Typing Animation on WebPage

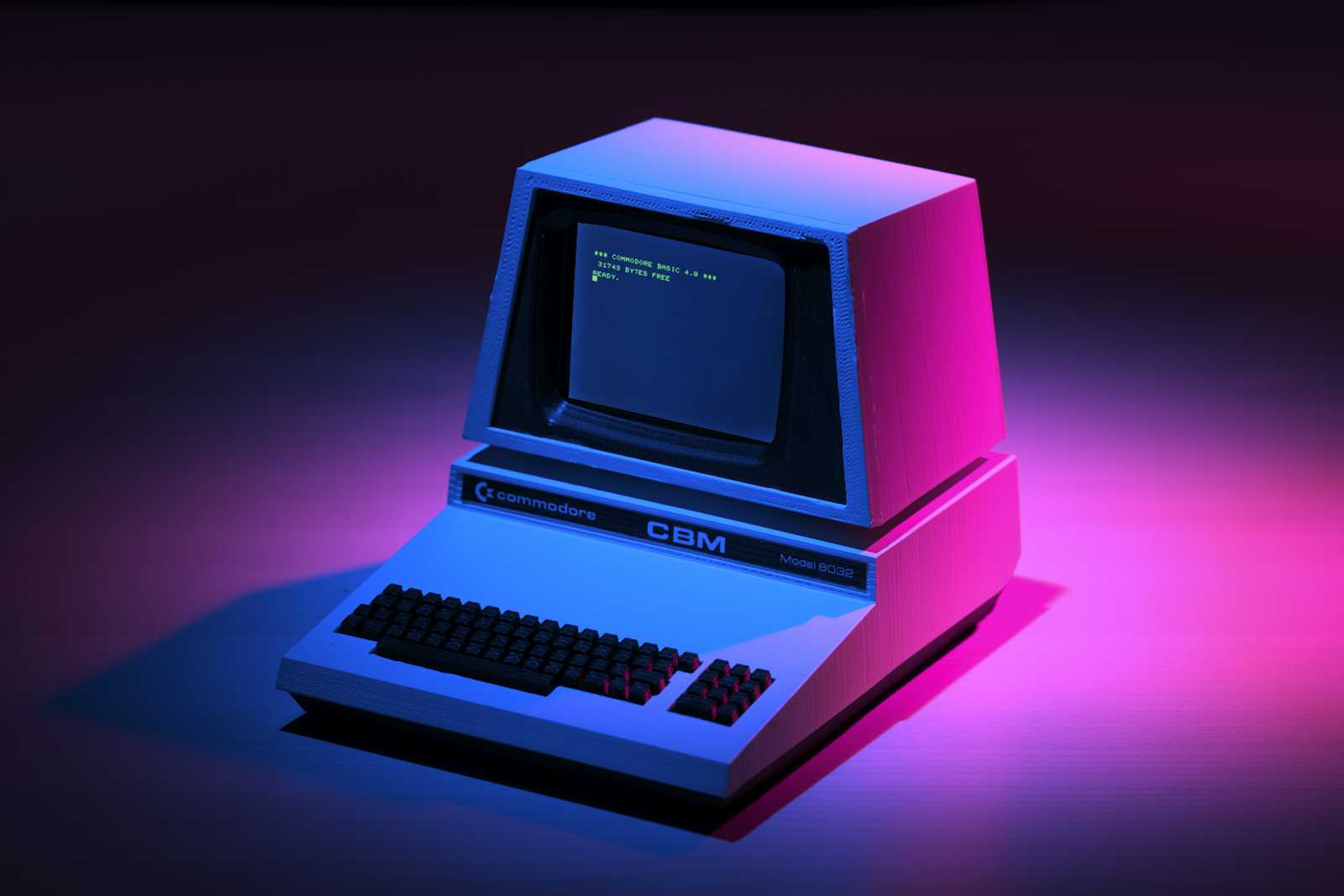
I wanted to add animation elements to my website to make it more interesting and attractive… I wanted something similar to this:
So, I started researching how to add animation to a Vite x React project. One idea was to create an actual GIF and display it, although if I needed to make any changes, I would have to recreate the GIF all over again. Another idea was to use the inbuilt CSS keyframe feature that I had used in another part of the webpage, which was the skills section.
Since I was already familiar with keyframes and I required it to only trigger when the user scrolls to a particular section, I decided to explore whether I could dynamically apply animations using React useEffect
and useRef
hooks. After some research, I came across the Intersection Observer API, which allows elements to detect when they enter or leave the viewport. This seemed like a great solution because it meant I could trigger animations only when the element became visible, improving performance and making interactions way more dynamic.
I implemented this by creating a reference (useRef
) to track the element and then used useEffect
to set up an IntersectionObserver
. The observer checked when at least 50% of the element was visible, applying the typewriter animation dynamically. If the element left the viewport, the animation was reset, ensuring it would play again the next time it appeared…
With this approach, I achieved a smooth typewriter effect without relying on static GIFs, making the animation more flexible and reusable. Plus, it aligned well with the CSS keyframe techniques I had already used, maintaining a consistent design throughout the website.
Here is the program I wrote:
const typewriterRef = useRef(null);
useEffect(() => {
// Add Intersection Observer
const observer = new IntersectionObserver(
(entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
// Add typewriter animation dynamically
const element = entry.target;
element.style.animation =
"typing 2s steps(40, end), blink 0.8s steps(40,end)";
} else {
// Reset animation when leaving the viewport
const element = entry.target;
element.style.animation = "none";
}
});
},
{ threshold: 0.5 } // Trigger when 50% of the element is visible
);
// Observe the h1 element
if (typewriterRef.current) {
observer.observe(typewriterRef.current);
}
// Cleanup the observer
return () => {
if (typewriterRef.current) {
observer.unobserve(typewriterRef.current);
}
};
}, []);
Here is the link to my final output: Typewriting Animation
Subscribe to my newsletter
Read articles from Mayur K Setty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayur K Setty
Mayur K Setty
Student at RV University x Kalvium (BCA in Software Product Engineering) | MERN Stack Dev {} | Wildlife Enthusiast | Photographer | Runner | Guitarist