API Rate Limits: How They Work and Why They're Crucial for Applications
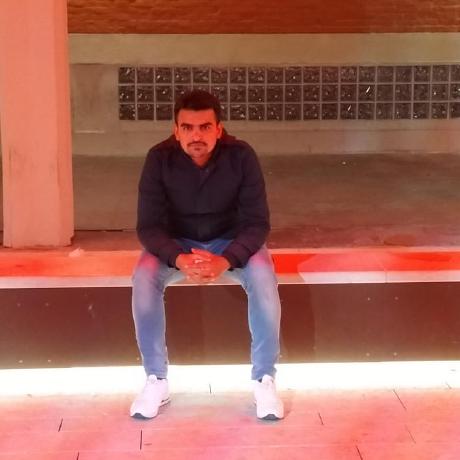
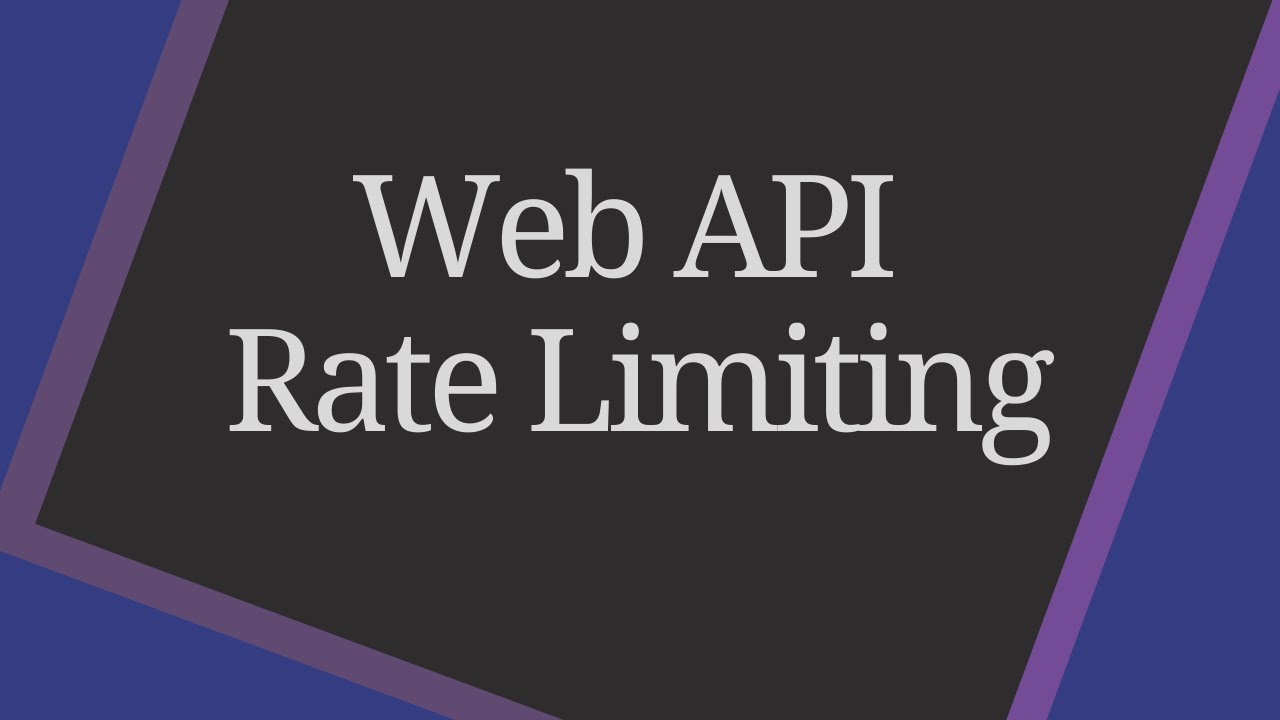
APIs (Application Programming Interfaces) have become the backbone of modern software, facilitating communication between systems, applications, and services. However, with great power comes great responsibility. One critical aspect of API design is the concept of rate limiting. Rate limits ensure that API consumers don’t overload the server, maintaining stability, reliability, and fairness in a shared environment. In this article, we’ll dive into what rate limits are, how they work, and why they are essential, followed by practical examples of rate limiting implementations in Golang.
What Is Rate Limiting?
Rate limiting is a technique used to control the amount of incoming traffic to an API. It ensures that clients (users, applications, or systems) do not exceed a predefined number of requests within a specific time window, such as 100 requests per minute or 1,000 requests per day. Rate limits are imposed to protect server resources, ensure fair use, and prevent abuse or accidental overloads from legitimate users.
Types of Rate Limiting:
Fixed Window Rate Limiting: Requests are limited to a fixed time window (e.g., 1 minute). After the window expires, the limit resets.
Sliding Window Rate Limiting: Similar to fixed windows but more dynamic. The window shifts forward as time progresses, allowing for a smoother and more consistent flow.
Token Bucket: A more flexible system where tokens are replenished at a fixed rate. Each request requires a token, and when the bucket is empty, the system rejects the request.
Leaky Bucket: Similar to token bucket but focuses on smoothing out bursts of traffic. The "leak" happens at a constant rate, and requests accumulate when the system is overwhelmed.
Why Are Rate Limits Important?
Protecting Server Resources: API servers have finite computational and bandwidth resources. Without rate limiting, malicious users or overly aggressive applications could overwhelm the server, leading to downtime or degraded service.
Fairness: Rate limits ensure that all users or applications get a fair share of the resources. If a single user consumes too many resources, others might be deprived of service.
Preventing Abuse: Malicious actors may attempt to abuse the API by sending an overwhelming number of requests in a short period, causing denial-of-service (DoS) conditions. Rate limits help mitigate this risk.
Cost Control: In some cases, particularly for third-party APIs, excessive usage can incur additional costs. Rate limiting allows businesses to monitor and control their API consumption.
User Experience: By preventing server overload, rate limits contribute to better performance and reliability, improving the overall user experience.
Implementing Rate Limiting in Golang
Let’s look at how to implement rate limiting in a Go (Golang) application. We'll implement a simple rate-limited API using the golang.org/x/time/rate package, which provides rate-limiting functionality.
Example 1: Implementing Fixed Window Rate Limiting
In this example, we will limit an API to allow only 5 requests per minute.
package main
import (
"fmt"
"net/http"
"time"
"golang.org/x/time/rate"
)
func main() {
// Create a rate limiter that allows 5 requests per minute (1 req per 12 seconds)
limiter := rate.NewLimiter(rate.Every(12*time.Second), 5)
http.HandleFunc("/api", func(w http.ResponseWriter, r *http.Request) {
// Check if the request is allowed by the rate limiter
if !limiter.Allow() {
http.Error(w, "Rate limit exceeded", http.StatusTooManyRequests)
return
}
// Handle the API request
w.Write([]byte("Request successful"))
})
fmt.Println("Server starting at http://localhost:8080...")
http.ListenAndServe(":8080", nil)
}
In this example:
We create a new rate limiter that allows 5 requests every minute (
rate.Every(12 * time.Second)
means one request every 12 seconds).When the client makes a request, the API checks if the request exceeds the rate limit. If it does, the server responds with a
429 Too Many Requests
status.If the request is within the allowed limit, it processes the request normally.
Example 2: Implementing Token Bucket Rate Limiting
Now, let’s implement a more advanced rate limiter using the token bucket algorithm. In this example, we’ll allow up to 10 requests per minute, but burst traffic will be allowed up to a maximum of 20 requests.
package main
import (
"fmt"
"net/http"
"time"
"golang.org/x/time/rate"
)
func main() {
// Token bucket: 10 requests per minute, maximum burst size of 20
limiter := rate.NewLimiter(rate.Every(6*time.Second), 20)
http.HandleFunc("/api", func(w http.ResponseWriter, r *http.Request) {
// Check if the request is allowed by the rate limiter
if !limiter.Allow() {
http.Error(w, "Rate limit exceeded", http.StatusTooManyRequests)
return
}
// Handle the API request
w.Write([]byte("Request successful"))
})
fmt.Println("Server starting at http://localhost:8080...")
http.ListenAndServe(":8080", nil)
}
In this implementation:
The token bucket allows up to 20 requests in a burst, but after that, only 10 requests per minute are permitted.
The
limiter.Allow()
method checks if the request can proceed by verifying if there are enough tokens in the bucket.
Example 3: Sliding Window Rate Limiting
For sliding window rate limiting, the Go standard library doesn’t provide direct support, but it can be implemented with a custom algorithm. You’d typically maintain timestamps for each request and calculate the number of requests within the time window dynamically.
Conclusion
Rate limiting plays a vital role in API management by maintaining server stability, preventing misuse, and enhancing user experience. In this article, we explored its mechanics, significance, and practical implementation in Go. Whether managing public APIs or internal systems, enforcing rate limits helps protect resources, ensure fair usage, and optimize performance.
By leveraging the provided examples, you can implement effective rate-limiting strategies in your applications, fostering a more secure and reliable API environment.
Thanks for reading, and see you in my next article! Happy hacking! 🚀
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
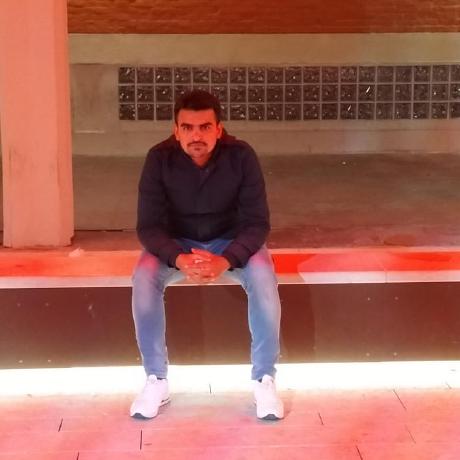
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.