useForm Like a Pro: Mistakes You Might Be Making (And How to Fix Them)
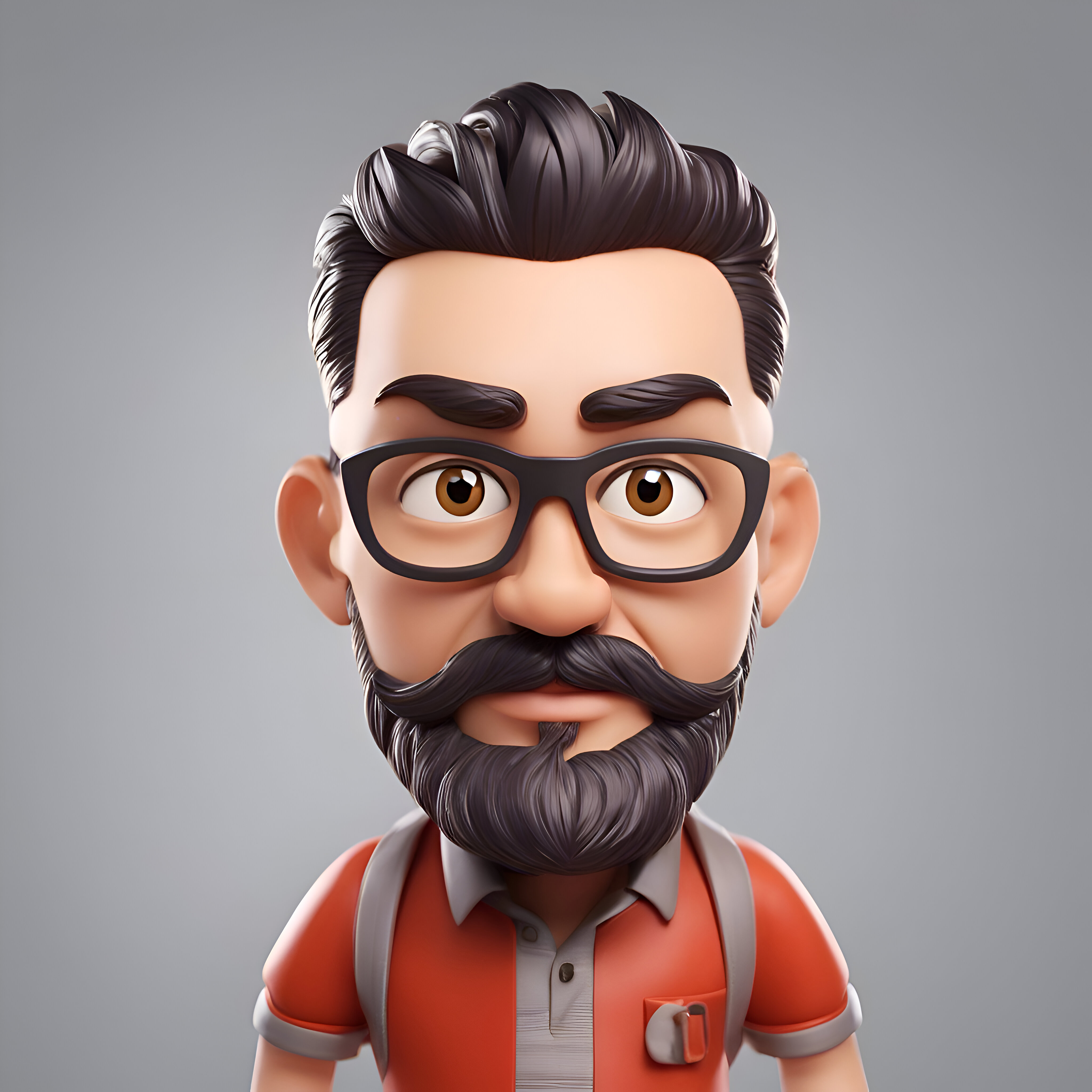
Table of contents
- The Problem (Or: Why Forms Make Developers Cry)
- The Solution: useForm Hook (A.K.A. Form Therapy)
- Key Features (Or: Why You’ll Delete Your Old Form Code)
- Using the Register API (Because Less Code = More Happiness)
- Validation Rules (For All Those Users Who Type “password123”)
- Conditional Validation (For When Life Gets Complicated)
- Complete Sign-Up Form Example (That Actually Works!)
- Best Practices (From Developers Who’ve Made All the Mistakes)
- Full Implementation Reference
- Conclusion
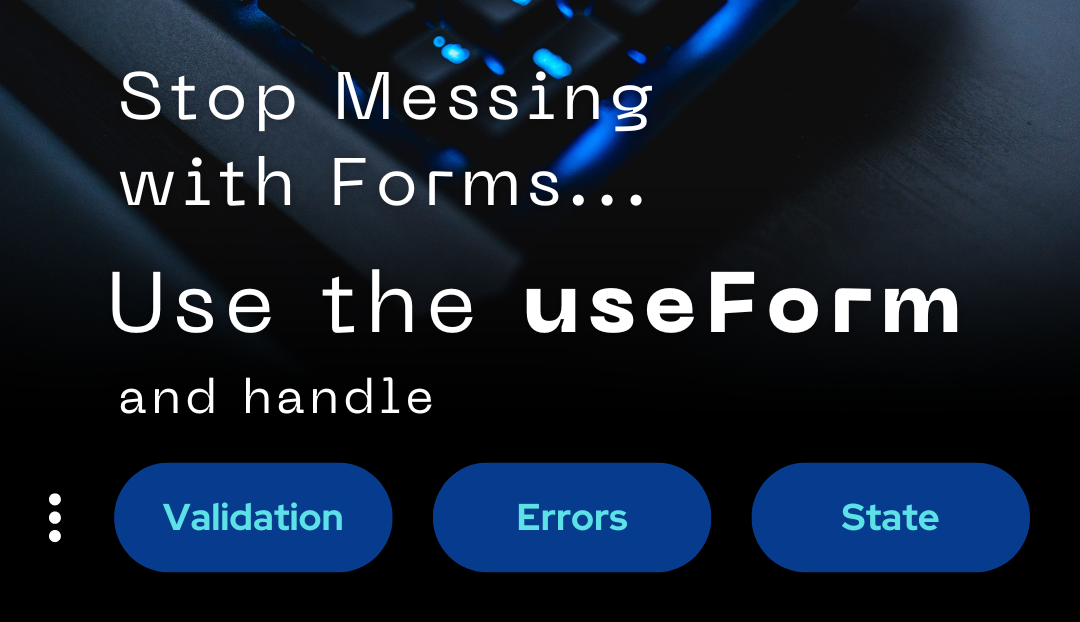
Let’s face it — building forms in React is about as enjoyable as stepping on a LEGO at 3 AM. You start with the best intentions, telling yourself “it’s just a simple form,” and six hours later you’re surrounded by coffee cups, questioning your career choices.
This is your intervention. Put down that seventh cup of coffee and let me introduce you to useForm
— the hook that will make you hate forms slightly less than you do now.
The Problem (Or: Why Forms Make Developers Cry)
If you’ve ever built a form in React, you’re probably familiar with code that looks like this monstrosity:
function TraditionalForm() {
const [name, setName] = useState("");
const [email, setEmail] = useState("");
const [nameError, setNameError] = useState("");
const [emailError, setEmailError] = useState("");
// …27 more state variables because why not?
const handleNameChange = e => {
setName(e.target.value);
if (e.target.value.trim() === "") {
setNameError("Name is required");
} else if (e.target.value.length < 3) {
setNameError("Name must be at least 3 characters");
} else {
setNameError("");
}
};
const handleEmailChange = e => {
// Copy and paste the function above with minor tweaks
// Developer skills at work!
};
// Several more nearly-identical functions here
const handleSubmit = e => {
e.preventDefault();
// Validate everything again because trust issues
// Submit if the stars align and Mercury isn't in retrograde
};
return (
<form onSubmit={handleSubmit}>
{/* A UI that took 30% of your development time */}
</form>
);
}
This approach has several drawbacks:
State Explosion: More state variables than you have fingers and toes
Copy-Paste Engineering: Spread that validation logic like it’s going out of style
Bug Breeding Ground: “Why isn’t my form validating?” (Narrator: It was.)
TypeScript Nightmares: Type errors that make you question if you really understand programming after all
The Solution: useForm Hook (A.K.A. Form Therapy)
Our custom useForm
hook provides a simple API that won’t make you want to become a sheep farmer:
import { useForm } from "./lib/hooks/useForm";
import { required, email } from "./lib/validation";
function SimpleForm() {
const { values, errors, handleChange, handleSubmit } = useForm({
initialValues: {
name: "",
email: "",
},
validationSchema: {
name: [required()],
email: [required(), email()],
},
onSubmit: values => {
console.log("Form submitted:", values);
// Time to celebrate with a victory dance
},
});
return (
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="name">Name</label>
<input id="name" name="name" value={values.name} onChange={handleChange} />
{errors.name && <div className="error">{errors.name}</div>}
</div>
<div>
<label htmlFor="email">Email</label>
<input id="email" name="email" type="email" value={values.email} onChange={handleChange} />
{errors.email && <div className="error">{errors.email}</div>}
</div>
<button type="submit">Submit</button>
</form>
);
}
Key Features (Or: Why You’ll Delete Your Old Form Code)
The useForm
hook provides:
One State to Rule Them All: All form values and errors in one place, like a neat little package that won’t explode in your face
Validation That Actually Makes Sense: Declarative validation rules that don’t make you question your life choices
TypeScript That Helps Instead of Hurts: Full type safety without wanting to throw your computer out the window
Less Code, More Coffee Time: Do more with less so you can actually take that lunch break
Field Tracking: Knows which fields have been touched, unlike your memory after debugging for 5 hours straight
Using the Register API (Because Less Code = More Happiness)
Our hook provides a register
function that helps you connect form fields with less code than your average tweet: The register
function bundles everything together like a burrito of form functionality — all the good stuff wrapped in a neat package.
import { FormField } from "./components/FormField";
import { useForm } from "./lib/hooks/useForm";
import { required, email, minLength } from "./lib/validation";
function RegisterAPIForm() {
const { handleSubmit, register } = useForm({
initialValues: {
name: "",
email: "",
password: "",
},
validationSchema: {
name: [required(), minLength(3)],
email: [required(), email()],
password: [required(), minLength(8)], // Because "password" is not secure
},
onSubmit: values => {
console.log("Form submitted:", values);
// Tell your PM you spent days on this
},
});
return (
<form onSubmit={handleSubmit}>
<FormField {...register("name")} label="Name" />
<FormField {...register("email")} label="Email" type="email" />
<FormField {...register("password")} label="Password" type="password" />
<button type="submit">Submit</button>
</form>
);
}
The register
function bundles everything together like a burrito of form functionality — all the good stuff wrapped in a neat package.
The FormField
component is like the Swiss Army knife of form fields — it handles labels, inputs, and error messages so you don’t have to. For the full code (and to see how the magic happens), check out The FormField Component.
Validation Rules (For All Those Users Who Type “password123”)
Our validation system has more rules than a bureaucratic handbook:
import {
required,
email,
minLength,
maxLength,
pattern,
url,
numeric,
alphanumeric,
range,
passwordStrength,
passwordMatch,
phoneNumber,
date,
} from "./lib/validation";
// Example usage for those users who think "123" is a strong password:
const validationSchema = {
username: [
required("Please provide a username, or we'll just call you 'Mystery Guest'"),
minLength(3, "Your username needs at least 3 characters—brevity isn't always the soul of wit"),
],
email: [
required("We need your email, but don't worry, we won't send you cat memes... probably"),
email("That doesn't look like a valid email—did you forget the '@'?"),
],
password: [
required("A password is required—security first!"),
minLength(8, "Make it at least 8 characters—short passwords are so last decade"),
passwordStrength("Your password needs to be stronger—think 'Fort Knox,' not '1234'"),
],
confirmPassword: [
required("Please confirm your password—we're not mind readers"),
passwordMatch("password", "Your passwords don't match—try again, champ"),
],
age: [
numeric("Enter your age as a number, not 'forever young'"),
range(18, 99, "You must be between 18 and 99—no exceptions for time travelers"),
],
};
Conditional Validation (For When Life Gets Complicated)
Sometimes validation depends on other form values. The when
helper lets you add conditions dynamically, making your forms smarter and more adaptable:
import { when, required } from "./lib/validation";
const validationSchema = {
shippingAddress: [
when(
values => values.needsShipping === true,
required("We need to know where to send your stuff unless you're telepathic")
),
],
companyName: [
when(
values => values.accountType === "business",
required("Businesses typically have names, unless you're in the witness protection program")
),
],
};
Complete Sign-Up Form Example (That Actually Works!)
Here’s a real-world example of a sign-up form that won’t make you question your career choices:
import { FormField } from "./components/FormField";
import { useForm } from "./lib/hooks/useForm";
import { email, minLength, passwordMatch, passwordStrength, required } from "./lib/validation";
const SignUpForm = () => {
const { handleSubmit, register } = useForm({
initialValues: {
name: "",
email: "",
password: "",
confirmPassword: "",
},
validateOnBlur: true,
validateOnChange: true,
validationSchema: {
name: [required(), minLength(3)],
email: [required(), email()],
password: [required(), minLength(8), passwordStrength()],
confirmPassword: [required(), passwordMatch("password")],
},
onSubmit: values => {
console.log("Form submitted with values:", values);
// In a real app, you would call an API here
alert("Sign up successful! We now own your data!");
},
});
return (
<div className="flex flex-col items-center justify-center min-h-screen bg-slate-200">
<form onSubmit={handleSubmit} className="w-full max-w-md p-6 bg-white rounded-lg shadow-md space-y-4">
<h2 className="text-2xl font-bold text-center mb-6">Join Our Cult (Er, Platform)</h2>
<FormField {...register("name")} label="Name" placeholder="Your name, not your gamer tag" type="text" />
<FormField {...register("email")} label="Email" placeholder="The one you actually check" type="email" />
<FormField {...register("password")} label="Password" placeholder="Not 'password123' please" type="password" />
<FormField
{...register("confirmPassword")}
label="Confirm Password"
placeholder="Same as above, we're checking"
type="password"
/>
<button
type="submit"
className="w-full py-2 px-4 bg-purple-500 text-white cursor-pointer rounded-md hover:bg-purple-500/90 transition-colors mt-6"
>
Sign Up (No Soul Required)
</button>
</form>
</div>
);
};
Best Practices (From Developers Who’ve Made All the Mistakes)
- Type your form values (unless you enjoy debugging at 2 AM):
interface LoginFormValues {
email: string;
password: string;
rememberMe: boolean; // For those who trust their browser more than their memory
}
const { values } = useForm<LoginFormValues>({
initialValues: {
email: "",
password: "",
rememberMe: false,
},
// ...other stuff that makes forms work
});
- Customize validation timing to balance user experience and your sanity:
useForm({
// ...
validateOnChange: true, // For the anxious who need immediate feedback
validateOnBlur: true, // For the reflective who prefer feedback after thinking
});
- Use custom error messages that don’t make users feel like they’re being scolded by a robot:
minLength(8, "Think of a password as a toothbrush: longer is better and don't share it with friends");
- Separate form logic from UI because mixing concerns is like putting pineapple on pizza — technically possible but fundamentally wrong.
Full Implementation Reference
For those who want to copy-paste their way to productivity (we don’t judge), here are the full implementations of the core components:
The useForm Hook
// lib/hooks/useForm.ts
import { ChangeEvent, FormEvent, useState } from "react";
import { ValidationRule, validateField, validateForm } from "../validation";
export interface UseFormOptions<T extends Record<string, any>> {
initialValues: T;
onSubmit: (values: T) => Promise<void> | void;
validationSchema?: {
[K in keyof Partial<T>]?: ValidationRule<T, T[K]>[];
};
validateOnChange?: boolean;
validateOnBlur?: boolean;
}
export function useForm<T extends Record<string, any>>({
initialValues,
onSubmit,
validationSchema = {},
validateOnChange = false,
validateOnBlur = false,
}: UseFormOptions<T>) {
const [values, setValues] = useState<T>(initialValues);
const [errors, setErrors] = useState<Partial<Record<keyof T, string>>>({});
const [touched, setTouched] = useState<Partial<Record<keyof T, boolean>>>({});
const [isSubmitting, setIsSubmitting] = useState(false);
const validate = (fieldName?: keyof T) => {
if (fieldName) {
// Validate single field
const fieldRules = validationSchema[fieldName] as ValidationRule<T, T[typeof fieldName]>[] | undefined;
if (fieldRules) {
const error = validateField(values[fieldName], fieldRules, values);
setErrors(prev => ({
...prev,
[fieldName]: error,
}));
return !error;
}
return true;
} else {
// Validate all fields
const newErrors = validateForm(values, validationSchema);
setErrors(newErrors);
return Object.keys(newErrors).length === 0;
}
};
const handleChange = (e: ChangeEvent<HTMLInputElement | HTMLSelectElement | HTMLTextAreaElement>) => {
const { name, value, type } = e.target as HTMLInputElement;
const fieldName = name as keyof T;
// Handle checkbox inputs
const newValue = type === "checkbox" ? (e.target as HTMLInputElement).checked : value;
// Update values first
setValues(prev => ({
...prev,
[fieldName]: newValue,
}));
if (validateOnChange || errors[fieldName]) {
const fieldRules = validationSchema[fieldName] as ValidationRule<T, T[typeof fieldName]>[] | undefined;
if (fieldRules) {
const updatedValues = { ...values, [fieldName]: newValue };
const error = validateField(newValue as T[typeof fieldName], fieldRules, updatedValues);
setErrors(prev => ({
...prev,
[fieldName]: error,
}));
if (!touched[fieldName]) {
setTouched(prev => ({
...prev,
[fieldName]: true,
}));
}
}
}
};
const handleBlur = (e: React.FocusEvent<HTMLInputElement | HTMLSelectElement | HTMLTextAreaElement>) => {
const fieldName = e.target.name as keyof T;
setTouched(prev => ({
...prev,
[fieldName]: true,
}));
if (validateOnBlur) {
validate(fieldName);
}
};
const handleSubmit = async (e: FormEvent<HTMLFormElement>) => {
e.preventDefault();
// Mark all fields as touched
const touchedFields = Object.keys(validationSchema).reduce(
(acc, key) => ({ ...acc, [key]: true }),
{} as Record<keyof T, boolean>
);
setTouched(touchedFields as Partial<Record<keyof T, boolean>>);
// Validate all fields
const isValid = validate();
if (isValid) {
setIsSubmitting(true);
try {
await onSubmit(values);
} catch (error) {
console.error("Form submission error:", error);
} finally {
setIsSubmitting(false);
}
}
};
const setFieldValue = (fieldName: keyof T, value: T[keyof T]) => {
setValues(prev => ({
...prev,
[fieldName]: value,
}));
if (validateOnChange && touched[fieldName]) {
validate(fieldName);
}
};
const setFieldError = (fieldName: keyof T, error: string) => {
setErrors(prev => ({
...prev,
[fieldName]: error,
}));
};
const resetForm = () => {
setValues(initialValues);
setErrors({});
setTouched({});
setIsSubmitting(false);
};
const register = (fieldName: keyof T) => {
return {
name: fieldName,
value: values[fieldName],
onChange: handleChange,
onBlur: handleBlur,
error: errors[fieldName],
};
};
return {
values,
errors,
touched,
isSubmitting,
handleChange,
handleBlur,
handleSubmit,
setFieldValue,
setFieldError,
resetForm,
validate,
register,
};
}
Validation Library
// lib/validation.ts
/**
* Type-safe validation utilities for form fields
*/
// Generic validation rule type that can handle different value types
export type ValidationRule<T extends Record<string, unknown>, V = unknown> = {
validate: (value: V, formValues?: T) => boolean;
message: string | ((params: Record<string, unknown>) => string);
params?: Record<string, unknown>;
};
// Helper to create validation messages with parameters
const createMessage = (
message: string | ((params: Record<string, unknown>) => string),
params?: Record<string, unknown>
): string => {
if (typeof message === "function") {
return message(params || {});
}
if (!params) return message;
return Object.entries(params).reduce((msg, [key, value]) => msg.replace(new RegExp(`{${key}}`, "g"), String(value)), message);
};
export const required = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => value !== undefined && value !== null && value.trim() !== "",
message: message || "This field is required",
});
export const email = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return !value || emailRegex.test(value);
},
message: message || "Please enter a valid email address",
});
export const minLength = <T extends Record<string, unknown>>(length: number, message?: string): ValidationRule<T, string> => ({
validate: value => !value || value.length >= length,
message: message || "Must be at least {length} characters long",
params: { length },
});
export const maxLength = <T extends Record<string, unknown>>(length: number, message?: string): ValidationRule<T, string> => ({
validate: value => !value || value.length <= length,
message: message || "Cannot exceed {length} characters",
params: { length },
});
export const pattern = <T extends Record<string, unknown>>(regex: RegExp, message?: string): ValidationRule<T, string> => ({
validate: value => !value || regex.test(value),
message: message || "Invalid format",
});
export const url = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => {
if (!value) return true;
try {
new URL(value);
return true;
} catch {
return false;
}
},
message: message || "Please enter a valid URL",
});
export const numeric = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => !value || /^[0-9]+$/.test(value),
message: message || "Must contain only numbers",
});
export const alphanumeric = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => !value || /^[a-zA-Z0-9]+$/.test(value),
message: message || "Must contain only letters and numbers",
});
export const range = <T extends Record<string, unknown>>(
min: number,
max: number,
message?: string
): ValidationRule<T, string | number> => ({
validate: value => {
if (value === undefined || value === null || value === "") return true;
const numValue = typeof value === "string" ? parseFloat(value) : value;
return !isNaN(numValue as number) && numValue >= min && numValue <= max;
},
message: message || "Value must be between {min} and {max}",
params: { min, max },
});
export const passwordStrength = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => {
if (!value) return true;
const hasLetter = /[a-zA-Z]/.test(value);
const hasNumber = /\d/.test(value);
const hasSpecialChar = /[!@#$%^&*(),.?":{}|<>]/.test(value);
return hasLetter && hasNumber && hasSpecialChar;
},
message: message || "Password must contain letters, numbers, and special characters",
});
export const passwordMatch = <T extends Record<string, unknown>>(
matchField: keyof T,
message?: string
): ValidationRule<T, string> => ({
validate: (value, formValues) => !value || value === (formValues?.[matchField] as string),
message: message || "Passwords do not match",
});
export const phoneNumber = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => {
if (!value) return true;
const phoneRegex = /^\+?[0-9]{10,15}$/;
return phoneRegex.test(value.replace(/[-()\s]/g, ""));
},
message: message || "Please enter a valid phone number",
});
export const date = <T extends Record<string, unknown>>(message?: string): ValidationRule<T, string> => ({
validate: value => {
if (!value) return true;
const date = new Date(value);
return !isNaN(date.getTime());
},
message: message || "Please enter a valid date",
});
export const fileType = <T extends Record<string, unknown>>(
allowedTypes: string[],
message?: string
): ValidationRule<T, File | null> => ({
validate: file => {
if (!file) return true;
const extension = file.name.split(".").pop()?.toLowerCase() || "";
return allowedTypes.includes(extension);
},
message: message || "File type not allowed. Allowed types: {types}",
params: { types: allowedTypes.join(", ") },
});
export const fileSize = <T extends Record<string, unknown>>(
maxSizeInMB: number,
message?: string
): ValidationRule<T, File | null> => ({
validate: file => {
if (!file) return true;
const sizeInMB = file.size / (1024 * 1024);
return sizeInMB <= maxSizeInMB;
},
message: message || "File size cannot exceed {size}MB",
params: { size: maxSizeInMB },
});
export const when = <T extends Record<string, unknown>, V>(
condition: (formValues?: T) => boolean,
rule: ValidationRule<T, V>
): ValidationRule<T, V> => ({
validate: (value, formValues) => {
return !condition(formValues) || rule.validate(value, formValues);
},
message: rule.message,
params: rule.params,
});
/**
* Validates a field against provided rules
*/
export const validateField = <T extends Record<string, unknown>, V>(
value: V,
rules: ValidationRule<T, V>[],
formValues?: T
): string => {
for (const rule of rules) {
if (!rule.validate(value, formValues)) {
return createMessage(rule.message, rule.params);
}
}
return "";
};
/**
* Validates all fields in a form
*/
export const validateForm = <T extends Record<string, unknown>>(
formValues: T,
validationSchema: { [K in keyof T]?: ValidationRule<T, T[K]>[] }
): { [K in keyof T]?: string } => {
const errors: Partial<Record<keyof T, string>> = {};
for (const field in validationSchema) {
if (validationSchema[field]) {
const error = validateField(
formValues[field],
validationSchema[field] as ValidationRule<T, T[typeof field]>[],
formValues
);
if (error) {
errors[field] = error;
}
}
}
return errors;
};
FormField Component
// components/FormField.tsx
interface FormFieldProps extends React.InputHTMLAttributes<HTMLInputElement> {
label: string;
error?: string;
}
export const FormField: React.FC<FormFieldProps> = ({ label, error, ...props }) => {
return (
<div className={`mb-4 ${props.className}`}>
<label htmlFor={props.name} className="block text-sm font-medium text-gray-700 mb-1">
{label}
</label>
<input
id={props.name}
className={`w-full px-3 py-2 border rounded-lg shadow-sm focus:outline-none focus:ring-2 focus:border-transparent ${
error ? "border-red-500 focus:ring-red-200" : "border-gray-300 focus:ring-blue-200 focus:border-blue-500"
} ${props.disabled ? "bg-gray-100 text-gray-500" : ""}`}
{...props}
/>
{error && <p className="mt-1 text-sm text-red-600">{error}</p>}
</div>
);
};
Conclusion
The useForm
hook won’t fix all your problems (sorry about that receding hairline), but it will make your form development experience significantly less painful. No more state management nightmares, no more validation logic sprawling across your components like an invasive species.
With our beautifully crafted, lovingly maintained, and slightly over-engineered form solution, you can focus on the important things — like arguing about whether dark mode is superior or if that button should be 2 pixels to the left.
Now go forth and create forms that don’t make users (or developers) want to throw their devices out the window. Your users will thank you, your therapist will see you less often, and your code reviewers might actually smile for once.
This article was written by a developer who has spent way too much time dealing with forms and has the therapy bills to prove it. No forms were harmed in the making of this hook, though several keyboards suffered minor damage from frustrated typing.
Subscribe to my newsletter
Read articles from Sazzadur Rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
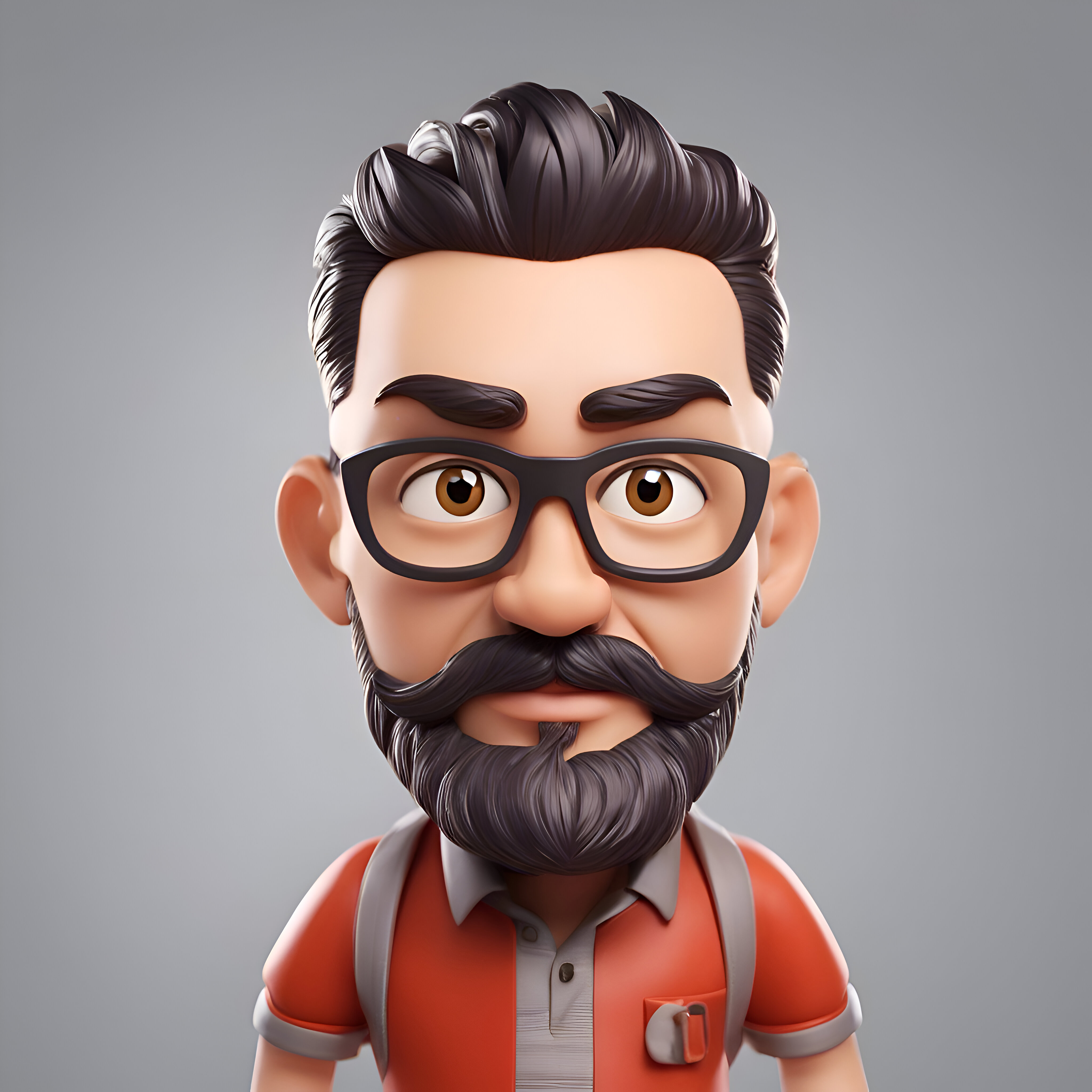
Sazzadur Rahman
Sazzadur Rahman
👋 Hey there! I'm a passionate developer with a knack for creating robust and user-friendly applications. My expertise spans across various technologies, including TypeScript, JavaScript, SolidJS, React, NextJS.