Rounding Figures in X++
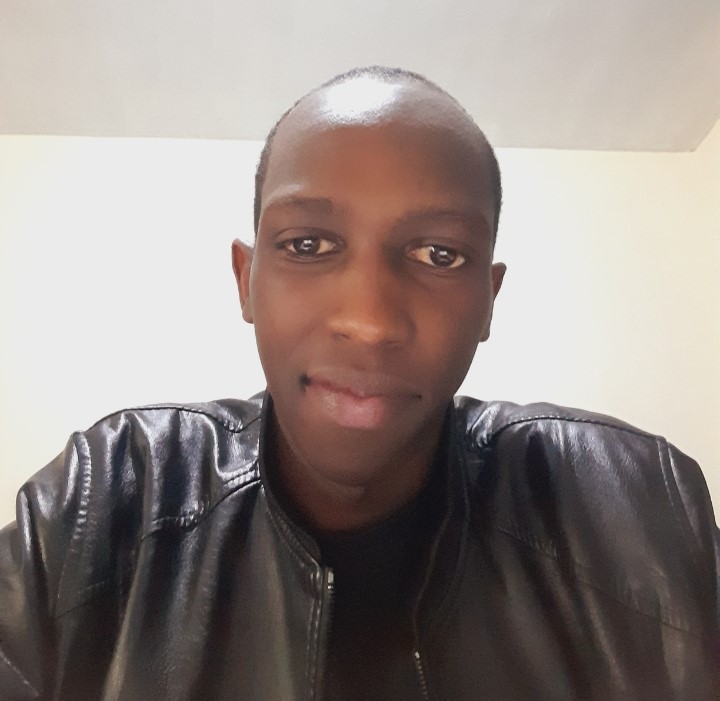
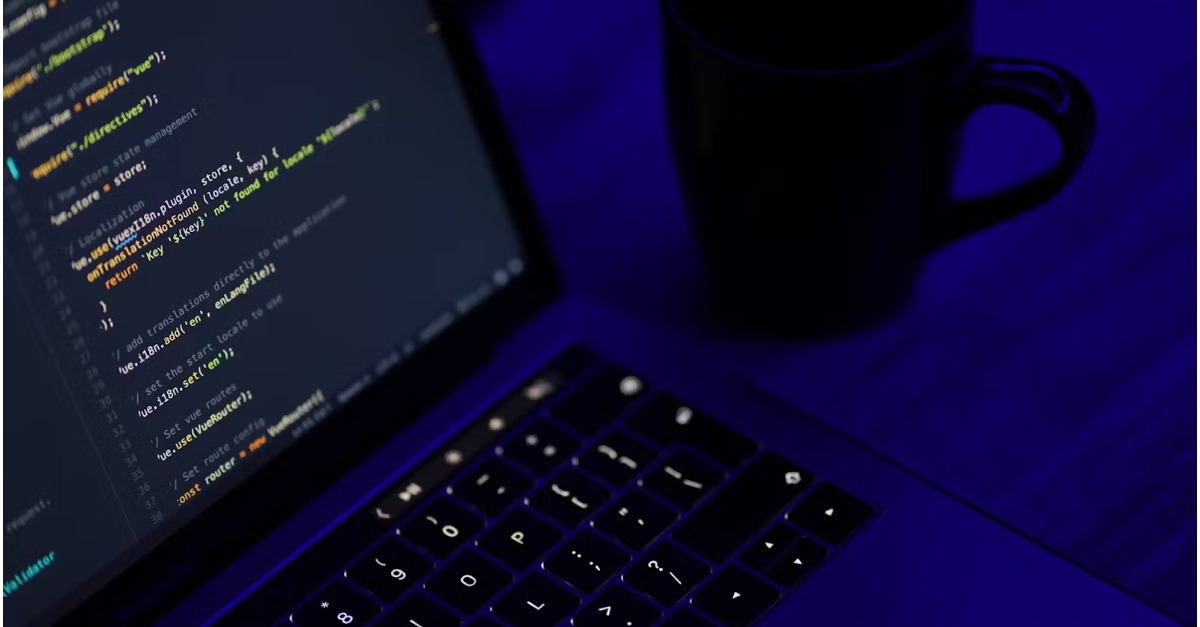
X++ provides built-in functions that round numerical values based on specific conditions. These functions allow developers to round numbers to a defined decimal place or to the nearest multiple of a specified value.
Common Rounding Functions in X++
The following rounding functions are available in X++:
round
– Rounds a number to the nearest multiple of a given value.decround
– Rounds a number to a specified number of decimal places.roundzero
– Rounds a number toward zero.rounddown
– It always rounds a number down.roundup
– It always rounds a number up.
Using the round
Function
The round
function rounds a number to the nearest multiple of the second argument.
Example:
round(1.2, 1); // Output: 1
round(1.2, 5); // Output: 0
round(6.4, 5); // Output: 5
round(7.5, 5); // Output: 10
round(1.2, 0.5); // Output: 1
round(1.12, 0.1); // Output: 1.1
📌 Key takeaway: The round
function rounds to the closest multiple of the second argument. If the value is exactly in the middle (For instance., 7.5
rounding to 5
), it rounds up to the nearest multiple.
Using the decround
Function
The decround
function rounds a number to a specified number of decimal places.
Example:
decround(1.2, 0); // Output: 1
decround(1.23, 0); // Output: 1
decround(1.23, 1); // Output: 1.2
decround(1.25, 1); // Output: 1.3
📌 Key takeaway: The decround
function is used when rounding is required at a specific decimal precision. It follows standard rounding rules where values >= 5
round up, and values < 5
round down.
Practical Use Case
A commonly used rounding method which aligns with what we were taught in schools, if the decimal value is 5 or greater, the number is rounded up; else, it is rounded down.
Example:
Amount amountCurDebit = 4056.65;
Amount amountCurCredit = 768.30;
// Applying decround
decround(amountCurDebit, 0); // Output: 4057.00
decround(amountCurCredit, 0); // Output: 768.00
Using the roundup
Function
The roundup
function always rounds a number up to the nearest multiple of the specified value, even if the number is already close to the next multiple.
Example:
roundup(1.2, 1); // Output: 2
roundup(1.2, 5); // Output: 5
roundup(6.4, 5); // Output: 10
roundup(7.5, 5); // Output: 10
roundup(1.2, 0.5); // Output: 1.5
roundup(1.12, 0.1); // Output: 1.2
📌 Key takeaway: roundup
ensures that the value always increases, it never decreases.
Using the rounddown
Function
The rounddown
function always rounds a number down to the nearest multiple of the specified value, ensuring that values always decreases after rounding.
Example:
rounddown(1.8, 1); // Output: 1
rounddown(1.8, 5); // Output: 0
rounddown(6.4, 5); // Output: 5
rounddown(7.5, 5); // Output: 5
rounddown(1.8, 0.5); // Output: 1.5
rounddown(1.88, 0.1); // Output: 1.8
📌 Key takeaway: rounddown
ensures that the value always decrease and never increases.
A Practical Use Case
When working with inventory management or financial transactions, there are scenarios where rounding up or rounding down is necessary. For example:
Rounding up is useful when calculating minimum order quantities.
Rounding down is useful when dealing with inventory levels to avoid exceeding available stock.
Example:
Amount orderQty = 2.3;
Amount availableStock = 9.7;
// Order quantity is always a whole number
roundup(orderQty, 1); // Output: 3
rounddown(availableStock, 1); // Output: 9
Conclusion
The choice of the rounding method differs based on the requirement. In this article, we’ve reviewed the common rounding methods with multiple scenarios of their application and expected output.
See Also
https://learn.microsoft.com/en-us/previous-versions/dynamics/ax-2012/reference/aa499511(v=ax.60)
https://d365ffo.com/2021/07/30/ax-d365fo-round-up-or-round-down-a-number-to-the-next-whole-number/
Subscribe to my newsletter
Read articles from Donald Kibet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
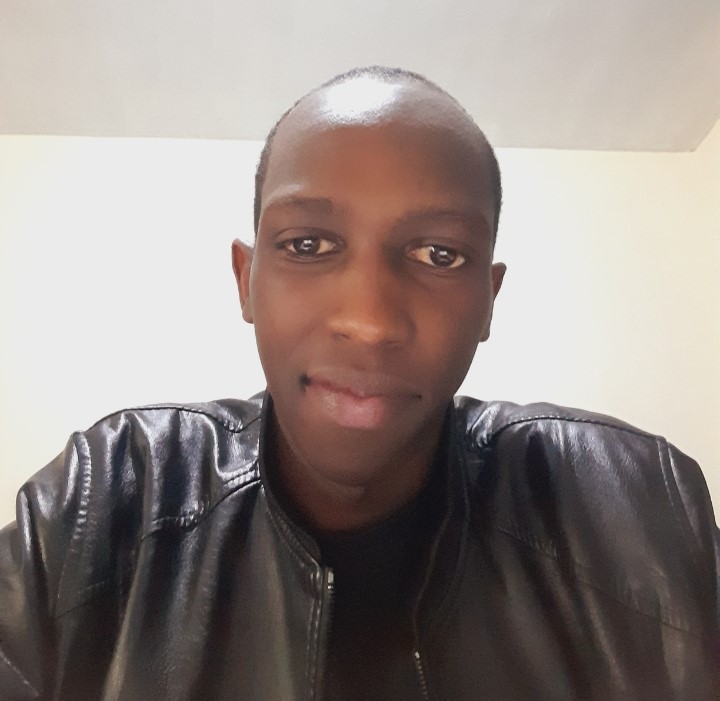
Donald Kibet
Donald Kibet
I'm a seasoned software developer specializing in customizing D365 F&O applications and creating impressive user interfaces using React and React Native for web and Android applications. Additionally, I develop secure and scalable APIs with Django (Python) or Spring Boot (Java).