Building an Automated S3 Bucket Security Analyzer in AWS

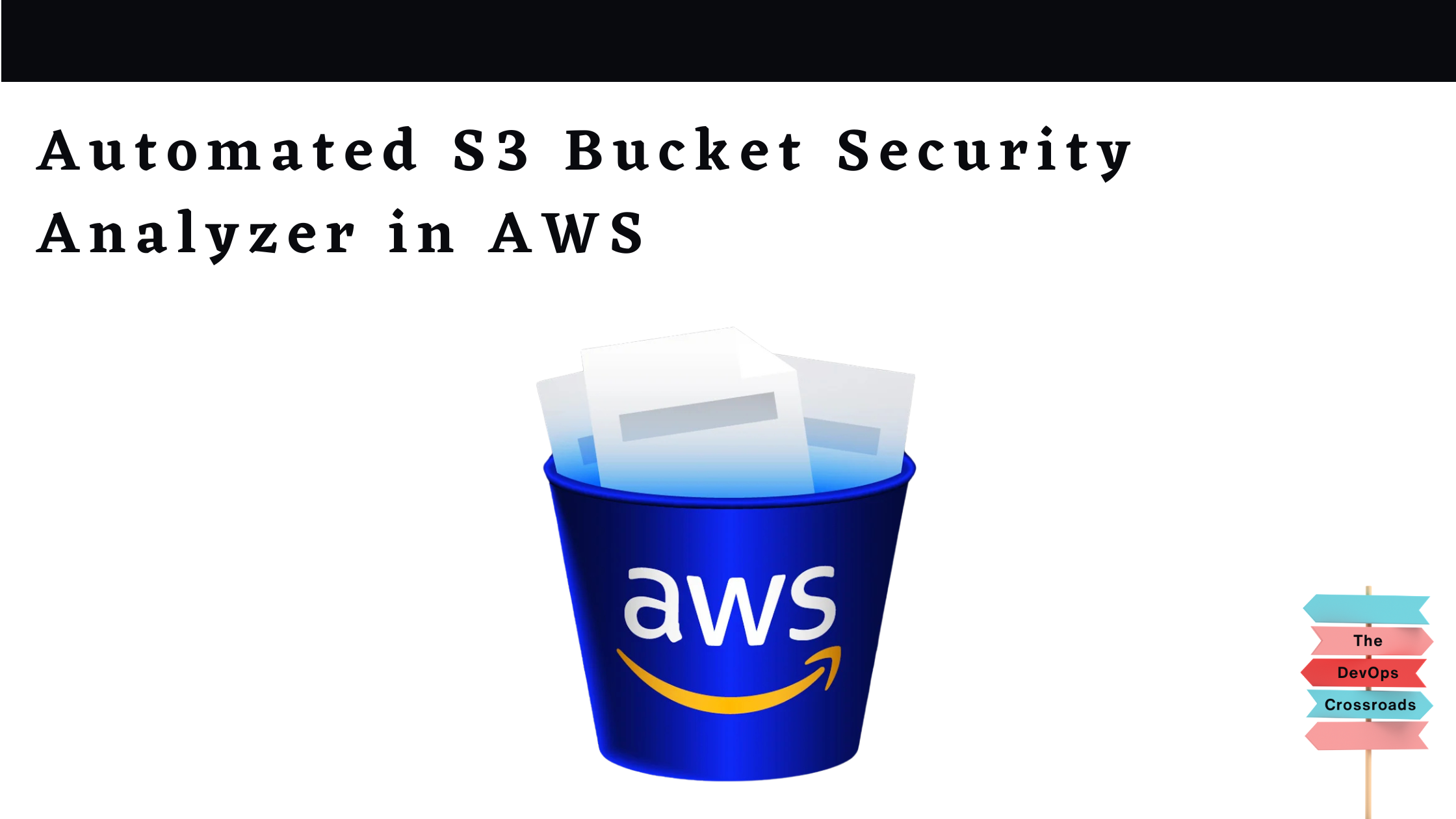
In today's cloud-first world, securing your data is more critical than ever. Amazon S3 buckets are one of the most popular storage solutions in the AWS ecosystem, but they can also be a significant security liability if not properly configured. In this blog post, I'll walk through my recent project: building an automated S3 Bucket Security Analyzer that continuously monitors your AWS environment for vulnerable S3 configurations.
Why S3 Bucket Security Matters
Before diving into the technical implementation, let's understand why S3 bucket security is so important:
Data Breaches from Public Buckets: Countless organizations have suffered data leaks due to misconfigured S3 buckets. Companies like Accenture, Verizon, and the Republican National Committee have all experienced data exposures due to improperly secured S3 buckets.
Compliance Requirements: Regulations like GDPR, HIPAA, and PCI DSS require organizations to protect sensitive data. Misconfigured S3 buckets can lead to non-compliance and significant penalties.
Malware and Ransomware Risks: Attackers can potentially upload malicious content to writeable public buckets, using your infrastructure for malware distribution or as part of larger attack chains.
Data Loss: Without proper versioning and lifecycle policies, accidental deletions or malicious actions can result in permanent data loss.
Cost Implications: Unauthorized access can lead to excessive data transfer costs if attackers exfiltrate large amounts of data.
Common S3 Security Misconfigurations
Through my research and experience, I've identified these common S3 security issues:
1. Public Access Settings
The most notorious S3 security issue is unintended public access. This can happen through:
Bucket ACLs allowing public read or write
Bucket policies with overly permissive permissions
Disabled S3 Block Public Access settings
2. Missing Encryption
Unencrypted data at rest poses compliance and security risks. Problems include:
Default encryption not enabled
Using outdated encryption algorithms
Improper key management
3. Inadequate Logging
Without proper logging, you can't detect unauthorized access or suspicious activities:
Server access logging disabled
No CloudTrail data event logging
No log analysis solution in place
4. Overly Permissive IAM Policies
Even without public access, IAM policies might grant excessive permissions:
Using wildcards (*) in IAM policy resources
Not implementing least privilege principles
Missing conditions to restrict access
5. Missing Lifecycle Management
Poor lifecycle management can create security vulnerabilities:
No versioning to protect against accidental deletion
No transition rules to secure older data
No expiration policies for temporary data
How the S3 Bucket Security Analyzer Works
My solution uses a serverless architecture to continuously monitor S3 buckets for security misconfigurations. Here's how it works:
Architecture Overview
The analyzer consists of:
Lambda Function: Contains the core security scanning logic
CloudWatch Events Rule: Triggers the Lambda function daily
SNS Topic: Delivers notifications when issues are detected
IAM Role: Provides the necessary permissions
The Security Scanning Process
When triggered, the Lambda function:
Retrieves a list of all S3 buckets in the account
For each bucket, it checks:
Public access block configuration
Bucket policy for public access grants
Default encryption settings
Server access logging configuration
Compiles a report of security issues found
Sends a detailed notification via SNS if any issues are detected
Sample Code Highlights
Here's a snippet from the analyzer showing how it checks for public access:
def check_bucket_security(s3_client, bucket_name):
"""
Check bucket for security misconfigurations
"""
issues = []
# Check for public access
try:
public_access_block = s3_client.get_public_access_block(Bucket=bucket_name)
block_config = public_access_block['PublicAccessBlockConfiguration']
if not all([
block_config['BlockPublicAcls'],
block_config['BlockPublicPolicy'],
block_config['IgnorePublicAcls'],
block_config['RestrictPublicBuckets']
]):
issues.append('Public access not fully blocked')
except s3_client.exceptions.NoSuchPublicAccessBlockConfiguration:
issues.append('No public access block configuration')
except Exception as e:
issues.append(f'Error checking public access: {str(e)}')
# Additional security checks...
return issues
Lessons Learned During Implementation
Building this analyzer taught me several valuable lessons:
1. S3 API Limitations and Throttling
When scanning large environments with hundreds of buckets, I encountered API throttling. I had to implement:
Exponential backoff retry logic
Pagination for bucket listing
Parallel processing with proper rate limiting
2. Permission Challenges
Getting the IAM permissions right was tricky. Initially, I granted too many permissions, violating the principle of least privilege. I refined them to:
Only read permissions for S3 configuration (no data access)
Specific SNS publish permissions rather than broader ones
Limited CloudWatch logging permissions
3. Dealing with False Positives
Not all "insecure" configurations are actual security issues. For example:
Some buckets may be intentionally public (hosting static websites)
Custom encryption solutions might not be detected by simple checks
Region-specific requirements needed different validation logic
I addressed this by:
Adding contextual awareness to the checks
Implementing a severity rating system
Supporting resource tagging to exclude specific buckets
4. Notification Fatigue
Daily scans caused notification fatigue when the same issues were repeatedly reported. I improved this by:
Implementing a state tracking mechanism
Only reporting new or changed issues
Adding summary metrics to highlight trends
How to Extend the Analyzer for Additional Checks
The current implementation covers the basics, but there are many ways to enhance it:
1. Advanced Security Checks
Add checks for:
CORS configuration security
Object-level ACL validation
Bucket policy complexity analysis
MFA Delete configuration
Replication settings for critical data
2. Integration with Security Hubs
Extend the analyzer to:
Send findings to AWS Security Hub
Integrate with third-party SIEM solutions
Generate compliance reports for standards like CIS or NIST
3. Auto-Remediation
Implement automatic fixes for common issues:
Enable default encryption
Configure logging
Apply recommended Block Public Access settings
Tag non-compliant resources
4. Comprehensive Visualization
Build a dashboard to:
Track security posture over time
Visualize compliance scores by bucket or team
Generate executive-level security reports
5. Multi-Account Support
Expand to support:
AWS Organizations integration
Centralized reporting across accounts
Custom security policies by account type
Conclusion
Building the S3 Bucket Security Analyzer has been a valuable exercise in implementing practical security automation. By identifying and addressing common S3 security misconfigurations, this tool helps maintain a strong security posture and prevent potential data breaches.
The complete source code, deployment templates, and documentation are available on my GitHub repository. Feel free to use it, contribute, and adapt it to your specific needs.
Remember, security is a continuous process. Tools like this analyzer are just one part of a comprehensive security strategy. Regular reviews, updates to security checks, and keeping up with AWS best practices are equally important.
What security automation tools have you built? I'd love to hear about your experiences in the comments!
Note: This blog post is intended for educational purposes. Always test security tools in a controlled environment before deploying to production.
Subscribe to my newsletter
Read articles from The DevOps Crossroads directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
