Understanding Overflow and Underflow in Java
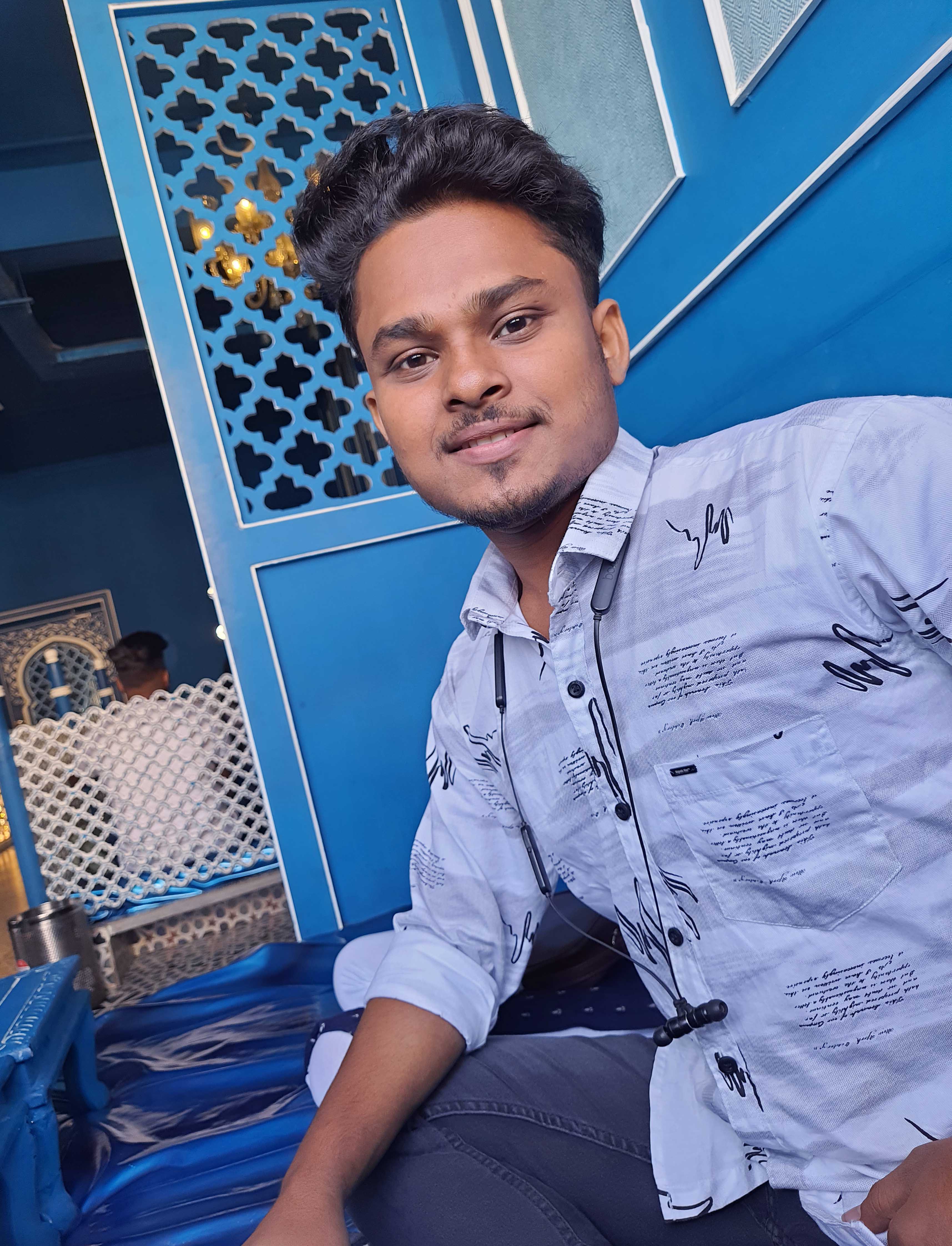
Overflow and Underflow Problem
In programming, overflow and underflow happen when a calculation gives a result that is too big or too small for the type of number you're using. This can cause unexpected and incorrect results.
What Are Overflow and Underflow?
Overflow
Overflow happens when a value exceeds the maximum limit for a data type. In Java, the int
type can hold values from -2,147,483,648
to 2,147,483,647
. Exceeding this range causes the value to "wrap around."
Example:
javaCopyint result = 2147483647 + 1;
System.out.println(result); // Output: -2147483648
Underflow
Underflow occurs when a value is smaller than the minimum limit for a data type. Subtracting below the int
minimum causes the value to wrap around to the maximum value.
Example:
javaCopyint result = -2147483648 - 1;
System.out.println(result); // Output: 2147483647
How to Prevent Overflow and Underflow
1. Use Larger Data Types
If the value could exceed the range of int
, use long
or BigInteger
.
Example:
javaCopylong result = 2147483647L + 1L;
System.out.println(result); // Output: 2147483648
2. Range Checking
Check if the result could exceed the data type limits before performing arithmetic.
Example:
javaCopyint a = 2147483647;
int b = 1;
if (a > Integer.MAX_VALUE - b) {
System.out.println("Overflow will occur");
} else {
int result = a + b;
System.out.println("Safe result: " + result);
}
3. Use Math
Methods
Use addExact()
or similar methods to catch overflow and underflow with exceptions.
Example:
javaCopytry {
int result = Math.addExact(2147483647, 1);
} catch (ArithmeticException e) {
System.out.println("Overflow occurred!");
}
Conclusion
Overflow and underflow can cause unexpected results in Java. To avoid these issues, use larger data types, perform range checks, and utilize Java's Math
methods to catch potential errors.
Subscribe to my newsletter
Read articles from RAJESH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
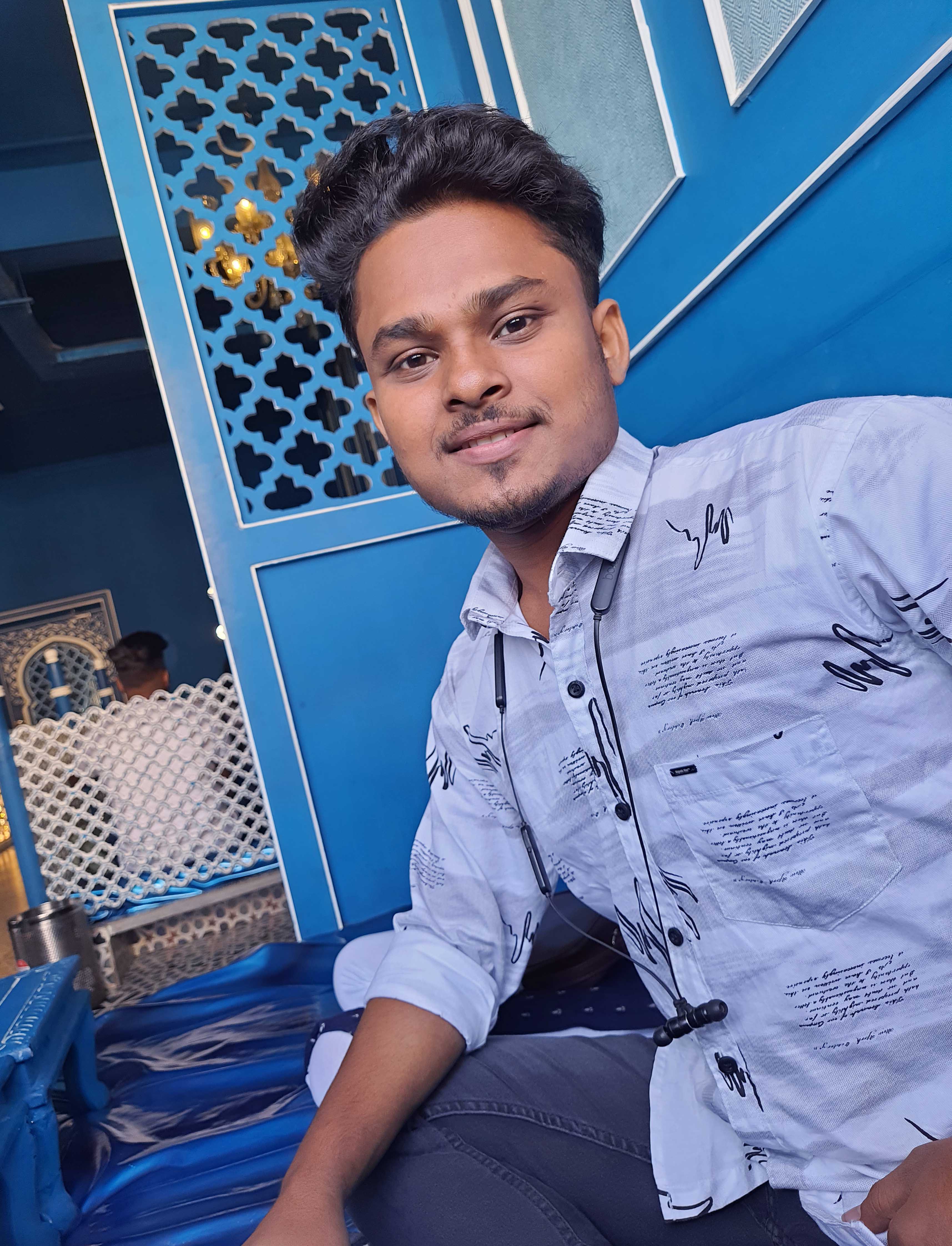