Why I Use Supabase for MVPs (and beyond)
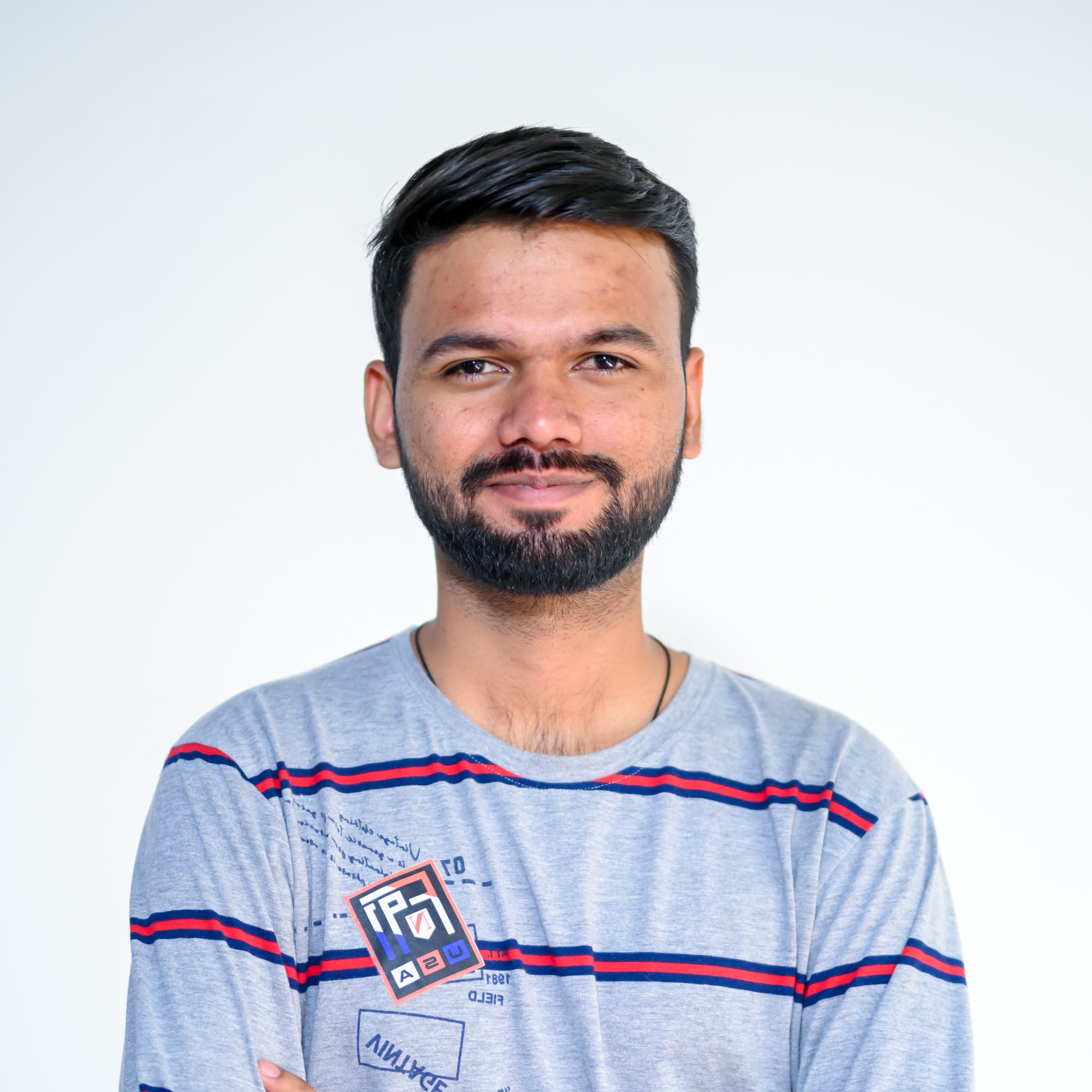
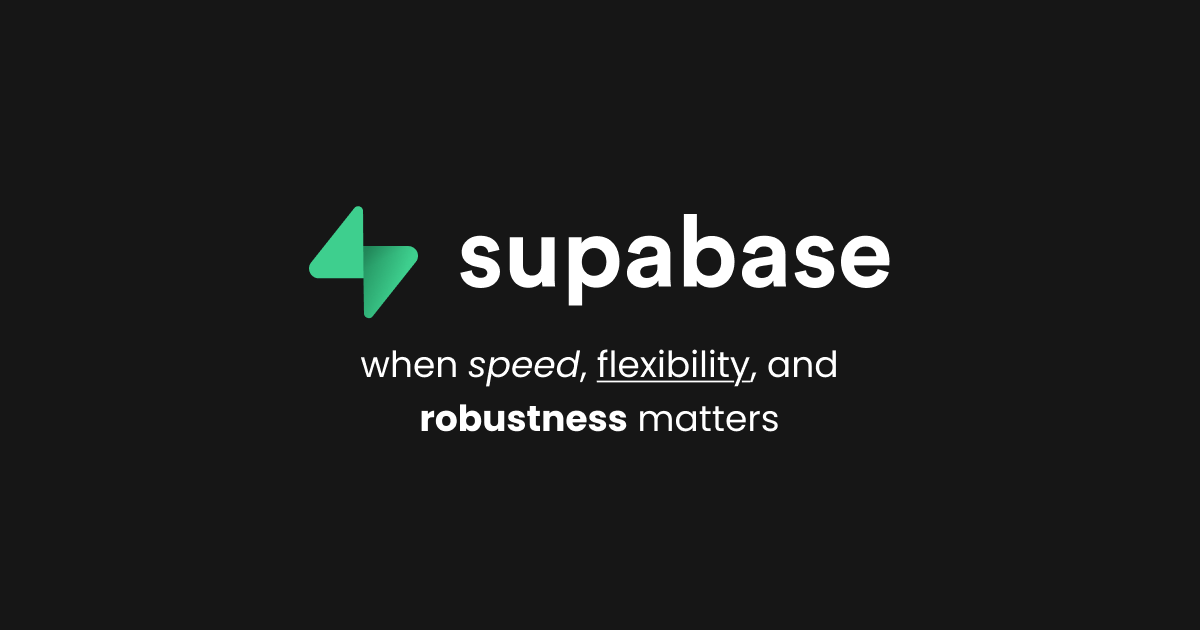
When you’re building MVPs, speed matters — but so does flexibility. You want a backend that’s fast to set up but doesn’t fall apart the moment your product grows.
Over the last couple of years, I’ve built several MVPs using Flutter for mobile and React/Next.js for web. And after trying Firebase, Hasura, and even rolling my own Express APIs, I eventually settled into a rhythm with Supabase. ✨
Here’s why.
The Real Need: Fast, Flexible, and Reliable
As a solo dev or small team, I don’t want to spend weeks managing auth flows or spinning up a custom backend just to persist a list of data.
At the same time, I also want:
Full control over data relationships
SQL-style querying
Auth with RLS (Row-Level Security)
Built-in Realtime (for live lists, chats, etc.)
A database I can export and query like a grown-up (Postgres)
Supabase hits that sweet spot.
The Stack I Usually Work With
Frontend (Web): React + Next.js
Frontend (Mobile): Flutter
Backend: Supabase (Auth + Postgres + Realtime + Edge Functions)
Deployment: Vercel (for Web), Play Store/TestFlight (for Flutter)
Let’s look at how Supabase fits into that, with a practical example.
Real Example: A Real-Time List App
Let’s say I’m building a “Live Guest List” web-app.
Anyone can add their name to a shared list.
The list updates in real time for all users.
Items expire after 7 days.
With Supabase, the backend is almost entirely done out-of-the-box.
Backend: Supabase Setup
1. Database Table
We create a guests
table in Postgres:
Column | Type |
id | UUID |
name | TEXT |
created_at | TIMESTAMP |
Set a policy to auto-delete rows after 7 days using PostgreSQL's ttl
cron or a scheduled function (Supabase supports this).
2. Row-Level Security (RLS)
We enable RLS and create a simple policy:
-- Anyone can insert
create policy "Public insert" on guests
for insert
using (true);
3. Enable Realtime
Turn on "Realtime" for the guests
table in the Supabase dashboard — this allows us to listen to any INSERT
events and push updates to clients in real time.
Frontend (Next.js) – Live List Display
Let’s walk through how data flows, using a simplified version of my actual MVP.
The core hook is useListData.js
, which:
Fetches the initial list from Supabase
Subscribes to real-time updates
Updates the UI accordingly
import { useEffect, useState } from 'react';
import { supabase } from './supabase';
export function useListData() {
const [list, setList] = useState([]);
useEffect(() => {
fetchInitial();
const sub = supabase
.channel('public:guests')
.on('postgres_changes', { event: 'INSERT', schema: 'public', table: 'guests' }, (payload) => {
setList((prev) => [payload.new, ...prev]);
})
.subscribe();
return () => {
supabase.removeChannel(sub);
};
}, []);
async function fetchInitial() {
const { data } = await supabase.from('guests').select('*').order('created_at', { ascending: false });
setList(data);
}
return list;
}
In the page.js
file (or [id].js
in Next’s route), we simply call useListData()
and map it to components like NamesList.jsx
.
The beauty is: no need to manage sockets yourself. Supabase handles it.
Here’s the full version of my MVPs data flow:
No Firebase Firestore triggers, no polling. Just clean Postgres + WebSocket events.
You can check out its GitHub and live hosted link.
Firebase vs Supabase: In the MVP World
Firebase is great for:
Fast auth integration
Push notifications (out-of-the-box with Firebase Messaging)
Small prototypes that don’t need relational querying
But here’s where it didn’t scale for my use:
No SQL: Firestore’s querying is shallow. Deep filters or joins get messy fast.
Pricing surprises: You get billed per read — real-time features spike usage.
Data modeling: Firestore pushes you into NoSQL patterns, which are harder to maintain if your schema grows.
What Supabase Gets Right
✅ Postgres, not NoSQL
I can design my schema like I would in a production app. Joins, indexes, foreign keys — everything works as expected.
✅ Row-Level Security
Secure your app directly in SQL. No extra backend logic needed.
✅ Realtime Built-In
Turn it on per table. No extra infra or Firebase-style "watchDocument" logic.
✅ One SDK, Multi-Platform
Supabase works equally well in web and mobile. You can even use it from a server or CLI tool.
✅ Edge Functions
Need to send an email or call an external API? Edge functions make it easy — no extra backend server required.
✅ Self-Hosting Option
If I need to move off Supabase for compliance or scaling, I can take the whole stack with me. It’s just Postgres + some open-source tooling.
What Could Be Better
🔸 Built-in Push Notifications
Firebase handles push with FCM. Supabase doesn’t have this out of the box — you’ll need to integrate a service like OneSignal or Firebase Cloud Messaging separately.
🔸 Docs for Advanced Features
Sometimes edge cases (especially around auth + RLS + Realtime) need digging through GitHub issues to fully understand.
Final Thoughts
Supabase is not just a Firebase alternative — it’s a solid choice in its own right, especially if you come from a SQL background or want more control over your backend.
For MVPs, it removes the need to spin up a server or write repetitive boilerplate just to handle auth or data.
For production apps, it’s robust enough to grow with you — and flexible enough to take with you if you ever need to scale out.
If you're building apps with Flutter or React/Next.js and want a backend that handles real-time data, secure auth, and doesn’t get in your way — Supabase is worth your time.
Thank you
Did you reach the bottom? Thank you for reading!
Feel free to connect with me. 👋
Subscribe to my newsletter
Read articles from Anant Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
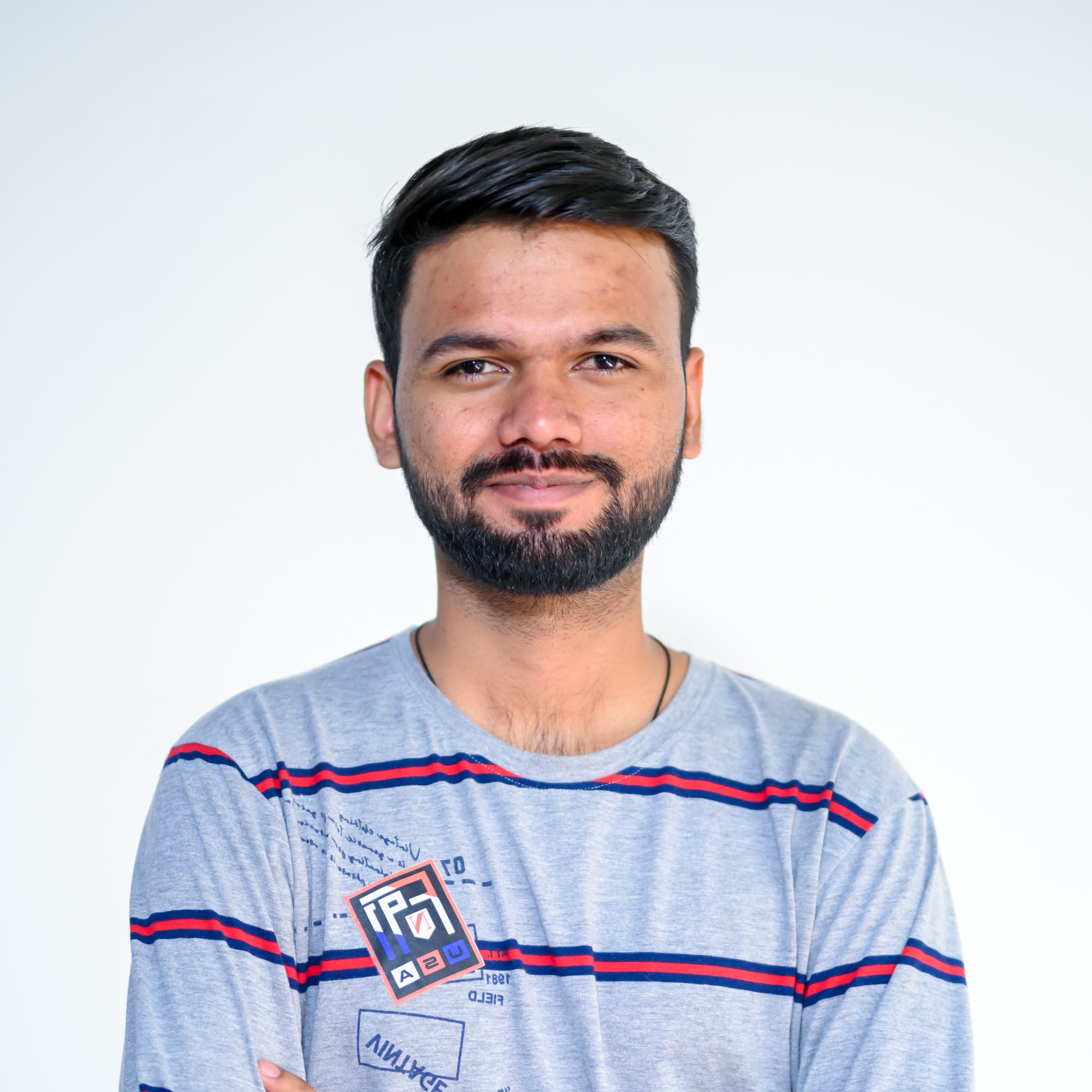
Anant Dubey
Anant Dubey
I'm Anant Dubey, a passionate software developer with experience in web and mobile applications. I specialize in native Android development using Java and Kotlin, designing intuitive user interfaces. Through my Hashnode profile, I share my knowledge on software development and cloud computing. I believe in open-source software and actively contribute to various projects on GitHub. In my free time, I enjoy reading, gaming, and playing sports, particularly cricket and chess.