Mastering JavaScript Array Functions: A Developer’s Guide
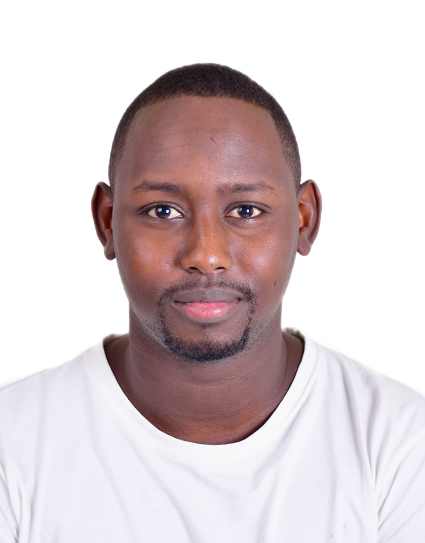
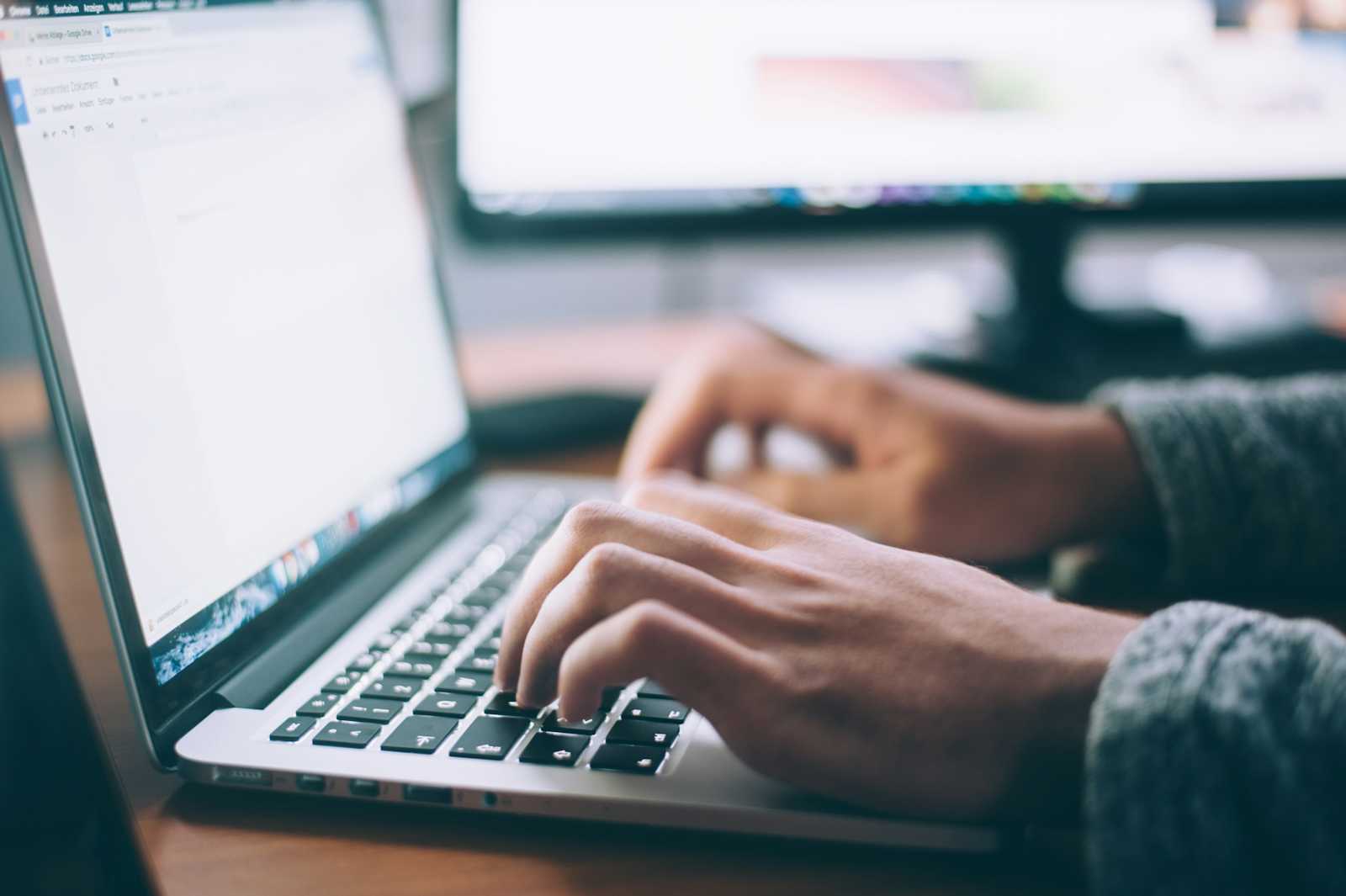
1. Introduction
JavaScript Arrays are a foundational data structure, widely used in front-end and back-end development. But beyond storing values, arrays in JavaScript come equipped with powerful built-in methods that enable elegant, functional-style operations on data.
In this article, we'll dive into must-know JavaScript array functions, how they work, and when to use them. Whether you're filtering API data, transforming collections, or optimizing code readability, mastering these methods will level up your JavaScript skills.
2. Commonly Used Array Functions
2.1 forEach()
Iterates through each element in an array, executing a callback.
const fruits = ['apple', 'banana', 'cherry'];
fruits.forEach(fruit => console.log(fruit));
Note: forEach()
does not return a new array, and you can’t break out of it early.
2.2 map()
Transforms each element in an array and returns a new array.
const numbers = [1, 2, 3];
const doubled = numbers.map(num => num * 2); // [2, 4, 6]
Great for transforming data (e.g., mapping IDs to objects or formatting strings).
2.3 filter()
Returns a new array with elements that pass a test.
const numbers = [1, 2, 3, 4];
const evens = numbers.filter(n => n % 2 === 0); // [2, 4]
Use filter()
to extract subsets of data (e.g., valid users, failed responses).
2.4 find()
Returns the first element that satisfies the condition.
const users = [
{ id: 1, name: 'Alex' },
{ id: 2, name: 'Jordan' }
];
const user = users.find(u => u.id === 2); // { id: 2, name: 'Jordan' }
Returns undefined if nothing matches. Use carefully with optional chaining.
2.5 reduce()
Reduces an array to a single value (sum, object, accumulator).
const nums = [1, 2, 3, 4];
const sum = nums.reduce((acc, val) => acc + val, 0); // 10
More advanced use – grouping values:
const data = ['apple', 'banana', 'apple'];
const count = data.reduce((acc, fruit) => {
acc[fruit] = (acc[fruit] || 0) + 1;
return acc;
}, {});
// { apple: 2, banana: 1 }
Powerful for aggregating data from arrays.
2.6 some() and every()
Check if some or all elements pass a condition.
const nums = [1, 3, 5];
nums.some(n => n % 2 === 0); // false
nums.every(n => n < 10); // true
Use some()
for validation checks like "at least one field is filled".
2.7 includes()
Checks if an array contains a specific value.
const tags = ['js', 'node', 'react'];
tags.includes('node'); // true
Great for simple membership checks. Case-sensitive.
2.8 sort()
Sorts elements in place.
const scores = [10, 1, 5]; scores.sort(); // [1, 10, 5] ❌ wrong order scores.sort((a, b) => a - b); // [1, 5, 10] ✅
Always pass a comparator for numeric or custom sorting.
2.9 flat()
Flattens nested arrays up to a specified depth.
const nested = [1, [2, [3, 4]]];
nested.flat(2); // [1, 2, 3, 4]
Useful for handling nested API response data or matrix-like structures.
2.10 flatMap()
Maps and flattens in a single operation.’
const phrases = ['hello world', 'hi there'];
const words = phrases.flatMap(str => str.split(' '));
// ['hello', 'world', 'hi', 'there']
Optimized alternative to map().flat()
.
3. Choosing the Right Function
Goal | Use |
Transform values | map() |
Filter items | filter() |
Aggregate to single value | reduce() |
Check membership | includes() |
Find a match | find() |
Side effects (logging, DOM ops) | forEach() |
Flatten arrays | flat() , flatMap() |
Conditional checks | some() , every() |
4. Real-World Use Case: API Response Transformation
Imagine receiving user data from an API:
const apiResponse = [
{ id: 1, active: true, role: 'admin' },
{ id: 2, active: false, role: 'user' },
{ id: 3, active: true, role: 'user' }
];
Get list of active user IDs:
const activeUserIds = apiResponse
.filter(user => user.active)
.map(user => user.id);
// [1, 3]
Group users by role:
const grouped = apiResponse.reduce((acc, user) => {
acc[user.role] = acc[user.role] || [];
acc[user.role].push(user);
return acc;
}, {});
// {
// admin: [{ id: 1, active: true, role: 'admin' }],
// user: [...]
// }
5. Conclusion
JavaScript array functions are more than just helpers—they’re fundamental to writing clean, expressive, and high-performance code. Whether you're transforming API responses or building reactive UIs, these methods let you manipulate data efficiently and declaratively.
✨ Key Takeaways:
Use
map()
,filter()
, andreduce()
as your core trio.Always understand if a method mutates the original array.
Combine functions (chaining) for expressive transformations.
Subscribe to my newsletter
Read articles from Muhire Josué directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
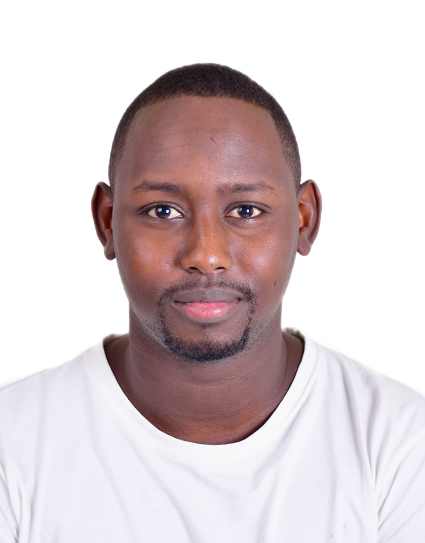
Muhire Josué
Muhire Josué
I am a backend developer, interested in writing about backend engineering, DevOps and tooling.