Introduction to React Native for Mobile Development

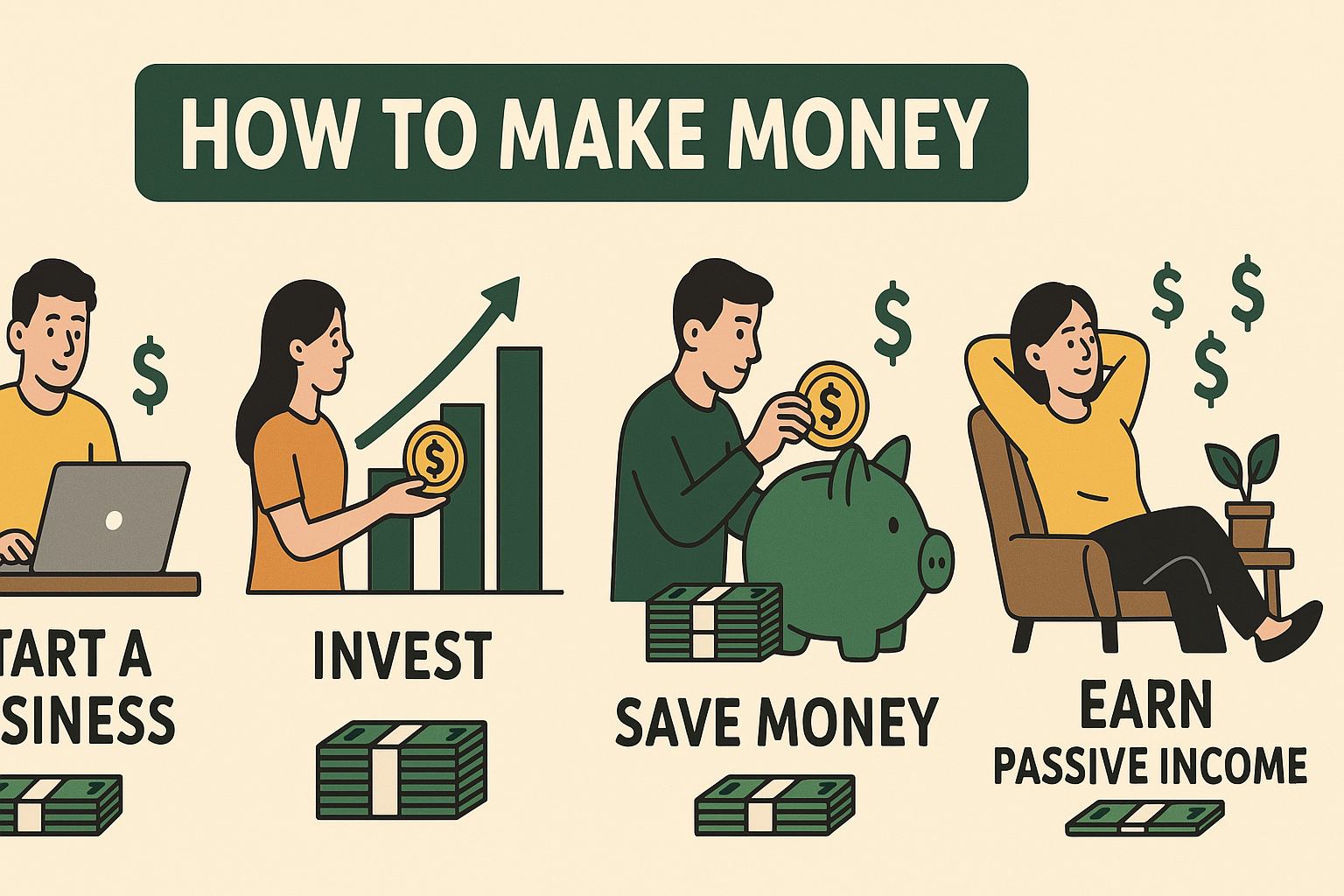
Introduction to React Native for Mobile Development
React Native has revolutionized mobile app development by enabling developers to build cross-platform applications using JavaScript and React. Whether you're a seasoned developer or just starting, React Native offers a powerful and efficient way to create high-performance mobile apps for both iOS and Android.
In this guide, we'll explore the fundamentals of React Native, its advantages, and how you can get started. Plus, if you're looking to monetize your programming skills, check out MillionFormula, a free platform to make money online without needing credit or debit cards.
What is React Native?
React Native is an open-source framework developed by Facebook (now Meta) that allows developers to build mobile apps using JavaScript and React. Unlike traditional hybrid frameworks (like Cordova or Ionic), React Native compiles to native components, providing near-native performance.
Key Features of React Native
Cross-Platform Development – Write once, deploy on both iOS and Android.
Native Performance – Uses native components instead of WebViews.
Hot Reloading – See changes instantly without recompiling.
Large Ecosystem – Access to npm packages and native modules.
Strong Community Support – Backed by Meta and a vast developer community.
Why Choose React Native Over Other Frameworks?
1. Faster Development
Since React Native allows code reuse between platforms, development is significantly faster compared to building separate iOS (Swift) and Android (Kotlin/Java) apps.
2. Cost-Effective
Businesses save money by maintaining a single codebase instead of hiring separate iOS and Android developers.
3. Performance Close to Native
Unlike hybrid frameworks that rely on WebViews, React Native renders native UI components, ensuring smoother animations and better responsiveness.
4. Strong Industry Adoption
Companies like Facebook, Instagram, Airbnb, and Shopify use React Native, proving its scalability and reliability.
Getting Started with React Native
Prerequisites
Before diving in, ensure you have:
Basic knowledge of JavaScript and React.
Node.js installed (for npm/yarn).
Android Studio (for Android development) or Xcode (for iOS).
Setting Up Your First React Native App
Using Expo (Easiest Way)
Expo is a toolchain that simplifies React Native development. bash Copy
npx create-expo-app MyFirstApp
cd MyFirstApp
npx expo start
This will launch the Expo Dev Tools in your browser. Scan the QR code with the Expo Go app (available on iOS/Android) to see your app live.
Using React Native CLI (More Control)
For a more customized setup, use the React Native CLI: bash Copy
npx react-native init MyFirstApp
cd MyFirstApp
npx react-native run-android # or run-ios
This will start a new project and run it on an emulator or connected device.
Core Concepts in React Native
1. Components
React Native uses reusable components, similar to React for the web. jsx Copy
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const App = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, React Native!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 24,
fontWeight: 'bold',
},
});
export default App;
2. Styling
Instead of CSS, React Native uses a JavaScript-based styling system with StyleSheet
.
3. Navigation
For screen navigation, libraries like React Navigation are commonly used: bash Copy
npm install @react-navigation/native @react-navigation/stack
Example of a simple stack navigator: jsx Copy
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
4. Handling API Calls
Fetching data in React Native is similar to web React: jsx Copy
import React, { useEffect, useState } from 'react';
import { View, Text } from 'react-native';
const DataFetchingExample = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((json) => setData(json))
.catch((error) => console.error(error));
}, []);
return (
<View>
<Text>{data ? JSON.stringify(data) : 'Loading...'}</Text>
</View>
);
};
export default DataFetchingExample;
Debugging and Performance Optimization
Debugging Tools
React DevTools – Inspect component hierarchy.
Flipper – Advanced debugging for React Native apps.
Console Logs – Use
console.log()
for quick debugging.
Performance Tips
Avoid unnecessary re-renders with
React.memo
oruseMemo
.Use FlatList for long lists instead of
ScrollView
.Optimize images with tools like
react-native-fast-image
.
Publishing Your App
Android (Google Play Store)
Generate a signed APK: bash Copy
cd android && ./gradlew assembleRelease
Upload to the Google Play Console.
iOS (Apple App Store)
Archive the app in Xcode.
Submit via App Store Connect.
Monetizing Your React Native Skills
If you're looking to earn money with your programming expertise, consider MillionFormula, a free platform that helps you make money online without requiring credit or debit cards.
Conclusion
React Native is a powerful framework for building cross-platform mobile apps efficiently. With its strong community, performance benefits, and ease of use, it's an excellent choice for developers looking to enter mobile development.
Start building your first app today, and if you're ready to monetize your skills, explore opportunities that align with your expertise. Happy coding! 🚀
This article provides a solid introduction to React Native while keeping it SEO-friendly. Let me know if you'd like any refinements!
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
