Getting Started with Node.js - The URLPattern API
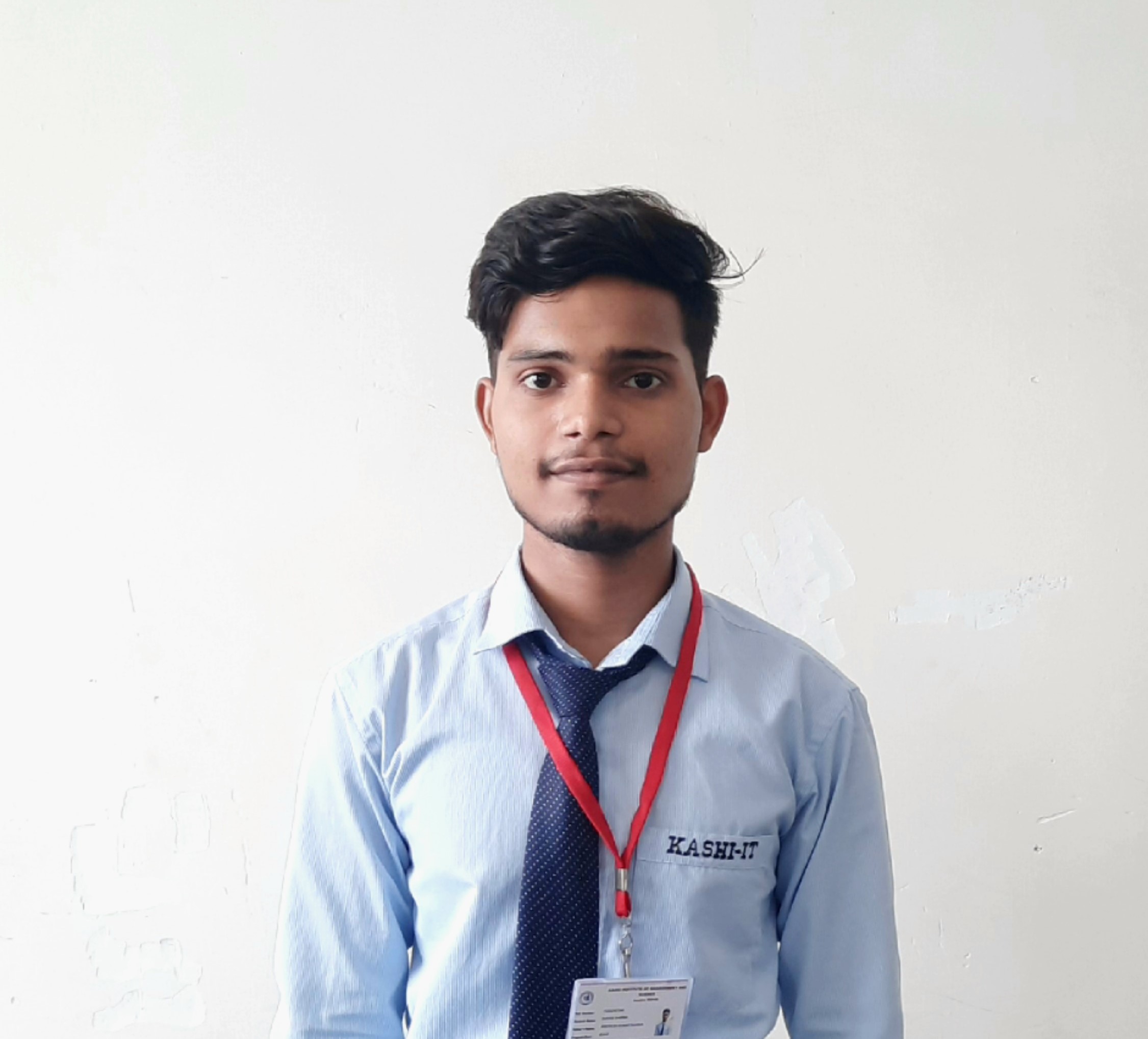
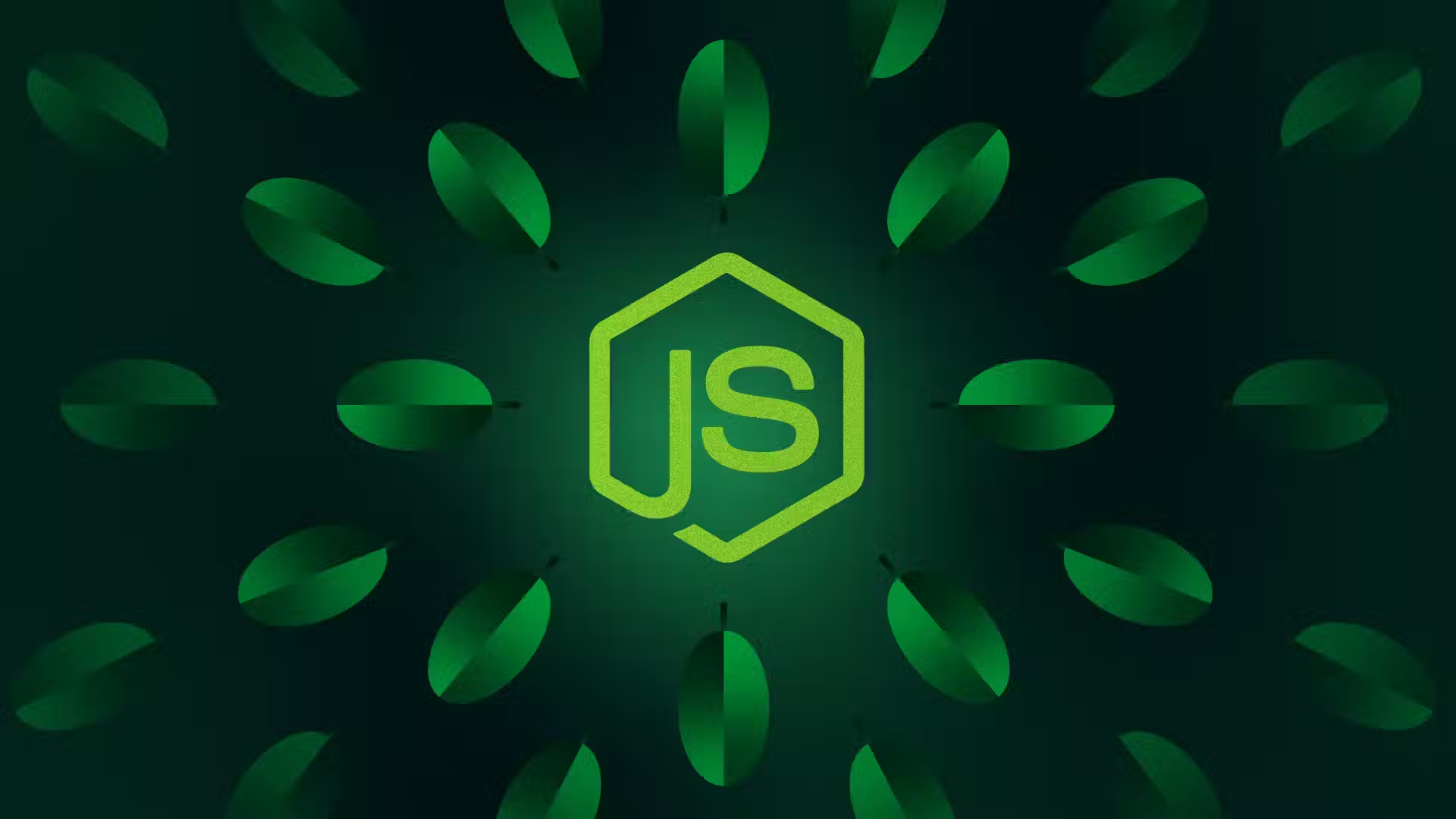
What is URLPattern?
URLPattern is a standard published by the WHATWG (Web Hypertext Application Technology Working Group) which provides a pattern-matching system for URLs. This specification is available at urlpattern.spec.whatwg.org.
URLPattern is part of the WinterTC Minimum Common API, a soon-to-be standardized subset of web platform APIs designed to ensure interoperability across JavaScript runtimes, particularly for server-side and non-browser environments, and includes other APIs such as URL and URLSearchParams.
The URLPattern API provides an interface to match URLs or parts of URLs against a pattern.
The following example demonstrates how URLPattern is used by creating a pattern that matches URLs with a /blog/:year/:month/:slug
path structure, then tests if one specific URL string matches this pattern, and extracts the named parameters from a second URL using the exec method.The URLPattern
constructor is exported from the node:url
module and will be available as a global in Node.js 24.
import { URLPattern } from 'node:url'
const pattern = new URLPattern({
pathname: '/blog/:year/:month/:slug'
});
if (pattern.test('https://example.com/blog/2025/03/urlpattern-launch')) {
console.log('Match found!');
}
const result = pattern.exec('https://example.com/blog/2025/03/urlpattern-launch');
console.log(result.pathname.groups.year); // "2025"
console.log(result.pathname.groups.month); // "03"
console.log(result.pathname.groups.slug); // "urlpattern-launch"
The URLPattern constructor accepts pattern strings or objects defining patterns for individual URL components. The test()
method returns a boolean indicating if a URL simply matches the pattern. The exec()
method provides detailed match results including captured groups. Behind this simple API, there’s sophisticated machinery working behind the scenes:
When a URLPattern is used, it internally breaks down a URL, matching it against eight distinct components: protocol, username, password, hostname, port, pathname, search, and hash. This component-based approach gives the developer control over which parts of a URL to match.
Upon creation of the instance, URLPattern parses your input patterns for each component and compiles them internally into eight specialized regular expressions (one for each component type). This compilation step happens just once when you create an URLPattern object, optimizing subsequent matching operations.
During a match operation (whether using
test()
orexec()
), these regular expressions are used to determine if the input matches the given properties. Thetest()
method tells you if there’s a match, whileexec()
provides detailed information about what was matched, including any named capture groups from your pattern.
Subscribe to my newsletter
Read articles from Shivam Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
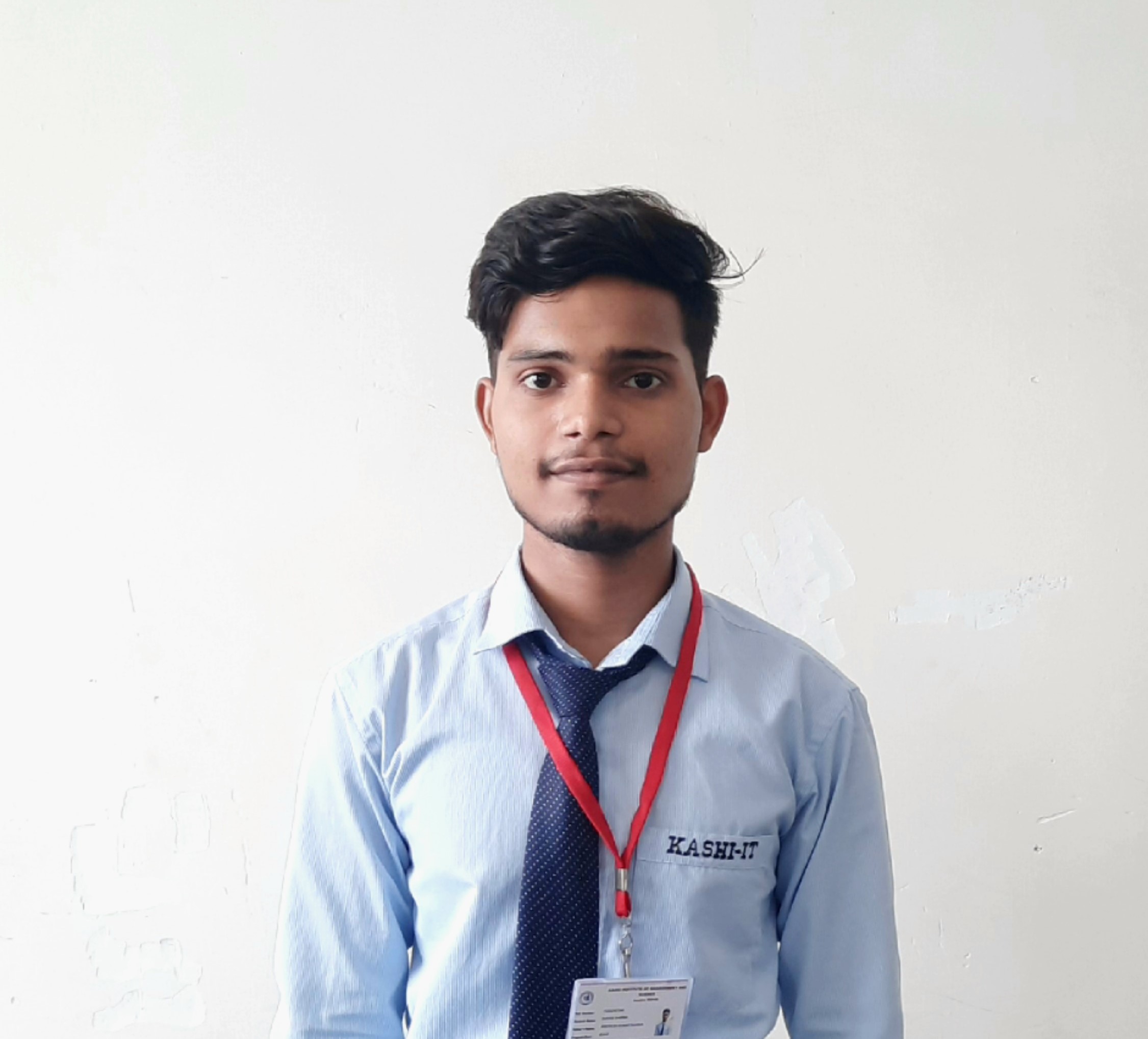
Shivam Sharma
Shivam Sharma
I’m an Open-Source enthusiast & Final year pursuing my Bachelors in Computer Science. I am passionate about Kubernetes, Web Development, DevOps & I enjoy learning new things.