Unlock Go Polymorphism: A Guide to Mastering Interfaces
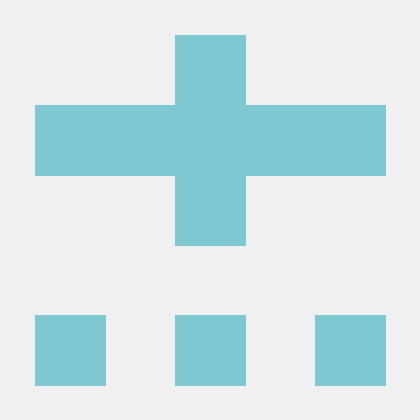
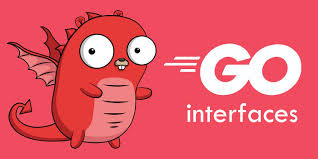
Introduction
Hey there, fellow Gophers! If you’re diving into Go and wondering how to make your code more flexible and reusable, you’ve probably come across interfaces. These little gems help you write cleaner, more modular code without the rigid structures of traditional object-oriented programming. And the best part? Go makes working with interfaces super easy thanks to its implicit implementation.
In this blog, we’ll break down what interfaces are, why they’re awesome, and how you can use them effectively in your Go projects—without getting bogged down in unnecessary complexity.
What are Interfaces in Go?
An interface in Go is basically a contract that defines a set of method signatures. Any type that implements these methods automatically satisfies the interface—no need for explicit declarations like in Java or C++.
Defining an Interface
Let’s check out a simple example:
package main
import "fmt"
// Define an interface
type Speaker interface {
Speak() string
}
// Implementing the interface
type Dog struct{}
func (d Dog) Speak() string {
return "Woof!"
}
type Cat struct{}
func (c Cat) Speak() string {
return "Meow!"
}
func main() {
var s Speaker
s = Dog{}
fmt.Println(s.Speak()) // Output: Woof!
s = Cat{}
fmt.Println(s.Speak()) // Output: Meow!
}
In this example:
Speaker
is an interface with a single method,Speak()
.Both
Dog
andCat
implementSpeaker
by defining theSpeak()
method.Thanks to polymorphism, we can assign different types of
s
and callSpeak()
without worrying about the underlying type.
No implements
Keyword? No Problem!
Unlike other languages, Go doesn’t require explicit declarations to implement an interface. If a type has the require methods it automatically satisfies the interface—no extra boilerplate needed.
Why Use Interfaces?
Here are some real-world benefits of using interfaces in Go:
Decoupling - Your code becomes more modular and easier to maintain.
Testability - You can swap out implementations easily, making unit testing a breeze.
Flexibility - Different types can behave the same way without being part of a rigid class hierarchy.
Practical Use Cases of Interfaces
Dependency Injection
Interfaces let you inject dependencies into functions instead of hardcoding them. This makes your code cleaner and more adaptable.
package main
import "fmt"
type Logger interface {
Log(message string)
}
type ConsoleLogger struct{}
func (c ConsoleLogger) Log(message string) {
fmt.Println("Log:", message)
}
func ProcessData(logger Logger) {
logger.Log("Processing data...")
}
func main() {
logger := ConsoleLogger{}
ProcessData(logger)
}
Mocking for Testing
Testing becomes way easier with interfaces. You can swap out real implementation for mock ones.
type MockLogger struct{}
func (m MockLogger) Log(message string) {
fmt.Println("Mock Log:", message)
}
Interface Composition
Go lets you combine multiple interfaces into a single one, keeping things modular.
type Writer interface {
Write(data string)
}
type Reader interface {
Read() string
}
type ReadWriter interface {
Writer
Reader
}
This means any type that implements both Write()
and Read()
will automatically satisfy ReadWriter
—neat right?
The Empty Interface (interface{}
)
Go has a special empty interface (interface{}
) that can hold any type. It’s useful but can sometimes lead to type assertions headaches.
func PrintValue(value interface{}) {
fmt.Println(value)
}
Use it wisely, and don’t overdo it, or you might lose the benefits of type safety.
Wrapping up!!
Interfaces in Go are a game-changer when it comes to writing flexible, maintainable code. With implicit implementation, dependency injection, and easy testing, they help you keep things clean and modular without unnecessary complexity.
So, are you using interfaces in your Go projects? Have any cool tricks or real-world examples? Drop a comment below—I’d love to hear about it!🚀
Subscribe to my newsletter
Read articles from Anand Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
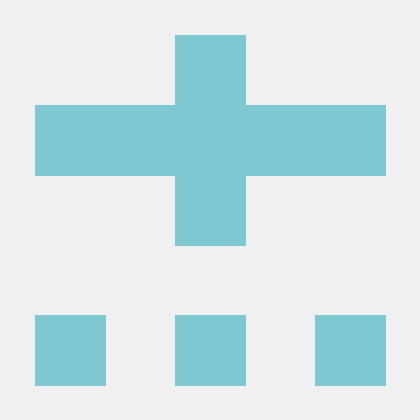
Anand Kumar
Anand Kumar
"Passionate about the art of storytelling and the logic of coding, I find joy in exploring new worlds through books and building innovative solutions through programming. Always eager to learn and grow, I blend creativity and technology to craft a unique narrative in everything I do. When I'm not lost in a novel, you'll find me debugging code or exploring the latest in tech."