How to Build Scalable Web Applications with ASP.NET

Table of contents
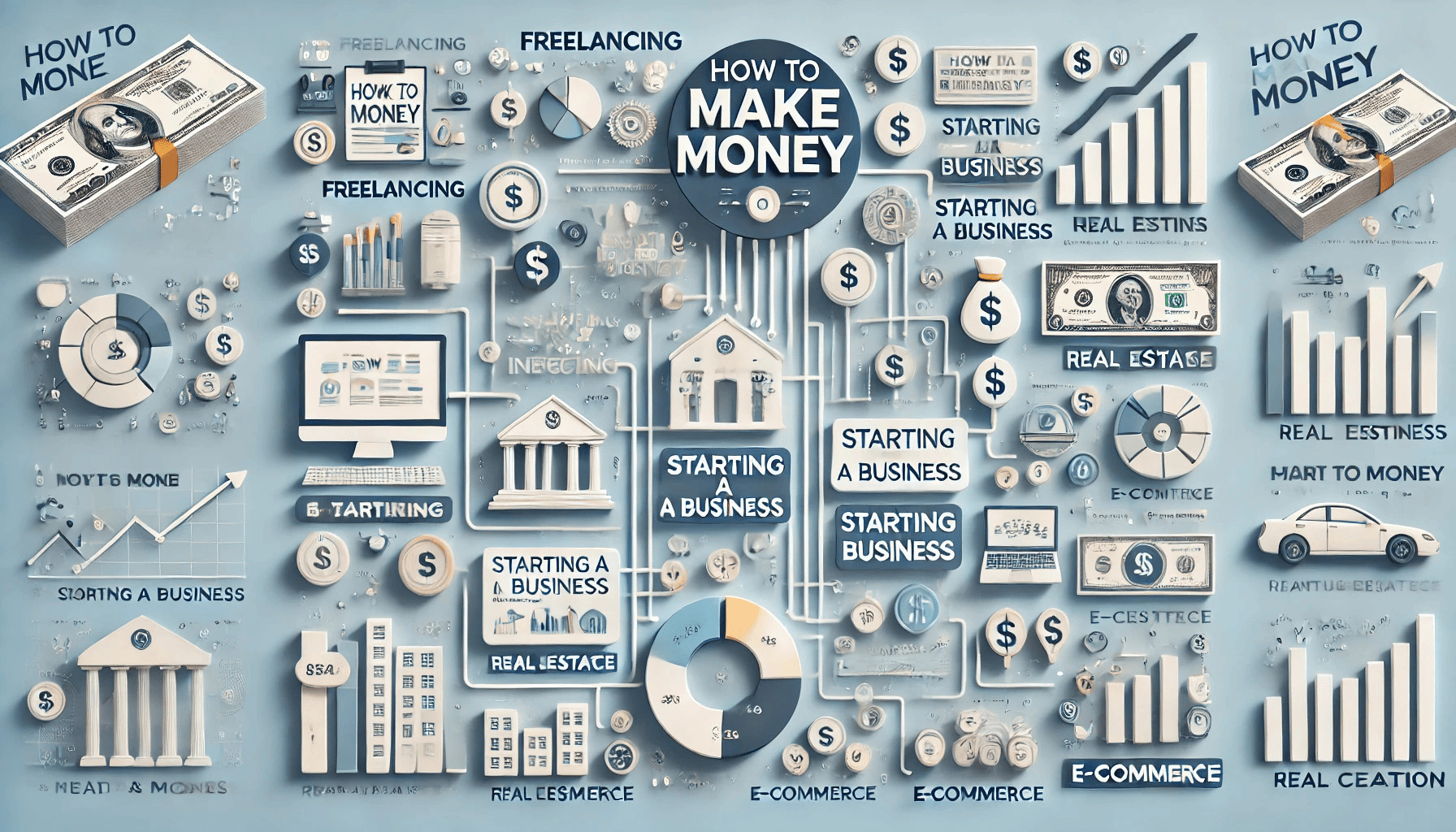
How to Build Scalable Web Applications with ASP.NET
Building scalable web applications is crucial for handling growing user demands while maintaining performance. ASP.NET, Microsoft’s robust web framework, provides powerful tools for creating scalable applications. Whether you're a beginner or an experienced developer, this guide will walk you through best practices for building high-performance, scalable web apps with ASP.NET.
If you're looking to monetize your programming skills, consider joining MillionFormula, a free platform where you can make money online without needing credit or debit cards.
1. Understanding Scalability in Web Applications
Scalability refers to an application's ability to handle increasing workloads efficiently. There are two types of scalability:
Vertical Scaling (Scaling Up): Increasing server resources (CPU, RAM).
Horizontal Scaling (Scaling Out): Adding more servers to distribute the load.
ASP.NET supports both approaches, but horizontal scaling is more sustainable for large applications.
2. Choosing the Right Architecture
A well-structured architecture is key to scalability. Consider these patterns:
a. Microservices Architecture
Instead of a monolithic application, break it into smaller, independent services. ASP.NET Core supports microservices with:
Docker for containerization.
Kubernetes for orchestration.
Example: csharp Copy
// Example of a simple Product Microservice in ASP.NET Core
[ApiController]
[Route("api/products")]
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
[HttpGet]
public async Task<IActionResult> GetAll()
{
var products = await _productService.GetAllProductsAsync();
return Ok(products);
}
}
b. Clean Architecture
Separate concerns into layers (Domain, Application, Infrastructure, Presentation) for better maintainability.
3. Database Optimization
Database bottlenecks are common in scaling. Use these strategies:
a. Caching with Redis
Reduce database load by caching frequently accessed data. csharp Copy
// Using Redis Cache in ASP.NET Core
services.AddStackExchangeRedisCache(options =>
{
options.Configuration = "localhost:6379";
});
// Inject IDistributedCache in your service
public class ProductService
{
private readonly IDistributedCache _cache;
public ProductService(IDistributedCache cache)
{
_cache = cache;
}
public async Task<List<Product>> GetProductsAsync()
{
var cachedProducts = await _cache.GetStringAsync("products");
if (cachedProducts != null)
return JsonSerializer.Deserialize<List<Product>>(cachedProducts);
var products = await _dbContext.Products.ToListAsync();
await _cache.SetStringAsync("products", JsonSerializer.Serialize(products));
return products;
}
}
b. Database Sharding
Split large databases into smaller, faster chunks.
c. Read Replicas
Use read replicas for reporting and analytics to offload the primary database.
4. Asynchronous Programming
Blocking calls slow down applications. Use async/await
for I/O operations: csharp Copy
// Asynchronous Controller Action
[HttpGet("{id}")]
public async Task<IActionResult> GetProduct(int id)
{
var product = await _productService.GetProductByIdAsync(id);
if (product == null) return NotFound();
return Ok(product);
}
5. Load Balancing & CDN Integration
a. Load Balancing with Azure / AWS
Distribute traffic across multiple servers using:
Azure Load Balancer
AWS Elastic Load Balancer (ELB)
b. Content Delivery Network (CDN)
Serve static files (images, CSS, JS) via CDN for faster delivery. csharp Copy
// Configure Static Files with CDN in ASP.NET Core
app.UseStaticFiles(new StaticFileOptions
{
FileProvider = new PhysicalFileProvider(Path.Combine(Directory.GetCurrentDirectory(), "wwwroot")),
RequestPath = "/static",
OnPrepareResponse = ctx =>
{
ctx.Context.Response.Headers.Append("Cache-Control", "public,max-age=31536000");
}
});
6. Monitoring & Performance Tuning
a. Application Insights
Track performance metrics with Azure Application Insights. csharp Copy
// Add Application Insights in Program.cs
builder.Services.AddApplicationInsightsTelemetry();
b. Profiling & Logging
Use Serilog or NLog for structured logging. csharp Copy
// Serilog Configuration
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.WriteTo.File("logs/log.txt", rollingInterval: RollingInterval.Day)
.CreateLogger();
// Inject ILogger in services
public class ProductService
{
private readonly ILogger<ProductService> _logger;
public ProductService(ILogger<ProductService> logger)
{
_logger = logger;
}
public async Task<List<Product>> GetProductsAsync()
{
_logger.LogInformation("Fetching products...");
return await _dbContext.Products.ToListAsync();
}
}
7. Auto-Scaling with Cloud Providers
Azure Auto-Scaling: Adjust server capacity based on demand.
AWS Auto Scaling Groups: Automatically add/remove EC2 instances.
8. Security Considerations
Rate Limiting: Prevent abuse with
Microsoft.AspNetCore.RateLimiting
.SQL Injection Protection: Always use parameterized queries.
HTTPS Enforcement:
csharp
Copy
// Enforce HTTPS in ASP.NET Core
app.UseHttpsRedirection();
Conclusion
Building scalable ASP.NET applications requires a combination of architecture, caching, async programming, and cloud optimizations. By following these best practices, your app can handle millions of users efficiently.
If you're looking to monetize your development skills, check out MillionFormula, a free platform to make money online without needing credit or debit cards.
For further reading:
Happy coding! 🚀
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
