Mastering OIDC: A Developer's Survival Guide to OpenID Connect
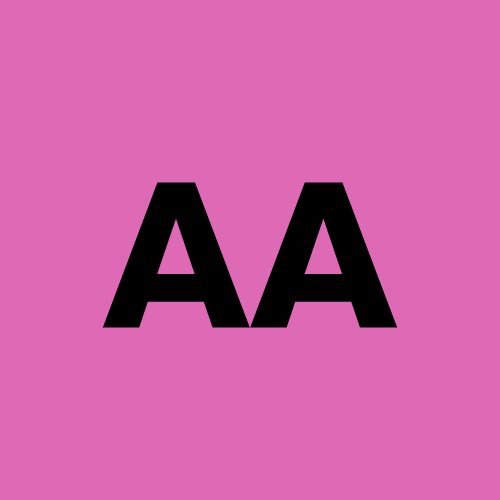
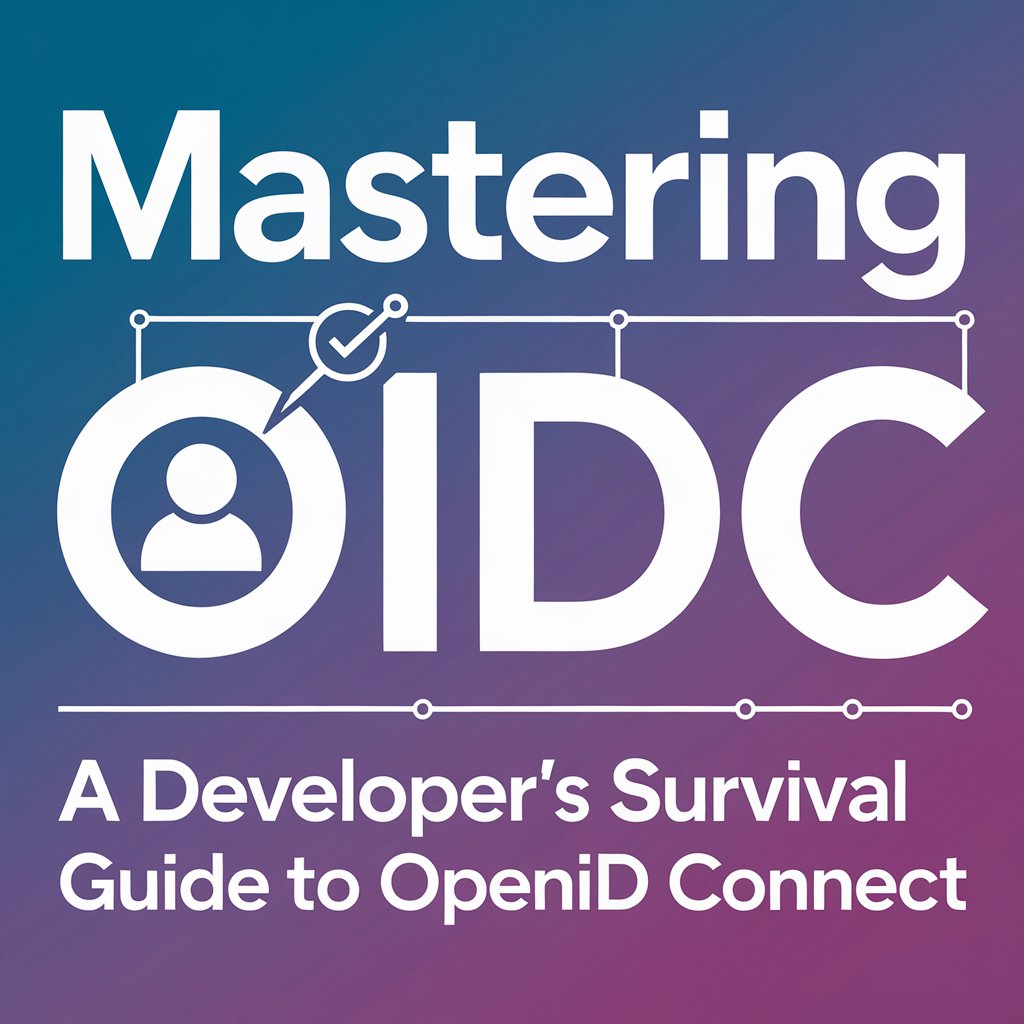
As developers, we've all faced the OIDC learning curve. The promise of secure authentication is enticing, but implementing it can feel like navigating a minefield. In this post, I'll walk you through OIDC challenges and share practical solutions - including a tool that makes testing OIDC flows simpler than ever.
The OIDC Conundrum: Why It Matters (But Often Feels Complicated)
OpenID Connect (OIDC) is the modern authentication protocol built on OAuth 2.0. While it offers significant security benefits, its implementation often leaves developers frustrated:
Complexity Overload: Multiple flows, token types, and configuration options
Security Pitfalls: Misconfigured implementations can create vulnerabilities
Debugging Nightmares: Opaque error messages and token validation issues
Integration Challenges: Making OIDC work with legacy systems
Let's break down these challenges and explore solutions.
Understanding OIDC Flows: When to Use What
Choosing the right authentication flow is critical for both security and functionality:
Authorization Code Flow (with PKCE)
Best for: Web applications
Why it's tricky: Requires state management and proper redirect URI handling
Pro tip: Always implement PKCE for added security in public clients
Client Credentials Flow
Best for: Machine-to-machine authentication
Common pitfall: Scope management and token expiration handling
Solution: Implement proper token refresh mechanisms
Implicit Flow (Now largely deprecated)
Status: Avoid in new implementations due to security concerns
Alternative: Use Authorization Code Flow with PKCE instead
Resource Owner Password Flow
When to use: Only when absolutely necessary (e.g., migrating legacy systems)
Warning: Exposes user credentials - use sparingly!
Real-World OIDC Implementation Challenges
Debugging Token Issues
One of the most frustrating aspects of OIDC is troubleshooting token problems:
Expired tokens: Check clock synchronization between client and provider
Invalid signatures: Verify JWKs endpoints are correctly configured
Scope mismatches: Ensure requested scopes match provider capabilities
Configuration Hell
OIDC providers often have:
Non-standard configuration endpoints
Different claim structures
Varying token formats
User Management Complexity
Handling user sessions across multiple providers
Managing user data synchronization
Dealing with conflicting user identities
The Developer's Secret Weapon: OIDC Tester
After struggling with these challenges myself, I discovered a game-changing tool: OIDC Tester.
This lightweight utility simplifies OIDC testing by letting you:
Quickly configure OIDC providers: Test against various implementations
Simulate user interactions: Create test users and authentication scenarios
Visualize authentication flows: See exactly where things go wrong
Validate token responses: Ensure proper signature and claim handling
Practical OIDC Testing Strategies
Here's how to leverage OIDC Tester in your workflow:
Step 1: Provider Configuration Testing
Verify discovery document endpoints
Test authorization and token endpoints
Validate JWKS endpoint responses
Step 2: Flow Simulation
Test different authentication flows
Simulate user consent scenarios
Validate redirect URI handling
Step 3: Token Validation
Check signature validation
Verify claim structure
Test token expiration handling
Step 4: Error Handling
Simulate invalid requests
Test error response handling
Validate fallback mechanisms
Code Examples: Making OIDC Work for You
Here's a practical example of implementing OIDC in a Node.js application:
JavaScriptCopy
const oidc = require('oidc-client');
const settings = {
authority: 'https://your-oidc-provider',
client_id: 'your-client-id',
redirect_uri: 'http://localhost:3000/callback',
response_type: 'code',
scope: 'openid profile email'
};
const userManager = new UserManager(settings);
async function login() {
try {
const user = await userManager.signinRedirectCallback();
console.log('User logged in:', user.profile);
} catch (error) {
console.error('Login failed:', error);
}
}
Common OIDC Pitfalls and How to Avoid Them
Ignoring token validation: Always validate signatures and claims
Hardcoding client secrets: Use environment variables instead
Skipping error handling: Implement comprehensive error catching
Overlooking security headers: Ensure proper CORS and CSRF protection
The Future of OIDC: What Developers Need to Know
As OIDC evolves, keep these trends in mind:
FAPI (Financial-grade API) compliance: Higher security standards for sensitive data
OAuth 2.1 adoption: Simplified specifications with deprecated flows
Privacy enhancements: More granular consent and data minimization
Conclusion: Take Control of Your OIDC Implementation
OIDC doesn't have to be a frustrating experience. By understanding the core concepts, leveraging tools like OIDC Tester, and following best practices, you can implement secure, reliable authentication in your applications.
Whether you're debugging token issues or testing new provider configurations, having a dedicated testing environment makes all the difference. Give OIDC Tester a try and focus more on building features rather than fighting authentication headaches!
Subscribe to my newsletter
Read articles from Andy Agarwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
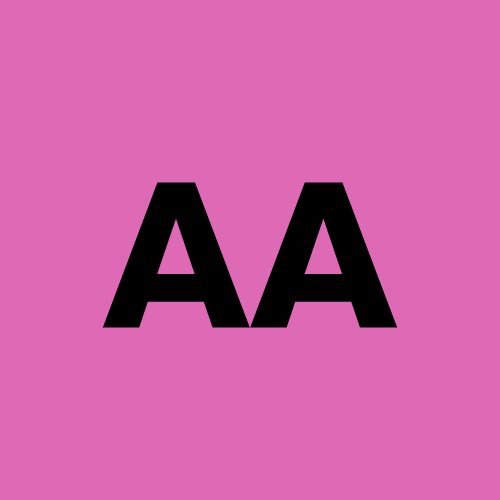