Building a Mindful Moment: Creating an Interactive Breathing Exercise Guide

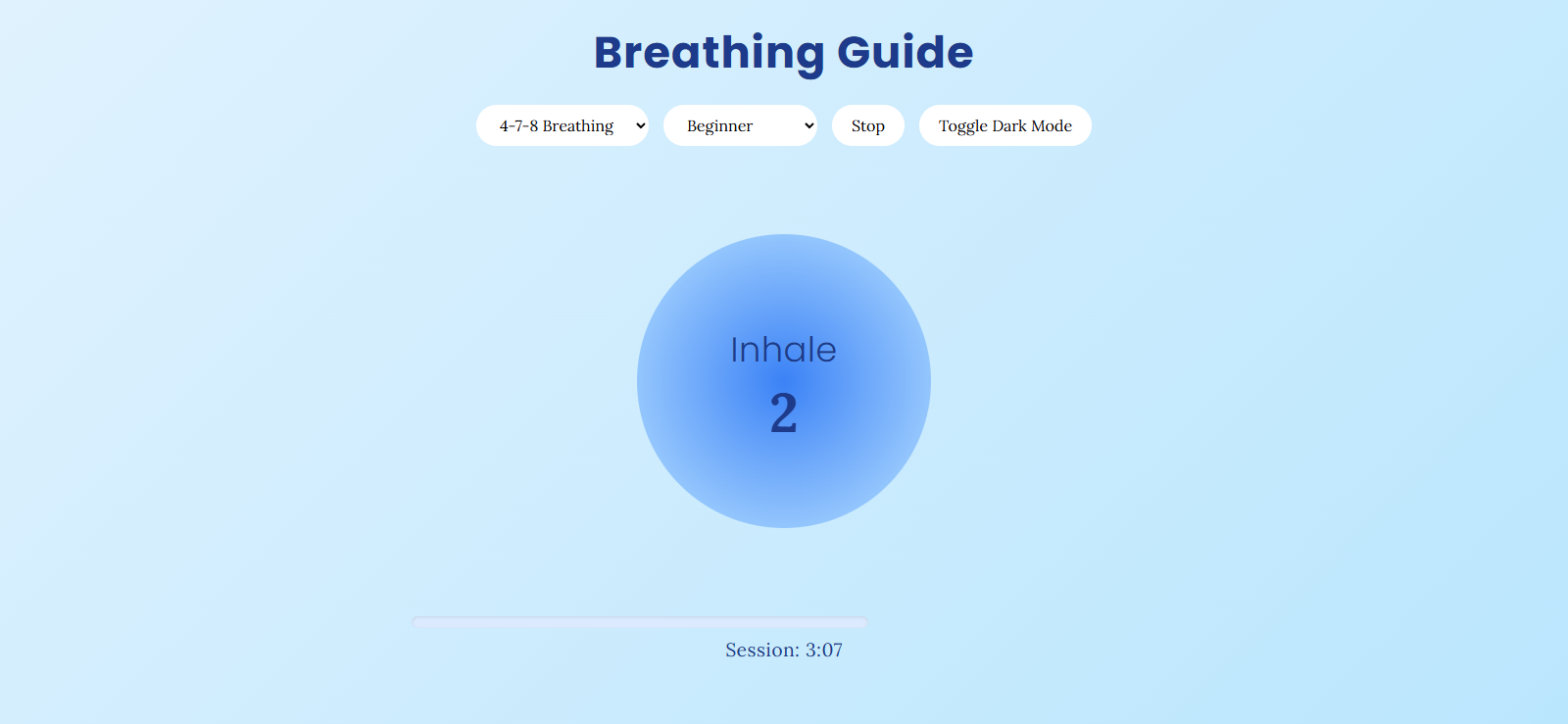
Have you ever found yourself overwhelmed by the constant digital noise of today's world? I certainly have! That's why I decided to build a simple yet powerful breathing exercise application that helps users practice different breathing techniques with visual guidance. In this blog post, I'll walk you through the process of creating this interactive breathing guide from scratch.
Checkout the live link here- https://playground.learncomputer.in/breathing-exercise/
Why Breathing Exercises? ๐งโโ๏ธ
Before diving into the code, let's briefly discuss why breathing exercises matter. Controlled breathing techniques have been scientifically proven to:
Reduce stress and anxiety
Improve focus and concentration
Lower blood pressure
Enhance overall well-being
Different breathing patterns serve different purposes, which is why I've included several options in this application.
Project Overview ๐
Our breathing exercise guide features:
Multiple breathing patterns (4-7-8, Box Breathing, Diaphragmatic)
Difficulty levels (Beginner, Intermediate, Advanced)
Visual animation that expands and contracts with your breath
A countdown timer for each breathing phase
Session tracking to monitor your practice time
Dark mode toggle for comfortable viewing
Let's break down how we built this step by step!
Setting Up the HTML Structure ๐
The HTML structure for our breathing guide is straightforward. We need container elements for our controls, the breathing animation, and progress tracking.
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Breathing Exercise Guide</title>
<link rel="stylesheet" href="styles.css">
<link href="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;700&family=Lora:wght@400;700&display=swap" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="controls">
<h1>Breathing Guide</h1>
<div class="settings">
<select id="pattern">
<option value="478">4-7-8 Breathing</option>
<option value="box">Box Breathing</option>
<option value="diaphragm">Diaphragmatic</option>
</select>
<select id="level">
<option value="beginner">Beginner</option>
<option value="intermediate">Intermediate</option>
<option value="advanced">Advanced</option>
</select>
<button id="startBtn">Start</button>
<button id="darkMode">Toggle Dark Mode</button>
</div>
</div>
<div class="breathing-container">
<div class="circle"></div>
<div class="instructions">
<span id="breathText">Breathe In</span>
<span id="timer">4</span>
</div>
</div>
<div class="progress">
<div class="progress-bar" id="progressBar"></div>
<span id="sessionTime">Session: 0:00</span>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Our HTML structure includes:
A control panel with dropdowns for pattern selection and difficulty level
Start/stop and dark mode toggle buttons
A central breathing circle with text instructions
A countdown timer display
A progress bar to track session duration
Styling with CSS for Visual Appeal ๐จ
The CSS is where we bring our breathing guide to life with smooth animations and a calming color palette. I've used a relaxing blue gradient background that shifts to a deeper blue in dark mode.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
background: linear-gradient(135deg, #e0f2fe, #bae6fd);
min-height: 100vh;
transition: background 0.3s;
}
body.dark {
background: linear-gradient(135deg, #1e3a8a, #3b82f6);
}
.container {
max-width: 800px;
margin: 0 auto;
padding: 20px;
}
.controls {
text-align: center;
margin-bottom: 40px;
color: #1e3a8a;
}
body.dark .controls {
color: #f1f5f9;
}
h1 {
font-family: 'Poppins', sans-serif;
font-weight: 700;
font-size: 2.8rem;
margin-bottom: 20px;
letter-spacing: 1px;
}
.settings {
display: flex;
justify-content: center;
gap: 15px;
flex-wrap: wrap;
}
select, button {
padding: 10px 20px;
border: none;
border-radius: 25px;
background: #ffffff;
cursor: pointer;
font-family: 'Lora', serif;
font-size: 1rem;
font-weight: 400;
transition: all 0.3s ease;
}
body.dark select,
body.dark button {
background: #1e40af;
color: #ffffff;
}
select:hover, button:hover {
transform: translateY(-2px);
box-shadow: 0 4px 15px rgba(0,0,0,0.1);
}
.breathing-container {
position: relative;
height: 400px;
display: flex;
justify-content: center;
align-items: center;
}
.circle {
width: 200px;
height: 200px;
background: radial-gradient(circle, #3b82f6 0%, #93c5fd 70%, transparent 100%);
border-radius: 50%;
position: absolute;
transition: all 1s ease-in-out;
}
body.dark .circle {
background: radial-gradient(circle, #60a5fa 0%, #1e40af 70%, transparent 100%);
}
.inhale {
transform: scale(1.5);
}
.hold {
transform: scale(1.2);
}
.exhale {
transform: scale(1);
}
.instructions {
position: relative;
text-align: center;
color: #1e3a8a;
z-index: 1;
}
body.dark .instructions {
color: #f1f5f9;
}
#breathText {
display: block;
font-family: 'Poppins', sans-serif;
font-weight: 300;
font-size: 2.2rem;
margin-bottom: 10px;
letter-spacing: 0.5px;
}
#timer {
font-family: 'Lora', serif;
font-weight: 700;
font-size: 3.5rem;
line-height: 1;
}
.progress {
margin-top: 40px;
text-align: center;
}
.progress-bar {
width: 100%;
height: 12px;
background: #dbeafe;
border-radius: 6px;
overflow: hidden;
margin-bottom: 10px;
position: relative;
box-shadow: inset 0 1px 3px rgba(0,0,0,0.1);
}
body.dark .progress-bar {
background: #1e40af;
}
.progress-bar::after {
content: '';
display: block;
height: 100%;
width: 0;
background: linear-gradient(90deg, #3b82f6, #10b981);
border-radius: 6px;
transition: width 0.5s ease-out;
position: absolute;
left: 0;
top: 0;
}
body.dark .progress-bar::after {
background: linear-gradient(90deg, #60a5fa, #34d399);
}
#sessionTime {
font-family: 'Lora', serif;
font-weight: 400;
color: #1e3a8a;
font-size: 1.2rem;
letter-spacing: 0.5px;
}
body.dark #sessionTime {
color: #f1f5f9;
}
Key styling features include:
Smooth gradient backgrounds that change with dark mode
A pulsating circle animation that grows and shrinks
Typography that combines sans-serif (Poppins) for headers and serif (Lora) for timers
Responsive design that works on mobile devices
Interactive elements with subtle hover effects
The most important part of our CSS is the animation classes that control the breathing circle:
.inhale {
transform: scale(1.5);
}
.hold {
transform: scale(1.2);
}
.exhale {
transform: scale(1);
}
These classes, combined with CSS transitions, create the smooth expansion and contraction that guides the user's breathing.
Building the JavaScript Functionality โ๏ธ
Now for the exciting part - the JavaScript that powers our breathing guide! I've structured the code using a class-based approach to encapsulate all functionality.
class BreathingGuide {
constructor() {
this.circle = document.querySelector('.circle');
this.breathText = document.getElementById('breathText');
this.timerDisplay = document.getElementById('timer');
this.startBtn = document.getElementById('startBtn');
this.patternSelect = document.getElementById('pattern');
this.levelSelect = document.getElementById('level');
this.progressBar = document.getElementById('progressBar');
this.sessionTimeDisplay = document.getElementById('sessionTime');
this.darkModeBtn = document.getElementById('darkMode');
this.isRunning = false;
this.sessionTime = 0;
this.patterns = {
'478': { inhale: 4, hold: 7, exhale: 8 },
'box': { inhale: 4, hold: 4, exhale: 4, holdOut: 4 },
'diaphragm': { inhale: 6, hold: 2, exhale: 6 }
};
this.levels = {
'beginner': 0.8,
'intermediate': 1,
'advanced': 1.2
};
this.initEvents();
}
initEvents() {
this.startBtn.addEventListener('click', () => this.toggleExercise());
this.darkModeBtn.addEventListener('click', () =>
document.body.classList.toggle('dark'));
}
toggleExercise() {
if (this.isRunning) {
this.stop();
} else {
this.start();
}
}
start() {
this.isRunning = true;
this.startBtn.textContent = 'Stop';
this.sessionTime = 0;
this.runSession();
this.sessionInterval = setInterval(() => this.updateSessionTime(), 1000);
}
stop() {
this.isRunning = false;
this.startBtn.textContent = 'Start';
clearInterval(this.currentInterval);
clearInterval(this.sessionInterval);
this.circle.className = 'circle';
this.breathText.textContent = 'Paused';
this.timerDisplay.textContent = '0';
}
async runSession() {
const pattern = this.patterns[this.patternSelect.value];
const speed = this.levels[this.levelSelect.value];
while (this.isRunning) {
await this.breathe('Inhale', pattern.inhale / speed, 'inhale');
if (!this.isRunning) break;
await this.breathe('Hold', pattern.hold / speed, 'hold');
if (!this.isRunning) break;
await this.breathe('Exhale', pattern.exhale / speed, 'exhale');
if (pattern.holdOut && this.isRunning) {
await this.breathe('Hold', pattern.holdOut / speed, 'hold');
}
}
}
breathe(text, duration, animation) {
return new Promise(resolve => {
this.breathText.textContent = text;
this.circle.className = `circle ${animation}`;
let timeLeft = Math.round(duration);
this.timerDisplay.textContent = timeLeft;
this.currentInterval = setInterval(() => {
timeLeft--;
this.timerDisplay.textContent = timeLeft;
if (timeLeft <= 0) {
clearInterval(this.currentInterval);
resolve();
}
}, 1000);
const progressPercentage = Math.min((this.sessionTime / 300) * 100, 100);
this.progressBar.style.width = `${progressPercentage}%`;
});
}
updateSessionTime() {
this.sessionTime++;
const minutes = Math.floor(this.sessionTime / 60);
const seconds = this.sessionTime % 60;
this.sessionTimeDisplay.textContent =
`Session: ${minutes}:${seconds < 10 ? '0' + seconds : seconds}`;
}
}
document.addEventListener('DOMContentLoaded', () => {
new BreathingGuide();
});
Let's break down the key aspects of our JavaScript implementation:
The BreathingGuide Class
I created a BreathingGuide
class that handles all the application logic. This approach keeps our code organized and maintainable.
Breathing Patterns Configuration
The app supports multiple breathing patterns, each with different timing:
this.patterns = {
'478': { inhale: 4, hold: 7, exhale: 8 },
'box': { inhale: 4, hold: 4, exhale: 4, holdOut: 4 },
'diaphragm': { inhale: 6, hold: 2, exhale: 6 }
};
4-7-8 Breathing: Inhale for 4 seconds, hold for 7, exhale for 8 (excellent for relaxation)
Box Breathing: Equal duration for all phases (great for focus)
Diaphragmatic Breathing: Longer inhale and exhale with a short hold (beneficial for lung capacity)
Difficulty Levels
To accommodate different experience levels, I implemented a speed multiplier:
this.levels = {
'beginner': 0.8,
'intermediate': 1,
'advanced': 1.2
};
Beginners get a slower pace (multiplying durations by 0.8), while advanced users practice at a faster pace (multiplying by 1.2).
The Breathing Cycle
The core functionality uses async/await with Promises to create a sequential breathing cycle:
async runSession() {
const pattern = this.patterns[this.patternSelect.value];
const speed = this.levels[this.levelSelect.value];
while (this.isRunning) {
await this.breathe('Inhale', pattern.inhale / speed, 'inhale');
if (!this.isRunning) break;
await this.breathe('Hold', pattern.hold / speed, 'hold');
if (!this.isRunning) break;
await this.breathe('Exhale', pattern.exhale / speed, 'exhale');
if (pattern.holdOut && this.isRunning) {
await this.breathe('Hold', pattern.holdOut / speed, 'hold');
}
}
}
This approach allows us to create a continuous loop that guides the user through each breathing phase in sequence.
The breathe() Method
The breathe()
method handles each individual phase of breathing:
breathe(text, duration, animation) {
return new Promise(resolve => {
this.breathText.textContent = text;
this.circle.className = `circle ${animation}`;
let timeLeft = Math.round(duration);
this.timerDisplay.textContent = timeLeft;
this.currentInterval = setInterval(() => {
timeLeft--;
this.timerDisplay.textContent = timeLeft;
if (timeLeft <= 0) {
clearInterval(this.currentInterval);
resolve();
}
}, 1000);
});
}
This method:
Updates the instruction text ("Inhale", "Hold", or "Exhale")
Applies the appropriate animation class to the circle
Sets up a countdown timer
Resolves the promise when the timer completes
Session Tracking
The app tracks session duration to help users monitor their practice:
updateSessionTime() {
this.sessionTime++;
const minutes = Math.floor(this.sessionTime / 60);
const seconds = this.sessionTime % 60;
this.sessionTimeDisplay.textContent =
`Session: ${minutes}:${seconds < 10 ? '0' + seconds : seconds}`;
}
Technical Challenges and Solutions ๐ ๏ธ
During development, I encountered a few interesting challenges:
Challenge 1: Sequential Animation Flow
I needed a way to flow from one breathing phase to the next without abrupt interruptions. Initially, I tried nested setTimeout functions, but this quickly became unmanageable.
Solution: Using async/await with Promises provided a clean, sequential way to move through the breathing phases while keeping the code readable.
Challenge 2: Responsive Design
The breathing circle needed to look good and function properly on screens of all sizes.
Solution: Using relative units (percentages) for the circle dimensions and CSS flexbox for layout ensured the app worked well across devices.
Challenge 3: User Experience Timing
Finding the right balance for beginners versus advanced users required testing different timing adjustments.
Solution: The difficulty level multiplier (0.8 for beginners, 1.2 for advanced) provided just enough difference to accommodate varying experience levels without creating drastically different experiences.
Future Enhancements ๐
While the current version works well, there are several enhancements I'm considering:
Sound guidance: Adding optional audio cues for each breathing phase
Custom patterns: Allowing users to create and save their own breathing patterns
Breathing statistics: Tracking and visualizing practice frequency and duration
Guided sessions: Adding prerecorded guided meditation sessions
PWA support: Making the app installable for offline use
Conclusion
Building this breathing exercise guide taught me a lot about creating meaningful web applications that can positively impact users' lives. By combining clean HTML structure, smooth CSS animations, and thoughtful JavaScript logic, we've created a tool that's both functional and pleasant to use.
The most satisfying part has been seeing how a relatively simple application can provide real value - helping people take a moment to breathe and find calm in their busy days.
You can try the application yourself at Breathing Exercise Guide. I'd love to hear your feedback or suggestions for improvements!
What breathing techniques do you find most helpful? Have you built applications focused on wellness or mindfulness? Share your thoughts in the comments below!
Subscribe to my newsletter
Read articles from Learn Computer Academy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
