Text("Count is: \(viewModel.count)")
Button("Increase count", action: {
viewModel.increaseUnrelatedCount()
})
}
.padding()
}
}
As a summary, we get fine-grained observation compared to ObservableObject
. We are no longer observing the entire object as a whole, but each property that we use in the view which always results in a performance increase ( and potentially huge ones depending on the usage )
When to use @Observable
All the time. It’s that simple. @Observable
macro is better for most cases. Unless you explicitly need it for older project compatibility etc, I do not see any reason to use ObservableObject
.
Migrating from Observable Object to @Observable
Let’s talk about migrating old code from ObservableObject
to Observable
.
I did this a lot for our codebase. Thankfully, it’s not too bad — most of the time.
Let’s consider a simple example. Below is the usual counter example class, with one added element.
import SwiftUI
class MyViewModel : ObservableObject {
@Published public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter <= 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
@Published var userName: String = ""
}
struct MyView : View {
@ObservedObject var vm = MyViewModel()
var body: some View {
VStack {
Text("Counter: \(vm.counterText) and \(vm.counter) times")
Button("Increment") {
vm.counter += 1
}
TextField("Enter your User Name", text: $vm.userName)
.padding(.all)
.frame(maxWidth: 200)
}
}
}
To convert it, we
Start with removing the
ObservableObject
protocol and add the@Observable
macro.Remove the
@Published
macros fromMyViewModel
. We no longer need them.We remove the
@ObservedObject
macro used when initializing the ViewModel.Mark the variable
vm
to be @Bindable.
Let us elaborate on the last point here since we discussed the rest earlier. When you are only reading from a variable, you do not need to include @Bindable
, but since we are writing to the userName
variable using a Binding
, we have to mark the variable with the @Bindable
macro.
As a general rule, use @Bindable
if you were using the ObservedObject
as a Binding
to one of your UI elements.
The final code after all the changes mentioned above is,
import SwiftUI
@Observable
class MyViewModel {
public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter <= 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
var userName: String = ""
}
struct MyView : View {
@Bindable var vm = MyViewModel()
var body: some View {
VStack {
Text("Counter: \(vm.counterText) and \(vm.counter) times")
Button("Increment") {
vm.counter += 1
}
TextField("Enter your User Name", text: $vm.userName)
.padding(.all)
.frame(maxWidth: 200)
}
}
}
Using @Observable with protocols for Mocking
I tend to use a lot of protocols with my codebase. Every service I write, I typically have a protocol, a mock implementation and the actual implementation.
For AuthenticationService, I would typically have a AuthenticationServiceProtocol
, a MockAuthenticationService
and an AuthenticationService
.
This makes it easy for me to test these services in isolation using Unit Tests and most importantly Previews in Xcode.
So, when I started using @Observable
macro, the question arose on whether a protocol can be marked @Observable
and how do I mock a protocol marked Observable.
While I eventually decided that a service that uses this protocol method should not contain any data of their own ( that we want to observe ) and should rather modify the data we send to it. But I found some interesting ways to mock an @Observable
protocol and am documenting that here for anyone who might run into this issue.
Here is an example of how you would implement a protocol that is Observable
. We expand on the earlier example.
import Foundation
import Observation
/* We use Observable as a protocol here instead of a macro.
The @Observable is not allowed on Protocols, but we can use the Observable Protocol.
We also use AnyObject since we want this to be a class ( we are modifying the
data in the object )
We also use @MainActor to reflect the changes immediately in the UI.
For the Main implementation, it might make sense to not block the main thread if
you are doing any REST API calls. In that case, Add the Main Actor to the functions as needed.
*/
@MainActor
public protocol NameServiceProtocol : Observable, AnyObject {
var names: [String] { get set }
func addName(name: String)
}
@MainActor @Observable
public class MockNameService : NameServiceProtocol {
init() {
self.names = ["Dummy", "Names"]
}
public var names: [String]
public func addName(name: String) {
names.append(name)
}
}
@Observable @MainActor
public class NameService : NameServiceProtocol {
public var names: [String]
init() {
self.names = [] // Get the data from a database instead.
}
public func addName(name: String) {
names.append(name)
// REST call to add it in the backend etc.
}
}
We use this function in Swift UI as follows.
import SwiftUI
@Observable
class MyViewModel {
public var counter: Int = 0
public var counterText: String {
if counter == 0 {
return "No Clicks"
}else if counter <= 2 {
return "Some Clicks"
}else{
return "A lot of clicks"
}
}
var userName: String = ""
}
struct MyView : View {
@Bindable var vm = MyViewModel()
var nameService: NameServiceProtocol = MockNameService()
var body: some View {
VStack {
Text("Counter: \(vm.counterText) and \(vm.counter) times")
Button("Increment") {
vm.counter += 1
}
.padding()
ForEach(nameService.names, id:\.self) { name in
Text(name)
}
TextField("Enter a Name", text: $vm.userName)
.padding(.all)
.frame(maxWidth: 200)
Button("Add it to the List") {
nameService.addName(name: vm.userName)
vm.userName = ""
}
}
}
}
This will give you a separation where we can use either MockNameService
in previews or NameService
in production to know exactly how it will function and since we use @MainActor
, we will update the UI.
Conclusion
In summary,
Always use
@Observable
instead ofObservableObject
going forward.Consider converting your existing
ObservableObjects
into@Observable
objects if you do not need any old code compatibility. This will greatly reduce boilerplate code and give you some performance benefits you might not have thought of.You can use
@Observable
via inheritance for mocking and previews.
More Reading:
https://developer.apple.com/documentation/observation/observable
https://developer.apple.com/documentation/observation
https://stackoverflow.com/questions/77794933/bindable-usage-with-protocols
https://www.avanderlee.com/swiftui/observable-macro-performance-increase-observableobject/
Subscribe to my newsletter
Read articles from Sravan Karuturi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
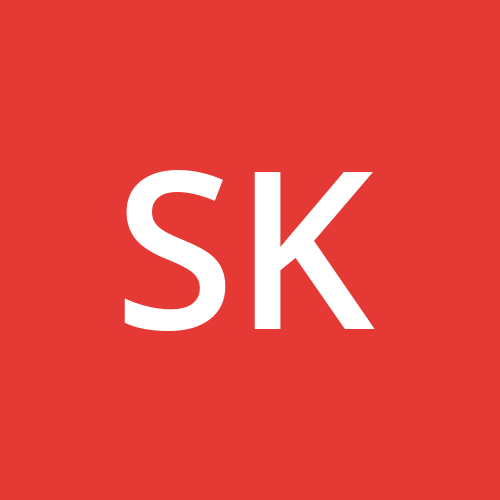