Enhancing AWS Security with IAM Access Analyzer

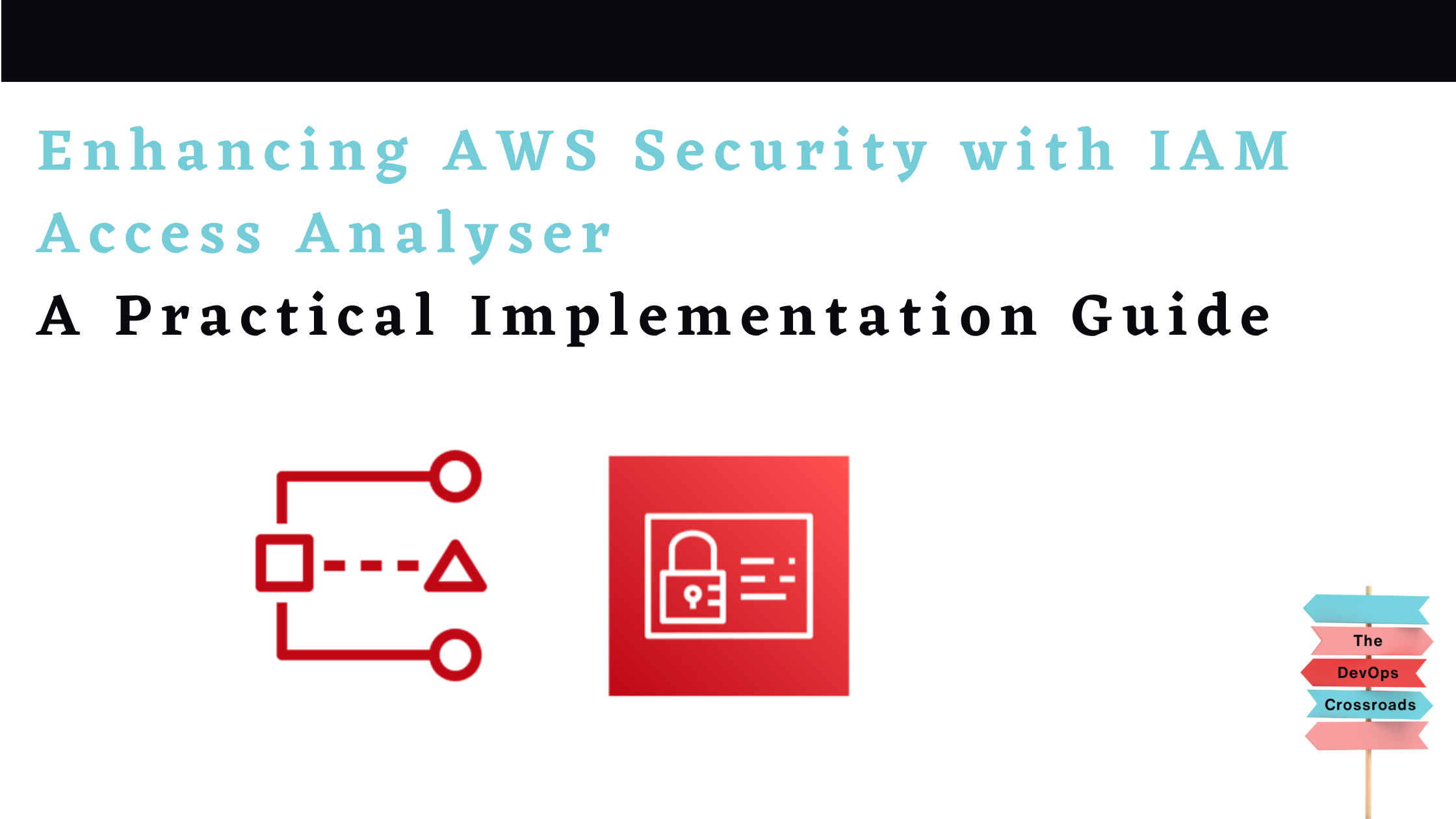
A Practical Implementation Guide
In the ever-evolving landscape of cloud security, managing access to resources is a critical concern. As AWS environments grow in complexity, identifying and addressing overly permissive IAM policies becomes increasingly challenging. This is where AWS IAM Access Analyzer comes to the rescue, providing valuable insights into resource access patterns.
In this post, I'll share my journey implementing AWS IAM Access Analyzer with automated monitoring and remediation as part of my "Cloud Security" project. You'll learn how to set up this powerful security tool using infrastructure as code, visualize findings, and implement automated remediation strategies.
The Challenge: Access Governance at Scale
Many organizations struggle with IAM governance as their AWS footprint expands. Some common challenges include:
Resource sprawl: As the number of S3 buckets, IAM roles, KMS keys, and other resources grows, managing access policies becomes increasingly complex
Cross-account access: Identifying which resources are accessible from outside your account or organization
Least privilege enforcement: Ensuring resources are only accessible to entities that genuinely need access
Compliance requirements: Meeting regulatory standards that require regular access reviews
When external access goes undetected, it can lead to data leakage, compliance violations, and potential security breaches. The challenge is not just detecting these issues but doing so at scale and implementing effective remediation.
The Solution: IAM Access Analyzer with Automated Remediation
AWS IAM Access Analyzer uses logic-based reasoning to analyze resource-based policies. It identifies resources that can be accessed from outside your AWS account or organization, highlighting potential security risks. My implementation consists of four core components:
IAM Access Analyzer: The foundation that identifies overly permissive policies
CloudWatch Dashboard: A custom visualization layer for security findings
AWS Config Rules: Automated detection and remediation for common issues
Lambda Function: Custom remediation logic for complex scenarios
Here's how these components work together:
Visualizing Findings with CloudWatch Dashboard
After deploying IAM Access Analyzer, I set up a CloudWatch dashboard to monitor findings in real-time:
Note: This screenshot shows a freshly deployed dashboard. The "No data available" message is normal for new deployments before findings are generated.
The dashboard includes:
External Access Findings graph (left) tracking the count of findings over time
Findings by Resource Type chart (right) showing findings categorized by AWS resource type
A markdown section explaining the dashboard's purpose
Recent Access Analyzer Findings log section at the bottom
Once findings start being generated, these graphs will populate with data, providing at-a-glance visibility into your security posture.
Setting Up IAM Access Analyzer with Terraform
I've chosen Terraform for this implementation to ensure reproducibility and infrastructure as code best practices. Let's walk through the key components.
1. Deploying the Analyzer
The core analyzer setup is straightforward:
resource "aws_accessanalyzer_analyzer" "analyzer" {
analyzer_name = var.analyzer_name
type = var.analyzer_type # "ACCOUNT" or "ORGANIZATION"
tags = {
Name = var.analyzer_name
Environment = "Production"
Project = "SecurityAvenue"
}
}
For organization-wide analysis, you can set type = "ORGANIZATION"
and deploy in the management account. This allows you to detect access issues across all accounts in your AWS organization.
2. Configuring Notifications
To ensure timely response to findings, I've set up an SNS topic with CloudWatch Events integration:
# SNS Topic for notifications
resource "aws_sns_topic" "analyzer_notifications" {
name = "access-analyzer-findings"
}
# CloudWatch Event Rule for Access Analyzer Findings
resource "aws_cloudwatch_event_rule" "analyzer_findings" {
name = "capture-access-analyzer-findings"
description = "Capture IAM Access Analyzer Findings"
event_pattern = jsonencode({
"source" : ["aws.access-analyzer"],
"detail-type" : ["Access Analyzer Finding"]
})
}
# CloudWatch Event Target for SNS
resource "aws_cloudwatch_event_target" "sns" {
rule = aws_cloudwatch_event_rule.analyzer_findings.name
target_id = "SendToSNS"
arn = aws_sns_topic.analyzer_notifications.arn
}
This configuration ensures that new findings trigger notifications, allowing security teams to respond quickly to critical issues.
Visualizing Findings with CloudWatch Dashboards
Raw security findings can be overwhelming, so I created a CloudWatch dashboard to visualize the data:
resource "aws_cloudwatch_dashboard" "access_analyzer_dashboard" {
dashboard_name = var.dashboard_name
dashboard_body = jsonencode({
widgets = [
{
type = "metric"
x = 0
y = 0
width = 12
height = 6
properties = {
metrics = [
["AWS/AccessAnalyzer", "FindingsCount", "AnalyzerName", var.analyzer_name, "FindingType", "ExternalAccess", { "stat" = "Sum" }]
]
view = "timeSeries"
stacked = false
region = "us-east-1"
title = "External Access Findings"
period = 86400
}
},
# Additional widgets...
]
})
}
The dashboard provides:
A count of findings by resource type
Trend analysis of findings over time
Recent findings with detailed information
Status breakdown of active vs. resolved findings
This visualization turns raw data into actionable insights, making it easier to prioritize remediation efforts.
Implementing Automated Remediation
Detecting security issues is only half the battle. Remediating them efficiently is equally important. I implemented two levels of remediation:
1. AWS Config Rules
First, I set up AWS Config rules with automated remediation actions:
# Config Rule for S3 Public Access
resource "aws_config_config_rule" "s3_bucket_public_access" {
name = "s3-bucket-public-access-prohibited"
description = "Checks if Amazon S3 buckets have public access enabled"
source {
owner = "AWS"
source_identifier = "S3_BUCKET_PUBLIC_READ_PROHIBITED"
}
}
# Remediation for S3 public access
resource "aws_config_remediation_configuration" "s3_remediation" {
config_rule_name = aws_config_config_rule.s3_bucket_public_access.name
target_type = "SSM_DOCUMENT"
target_id = "AWS-DisableS3BucketPublicReadWrite"
parameter {
name = "AutomationAssumeRole"
value = aws_iam_role.remediation_role.arn
resource_value = "RESOURCE_ID"
}
parameter {
name = "S3BucketName"
resource_value = "RESOURCE_ID"
}
automatic = true
maximum_automatic_attempts = 3
retry_attempt_seconds = 60
}
This configuration automatically blocks public access to S3 buckets when detected.
2. Custom Lambda Remediation
For more complex scenarios, I developed a Lambda function that:
Analyzes finding details
Applies appropriate remediation based on resource type
Archives the finding once resolved
Here's a simplified example of the Lambda code for remediating S3 buckets:
def remediate_s3_bucket(bucket_arn, finding):
bucket_name = bucket_arn.split(':')[-1]
logger.info(f"Remediating S3 bucket: {bucket_name}")
try:
# Block public access
s3.put_public_access_block(
Bucket=bucket_name,
PublicAccessBlockConfiguration={
'BlockPublicAcls': True,
'IgnorePublicAcls': True,
'BlockPublicPolicy': True,
'RestrictPublicBuckets': True
}
)
logger.info(f"Successfully blocked public access for bucket {bucket_name}")
# Archive the finding after remediation
access_analyzer.update_findings(
analyzerArn=finding['analyzerId'],
ids=[finding['id']],
status='ARCHIVED'
)
logger.info(f"Successfully archived finding {finding['id']}")
except Exception as e:
logger.error(f"Error remediating S3 bucket {bucket_name}: {str(e)}")
raise
The full Lambda function handles multiple resource types and applies appropriate remediation strategies for each.
Key Lessons Learned
Through this implementation, I gained several valuable insights:
Start with Monitoring: Before implementing automated remediation, run IAM Access Analyzer in monitoring-only mode to understand your environment's access patterns.
Risk Assessment: Not all findings require the same level of attention. External access to a logging bucket might be acceptable, while external access to a customer data bucket requires immediate remediation.
Test Remediation Actions: Automated remediation can break legitimate access patterns if not carefully tested. Always validate remediation actions in a non-production environment first.
Layered Approach: Combine automated and manual remediation. Automate for common, well-understood issues, and manually review more complex findings.
Continuous Improvement: Access patterns evolve over time. Regularly review and update your findings archive rules and remediation logic.
Results and Ongoing Benefits
After implementing this solution, I observed:
Reduced Security Exposure: Clear visibility into resources accessible from outside the account
Faster Remediation: Automated remediation of common issues without manual intervention
Improved Compliance: Better adherence to least-privilege principles
Centralized Monitoring: Single dashboard for access-related security findings
The most significant benefit has been the shift from reactive to proactive security governance. Rather than discovering access issues during security incidents or audits, we now identify and address them continuously.
Getting Started With Your Own Implementation
Ready to implement IAM Access Analyzer in your environment? Here's a simplified approach:
Start Small: Deploy the analyzer in a single account before rolling out organization-wide
Monitor First: Run in monitoring mode for a few weeks to understand your findings
Prioritize Remediation: Address critical findings first, such as public S3 buckets
Gradually Automate: Add automated remediation incrementally, starting with well-understood patterns
Document Exceptions: Create a process for documenting legitimate external access
Conclusion
AWS IAM Access Analyzer is a powerful tool for improving your security posture. By combining it with CloudWatch dashboards and automated remediation, you can build a robust security governance framework that continuously monitors and improves your AWS environment.
The complete code for this implementation is available in my GitHub repository as part of the "Security Avenue" collection. I encourage you to check out the implementation guide, adapt it to your environment, and share your experiences.
What security tools are you using to manage access in your cloud environment? Have you implemented IAM Access Analyzer? I'd love to hear about your experiences in the comments!
Are you interested in more cloud security content? Follow me for more practical implementations and security best practices.
Subscribe to my newsletter
Read articles from The DevOps Crossroads directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
