Understanding Java's Memory Model: Heap, Stack, and Generational GC
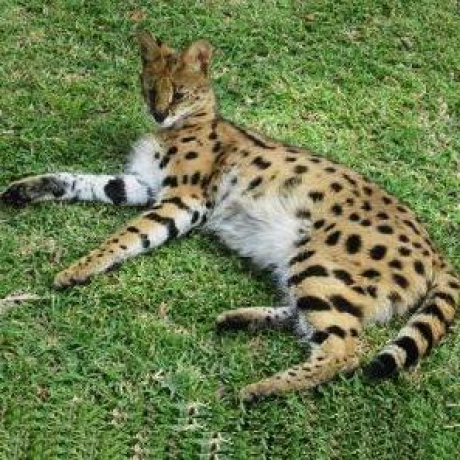
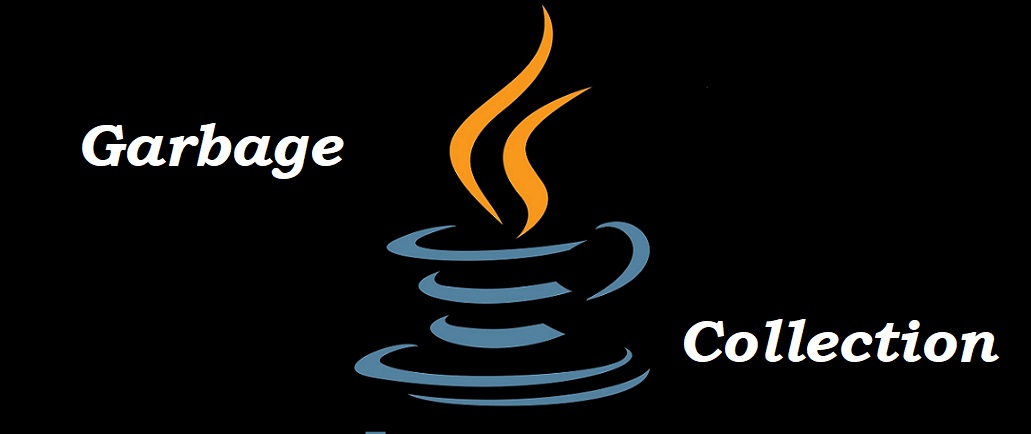
Efficient garbage collection (GC) has a significant effect on performance, so it’s important to know how it works. To understand GC, we need to first look at the underlying memory model.
This article discusses the components of the Java runtime memory, and how they interact with Java garbage collection.
JVM Runtime Memory
Runtime memory changed dramatically in Java 8. This article focuses on Java 8 and later versions.
Essentially, runtime memory is classified as:
Heap: An area shared between all classes, which is used for storing objects;
Non-heap: All other memory areas.
The diagram below illustrates the structure of the JVM runtime memory.
Fig: JVM Runtime Memory
Let’s briefly look first at non-heap memory, before taking a closer look at the heap.
Non-heap areas include:
Metaspace: Holds classes and interfaces, the runtime constant pool and byte code for each method;
Code Cache: Holds instructions compiled into machine code for ‘hot’ areas of the program;
Stack Space: For each running thread, the stack space has frames containing context for all active (uncompleted) methods.
Now let’s take a closer look at the heap, and the reason it’s split into generations.
The developers of the GC algorithms reasoned that many objects in Java are short-lived. Local variables within methods, for example, are used only for the duration of the method. It makes sense, therefore, to hold all newly-created objects in a smaller area, which is cleaned frequently.
Objects that survive garbage collection are likely to be needed for a while, and they’re promoted to the larger OG space.
Since the OG is bigger, it takes longer to clean, and it’s cleaned less frequently. This is known as generational GC.
Referring to the diagram above, you’ll see that in fact the YG is further subdivided into smaller spaces. The heap spaces are:
Young Generation: Divided into:
Eden Space: All new objects are created in this small space;
S1 and S2: These are used alternately to hold objects from Eden that survive GC.
- Old (Tenured) Generation: This holds all objects that have reached a certain age, known as the tenuring threshold.
Generational GC
GC cycles that clean only the YG are known as Minor GC Events, and occur frequently. Major GC events occur less frequently, and also work on OG and Metaspace. The GC doesn’t clean the stack space, since memory is automatically freed when a frame is popped from the stack.
GC events therefore work like this:
Identify objects that have no references pointing to them;
Mark them for finalization, and add them to a finalization queue;
Promote suitable remaining objects to the next space if applicable;
Sweep finalized objects from memory;
Compact the space.
Some of these tasks can be carried out in parallel with other running threads, while others must pause application operations, resulting in pauses. The duration of these pauses is known as latency, and the events that require them are known as stop-the-world events.
Newer GC algorithms reduce latency by:
Dividing each space into equal-sized regions for the purposes of GC. Only one space is cleaned at a time, resulting in fast cycles;
Maintaining a record of the percentage ‘live’ vs GC-eligible objects in each space. Spaces with a high percentage of garbage are cleaned first.
Each application is obviously different to any other, and it’s important to tailor the size of each heap space to the unique requirements of the task.
For more detailed information on this topic, and links to tuning guides, you may like to read this article on Java Garbage Collection.
Monitoring GC and Memory Usage
We should always monitor critical applications regularly, since memory usage and GC efficiency significantly affect performance.
The JDK provides useful monitoring tools such as jconsole
, jstat
and jvisualvm
. For fast and efficient ongoing analysis, with useful graphs and charts as well as performance tuning recommendations, these tools are worth looking at:
GCeasy: Quickly analyzes GC logs, identifies problems and recommends improvements;
HeapHero: Analyzes heap usage, identifies problem areas such as memory leaks, and gives tuning recommendations.
Conclusion
A good knowledge of both the JVM memory model and of GC is essential for troubleshooting and performance tuning.
If you understand GC concepts and terminology, it's not difficult to use JVM options to make your applications more efficient.
Subscribe to my newsletter
Read articles from Jill Thornhill directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
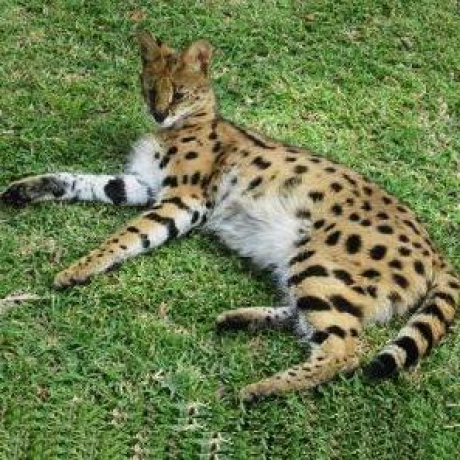