How to Standardize Code Style Across Teams: Linting & Formatting in JavaScript, Angular, and Node.js

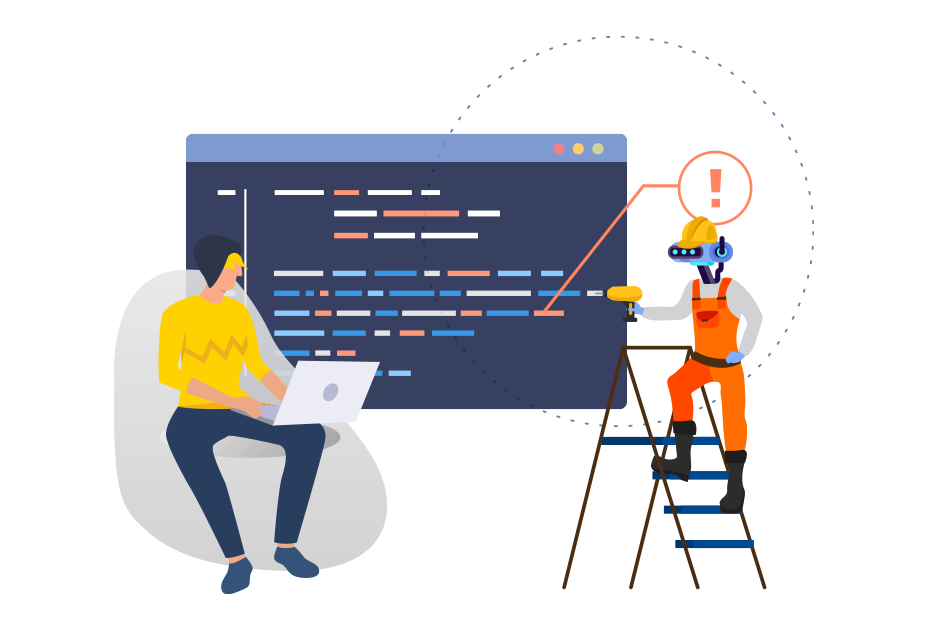
Maintaining a consistent code style across a development team is crucial for readability, maintainability, and collaboration. In JavaScript, Angular, and Node.js projects, enforcing a standardized coding style ensures that all developers follow the same conventions, reducing bugs and improving efficiency. This guide will walk you through the best practices for linting and formatting your JavaScript, Angular, and Node.js codebase.
Why Standardizing Code Style is Important
Improves Readability: A consistent style makes it easier to read and understand the code.
Reduces Merge Conflicts: When everyone follows the same formatting, unnecessary diffs in version control are minimized.
Enhances Maintainability: Future developers can easily pick up and modify the code without confusion.
Boosts Development Speed: Developers spend less time debating code styles and more time building features.
Setting Up Linting in JavaScript, Angular, and Node.js Projects
Linting helps catch syntax errors, enforce coding rules, and maintain consistency.
1. Install ESLint
ESLint is the most widely used linter for JavaScript and TypeScript projects. To set it up:
npm install eslint --save-dev
npx eslint --init
Follow the prompts to configure ESLint for your project.
2. Configure ESLint Rules
Modify the .eslintrc.json
file to enforce rules suitable for your team. A sample configuration:
{
"extends": ["eslint:recommended", "plugin:@typescript-eslint/recommended"],
"parserOptions": {
"ecmaVersion": 2020,
"sourceType": "module"
},
"rules": {
"indent": ["error", 2],
"quotes": ["error", "single"],
"semi": ["error", "always"],
"no-unused-vars": "warn"
}
}
3. Linting in Angular Projects
For Angular projects, use @angular-eslint
:
npm install --save-dev @angular-eslint/eslint-plugin @angular-eslint/eslint-plugin-template
Modify angular.json
to integrate ESLint:
"lint": {
"builder": "@angular-eslint/builder:lint",
"options": {
"lintFilePatterns": ["src/**/*.ts"]
}
}
Run the linter using:
npx eslint . --fix
4. Linting in Node.js Projects
For Node.js applications, ESLint can also be configured with Node-specific rules.
Install Node.js ESLint Plugin
npm install --save-dev eslint-plugin-node
Configure ESLint for Node.js
Modify .eslintrc.json
to include Node.js rules:
{
"extends": ["eslint:recommended", "plugin:node/recommended"],
"env": {
"node": true,
"es6": true
},
"rules": {
"node/no-unsupported-features/es-syntax": "off",
"node/no-missing-import": "off"
}
}
Run the Node.js linter:
npx eslint . --fix
Setting Up Code Formatting with Prettier
Prettier is an opinionated code formatter that works well with ESLint.
1. Install Prettier
npm install --save-dev prettier
2. Create a Prettier Configuration
Create a .prettierrc
file with the following content:
{
"singleQuote": true,
"semi": true,
"tabWidth": 2,
"trailingComma": "es5"
}
3. Integrate Prettier with ESLint
To avoid conflicts between ESLint and Prettier, install the ESLint Prettier plugin:
npm install --save-dev eslint-config-prettier eslint-plugin-prettier
Update .eslintrc.json
:
{
"extends": ["eslint:recommended", "plugin:prettier/recommended"]
}
Run Prettier:
npx prettier --write .
Automating Linting & Formatting with Git Hooks
Use Husky to run linters and formatters before committing code.
1. Install Husky and Lint-Staged
npm install --save-dev husky lint-staged
2. Configure Husky
npx husky install
npx husky add .husky/pre-commit "npx lint-staged"
3. Set Up Lint-Staged
Add this to package.json
:
"lint-staged": {
"*.{js,ts,html,scss}": [
"prettier --write",
"eslint --fix"
]
}
Enforcing Linting Checks in Branching Before Merging or Pushing Code
To ensure that code is properly linted before being pushed or merged, configure Git hooks and CI pipelines.
1. Prevent Pushes with Lint Errors
Modify .husky/pre-push
to enforce linting before code is pushed:
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npx eslint .
npx prettier --check .
2. Enforce Linting in Pull Requests with CI
Use GitHub Actions, GitLab CI, or Jenkins to check linting on pull requests.
Example GitHub Actions Workflow
Create .github/workflows/lint.yml
:
name: Lint Code
on: [pull_request]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Install Dependencies
run: npm install
- name: Run ESLint
run: npx eslint .
- name: Run Prettier Check
run: npx prettier --check .
This ensures that pull requests cannot be merged if linting errors exist.
Conclusion
Standardizing code style across JavaScript, Angular, and Node.js projects enhances collaboration and code quality. By setting up ESLint, Prettier, Git hooks, and CI pipelines, you ensure that every team member writes clean, consistent code. Implement these tools and best practices in your projects to improve productivity and maintainability.
Happy Coding!
Subscribe to my newsletter
Read articles from Raj Goldar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
