Are dart extension methods an example of open closed principle (OCP)?

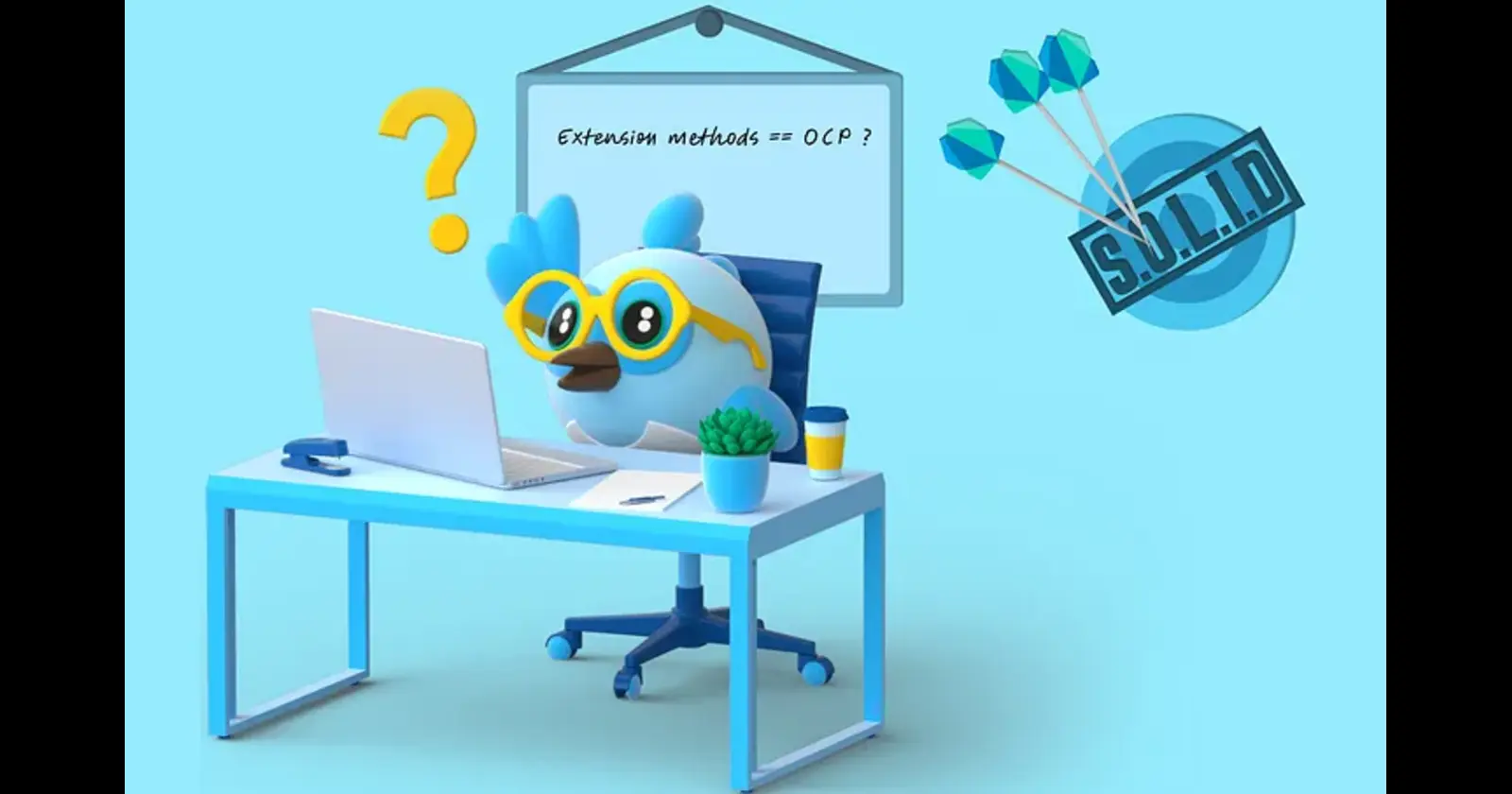
Many-a-times, we come across the terms in software engineering, and we try to relate them with the language features. One such term is the open-closed principle. The open closed principle is one of the SOLID principles, which Bertrand Meyer defines in his book Object Oriented Software Construction. The open-closed principle states that a class should be open for extension but closed for modification. Along similar lines, the extension methods in Dart are a way to add functionality to a class without modifying the class. So people might club these two things together and use them interchangeably. Let’s see, whether the dart extension methods are an example of the open closed principle.
Let’s see what are the dart extension methods first:
Dart extension methods are a way to add functionality to a class without modifying the class.
extension NumberParsing on String {
int parseInt() {
return int.parse(this);
}
}
The above extension can be used as follows:
void main() {
String number = '42';
print(number.parseInt());
}
Suppose you did not have the extension methods support in dart, you would write a utility class to parse the number as follows:
class NumberParsing {
static int parseInt(String number) {
return int.parse(number);
}
}
or non static method depending on the use case:
class NumberParsing {
int parseInt(String number) {
return int.parse(number);
}
}
So if you see what extension methods are doing, they add syntactic sugar to make the code more readable and maintainable. When you use an extension method on a type, you already get that type as an argument in the method. In the above example, when you call number.parseInt()
, the number
is already passed as an argument to the parseInt
method. So, you don't have to pass the type as an argument to the method, which makes the code more readable and maintainable.
Now let’s understand the open-closed principle:
In 1988 Bertrand Meyer defined one of the most important software engineering principles. The Open Closed Principle (OCP). In his book Object Oriented Software Construction he said:
“A satisfactory modular decomposition technique must satisfy one more requirement: It should yield modules that are both open and closed.
A module will be said to be open if it is available for extension. For example, it should be possible to add fields to the data structures it contains, or new elements to the set of functions it performs.
A module will be said to be closed if is available for use by other modules. This assumes that the module has been given a well-defined, stable description (the interface in the sense of information hiding). In the case of a programming language module, a closed module is one that may be compiled and stored in a library, for others to use. In the case of a design or specification module, closing a module simply means having it approved by management, adding it to the project’s official repository of accepted software items (often called the project baseline), and publishing its interface for the benefit of other module designers.”[1]
“This definition is obviously dated. Nowadays many languages don’t require that modules be compiled. And getting module specifications approved by management smacks of a waterfall mentality. Still, the essence of a great principle still shines through those old words. To wit:
You should be able to extend the behaviour of a system without having to modify that system.”[2]
According to Uncle Bob, a plugin architecture is a good example of the open-closed principle. You can add new plugins to a system without modifying the system itself.
The plugins are not just limited to the IDEs. The other systems such as an app architecture can also define the plugin architecture, and the consumers can add new plugins extending the functionality of the system as and when required.
“After all, the way you get a plugin architecture is to make sure that all dependencies inside the plugin, point at the system; and that nothing in the system points out towards the plugins. The system doesn’t know about the plugins. The plugins know about the system.”[2]
So, from the above definition, we can say that the OCP was coined around making a closed system extendable without modifying the system itself. But it involves a lot of design decisions and architecture to make it happen. It’s all about defining the baseline of the system and making sure that all the consumers can leverage the closed system’s baseline in order to extend the system. Again.. take an example of the plugin architecture, the plugins are able to extend the system because they follow the rules defined by the system.
What would “extending the functionality” look like in the context of the open-closed principle?
Consider the below scenario, a large team of mobile app developers is working on building a mobile app. There’s a framework that is written at an org level by architects, and there are different vertical teams of developers that are consuming it to write an app. The framework is locked for modification by the feature teams, only architects can modify it. But it provides apis/interfaces to add new features to the app leveraging the baseline that is defined at the framework level. One important feature provided by the framework is page rendering. Feature teams just have to define the page path and what components will reside in this path and their behavior. They don’t have any control over how the page is being rendered. One of the features that the framework provides is, that it is firing the ‘pageView’ event as soon as any new page is loaded, in order to report the analytics and to capture the user journey. The feature teams can only add the static attributes while declaring the page, and these attributes will be included in that respective page’s pageView event. They cannot add dynamic data, for ex. the data which is coming from the api. Because the framework only accepts static page-wise attributes. Now a requirement comes that the analytics team wants to capture some dynamic data in the pageView event. Since the feature teams have no control over how and when the pageView is being fired, it’s impossible to fulfill this requirement. The framework is not open for such an extension. The architect team decides that they will add an async callback mechanism in the framework so that the feature teams can add their own logic to the pageView event and also the pageView event can wait until the api data is available. If the callback is not provided, the framework will function in the old manner. This way, the feature teams can add their own logic to the pageView event, and the framework is still closed for modification. So now, while the pages are being declared, the feature teams can add their own logic to the pageView event. This can be stated as an example of how the system is made open for extension but close for modification.
Now, let’s come back to the dart extension methods. Are they an example of the open-closed principle?
Extension methods is a language feature, just to make the code more readable and maintainable. It’s not about “making the system extendable without modifying the system itself”. It’s just syntactic sugar to make the code more readable and maintainable. Yes, when you add an extension method to a class, you are “extending the functionality of the class without modifying the class”. You feel like the extension method is a native member of the class while reading it, But it’s just a helper method around the class leveraging one or more properties of the class.
Wherever you see a class, you can add an extension method to it, but the OCP is not about just extending the class functionality. It’s about defining the ‘close’ness and the ‘open’ness of the system. More specifically it’s about defining the ‘closeness’ of the system to make it ‘open’ for extension.
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.