Inference & Array

2 min read
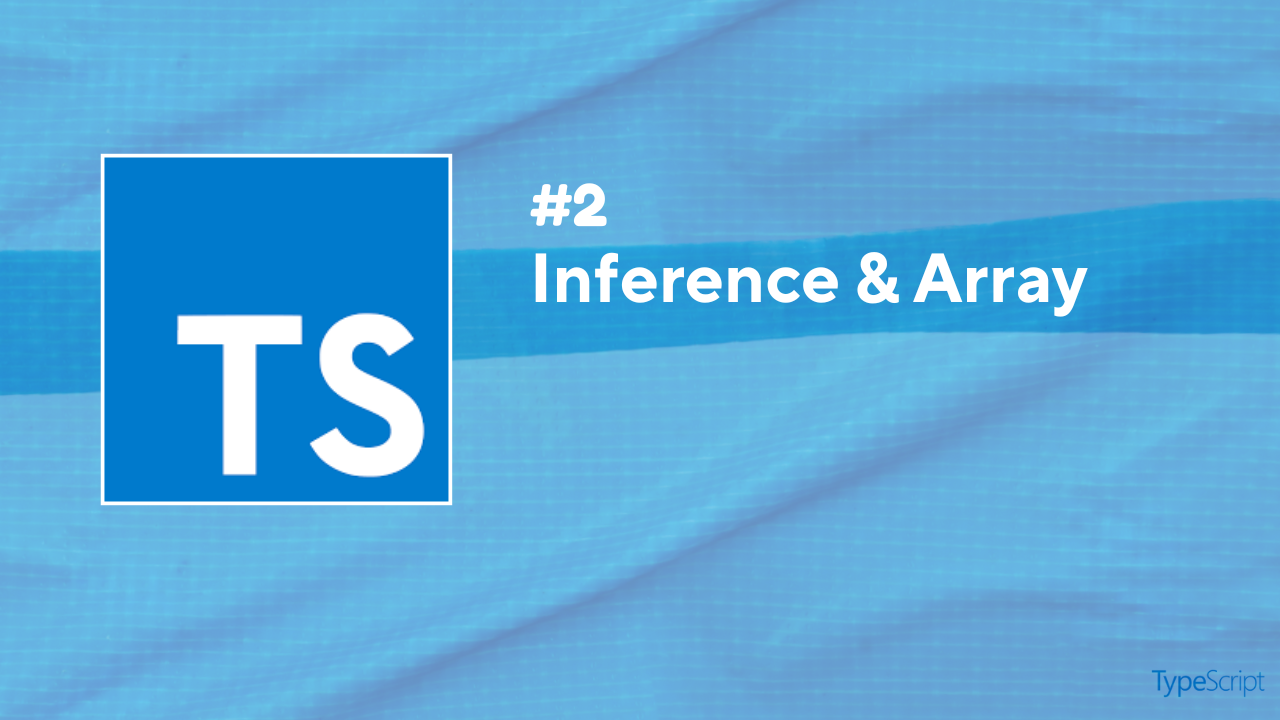
Type Inference in TypeScript
TypeScript can automatically determine variable types based on their initial values. This is called Type Inference.
→ Explicit Typing (Manually Specified Type)
let age: number = 25; // Type is explicitly set
→ Implicit Typing (Type Inferred Automatically)
let city = "Mumbai"; // TypeScript infers `string`
let isStudent = false; // TypeScript infers `boolean`
If you try to assign a different type later, TypeScript will show an error:
city = 123; // ❌ Error: Type 'number' is not assignable to type 'string'
→ any
Type (Disables Type Checking)
If you don't specify a type and TypeScript cannot infer it, it assigns any
.
let data: any = "Hello";
data = 123; // No error (not recommended)
Using any
removes type safety, so it's best to avoid it.
Arrays in TypeScript
Arrays in TypeScript can store multiple values of the same type.
Defining an Array
let numbers: number[] = [1, 2, 3, 4, 5];
let names: string[] = ["Alice", "Bob", "Charlie"];
Accessing and Modifying Elements
console.log(numbers[0]); // Output: 1
numbers.push(6); // Adds 6 to the array
console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
Using Array<T>
Syntax (Alternative)
let scores: Array<number> = [90, 80, 70];
Arrays ensure type safety, preventing unintended data types from being added. ✅
In TypeScript, the readonly
modifier must be used directly with an array declaration.
const colors: ReadonlyArray<string> = ["red", "green", "blue"]; // ✅ Correct
0
Subscribe to my newsletter
Read articles from Snehal Pradhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Snehal Pradhan
Snehal Pradhan
Just a fellow student !!