Transforming Python Scripts into Interactive Data Apps with Preswald

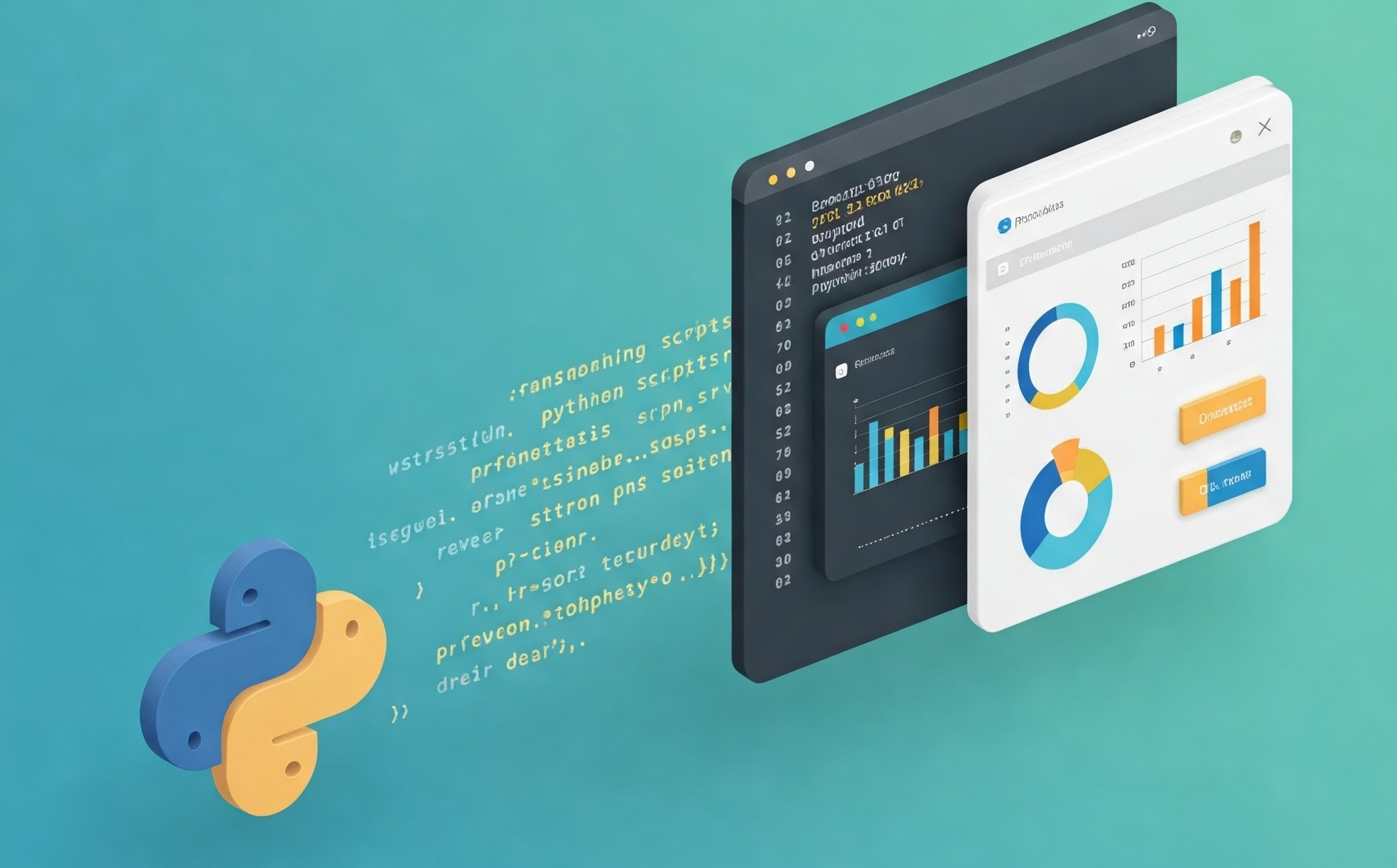
Building and deploying data apps used to mean juggling a mishmash of tools — front-end frameworks, complex back-end setups, and endless boilerplate code. Today, Preswald is changing the game by allowing you to turn your Python scripts into fully interactive apps, dashboards, and internal tools — without writing a single line of frontend code.
What is Preswald?
Preswald is an open-source framework designed specifically for data engineers, analysts, and developers who want to create production-ready data applications fast. It takes your standard Python scripts and, with minimal adjustments, transforms them into interactive web apps that you can run locally or deploy to the cloud in just one command.
Some of its key benefits include:
Code-First Simplicity: Write your logic in Python and let Preswald handle the user interface.
Pre-Built UI Components: Add tables, charts, text inputs, and buttons without having to learn HTML, CSS, or JavaScript.
Stateful Execution: Thanks to its DAG-based execution model, only the parts of your code that change are re-run, making your apps both efficient and predictable.
Instant Deployment: With a single command, your app can go live and be shared with colleagues or clients instantly.
Why Preswald?
Traditional dashboards often require multiple layers of configuration and a steep learning curve for those not well-versed in frontend development. Preswald bridges that gap by offering:
Rapid Prototyping: Quickly build interactive dashboards to explore data in real time.
Seamless Collaboration: Share a simple URL with stakeholders who can interact with your app without any local setup.
Reduced Overhead: Focus on data analysis and insights instead of the intricacies of web development.
In a world where speed and simplicity are key, Preswald empowers teams to iterate faster and communicate insights more effectively.
Getting Started: A Quick Tutorial
Let’s dive into how you can build your first Preswald app. We’ll cover installation, project initialization, writing a simple app, and running it locally.
Step 1: Install Preswald
Install Preswald via pip:
pip install preswald
Step 2: Initialize a New Project
Create a new project directory and get the initial scaffold set up:
preswald init my_project
cd my_project
This command creates essential files including:
hello.py
: Starter application script.preswald.toml
: Configuration file for your app.secrets.toml
: Secure file for storing sensitive data.README.md
: Simple introduction to the preswald project.data/
: Directory for some sample data.images/
: Directory with logo, favicon, and readme gif.
Step 3: Write Your First App
Open the hello.py
file and add the following code:
from preswald import text, plotly, connect, get_df, table
import pandas as pd
import plotly.express as px
# Display a welcome message
text("# Welcome to Preswald!")
text("This is your first interactive app built entirely in Python.")
# Connect to data sources (by default, a sample CSV is loaded)
connect()
df = get_df('sample_csv')
# Create a scatter plot to visualize data
fig = px.scatter(
df,
x='quantity',
y='value',
text='item',
title='Quantity vs. Value',
labels={'quantity': 'Quantity', 'value': 'Value'}
)
fig.update_traces(textposition='top center', marker=dict(size=12, color='lightblue'))
fig.update_layout(template='plotly_white')
# Render the plot and data table in your app
plotly(fig)
table(df)
This script does the following:
Uses the
text
function to add headers and descriptive text.Loads data with
connect()
andget_df()
.Creates a Plotly scatter plot that updates interactively.
Displays the underlying data in a neat table.
Step 4: Run Your App Locally
Launch your development server with:
preswald run
The tool will output a URL (typically something like http://localhost:8501
) where you can view your interactive app in your browser.
Step 5: Generate an API key
Go to https://app.preswald.com
Sign in using your GitHub account
Generate an API key in: Organization > Manage > Settings > API Keys
Step 5: Deploy with a Single Command
Once you’re satisfied with your local app, deploying is just as simple:
preswald deploy - target structured - github <github-username> - api-key <structured-api-key> hello.py
This command packages your app and makes it accessible online, so you can instantly share your work with others.
What Preswald Handles
Cloud deployment: Automates the process of containerizing your app, configuring the environment, and deploying it to the target platform.
Local testing: Builds a container locally to let you verify your app before cloud deployment.
Live preview: Provides a shareable URL (for cloud deployments) so others can interact with your app.
Preswald Studio Setup
Go to https://app.preswald.com
Sign in using your GitHub account
Click “+ New project” to create a fresh workspace
Upload the provided CSV file via the Upload button
Choose a Dataset
Select/download a dataset from any of these sources:
Any csv (e.g., weather, finance, sports stats)
Place your dataset in the data/ folder of your project.
Implement Your Preswald App
Modify hello.py
to include the following:
- Load the dataset
from preswald import connect, get_df
connect() # Initialize connection to preswald.toml data sources
df = get_df("my_dataset") # Load data
2. Query or manipulate the data (For SQL database or Use Pandas as pd for other data manipulation)
from preswald import query
sql = "SELECT * FROM my_dataset WHERE value > 50" filtered_df = query(sql, "my_dataset")
3. Build an interactive UI
from preswald import table, text
text("# My Data Analysis App")
table(filtered_df, title="Filtered Data")
- Add user controls:
from preswald import slider, view
threshold = slider("Threshold", min_val=0, max_val=100, default=50)
table(df[df["value"] > threshold], title="Dynamic Data View")
4. Create a visualization
from preswald import plotly
import plotly.express as px
fig = px.scatter(df, x="column1", y="column2", color="category")
plotly(fig)
🔹 Add Other Widgets
Add other widgets to your app. Reference the docs here: Preswald SDK
5. Customize the Branding
Edit the preswald.toml
file to customize the branding.
Change the title of the app
Change the favicon
(Optional) Change the logo (will be visibile if you use sidebar() component)
(Optional) Change the color scheme (will be visibile if you use sidebar() component)
👀 6. Preview Your App
Use the Preview tab in the IDE to view your app.
🚀 5. Get a Shareable Link
Generate an API key in: Organization > Manage > Settings > API Keys
Deploy your app using the Share button
- Enter your GitHub username and API key
3. View the status of the deployment in the Apps tab
Final Thoughts
Preswald is revolutionizing the way data apps are built by minimizing setup time and eliminating the need to write frontend code. Whether you’re a data scientist needing a quick dashboard to explore insights or an engineer looking to prototype internal tools rapidly, Preswald offers a powerful, streamlined solution.
By leveraging its code-first approach, stateful execution, and one-command deployment, you can transform your Python scripts into engaging, interactive applications in minutes — saving time, reducing complexity, and focusing on what really matters: your data.
Check Out My Project
I have also made one using Preswald.
GitHub: https://github.com/bibekkd/preswald_intern_project
Live Demo: https://my-project-602050-eyh7t8yg.preswald.app/
Check this out and let’s build more together!
Subscribe to my newsletter
Read articles from Bibek Kumar Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bibek Kumar Dey
Bibek Kumar Dey
👨💻 Python Enthusiast | Machine Learning Practitioner | Data Science Explorer | Django & Scrapy Developer | Aspiring MLOps & AIOps Enthusiast 🚀 Hey there! 👋 I'm diving deep into the realms of Python, machine learning, and data science, constantly expanding my horizons in these exciting fields. With a solid foundation in machine learning Python frameworks and hands-on experience in Django and Scrapy projects, I'm on a journey to master the art of transforming data into actionable insights. 🔍 Currently, I'm exploring the fascinating world of MLOps and AIOps, eager to bridge the gap between development and operations in AI-driven environments. With a knack for integrating technologies and automating processes, I'm passionate about optimizing workflows and driving efficiency through the power of automation. 🌟 Let's connect and embark on this exhilarating journey together, as we unravel the mysteries of data science and machine learning while venturing into the promising domains of MLOps and AIOps. Together, we'll push the boundaries of what's possible and pave the way for a future powered by data-driven intelligence.