Shipment App with Java - Spring Boot

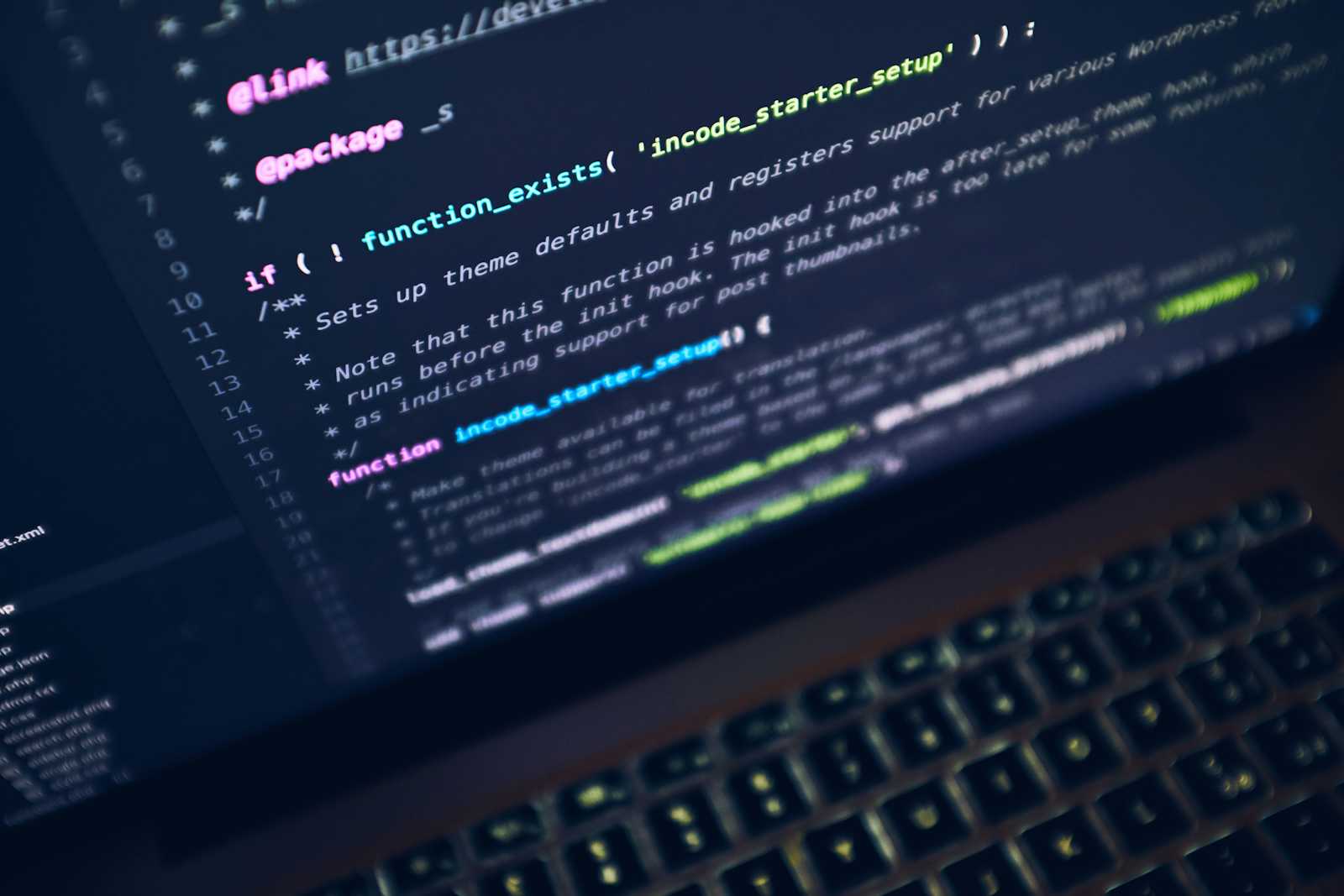
So, this is gonna be my first article on my blog. I wanna start writing down any project that I think are worth to share with people. Alright, enough with the prolog.
As the title says, this is a simple shipment app using Spring Boot, a framework based on Java language. I made this project as a simple coding test for my interview on a company. They say they needed a Backend Programmer, especially one that can utilize Java, so they test me to build a simple app. With helps of tutorials online, I finished this in a week.
I recommend checking out this video below, as it’s a complete and easy to understand tutorial that had guide me to build this app.
CRUD Operations using Spring Boot + Spring MVC + MySQL + Thymeleaf | Create, Read, Update and Delete
You can also get the code here from my Github : https://github.com/Kenjirl/shipment-app
Spring Initializr
Spring Initializr, as the name imply, is an initializer for Spring Boot project. From this web you can choose various specification for your Spring Boot app.
From the image below, the specifications are :
Project : Maven
Language : Java
Spring Boot : 3.4.4
Project Metadata :
Group : com.kencong (you can freely rename it to anything you want)
Artifact : shipment-app
Packaging : Jar
Java : 17 (version)
Then I add some dependencies that would help easily build this app, like :
Spring Web
Spring Boot Dev Tools
MySQL Driver (since I use MySQL as database)
Spring Data JPA
Validation, and
Thymeleaf (to easily manage data in HTML)
After hit “Generate”, we can then use the project after we extracted it.
Final Structure
For the reference, the final structure of the project should be like this.
| shipment-app
| - scr/main/java
| --- com.kencong.shipment-app
| ----- ShipmentAppApplication.java (main function)
|
| --- com.kencong.shipment-app.controllers
| ----- CustomersController.java
| ----- MercahntsController.java
| ----- ProductsController.java
| ----- ShipmentsController.java
|
| --- com.kencong.shipment-app.models
| ----- Customer.java
| ----- CustomerDTO.java
| ----- Merchant.java
| ----- MerchantDTO.java
| ----- Product.java
| ----- ProductDTO.java
| ----- Shipment.java
| ----- ShipmentDTO.java
|
| --- com.kencong.shipment-app.repositories
| ----- CustomersRepository.java
| ----- MerchantsRepository.java
| ----- ProductsRepository.java
| ----- ShipmentsRepository.java
|
| - scr/main/resource
| --- static
| ----- index.html
|
| --- templates
| ----- customers
| ------- create.html
| ------- detail.html
| ------- index.html
| ------- update.html
|
| ----- merchants
| ------- create.html
| ------- detail.html
| ------- index.html
| ------- update.html
|
| ----- products
| ------- create.html
| ------- detail.html
| ------- index.html
| ------- update.html
|
| ----- shipments
| ------- create.html
| ------- detail.html
| ------- index.html
| ------- update.html
|
| --- application.properties
|
...
| - public
| --- images
| ----- images for products will be stored here
| --- logo.ico
Building the App
When building this app, I recommend using IDE that are specifically for Java language, like Eclipse IDE which you can get from here.
Application Properties
After opening the project on Eclipse IDE, the first thing we wanna do is to mess with the application properties. You can find it on shipment-app > src/main/resource > application.properties
.
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/db_shipment_2
spring.datasource.username=root
spring.datasource.password=
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.servlet.multipart.max-file-size=5MB
spring.servlet.multipart.max-request-size=10MB
server.servlet.session.tracking-modes=cookie
On the code above, we define a few things, like DB connection, JPA, and some file size configuration. You can adjust the database name [db_shipment_2]
with your own. This JPA setting is so that whenever the app is saving a change, it will automatically reload the app to use the latest change that we’ve made.
Static Landing Page (Index.html)
For the main page or the first page that user will see when they access this app, you can use the index.html
that you can find on src/main/resource > static
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--color-dark-blue: #051937;
--color-light-lime: #A8EB12;
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
#storeModal input,
#storeModal textarea {
outline-color: #f97316;
}
#showModal input {
outline: none;
}
#updateModal input,
#updateModal textarea {
outline-color: #eab308;
}
</style>
<title>Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen flex flex-col items-center justify-center gap-8 font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full">
<h1 class="w-fit mx-auto text-center text-[5rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</header>
<section class="w-fit">
<a class="w-fit px-4 py-2 flex items-center justify-center gap-2 border border-orange-500 rounded-lg text-orange-500 font-semibold shadow-sm
hover:bg-orange-500 hover:text-white hover:-translate-y-2 hover:shadow-lg
focus:bg-orange-500 focus:text-white focus:-translate-y-2 focus:shadow-lg
active:bg-orange-700 active:translate-y-2 active:shadow-sm transition-all duration-[400ms]"
href="/shipments">
<i class="fa-solid fa-table-list"></i>
Show Shipment Table
</a>
</section>
</div>
</body>
</html>
I’m using Tailwind with it’s CDN here, so it might look quite messy 😅.
For the Logo on this app, you can get it here and store it on public folder like I’ve shown on the structure before.
Package - Models
Now that we have the landing page, we can move on to make the models that we need. Models here are kinda like the soon to be tables on the database. We can define variables that representing columns on the database’s table. Before that, we need to know the columns that we’re gonna need for this project.
# Customer Table
- ID
- Name (varchar)
- Phone (varchar)
- Address (varchar)
# Merchant Table
- ID
- Name (varchar)
- Phone (varchar)
- Address (varchar)
# Product Table
- ID
- Merchant ID
- Name (varchar)
- Image (varchar for it's url)
- Price (double)
- Unit (varchar)
# Shipment Table
- ID
- Customer ID
- Product ID
- Product Quantity (integer)
- Shipment Price (double)
- Total Price (double)
- Status (varchar)
- Created At (datetime, can be changed to shipped at)
- Arrived At (datetime)
Now that we had a grasp of the required tables, we need to make the model package. To do this we can just right-click on the package that contain the main Java program, for example com.kencong.shipment-app
, select new
, and then select package
. After that for model package, obviously we gonna name it models
.
In a CRUD operation in Java, there is something called DTO (Data Transfer Object). Basically, we need this DTO on Create
and Update
operation, so that only necessary data is transferred between server and client. Now, that means, for each of the models that we had, we are gonna need a DTO model along with it.
Customer & CustomerDTO
To make a Model, we can just right-click on the models
package that we just made, select new
, and then select class
. For this one, we’re gonna name it Customer
. It’ll contain all the column needed for a customer, like name, phone, and address, and also it’s going to have a relation with Shipment
model. Since each customer can have more than one shipment, the data type for Shipment
on Customer
will be List
.
To set or give value to each column we’re gonna need a Setter
, and to get the value from each column we need Getter
. We can easily make Getter and Setter for each column with right-click anywhere on the code, select Source
, and then select Generate Getters and Setters
after you done typing all the needed column. You will need to choose all the column on the generator, and then click Generate
.
package com.kencong.shipment-app.models;
import java.util.List;
import jakarta.persistence.*;
@Entity
@Table(name = "customers")
public class Customer {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private int id;
private String name;
private String phone;
private String address;
@OneToMany(mappedBy = "customer", cascade = CascadeType.ALL, orphanRemoval = true)
private List<Shipment> shipments;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
Now, we need to make the DTO for this Customer
, lets call it CustomerDTO
. On a DTO, we can define a few rules using the Validation
dependencies that we’ve installed before. This can later provide an error message for each column when users make any input that doesn’t meet the rules defined. This DTO will also need Getters
and Setters
.
package com.kencong.shipment-app.models;
import jakarta.validation.constraints.NotEmpty;
import jakarta.validation.constraints.Size;
public class CustomerDTO {
@NotEmpty(message = "Name is required")
@Size(min=3, message="Name should be at least 3 characters")
@Size(max=50, message="Name cannot exceed 50 characters")
private String name;
@NotEmpty(message = "Phone number is required")
@Size(min=12, message="Phone number should be at least 12 characters")
@Size(max=15, message="Phone number cannot exceed 15 characters")
private String phone;
@NotEmpty(message = "Address is required")
@Size(min=3, message="Address should be at least 3 characters")
@Size(max=200, message="Address cannot exceed 200 characters")
private String address;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
Merchant and MerchantDTO
Because this Merchant model is completely the same as Customer, I’ll be just paste down the code below.
package com.kencong.shipment-app.models;
import java.util.List;
import jakarta.persistence.*;
@Entity
@Table(name = "merchants")
public class Merchant {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private int id;
private String name;
private String phone;
private String address;
@OneToMany(mappedBy = "merchant")
private List<Product> products;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public List<Product> getProducts() {
return products;
}
public void setProducts(List<Product> products) {
this.products = products;
}
}
package com.kencong.shipment-app.models;
import jakarta.validation.constraints.NotEmpty;
import jakarta.validation.constraints.Size;
public class MerchantDTO {
@NotEmpty(message = "Name is required")
@Size(min=3, message="Name should be at least 3 characters")
@Size(max=50, message="Name cannot exceed 50 characters")
private String name;
@NotEmpty(message = "Phone number is required")
@Size(min=12, message="Phone number should be at least 12 characters")
@Size(max=15, message="Phone number cannot exceed 15 characters")
private String phone;
@NotEmpty(message = "Address is required")
@Size(min=3, message="Address should be at least 3 characters")
@Size(max=200, message="Address cannot exceed 200 characters")
private String address;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
Product and ProductDTO
Now this one is gonna have a slight difference, because now it has a parent table. Every product will need to have at least one and only one Merchant
. To make a foreign key, we need to define the column name, merchant_id
, and then use the model of the parent table that are being referred to, Merchant
.
package com.kencong.shipment-app.models;
import java.util.List;
import org.hibernate.annotations.OnDelete;
import org.hibernate.annotations.OnDeleteAction;
import jakarta.persistence.*;
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private int id;
@ManyToOne
@JoinColumn(name = "merchant_id", nullable = false)
@OnDelete(action = OnDeleteAction.CASCADE)
private Merchant merchant;
private String name;
private String image;
private double price;
private String unit;
@OneToMany(mappedBy = "product")
private List<Shipment> shipments;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Merchant getMerchant() {
return merchant;
}
public void setMerchant(Merchant merchant) {
this.merchant = merchant;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getImage() {
return image;
}
public void setImage(String image) {
this.image = image;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getUnit() {
return unit;
}
public void setUnit(String unit) {
this.unit = unit;
}
public List<Shipment> getShipments() {
return shipments;
}
public void setShipments(List<Shipment> shipments) {
this.shipments = shipments;
}
}
For the ProductDTO
is going to be slightly different too, because now instead of a string
of url where the image is being stored, to transfer the image data we need an actual image file to be transferred. Therefore, we’re going to use MultipartFile
as the data type of image.
package com.kencong.shipment-app.models;
import org.springframework.web.multipart.MultipartFile;
import jakarta.validation.constraints.Min;
import jakarta.validation.constraints.NotEmpty;
import jakarta.validation.constraints.NotNull;
import jakarta.validation.constraints.Size;
public class ProductDTO {
@NotNull(message = "Merchant is required")
private Merchant merchantId;
@NotEmpty(message = "Name is required")
@Size(min=3, message="Name should be at least 3 characters")
@Size(max=100, message="Name cannot exceed 100 characters")
private String name;
private MultipartFile image;
@Min(0)
private double price;
@NotEmpty(message = "Unit is required")
@Size(min=3, message="Unit should be at least 3 characters")
@Size(max=20, message="Unit cannot exceed 20 characters")
private String unit;
public Merchant getMerchantId() {
return merchantId;
}
public void setMerchantId(Merchant merchantId) {
this.merchantId = merchantId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public MultipartFile getImage() {
return image;
}
public void setImage(MultipartFile image) {
this.image = image;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getUnit() {
return unit;
}
public void setUnit(String unit) {
this.unit = unit;
}
}
Shipment and ShipmentDTO
In this Shipment
, we need 2 IDs from other models or tables.
package com.kencong.shipment-app.models;
import java.time.LocalDateTime;
import org.hibernate.annotations.OnDelete;
import org.hibernate.annotations.OnDeleteAction;
import jakarta.persistence.*;
@Entity
@Table(name = "shipments")
public class Shipment {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private int id;
@ManyToOne
@OnDelete(action = OnDeleteAction.CASCADE)
@JoinColumn(name = "customer_id", nullable = false)
private Customer customer;
@ManyToOne
@OnDelete(action = OnDeleteAction.CASCADE)
@JoinColumn(name = "product_id", nullable = false)
private Product product;
private int productQuantity;
private double shipmentPrice;
private double totalPrice;
private String status;
@Column(name = "created_at")
private LocalDateTime createdAt;
private LocalDateTime arrivedAt;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Customer getCustomer() {
return customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
}
public Product getProduct() {
return product;
}
public void setProduct(Product product) {
this.product = product;
}
public int getProductQuantity() {
return productQuantity;
}
public void setProductQuantity(int productQuantity) {
this.productQuantity = productQuantity;
}
public double getShipmentPrice() {
return shipmentPrice;
}
public void setShipmentPrice(double shipmentPrice) {
this.shipmentPrice = shipmentPrice;
}
public double getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(double totalPrice) {
this.totalPrice = totalPrice;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public LocalDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
}
public LocalDateTime getArrivedAt() {
return arrivedAt;
}
public void setArrivedAt(LocalDateTime arrivedAt) {
this.arrivedAt = arrivedAt;
}
}
package com.kencong.shipment-app.models;
import java.time.LocalDateTime;
import org.springframework.format.annotation.DateTimeFormat;
import jakarta.validation.constraints.*;
public class ShipmentDTO {
@NotNull(message = "Customer is required")
private Customer customerId;
@NotNull(message = "Product is required")
private Product productId;
@Min(1)
private int productQuantity;
@Min(0)
private double shipmentPrice;
@Min(0)
private double totalPrice;
@NotEmpty(message = "Status is required")
private String status;
@NotNull(message = "Delivery date is required")
@DateTimeFormat(pattern = "yyyy-MM-dd'T'HH:mm")
private LocalDateTime createdAt;
@NotNull(message = "Estimated arrival date is required")
@DateTimeFormat(pattern = "yyyy-MM-dd'T'HH:mm")
private LocalDateTime arrivedAt;
@AssertTrue(message = "Estimated arrival date must be after or on the same day as delivery date")
public boolean isArrivedAtValid() {
if (createdAt == null || arrivedAt == null) {
return true;
}
return !arrivedAt.isBefore(createdAt);
}
public Customer getCustomerId() {
return customerId;
}
public void setCustomerId(@NotNull(message = "Customer ID is required") Customer customerId) {
this.customerId = customerId;
}
public Product getProductId() {
return productId;
}
public void setProductId(@NotNull(message = "Product ID is required") Product productId) {
this.productId = productId;
}
public int getProductQuantity() {
return productQuantity;
}
public void setProductQuantity(int productQuantity) {
this.productQuantity = productQuantity;
}
public double getShipmentPrice() {
return shipmentPrice;
}
public void setShipmentPrice(double shipmentPrice) {
this.shipmentPrice = shipmentPrice;
}
public double getTotalPrice() {
return totalPrice;
}
public void setTotalPrice(double totalPrice) {
this.totalPrice = totalPrice;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public LocalDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(@NotNull(message = "Delivery date is required") LocalDateTime createdAt) {
this.createdAt = createdAt;
}
public @NotNull(message = "Estimated arrival date is required") LocalDateTime getArrivedAt() {
return arrivedAt;
}
public void setArrivedAt(@NotNull(message = "Estimated arrival date is required") LocalDateTime arrivedAt) {
this.arrivedAt = arrivedAt;
}
}
And that’s all for the Models
needed in this project.
Package - Repositories
A repository is an interface that provides an abstraction for data access and interaction with a database.
… so is the GPT says, we use repository to do interaction with database, whether we need to take, store, update, or delete the data from database. To make a repository, we need to first make it’s package the same way as we did to model package. Right-click on the main package, for my case it’s com.kencong.shipment-app
, select new
, and then select package
. We can name it repositories
.
CustomersRepositories
To make a Repository, we can just right-click on the repositories
package, select new
, but now we need to select interface
. For this one, we’re gonna name it CustomersRepository
. Now, because we are using JPA
dependencies, we don’t really need to type anything in this file, but we need to make sure it extends the JPARepository
. Also, JPARepository are gonna required a few “parameter like” thing, they are <Model Name, Data Type of Model’s ID>
.
package com.kencong.shipment-app.repositories;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.shipment_2.models.Customer;
public interface CustomersRepository extends JpaRepository<Customer, Integer> {
}
MerchantsRepository
package com.kencong.shipment-app.repositories;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.shipment_2.models.Merchant;
public interface MerchantsRepository extends JpaRepository<Merchant, Integer> {
}
ProductsRepository
This repository have some additional code, to provide an easier way to access a certain data. In here, we have a findByMerchant
function with the parameter of Merchant
model. As we knew earlier, that Product
needs to have it’s Merchant
. So now, we provide a function in Product
’s repository to easily access additional information about it’s Merchant
.
package com.kencong.shipment-app.repositories;
import java.util.List;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.shipment_2.models.Merchant;
import com.example.shipment_2.models.Product;
public interface ProductsRepository extends JpaRepository<Product, Integer> {
List<Product> findByMerchant(Merchant merchant);
}
ShipmentsRepository
For Shipment
’s repository we need a few additional code, since it has many parents table. We need to be able to access the Customer
, the Product
, and the Merchant
from each of the Shipment
. And, it can also goes both ways. Like when we delete a Merchant
, of course it’s gonna affect the Product
model. And if we mess with the Product
, it’s gonna affect the Shipment
model. This additional functions serve to made it easier to manage data and to made our function in controller look more organized.
package com.kencong.shipment-app.repositories;
import java.util.List;
import org.springframework.data.jpa.repository.JpaRepository;
import com.example.shipment_2.models.Customer;
import com.example.shipment_2.models.Product;
import com.example.shipment_2.models.Shipment;
public interface ShipmentsRepository extends JpaRepository<Shipment, Integer> {
List<Shipment> findByCustomer(Customer customer);
List<Shipment> findByProduct(Product product);
List<Shipment> findByProductIn(List<Product> products);
void deleteByCustomer(Customer customer);
void deleteByProduct(Product product);
}
Package - Controllers
Controller is used to control how the data shown or operate on the app. Like, when a user access a certain route or url, we can set what the user will get on this controller. For example, if user access the route /shipment
, we can set it on controller so that they will get data of Shipment
. The Repository
that we made earlier are used in these controllers.
To start it, we need to make the package for controller, by bight-click on the main package, select new
, and then select package
. We can name it controllers
.
CustomersController
After we made the controllers
package, we can make our first controller by right-clicking on the controllers
package, select new
, then select class
, and let’s name it CustomersController
.
A few things that we need to know when making a controller, we need to add @Controller
and @RequestMapping("/customers")
right before the main public class
. Using @RequestMapping("/customers")
means that any action or function in this controller will be accessed via route /customers
and then it can be followed by anything, like /create
, /edit
, etc.
To use a repository, we need to add @Autowired
before each of the repository that we use in the controller, then followed by the name of the repository, and the variable name (like how we wanna call it in this controller).
Then, we need to make each CRUD function in this controller. Usually, the basic function are gonna be an index
to show all data in a list, create
to show a form to add new data, store
to save those new data to database, show detail
to show the detail of a certain selected data, edit
to show a form to made an update on data, update
to save the changes, and delete
to remove the data from database.
For the route of each function, we need to define it with either @GetMapping
or @PostMapping
. @GetMapping
is used for a route that will need to retrieve data from database or just basically to show a page to user, like for /
, /detail/{id}
, /create
, and /update/{id}
. @PostMapping
is used on a form route, like when we want to store, make some update, or delete data from database. In this case, the routes are /create
, /update/{id}
, and /delete/{id}
.
The {id}
in those routes are used to determine which data will be affected by the action.
Now, after some code to fetch data from database, we need to send those data to user end. To do this, we need Model
from Spring Boot. We can use Model
’s function addAttribute()
, followed by the name of the attribute that we going to send to user and the name of the variable use to fetch the data.
The last important thing in this function is, because this isn’t an API, we need to show a page to user along with the data that we want to provide to user. All these function below is String
type because it needs a string value that determine which page are going to be shown to user when the function is executed. For example, the showCustomerList()
returns "customers/index"
. This means to show the customer an index.html file that are stored in src/main/resources/ > templates > customers
.
package com.example.shipment_2.controllers;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.example.shipment_2.models.Customer;
import com.example.shipment_2.models.CustomerDTO;
import com.example.shipment_2.models.Shipment;
import com.example.shipment_2.repositories.CustomersRepository;
import com.example.shipment_2.repositories.ShipmentsRepository;
import jakarta.transaction.Transactional;
import jakarta.validation.Valid;
@Controller
@RequestMapping("/customers")
public class CustomersController {
@Autowired
private CustomersRepository repo;
@Autowired
private ShipmentsRepository shipmentRepo;
@GetMapping({"", "/"})
public String showCustomerList(Model model) {
List<Customer> customers = repo.findAll();
model.addAttribute("customers", customers);
return "customers/index";
}
@GetMapping("/detail/{id}")
public String showCustomerDetail(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Customer customer = repo.findById(id).get();
List<Shipment> shipments = shipmentRepo.findByCustomer(customer);
model.addAttribute("customer", customer);
model.addAttribute("shipments", shipments);
return "customers/detail";
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Customer not found!");
return "redirect:/customers";
}
}
@GetMapping("/create")
public String createCustomerPage(Model model) {
CustomerDTO customerDTO = new CustomerDTO();
model.addAttribute("customerDTO", customerDTO);
return "customers/create";
}
@PostMapping("/create")
public String createCustomer(
@Valid @ModelAttribute CustomerDTO customerDTO,
BindingResult result, RedirectAttributes redirectAttributes
) {
Customer customer = new Customer();
customer.setName(customerDTO.getName());
customer.setPhone(customerDTO.getPhone());
customer.setAddress(customerDTO.getAddress());
repo.save(customer);
redirectAttributes.addFlashAttribute("successMessage", "New customer has been added!");
return "redirect:/customers";
}
@GetMapping("/update/{id}")
public String updateCustomerPage(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Customer customer = repo.findById(id).get();
CustomerDTO customerDTO = new CustomerDTO();
customerDTO.setName(customer.getName());
customerDTO.setPhone(customer.getPhone());
customerDTO.setAddress(customer.getAddress());
model.addAttribute("customer", customer);
model.addAttribute("customerDTO", customerDTO);
return "customers/update";
} catch (Exception e){
redirectAttributes.addFlashAttribute("errorMessage", "Customer not found!");
return "redirect:/customers";
}
}
@PostMapping("/update/{id}")
public String updateCustomer(Model model, @PathVariable("id") int id, RedirectAttributes redirectAttributes, @Valid @ModelAttribute CustomerDTO customerDTO, BindingResult result) {
try {
Customer customer = repo.findById(id).get();
model.addAttribute("customer", customer);
if (result.hasErrors()) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update customer information!");
return "redirect:/customers";
}
customer.setName(customerDTO.getName());
customer.setPhone(customerDTO.getPhone());
customer.setAddress(customerDTO.getAddress());
repo.save(customer);
redirectAttributes.addFlashAttribute("successMessage", "Customer information updated!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update customer information!");
}
return "redirect:/customers";
}
@PostMapping("/delete/{id}")
@Transactional
public String deleteCustomer(@PathVariable("id") int id, RedirectAttributes redirectAttributes) {
try {
Optional<Customer> customerOpt = repo.findById(id);
if (customerOpt .isPresent()) {
Customer customer = customerOpt .get();
shipmentRepo.deleteByCustomer(customer);
repo.delete(customer);
redirectAttributes.addFlashAttribute("successMessage", "Customer and related shipments deleted!");
} else {
redirectAttributes.addFlashAttribute("errorMessage", "Customer not found!");
}
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Failed to delete customer information!");
}
return "redirect:/customers";
}
}
This CustomerController
need ShipmentsRepository
for whenever the user wants to check the detail of any customer’s shipment, and for when a customer is deleted, all the shipment that contains ID of the customer will be deleted too.
A few addition here in my code will be RedirectAttributes
, which I like to use to send like an alert whenever an action is successfully done or if it has an error during the process. If any of the action is success, I want to send a success alert to user’s end. The attribute is successMessage
followed by it’s message. Otherwise, I wanna send an errorMessage
attribute followed by what the error is.
MerchantsController
package com.example.shipment_2.controllers;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.example.shipment_2.models.Merchant;
import com.example.shipment_2.models.MerchantDTO;
import com.example.shipment_2.models.Product;
import com.example.shipment_2.models.Shipment;
import com.example.shipment_2.repositories.MerchantsRepository;
import com.example.shipment_2.repositories.ProductsRepository;
import com.example.shipment_2.repositories.ShipmentsRepository;
import jakarta.transaction.Transactional;
import jakarta.validation.Valid;
@Controller
@RequestMapping("/merchants")
public class MerchantsController {
@Autowired
private MerchantsRepository repo;
@Autowired
private ProductsRepository productRepo;
@Autowired
private ShipmentsRepository shipmentRepo;
@GetMapping({"", "/"})
public String showMerchantList(Model model) {
List<Merchant> merchants = repo.findAll();
model.addAttribute("merchants", merchants);
return "merchants/index";
}
@GetMapping("/detail/{id}")
public String showMerchantDetail(@PathVariable("id") int id, @RequestParam(value = "tab", defaultValue = "products") String tab, RedirectAttributes redirectAttributes, Model model) {
try {
if (!tab.equals("products") && !tab.equals("shipments")) {
tab = "products";
}
model.addAttribute("activeTab", tab);
Merchant merchant = repo.findById(id).get();
List<Product> products = productRepo.findByMerchant(merchant);
List<Shipment> shipments = products.isEmpty() ? new ArrayList<>() : shipmentRepo.findByProductIn(products);
model.addAttribute("merchant", merchant);
model.addAttribute("products", products);
model.addAttribute("shipments", shipments);
return "merchants/detail";
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Merchant not found!");
return "merchants/detail";
}
}
@GetMapping("/create")
public String createMerchantPage(Model model) {
MerchantDTO merchantDTO = new MerchantDTO();
model.addAttribute("merchantDTO", merchantDTO);
return "merchants/create";
}
@PostMapping("/create")
public String createMerchant(
@Valid @ModelAttribute MerchantDTO merchantDTO,
BindingResult result, RedirectAttributes redirectAttributes
) {
Merchant merchant = new Merchant();
merchant.setName(merchantDTO.getName());
merchant.setPhone(merchantDTO.getPhone());
merchant.setAddress(merchantDTO.getAddress());
repo.save(merchant);
redirectAttributes.addFlashAttribute("successMessage", "New merchant has been added!");
return "redirect:/merchants";
}
@GetMapping("/update/{id}")
public String updateMerchantPage(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Merchant merchant = repo.findById(id).get();
MerchantDTO merchantDTO = new MerchantDTO();
merchantDTO.setName(merchant.getName());
merchantDTO.setPhone(merchant.getPhone());
merchantDTO.setAddress(merchant.getAddress());
model.addAttribute("merchant", merchant);
model.addAttribute("merchantDTO", merchantDTO);
return "merchants/update";
} catch (Exception e){
redirectAttributes.addFlashAttribute("errorMessage", "Merchant not found!");
return "redirect:/merchants";
}
}
@PostMapping("/update/{id}")
public String updateMerchant(Model model, @PathVariable("id") int id, RedirectAttributes redirectAttributes, @Valid @ModelAttribute MerchantDTO merchantDTO, BindingResult result) {
try {
Merchant merchant = repo.findById(id).get();
model.addAttribute("merchant", merchant);
if (result.hasErrors()) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update merchant information!");
return "redirect:/merchants";
}
merchant.setName(merchantDTO.getName());
merchant.setPhone(merchantDTO.getPhone());
merchant.setAddress(merchantDTO.getAddress());
repo.save(merchant);
redirectAttributes.addFlashAttribute("successMessage", "Merchant information updated!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update merchant information!");
}
return "redirect:/merchants";
}
@PostMapping("/delete/{id}")
@Transactional
public String deleteMerchant(@PathVariable("id") int id, RedirectAttributes redirectAttributes) {
try {
Optional<Merchant> merchantOpt = repo.findById(id);
if (merchantOpt .isPresent()) {
Merchant merchant = merchantOpt .get();
List<Product> products = productRepo.findByMerchant(merchant);
if (!products.isEmpty()) {
List<Shipment> shipments = shipmentRepo.findByProductIn(products);
shipmentRepo.deleteAll(shipments);
productRepo.deleteAll(products);
}
repo.delete(merchant);
redirectAttributes.addFlashAttribute("successMessage", "Merchant with it's related products and shipments deleted!");
} else {
redirectAttributes.addFlashAttribute("errorMessage", "Merchant not found!");
}
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Failed to delete merchant information!");
}
return "redirect:/merchants";
}
}
This MerchantsController
need ProductsRepository
and ShipmentsRepository
for whenever the user wants to check the detail of any merchant, and for when a merchant is deleted, all the product and shipment that linked to them will be deleted too.
ProductsController
package com.example.shipment_2.controllers;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.util.Date;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.validation.FieldError;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.example.shipment_2.models.Merchant;
import com.example.shipment_2.models.Product;
import com.example.shipment_2.models.ProductDTO;
import com.example.shipment_2.models.Shipment;
import com.example.shipment_2.repositories.MerchantsRepository;
import com.example.shipment_2.repositories.ProductsRepository;
import com.example.shipment_2.repositories.ShipmentsRepository;
import jakarta.transaction.Transactional;
import jakarta.validation.Valid;
@Controller
@RequestMapping("/products")
public class ProductsController {
@Autowired
private ProductsRepository repo;
@Autowired
private ShipmentsRepository shipmentRepo;
@Autowired
private MerchantsRepository merchantRepo;
@GetMapping({"", "/"})
public String showProductList(Model model) {
List<Product> products = repo.findAll();
model.addAttribute("products", products);
return "products/index";
}
@GetMapping("/detail/{id}")
public String showProductDetail(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Product product = repo.findById(id).get();
List<Shipment> shipments = shipmentRepo.findByProduct(product);
model.addAttribute("product", product);
model.addAttribute("shipments", shipments);
return "products/detail";
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Product not found!");
return "redirect:/products";
}
}
@GetMapping("/create")
public String createProductPage(Model model) {
ProductDTO productDTO = new ProductDTO();
List<Merchant> merchants = merchantRepo.findAll(Sort.by(Sort.Direction.ASC, "name"));
model.addAttribute("productDTO", productDTO);
model.addAttribute("merchants", merchants);
return "products/create";
}
@PostMapping("/create")
public String createProduct(
@Valid @ModelAttribute ProductDTO productDTO,
BindingResult result, RedirectAttributes redirectAttributes
) {
if (productDTO.getImage().isEmpty()) {
result.addError(new FieldError("productDTO", "image", "The product image file is required"));
}
if (result.hasErrors()) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to store product!");
return "products/index";
}
MultipartFile image = productDTO.getImage();
Date created_at = new Date();
String storageFileName = created_at.getTime() + "_" + image.getOriginalFilename();
try {
String uploadDir = "public/images/";
Path uploadPath = Paths.get(uploadDir);
if (!Files.exists(uploadPath)) {
Files.createDirectories(uploadPath);
}
try (InputStream inputStream = image.getInputStream()) {
Files.copy(inputStream, Paths.get(uploadDir + storageFileName),
StandardCopyOption.REPLACE_EXISTING);
}
} catch (Exception ex) {
System.out.println("Exception: " + ex.getMessage());
}
Product product = new Product();
product.setMerchant(productDTO.getMerchantId());
product.setName(productDTO.getName());
product.setImage(storageFileName);
product.setPrice(productDTO.getPrice());
product.setUnit(productDTO.getUnit());
repo.save(product);
redirectAttributes.addFlashAttribute("successMessage", "New product has been added!");
return "redirect:/products";
}
@GetMapping("/update/{id}")
public String showUpdatePage(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Product product = repo.findById(id).get();
ProductDTO productDTO = new ProductDTO();
List<Merchant> merchants = merchantRepo.findAll(Sort.by(Sort.Direction.ASC, "name"));
productDTO.setMerchantId(product.getMerchant());
productDTO.setName(product.getName());
productDTO.setPrice(product.getPrice());
productDTO.setUnit(product.getUnit());
model.addAttribute("product", product);
model.addAttribute("merchants", merchants);
model.addAttribute("productDTO", productDTO);
return "products/update";
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Product not found!");
return "redirect:/products";
}
}
@PostMapping("/update/{id}")
public String updateProduct(@PathVariable("id") int id, @Valid @ModelAttribute ProductDTO productDTO, Model model, BindingResult result, RedirectAttributes redirectAttributes) {
try {
Product product = repo.findById(id).get();
model.addAttribute("product", product);
if (result.hasErrors()) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to store product!");
return "products/index";
}
if (!productDTO.getImage().isEmpty()) {
String uploadDir = "public/images/";
Path oldImagePath = Paths.get(uploadDir + product.getImage());
try {
Files.delete(oldImagePath);
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
MultipartFile image = productDTO.getImage();
Date created_at = new Date();
String storageFileName = created_at.getTime() + "_" + image.getOriginalFilename();
try (InputStream inputStream = image.getInputStream()) {
Files.copy(inputStream, Paths.get(uploadDir + storageFileName),
StandardCopyOption.REPLACE_EXISTING);
}
product.setImage(storageFileName);
}
product.setMerchant(productDTO.getMerchantId());
product.setName(productDTO.getName());
product.setPrice(productDTO.getPrice());
product.setUnit(productDTO.getUnit());
repo.save(product);
redirectAttributes.addFlashAttribute("successMessage", "Product information has been updated!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("successMessage", "Fail to update product information!");
}
return "redirect:/products";
}
@PostMapping("/delete/{id}")
@Transactional
public String deleteProduct(@PathVariable("id") int id, RedirectAttributes redirectAttributes) {
try {
Optional<Product> productOpt = repo.findById(id);
if (productOpt .isPresent()) {
Product product = productOpt.get();
shipmentRepo.deleteByProduct(product);
Path imagePath = Paths.get("public/images/" + product.getImage());
try {
Files.delete(imagePath);
} catch (Exception e) {
System.out.println("Exception: " + e.getMessage());
}
repo.delete(product);
redirectAttributes.addFlashAttribute("successMessage", "Product and related shipments deleted!");
} else {
redirectAttributes.addFlashAttribute("errorMessage", "Product not found!");
}
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Failed to delete product information!");
}
return "redirect:/products";
}
}
This ProductsController
need ShipmentsRepository
for whenever the user wants to check the detail of any product’s shipment, and for when a product is deleted, all the shipment that contains it’s ID will be deleted too.
A few addition code in this ProductsController
will be the flow to store the product image whenever we store a new product, update product information, or when the product is deleted. The product pictures will be stored in public/images/
, and the name of each picture will be the timestamp when they are being stored + it’s default name.
MerchantsController
package com.example.shipment_2.controllers;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Sort;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.example.shipment_2.models.Customer;
import com.example.shipment_2.models.Product;
import com.example.shipment_2.models.Shipment;
import com.example.shipment_2.models.ShipmentDTO;
import com.example.shipment_2.repositories.CustomersRepository;
import com.example.shipment_2.repositories.ProductsRepository;
import com.example.shipment_2.repositories.ShipmentsRepository;
import jakarta.validation.Valid;
@Controller
@RequestMapping("/shipments")
public class ShipmentsController {
@Autowired
private ShipmentsRepository repo;
@Autowired
private CustomersRepository customerRepo;
@Autowired
private ProductsRepository productRepo;
@GetMapping({"", "/"})
public String showShipmentList(Model model) {
List<Shipment> shipments = repo.findAll(Sort.by(Sort.Direction.DESC, "createdAt"));
model.addAttribute("shipments", shipments);
return "shipments/index";
}
@GetMapping("/detail/{id}")
public String showShipmentDetail(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
Optional<Shipment> shipment = repo.findById(id);
if (shipment.isPresent()) {
model.addAttribute("shipment", shipment.get());
return "shipments/detail";
} else {
redirectAttributes.addFlashAttribute("errorMessage", "Shipment not found!");
return "redirect:/shipments";
}
}
@GetMapping("/create")
public String createShipmentPage(Model model) {
ShipmentDTO shipmentDTO = new ShipmentDTO();
List<Customer> customers = customerRepo.findAll(Sort.by(Sort.Direction.ASC, "name"));
List<Product> products = productRepo.findAll(Sort.by(Sort.Direction.ASC, "merchant.name", "name"));
model.addAttribute("shipmentDTO", shipmentDTO);
model.addAttribute("products", products);
model.addAttribute("customers", customers);
return "shipments/create";
}
@PostMapping("/create")
public String createShipment(
@Valid @ModelAttribute ShipmentDTO shipmentDTO,
BindingResult result, RedirectAttributes redirectAttributes
) {
Shipment shipment = new Shipment();
shipment.setProduct(shipmentDTO.getProductId());
shipment.setCustomer(shipmentDTO.getCustomerId());
shipment.setProductQuantity(shipmentDTO.getProductQuantity());
shipment.setShipmentPrice(shipmentDTO.getShipmentPrice());
shipment.setTotalPrice(shipmentDTO.getTotalPrice());
shipment.setStatus("Shipped");
shipment.setCreatedAt(shipmentDTO.getCreatedAt());
shipment.setArrivedAt(shipmentDTO.getArrivedAt());
repo.save(shipment);
redirectAttributes.addFlashAttribute("successMessage", "New delivery has been sent out!");
return "redirect:/shipments";
}
@GetMapping("/update/{id}")
public String updateShipmentPage(@PathVariable("id") int id, RedirectAttributes redirectAttributes, Model model) {
try {
Shipment shipment = repo.findById(id).get();
ShipmentDTO shipmentDTO = new ShipmentDTO();
List<Customer> customers = customerRepo.findAll(Sort.by(Sort.Direction.ASC, "name"));
List<Product> products = productRepo.findAll(Sort.by(Sort.Direction.ASC, "merchant.name", "name"));
shipmentDTO.setCustomerId(shipment.getCustomer());
shipmentDTO.setProductId(shipment.getProduct());
shipmentDTO.setProductQuantity(shipment.getProductQuantity());
shipmentDTO.setShipmentPrice(shipment.getShipmentPrice());
shipmentDTO.setStatus(shipment.getStatus());
shipmentDTO.setCreatedAt(shipment.getCreatedAt());
shipmentDTO.setArrivedAt(shipment.getArrivedAt());
model.addAttribute("shipment", shipment);
model.addAttribute("shipmentDTO", shipmentDTO);
model.addAttribute("products", products);
model.addAttribute("customers", customers);
return "shipments/update";
} catch (Exception e){
redirectAttributes.addFlashAttribute("errorMessage", "Shipment not found!");
return "redirect:/shipments";
}
}
@PostMapping("/update/{id}")
public String updateShipment(
Model model,
@PathVariable("id") int id, RedirectAttributes redirectAttributes,
@Valid @ModelAttribute ShipmentDTO shipmentDTO,
BindingResult result
) {
try {
Shipment shipment = repo.findById(id).get();
model.addAttribute("shipment", shipment);
if (result.hasErrors()) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update shipment information!");
return "redirect:/shipments";
}
shipment.setCustomer(shipmentDTO.getCustomerId());
shipment.setProduct(shipmentDTO.getProductId());
shipment.setProductQuantity(shipmentDTO.getProductQuantity());
shipment.setShipmentPrice(shipmentDTO.getShipmentPrice());
shipment.setTotalPrice((shipment.getProduct().getPrice() * shipmentDTO.getProductQuantity()) + shipmentDTO.getShipmentPrice());
shipment.setStatus(shipmentDTO.getStatus());
shipment.setCreatedAt(shipmentDTO.getCreatedAt());
shipment.setArrivedAt(shipmentDTO.getArrivedAt());
repo.save(shipment);
redirectAttributes.addFlashAttribute("successMessage", "Shipment updated!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Fail to update shipment information!");
}
return "redirect:/shipments";
}
@PostMapping("delete/{id}")
public String deleteShipment(@PathVariable("id") int id, RedirectAttributes redirectAttributes) {
try {
Shipment shipment = repo.findById(id).get();
repo.delete(shipment);
redirectAttributes.addFlashAttribute("successMessage", "Shipment deleted!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("errorMessage", "Failed to delete shipment information!");
}
return "redirect:/shipments";
}
}
This ShipmentsController
need CustomersRepository
and ProductsRepository
for whenever the user wants to check the detail of any shipment.
View
For the views, you can just make a new folder inside src/main/resources > templates
, and name it customers
, merchants
, products
, and shipments
.
Now I’m not gonna really explain the HTML code really detail, since building and styling a web page is really up to each of our creativity, am I right? :D
But I will be explaining a view code about how to show the data on page or to store data to database with Thymeleaf
dependencies.
Thymeleaf Concept
Show Data
Take a look at this code below. I had to remove the Tailwind classes since it’s quite an eyesore :D
<table>
<thead>
<tr>
<th>Name</th>
<th>Phone</th>
<th>Address</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr th:each="customer: ${customers}">
<td th:text="${customer.name}"></td>
<td th:text="${customer.phone}"></td>
<td th:text="${customer.address}"></td>
<td>
<a th:href="@{/customers/detail/{id}(id=${customer.id})}">
<i class="fa-regular fa-file-lines"></i>
</a>
<a th:href="@{/customers/update/{id}(id=${customer.id})}">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/customers/delete/' + ${customer.id}}">
<button type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
To show the data using Thymeleaf
, we need to use th:
like an attribute in our tag, and then followed by the attribute sent from controller. We can’t put it directly inside the tag, since it won’t give us the value we needed.
To make a looping based on List of our data, we can just use th:each
, followed by any name that we set for what we want to call it in this loop, and then the attribute from controller. If we want the value to be text, we can use th:text
. If we want the value to be an anchor’s link, we can use th:href
. And for a form, we can use th:action
.
Input Data using Form
<form th:object="${customerDTO}" th:action="@{/customers/create}" method="post">
<div>
<div>
<label for="name">Name</label>
<input type="text" name="name" id="name" th:field="${customerDTO.name}" placeholder="name"
minlength="3" maxlength="50" required>
<p th:if="${#fields.hasErrors('name')}" th:errors="${customerDTO.name}"></p>
</div>
<div>
<label for="phone">Phone</label>
<input type="text" name="phone" id="phone" th:field="${customerDTO.phone}" placeholder="phone"
minlength="12" maxlength="15" required>
<p th:if="${#fields.hasErrors('phone')}" th:errors="${customerDTO.phone}"></p>
</div>
<div>
<label for="address">Address</label>
<textarea name="address" id="address" placeholder="address" th:field="${customerDTO.address}"
minlength="3" maxlength="200" required></textarea>
<p th:if="${#fields.hasErrors('address')}" th:errors="${customerDTO.address}"></p>
</div>
</div>
<div>
<button type="submit">
Add New Customer
</button>
</div>
</form>
Another example will be from the from like create.html
or update.html
. Whenever we want to add or update data, we need the DTO that we’ve made earlier. On form
tag, we need to define the object of this action by using th:object
. Now, for each of the input field that representing the column in database we gonna need to use th:field
. Some additional I provided here are for whenever there is an error on user’s input, we can use th:if
and th:errors
to show the text when an error happen on each field.
Okay, I think that’s all I can explain to you about Thymeleaf
usage in this project. So for now I’d just paste all the HTML code for my project below.
Customers
Customer - Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; }
</style>
<title>Customers | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-end">
<a class="w-fit px-4 aspect-square flex items-center justify-center border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="customers/create" data-tippy-content="Add New Customer">
<i class="fa-solid fa-plus"></i>
</a>
</div>
<div class="w-full px-4">
<div class="w-full p-4 bg-white rounded-lg">
<table class="w-full stripe row-border order-column text-sm"
id="customerTable">
<thead>
<tr>
<th>Name</th>
<th>Phone</th>
<th>Address</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="customer: ${customers}" class="border-b border-slate-300">
<td th:text="${customer.name}"></td>
<td th:text="${customer.phone}"></td>
<td th:text="${customer.address}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/customers/detail/{id}(id=${customer.id})}" data-tippy-content="Show Customer">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/customers/update/{id}(id=${customer.id})}" data-tippy-content="Update Customer">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/customers/delete/' + ${customer.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Customer" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://unpkg.com/@popperjs/core@2/dist/umd/popper.min.js"></script>
<script src="https://unpkg.com/tippy.js@6/dist/tippy-bundle.umd.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let table = new DataTable('#customerTable', {
fixedColumns: {
start: 0,
end: 1
},
scrollCollapse: true,
scrollX: true
});
tippy('.action-btn');
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
<script th:if="${errorMessage}" th:inline="javascript">
Swal.fire({
icon: 'error',
title: 'Oops...',
text: [[${errorMessage}]],
});
</script>
<script th:if="${successMessage}" th:inline="javascript">
Swal.fire({
icon: 'success',
title: 'Yippiee!!',
text: [[${successMessage}]],
});
</script>
</body>
</html>
Customer - Create.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Add New Customer | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[400px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/customers">
<i class="fa-solid fa-arrow-left"></i>
Back to Customers
</a>
</div>
<div class="w-full px-4">
<div class="w-full bg-white rounded-lg">
<form th:object="${customerDTO}" th:action="@{/customers/create}" method="post">
<div class="w-full p-4">
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="name">Name</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="name" id="name" th:field="${customerDTO.name}" placeholder="name" minlength="3" maxlength="50" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${customerDTO.name}"></p>
</div>
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="phone">Phone</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="phone" id="phone" th:field="${customerDTO.phone}" placeholder="phone" minlength="12" maxlength="15" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('phone')}" th:errors="${customerDTO.phone}"></p>
</div>
<div class="w-full">
<label class="block w-full mb-2 font-semibold text-slate-300" for="address">Address</label>
<textarea class="w-full p-2 border border-slate-300 rounded-lg"
name="address" id="address" placeholder="address" th:field="${customerDTO.address}" minlength="3" maxlength="200" required
></textarea>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('address')}" th:errors="${customerDTO.address}"></p>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-full px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Add New Customer
</button>
</div>
</form>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
</body>
</html>
Customer - Detail.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Customer Detail | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-between">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/customers">
<i class="fa-solid fa-arrow-left"></i>
Back to Customers
</a>
<div class="w-fit flex items-center justify-center gap-2 text-white">
<a class="size-[41.6px] flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/customers/update/{id}(id=${customer.id})}" data-tippy-content="Update Customer">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/customers/delete/' + ${customer.id}}">
<button class="delete-btn size-[41.6px] flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Customer" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</div>
</div>
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-between gap-4 bg-white rounded-lg">
<div class="sticky top-4 flex-1 w-full max-w-[300px] flex flex-col items-end justify-normal rounded-md bg-slate-100 p-4">
<div class="w-full">
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Name</p>
<p th:text="${customer.name}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Phone</p>
<p th:text="${customer.phone}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Address</p>
<p th:text="${customer.address}" class="w-full text-lg font-semibold"></p>
</div>
</div>
</div>
<!-- SHIPMENTS -->
<div class="w-full max-w-[800px]">
<table class="w-full max-w-full stripe row-border order-column text-sm"
id="shipmentTable">
<thead>
<tr>
<th>Shipment</th>
<th>Product</th>
<th>Merchant</th>
<th>Qty</th>
<th>Total Price</th>
<th>Status</th>
<th>Estimate</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="shipment: ${shipments}" class="border-b border-slate-300">
<td th:text="${shipment.createdAt.toString().substring(0,10)}"></td>
<td th:text="${shipment.product.name}"></td>
<td th:text="${shipment.product.merchant.name}"></td>
<td th:text="${shipment.productQuantity}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.totalPrice, 0, 'POINT', 0, 'COMMA')}}" class="text-end"></td>
<td class="text-center">
<span th:text="${shipment.status}"
th:classappend="${shipment.status == 'Shipped'} ? 'text-blue-500' : (${shipment.status == 'Delivered'} ? 'text-green-500' : 'text-red-500')"
class="px-2 py-1 rounded-full text-xs font-medium">
</span>
</td>
<td th:text="${shipment.arrivedAt.toString().substring(0,10)}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/shipments/detail/{id}(id=${shipment.id})}" data-tippy-content="Show Shipment">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/shipments/update/{id}(id=${shipment.id})}" data-tippy-content="Update Shipment">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/shipments/delete/' + ${shipment.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Shipment" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let table = new DataTable('#shipmentTable', {
fixedColumns: {
start: 0,
end: 1
},
paging: false,
scrollCollapse: true,
scrollX: true
});
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
</body>
</html>
Customer - Update.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Update Customer Information | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[400px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/customers">
<i class="fa-solid fa-arrow-left"></i>
Back to Customers
</a>
</div>
<div class="w-full px-4">
<div class="w-full bg-white rounded-lg">
<form th:object="${customerDTO}" th:action="@{'/customers/update/' + ${customer.id}}" method="post">
<div class="w-full p-4">
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="name">Name</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="name" id="name" th:field="${customerDTO.name}" placeholder="name" minlength="3" maxlength="50" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${customerDTO.name}"></p>
</div>
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="phone">Phone</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="phone" id="phone" th:field="${customerDTO.phone}" placeholder="phone" minlength="12" maxlength="15" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('phone')}" th:errors="${customerDTO.phone}"></p>
</div>
<div class="w-full">
<label class="block w-full mb-2 font-semibold text-slate-300" for="address">Address</label>
<textarea class="w-full p-2 border border-slate-300 rounded-lg"
name="address" id="address" placeholder="address" th:field="${customerDTO.address}" minlength="3" maxlength="200" required
></textarea>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('address')}" th:errors="${customerDTO.address}"></p>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-full px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Update Customer Information
</button>
</div>
</form>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
</body>
</html>
Merchants
Merchant - Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; }
</style>
<title>Merchants | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-end">
<a class="w-fit px-4 aspect-square flex items-center justify-center border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="merchants/create" data-tippy-content="Add New Merchant">
<i class="fa-solid fa-plus"></i>
</a>
</div>
<div class="w-full px-4">
<div class="w-full p-4 bg-white rounded-lg">
<table class="w-full stripe row-border order-column text-sm"
id="customerTable">
<thead>
<tr>
<th>Name</th>
<th>Phone</th>
<th>Address</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="merchant: ${merchants}" class="border-b border-slate-300">
<td th:text="${merchant.name}"></td>
<td th:text="${merchant.phone}"></td>
<td th:text="${merchant.address}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/merchants/detail/{id}(id=${merchant.id})}" data-tippy-content="Show Merchant">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/merchants/update/{id}(id=${merchant.id})}" data-tippy-content="Update Merchant">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/merchants/delete/' + ${merchant.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Merchant" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://unpkg.com/@popperjs/core@2/dist/umd/popper.min.js"></script>
<script src="https://unpkg.com/tippy.js@6/dist/tippy-bundle.umd.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let table = new DataTable('#customerTable', {
fixedColumns: {
start: 0,
end: 1
},
scrollCollapse: true,
scrollX: true
});
tippy('.action-btn');
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
<script th:if="${errorMessage}" th:inline="javascript">
Swal.fire({
icon: 'error',
title: 'Oops...',
text: [[${errorMessage}]],
});
</script>
<script th:if="${successMessage}" th:inline="javascript">
Swal.fire({
icon: 'success',
title: 'Yippiee!!',
text: [[${successMessage}]],
});
</script>
</body>
</html>
Merchant - Create.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Add New Merchant | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[400px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/merchants">
<i class="fa-solid fa-arrow-left"></i>
Back to Merchants
</a>
</div>
<div class="w-full px-4">
<div class="w-full bg-white rounded-lg">
<form th:object="${merchantDTO}" th:action="@{/merchants/create}" method="post">
<div class="w-full p-4">
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="name">Name</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="name" id="name" th:field="${merchantDTO.name}" placeholder="name" minlength="3" maxlength="50" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${merchantDTO.name}"></p>
</div>
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="phone">Phone</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="phone" id="phone" th:field="${merchantDTO.phone}" placeholder="phone" minlength="12" maxlength="15" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('phone')}" th:errors="${merchantDTO.phone}"></p>
</div>
<div class="w-full">
<label class="block w-full mb-2 font-semibold text-slate-300" for="address">Address</label>
<textarea class="w-full p-2 border border-slate-300 rounded-lg"
name="address" id="address" placeholder="address" th:field="${merchantDTO.address}" minlength="3" maxlength="200" required
></textarea>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('address')}" th:errors="${merchantDTO.address}"></p>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-full px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Add New Merchant
</button>
</div>
</form>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
</body>
</html>
Merchant - Detail.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Merchant Detail | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-between">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/merchants">
<i class="fa-solid fa-arrow-left"></i>
Back to Customers
</a>
<div class="w-fit flex items-center justify-center gap-2 text-white">
<a class="size-[41.6px] flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/merchants/update/{id}(id=${merchant.id})}" data-tippy-content="Update Customer">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/merchants/delete/' + ${merchant.id}}">
<button class="delete-btn size-[41.6px] flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Customer" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</div>
</div>
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-between gap-4 bg-white rounded-lg">
<div class="sticky top-4 flex-1 w-full max-w-[300px] flex flex-col items-end justify-normal rounded-md bg-slate-100 p-4">
<div class="w-full">
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Name</p>
<p th:text="${merchant.name}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Phone</p>
<p th:text="${merchant.phone}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-500">Address</p>
<p th:text="${merchant.address}" class="w-full text-lg font-semibold"></p>
</div>
</div>
</div>
<!-- DATAS -->
<div class="w-full max-w-[800px]">
<div class="w-full flex items-center justify-normal gap-4">
<a class="block w-fit px-4 py-2 border border-orange-500 font-medium rounded-md transition-colors"
th:href="@{/merchants/detail/{id}(id=${merchant.id}, tab='products')}"
th:classappend="${activeTab == 'products'} ? 'bg-orange-500 text-white' : 'text-orange-500 hover:bg-orange-500 hover:text-white'">
Products
</a>
<a class="block w-fit px-4 py-2 border border-orange-500 font-medium rounded-md transition-colors"
th:href="@{/merchants/detail/{id}(id=${merchant.id}, tab='shipments')}"
th:classappend="${activeTab == 'shipments'} ? 'bg-orange-500 text-white' : 'text-orange-500 hover:bg-orange-500 hover:text-white'">
Shipments
</a>
</div>
<!-- PRODUCT -->
<div th:if="${activeTab == 'products'}">
<table class="w-full max-w-full stripe row-border order-column text-sm"
id="productTable">
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Unit</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="product: ${products}" class="border-b border-slate-300">
<td th:text="${product.name}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(product.price, 0, 'POINT', 0, 'COMMA')}}"></td>
<td th:text="${product.unit}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/products/detail/{id}(id=${product.id})}" data-tippy-content="Show Product">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/products/update/{id}(id=${product.id})}" data-tippy-content="Update Product">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/products/delete/' + ${product.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Product" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
<!-- SHIPMENTS -->
<div th:if="${activeTab == 'shipments'}">
<table class="w-full font-light max-w-full stripe row-border order-column text-sm"
id="shipmentTable">
<thead>
<tr>
<th>Shipment</th>
<th>Product</th>
<th>Customer</th>
<th>Qty</th>
<th>Total Price</th>
<th>Status</th>
<th>Estimate</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="shipment: ${shipments}" class="border-b border-slate-300">
<td th:text="${shipment.createdAt.toString().substring(0,10)}"></td>
<td th:text="${shipment.product.name}"></td>
<td th:text="${shipment.customer.name}"></td>
<td th:text="${shipment.productQuantity}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.totalPrice, 0, 'POINT', 0, 'COMMA')}}" class="text-end"></td>
<td class="text-center">
<span th:text="${shipment.status}"
th:classappend="${shipment.status == 'Shipped'} ? 'text-blue-500' : (${shipment.status == 'Delivered'} ? 'text-green-500' : 'text-red-500')"
class="px-2 py-1 rounded-full text-xs font-medium">
</span>
</td>
<td th:text="${shipment.arrivedAt.toString().substring(0,10)}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/shipments/detail/{id}(id=${shipment.id})}" data-tippy-content="Show Shipment">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/shipments/update/{id}(id=${shipment.id})}" data-tippy-content="Update Shipment">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/shipments/delete/' + ${shipment.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Shipment" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let productTable = new DataTable('#productTable', {
fixedColumns: {
start: 0,
end: 1
},
paging: false,
scrollCollapse: true,
scrollX: true
});
let shipmentTable = new DataTable('#shipmentTable', {
fixedColumns: {
start: 0,
end: 1
},
paging: false,
scrollCollapse: true,
scrollX: true
});
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
</body>
</html>
Merchant - Update.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Update Merchant Information | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipment
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[400px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/merchants">
<i class="fa-solid fa-arrow-left"></i>
Back to Merchants
</a>
</div>
<div class="w-full px-4">
<div class="w-full bg-white rounded-lg">
<form th:object="${merchantDTO}" th:action="@{'/merchants/update/' + ${merchant.id}}" method="post">
<div class="w-full p-4">
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="name">Name</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="name" id="name" th:field="${merchantDTO.name}" placeholder="name" minlength="3" maxlength="50" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${merchantDTO.name}"></p>
</div>
<div class="w-full mb-4">
<label class="block w-full mb-2 font-semibold text-slate-300" for="phone">Phone</label>
<input class="w-full p-2 border border-slate-300 rounded-lg"
type="text" name="phone" id="phone" th:field="${merchantDTO.phone}" placeholder="phone" minlength="12" maxlength="15" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('phone')}" th:errors="${merchantDTO.phone}"></p>
</div>
<div class="w-full">
<label class="block w-full mb-2 font-semibold text-slate-300" for="address">Address</label>
<textarea class="w-full p-2 border border-slate-300 rounded-lg"
name="address" id="address" placeholder="address" th:field="${merchantDTO.address}" minlength="3" maxlength="200" required
></textarea>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('address')}" th:errors="${merchantDTO.address}"></p>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-full px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Update Merchant Information
</button>
</div>
</form>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
</body>
</html>
Products
Product - Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; }
</style>
<title>Products | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-end">
<a class="w-fit px-4 aspect-square flex items-center justify-center border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="products/create" data-tippy-content="Add New Product">
<i class="fa-solid fa-plus"></i>
</a>
</div>
<div class="w-full px-4">
<div class="w-full p-4 bg-white rounded-lg">
<table class="w-full stripe row-border order-column text-sm"
id="productTable">
<thead>
<tr>
<th>Name</th>
<th>Merchant</th>
<th>Price</th>
<th>Unit</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="product: ${products}" class="border-b border-slate-300">
<td th:text="${product.name}"></td>
<td th:text="${product.merchant.name}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(product.price, 0, 'POINT', 0, 'COMMA')}}" class="text-end"></td>
<td th:text="${product.unit}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/products/detail/{id}(id=${product.id})}" data-tippy-content="Show Product">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/products/update/{id}(id=${product.id})}" data-tippy-content="Update Product">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/products/delete/' + ${product.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Product" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://unpkg.com/@popperjs/core@2/dist/umd/popper.min.js"></script>
<script src="https://unpkg.com/tippy.js@6/dist/tippy-bundle.umd.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let table = new DataTable('#productTable', {
fixedColumns: {
start: 0,
end: 1
},
scrollCollapse: true,
scrollX: true
});
tippy('.action-btn');
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
<script th:if="${errorMessage}" th:inline="javascript">
Swal.fire({
icon: 'error',
title: 'Oops...',
text: [[${errorMessage}]],
});
</script>
<script th:if="${successMessage}" th:inline="javascript">
Swal.fire({
icon: 'success',
title: 'Yippiee!!',
text: [[${successMessage}]],
});
</script>
</body>
</html>
Product - Create.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Add New Product | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[800px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/products">
<i class="fa-solid fa-arrow-left"></i>
Back to Products
</a>
</div>
<form method="post" enctype="multipart/form-data" th:object="${productDTO}" th:action="@{/products/create}">
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-normal gap-4 bg-white rounded-t-lg">
<!-- IMAGE -->
<div class="w-full max-w-[300px]">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
src="#" alt="no image found" id="preview_product_image">
<input class="w-full rounded-lg border border-slate-300 cursor-pointer
file:mr-2 file:bg-orange-500 file:rounded-l-md file:px-4 file:py-2 file:font-semibold file:text-white"
type="file" name="image" id="image" placeholder="choose file" accept="image/*" required th:field="${productDTO.image}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('image')}" th:errors="${productDTO.image}"></p>
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<!-- INFO -->
<div class="w-full">
<!-- MERCHANT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="merchant_id">Merchant</label>
<select name="merchant_id" id="merchant_id" th:field="${productDTO.merchantId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Merchant --</option>
<option th:each="merchant : ${merchants}"
th:value="${merchant.id}"
th:text="@{'(ID-' + ${merchant.id} + ') - ' + ${merchant.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('merchantId')}" th:errors="${productDTO.merchantId}"></p>
</div>
<!-- NAME -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="name">Name</label>
<input type="text" name="name" id="name" th:field="${productDTO.name}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="name" minlength="3" maxlength="100" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${productDTO.name}"></p>
</div>
<!-- NAME -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="price">Price</label>
<input type="number" name="price" id="price" th:field="${productDTO.price}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="price" min="0" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('price')}" th:errors="${productDTO.price}"></p>
</div>
<!-- UNIT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="unit">Unit</label>
<input type="text" name="unit" id="unit" th:field="${productDTO.unit}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="unit" minlength="3" maxlength="20" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('unit')}" th:errors="${productDTO.unit}"></p>
</div>
</div>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-fit px-4 py-2 flex items-center justify-center gap-1 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Add New Product
</button>
</div>
</div>
</form>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script>
$(document).ready(function () {
$("#image").on("change", function(event) {
var file = event.target.files[0];
if (file) {
var imageUrl = URL.createObjectURL(file);
$("#preview_product_image").attr("src", imageUrl);
}
});
});
</script>
</body>
</html>
Product - Detail.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.css"/>
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Product Detail | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[800px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-between">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/products">
<i class="fa-solid fa-arrow-left"></i>
Back to Products
</a>
<div class="w-fit flex items-center justify-center gap-2 text-white">
<a class="size-[41.6px] flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/products/update/{id}(id=${product.id})}" data-tippy-content="Update Product">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/products/delete/' + ${product.id}}">
<button class="delete-btn size-[41.6px] flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Product" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</div>
</div>
<div class="w-full px-4">
<div class="w-full p-4 bg-white rounded-lg">
<!-- DETAIL -->
<div class="w-full flex items-start justify-normal gap-4">
<!-- IMAGE -->
<div class="w-full max-w-[300px]">
<a th:href="@{'/images/'+${product.image}}" data-fancybox="product-img" th:data-caption="${product.name}" id="zoom-pic">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
th:src="@{'/images/'+${product.image}}" th:alt="${product.name}">
</a>
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<div class="w-full flex items-start justify-normal gap-2">
<div class="w-full">
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Name</p>
<p th:text="${product.name}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Price</p>
<p th:text="@{'Rp ' + ${#numbers.formatDecimal(product.price, 0, 'POINT', 0, 'COMMA')}}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Unit</p>
<p th:text="${product.unit}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Merchant</p>
<p th:text="${product.merchant.name}" class="w-full text-lg font-semibold"></p>
</div>
</div>
</div>
</div>
</div>
<div class="w-full h-[2px] my-2 rounded-full bg-slate-300"></div>
<!-- SHIPMENTS -->
<div class="w-full">
<table class="w-full max-w-full stripe row-border order-column text-sm"
id="shipmentTable">
<thead>
<tr>
<th>Shipment</th>
<th>Qty</th>
<th>Total Price</th>
<th>Status</th>
<th>Estimate</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="shipment: ${shipments}" class="border-b border-slate-300">
<td th:text="${shipment.createdAt.toString().substring(0,10)}"></td>
<td th:text="${shipment.productQuantity}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.totalPrice, 0, 'POINT', 0, 'COMMA')}}" class="text-end"></td>
<td class="text-center">
<span th:text="${shipment.status}"
th:classappend="${shipment.status == 'Shipped'} ? 'text-blue-500' : (${shipment.status == 'Delivered'} ? 'text-green-500' : 'text-red-500')"
class="px-2 py-1 rounded-full text-xs font-medium">
</span>
</td>
<td th:text="${shipment.arrivedAt.toString().substring(0,10)}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/shipments/detail/{id}(id=${shipment.id})}" data-tippy-content="Show Shipment">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/shipments/update/{id}(id=${shipment.id})}" data-tippy-content="Update Shipment">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/shipments/delete/' + ${shipment.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Shipment" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.umd.js"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
Fancybox.bind('[data-fancybox="product-img"]', {
// Your custom options for a specific gallery
});
let table = new DataTable('#shipmentTable', {
fixedColumns: {
start: 0,
end: 1
},
paging: false,
scrollCollapse: true,
scrollX: true
});
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
</body>
</html>
Product - Update.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.css"/>
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Update Product Information | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[800px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/products">
<i class="fa-solid fa-arrow-left"></i>
Back to Products
</a>
</div>
<form method="post" enctype="multipart/form-data" th:object="${productDTO}" th:action="@{'/products/update/' + ${product.id}}">
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-normal gap-4 bg-white rounded-t-lg">
<!-- IMAGE -->
<div class="w-full max-w-[300px]">
<a th:href="@{'/images/'+${product.image}}" data-fancybox="product-img" th:data-caption="${product.name}" id="zoom-pic">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
th:src="@{'/images/'+${product.image}}" th:alt="${product.name}" id="preview_product_image">
</a>
<input class="w-full rounded-lg border border-slate-300 cursor-pointer
file:mr-2 file:bg-orange-500 file:rounded-l-md file:px-4 file:py-2 file:font-semibold file:text-white"
type="file" name="image" id="image" placeholder="choose file" accept="image/*" th:field="${productDTO.image}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('image')}" th:errors="${productDTO.image}"></p>
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<!-- INFO -->
<div class="w-full">
<!-- MERCHANT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="merchant_id">Merchant</label>
<select name="merchant_id" id="merchant_id" th:field="${productDTO.merchantId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Merchant --</option>
<option th:each="merchant : ${merchants}"
th:value="${merchant.id}"
th:text="@{'(ID-' + ${merchant.id} + ') - ' + ${merchant.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('merchantId')}" th:errors="${productDTO.merchantId}"></p>
</div>
<!-- NAME -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="name">Name</label>
<input type="text" name="name" id="name" th:field="${productDTO.name}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="name" minlength="3" maxlength="100" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('name')}" th:errors="${productDTO.name}"></p>
</div>
<!-- NAME -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="price">Price</label>
<input type="number" name="price" id="price" th:field="${productDTO.price}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="price" min="0" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('price')}" th:errors="${productDTO.price}"></p>
</div>
<!-- UNIT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="unit">Unit</label>
<input type="text" name="unit" id="unit" th:field="${productDTO.unit}" class="w-full p-2 border border-slate-300 rounded-lg" placeholder="unit" minlength="3" maxlength="20" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('unit')}" th:errors="${productDTO.unit}"></p>
</div>
</div>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-fit px-4 py-2 flex items-center justify-center gap-1 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Update Product Information
</button>
</div>
</div>
</form>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.umd.js"></script>
<script>
$(document).ready(function () {
Fancybox.bind('[data-fancybox="product-img"]', {
// Your custom options for a specific gallery
});
$("#image").on("change", function(event) {
var file = event.target.files[0];
if (file) {
var imageUrl = URL.createObjectURL(file);
$("#preview_product_image").attr("src", imageUrl);
$("#preview_product_image").closest("a").attr("href", imageUrl ? imageUrl : "#").attr("data-caption", "New Image");
}
});
});
</script>
</body>
</html>
Shipments
Shipment - Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.datatables.net/2.2.2/css/dataTables.dataTables.min.css">
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; }
</style>
<title>Shipments | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-end">
<a class="w-fit px-4 aspect-square flex items-center justify-center border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="shipments/create" data-tippy-content="Make New Shipment">
<i class="fa-solid fa-plus"></i>
</a>
</div>
<div class="w-full px-4">
<div class="w-full p-4 bg-white rounded-lg">
<table class="w-full stripe row-border order-column text-sm"
id="shipmentTable">
<thead>
<tr>
<th>Shipment</th>
<th>Customer</th>
<th>Product</th>
<th>Merchant</th>
<th>Qty</th>
<th>Total Price</th>
<th>Status</th>
<th>Estimate</th>
<th class="bg-white">Action</th>
</tr>
</thead>
<tbody>
<tr th:each="shipment: ${shipments}" class="border-b border-slate-300">
<td th:text="${shipment.createdAt.toString().substring(0,10)}"></td>
<td th:text="${shipment.customer.name}"></td>
<td th:text="${shipment.product.name}"></td>
<td th:text="${shipment.product.merchant.name}"></td>
<td th:text="${shipment.productQuantity}"></td>
<td th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.totalPrice, 0, 'POINT', 0, 'COMMA')}}" class="text-end"></td>
<td class="text-center">
<span th:text="${shipment.status}"
th:classappend="${shipment.status == 'Shipped'} ? 'text-blue-500' : (${shipment.status == 'Delivered'} ? 'text-green-500' : 'text-red-500')"
class="px-2 py-1 rounded-full text-xs font-medium">
</span>
</td>
<td th:text="${shipment.arrivedAt.toString().substring(0,10)}"></td>
<td class="w-fit flex items-center justify-center gap-2 text-white bg-white">
<a class="action-btn show-btn size-10 flex items-center justify-center rounded-md border border-blue-500 text-blue-500
hover:bg-blue-500 hover:text-white focus:bg-blue-500 focus:text-white active:bg-blue-700 transition-colors"
th:href="@{/shipments/detail/{id}(id=${shipment.id})}" data-tippy-content="Show Shipment">
<i class="fa-regular fa-file-lines"></i>
</a>
<a class="action-btn update-btn size-10 flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/shipments/update/{id}(id=${shipment.id})}" data-tippy-content="Update Shipment">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/shipments/delete/' + ${shipment.id}}">
<button class="action-btn delete-btn size-10 flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Shipment" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.datatables.net/2.2.2/js/dataTables.min.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/dataTables.fixedColumns.js"></script>
<script src="https://cdn.datatables.net/fixedcolumns/5.0.4/js/fixedColumns.dataTables.js"></script>
<script src="https://unpkg.com/@popperjs/core@2/dist/umd/popper.min.js"></script>
<script src="https://unpkg.com/tippy.js@6/dist/tippy-bundle.umd.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
let table = new DataTable('#shipmentTable', {
fixedColumns: {
start: 0,
end: 1
},
scrollCollapse: true,
scrollX: true
});
tippy('.action-btn');
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
<script th:if="${errorMessage}" th:inline="javascript">
Swal.fire({
icon: 'error',
title: 'Oops...',
text: [[${errorMessage}]],
});
</script>
<script th:if="${successMessage}" th:inline="javascript">
Swal.fire({
icon: 'success',
title: 'Yippiee!!',
text: [[${successMessage}]],
});
</script>
</body>
</html>
Shipment - Create.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Make New Shipment | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/shipments">
<i class="fa-solid fa-arrow-left"></i>
Back to Shipments
</a>
</div>
<form method="post" th:object="${shipmentDTO}" th:action="@{/shipments/create}">
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-normal gap-4 bg-white rounded-t-lg">
<!-- PRODUCT -->
<div class="w-full max-w-[400px]">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
src="#" alt="no image found" id="preview_product_image">
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<!-- INFO -->
<div class="w-full">
<!-- PRODUCT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="product_id">Product</label>
<select name="product_id" id="product_id" th:field="${shipmentDTO.productId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Product --</option>
<option th:each="product : ${products}"
th:value="${product.id}"
th:data-image="${product.image}"
th:data-price="${product.price}"
th:text="@{'(' + ${product.merchant.name} + ') - (ID-' + ${product.id} + ') -' + ${product.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('productId')}" th:errors="${shipmentDTO.productId}"></p>
</div>
<!-- RECIEVER -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="customer_id">Deliver To</label>
<select name="customer_id" id="customer_id" th:field="${shipmentDTO.customerId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Customer --</option>
<option th:each="customer : ${customers}"
th:value="${customer.id}"
th:text="@{'(ID-' + ${customer.id} + ') - ' + ${customer.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('customerId')}" th:errors="${shipmentDTO.customerId}"></p>
</div>
<!-- DATES -->
<div class="w-full flex items-start justify-center gap-4">
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="created_at">Deliver At</label>
<input type="datetime-local" name="created_at" id="created_at" th:field="${shipmentDTO.createdAt}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('createdAt')}" th:errors="${shipmentDTO.createdAt}"></p>
</div>
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="arrived_at">Estimated Arrival At</label>
<input type="datetime-local" name="arrived_at" id="arrived_at" th:field="${shipmentDTO.arrivedAt}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('arrivedAt')}" th:errors="${shipmentDTO.arrivedAt}"></p>
</div>
</div>
</div>
<div class="w-full h-[2px] mb-2 rounded-full bg-slate-300"></div>
<!-- PRICES -->
<div class="w-2/3">
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="product_quantity">Quantity</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="product_quantity" id="product_quantity" placeholder="product quantity" value="1" min="1" max="100" required th:field="${shipmentDTO.productQuantity}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('productQuantity')}" th:errors="${shipmentDTO.productQuantity}"></p>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="price_per_unit">Price per unit</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="price_per_unit" id="price_per_unit" placeholder="price per unit" value="0" readonly>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="shipment_price">Shipment Cost</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="shipment_price" id="shipment_price" placeholder="shipment price" value="0" min="0" required th:field="${shipmentDTO.shipmentPrice}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('shipmentPrice')}" th:errors="${shipmentDTO.shipmentPrice}"></p>
</div>
<div class="w-full pt-2 flex items-center justify-between gap-2">
<label class="w-full font-semibold text-slate-300" for="total_price">Total Cost</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="total_price" id="total_price" value="" placeholder="total price" min="0" readonly required th:field="${shipmentDTO.totalPrice}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('totalPrice')}" th:errors="${shipmentDTO.totalPrice}"></p>
</div>
</div>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-fit px-4 py-2 flex items-center justify-center gap-1 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Ship 'em out!
<i class="fa-solid fa-truck-arrow-right"></i>
</button>
</div>
</div>
</form>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script>
$(document).ready(function () {
$('input[type="datetime-local"]')
.attr('min', new Date().toISOString().slice(0, 16))
.val(new Date().toISOString().slice(0, 16));
function updateTotalPrice() {
let pricePerUnit = parseFloat($("#price_per_unit").val()) || 0;
let quantity = parseInt($("#product_quantity").val()) || 1;
let shipmentPrice = parseFloat($("#shipment_price").val()) || 0;
let totalPrice = (pricePerUnit * quantity) + shipmentPrice;
$("#total_price").val(totalPrice.toFixed(2));
}
$("#product_id").on("change", function () {
let selectedOption = $(this).find("option:selected");
let imageUrl = selectedOption.data("image");
let price = selectedOption.data("price");
$("#preview_product_image").attr("src", imageUrl ? '/images/'+imageUrl : "#");
$("#price_per_unit").val(price || 0);
updateTotalPrice();
});
$("#product_quantity").on("input", updateTotalPrice);
$("#shipment_price").on("input", updateTotalPrice);
});
</script>
</body>
</html>
Shipment - Detail.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.css"/>
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Shipment Detail | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4 flex items-center justify-between">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/shipments">
<i class="fa-solid fa-arrow-left"></i>
Back to Shipments
</a>
<div class="w-fit flex items-center justify-center gap-2 text-white">
<a class="size-[41.6px] flex items-center justify-center rounded-md border border-yellow-500 text-yellow-500
hover:bg-yellow-500 hover:text-white focus:bg-yellow-500 focus:text-white active:bg-yellow-700 transition-colors"
th:href="@{/shipments/update/{id}(id=${shipment.id})}" data-tippy-content="Update Shipment">
<i class="fa-regular fa-pen-to-square"></i>
</a>
<form id="deleteForm" method="POST" th:action="@{'/shipments/delete/' + ${shipment.id}}">
<button class="delete-btn size-[41.6px] flex items-center justify-center rounded-md border border-red-500 text-red-500
hover:bg-red-500 hover:text-white focus:bg-red-500 focus:text-white active:bg-red-700 transition-colors"
data-tippy-content="Delete Shipment" type="button">
<i class="fa-regular fa-trash-can"></i>
</button>
</form>
</div>
</div>
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-normal gap-4 bg-white rounded-lg">
<!-- PRODUCT -->
<div class="w-full max-w-[400px]">
<a th:href="@{'/images/'+${shipment.product.image}}" data-fancybox="product-img" th:data-caption="${shipment.product.name}" id="zoom-pic">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
th:src="@{'/images/'+${shipment.product.image}}" th:alt="${shipment.product.name}">
</a>
<p th:text="@{'ID - ' + ${shipment.id}}" class="font-semibold text-[1.3em]"></p>
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<!-- INFO -->
<div class="w-full flex items-start justify-normal gap-2">
<!-- SENDER -->
<div class="w-full">
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Sender</p>
<p th:text="${shipment.product.merchant.name}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Phone</p>
<p th:text="${shipment.product.merchant.phone}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Origin</p>
<p th:text="${shipment.product.merchant.address}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Delivered At</p>
<p th:text="${shipment.createdAt.toString().substring(0,10)}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Product</p>
<p th:text="${shipment.product.name}" class="w-full text-lg font-semibold"></p>
</div>
</div>
<!-- RECIEVER -->
<div class="w-full">
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Receiver</p>
<p th:text="${shipment.customer.name}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Phone</p>
<p th:text="${shipment.customer.phone}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Destination</p>
<p th:text="${shipment.customer.address}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Estimated Arrival Date</p>
<p th:text="${shipment.arrivedAt.toString().substring(0,10)}" class="w-full text-lg font-semibold"></p>
</div>
<div class="w-full mb-4">
<p class="w-full font-semibold text-slate-300">Status</p>
<p th:text="${shipment.status}" th:classappend="${shipment.status == 'Shipped'} ? 'text-blue-500' : (${shipment.status == 'Delivered'} ? 'text-green-500' : 'text-red-500')" class="w-full text-lg font-semibold"></p>
</div>
</div>
</div>
<div class="w-full h-[2px] mb-2 rounded-full bg-slate-300"></div>
<!-- PRICES -->
<div class="w-2/3">
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<p class="w-full font-semibold text-slate-300">Quantity</p>
<p th:text="@{${shipment.productQuantity} + ' ' + ${shipment.product.unit}}" class="w-full font-semibold text-end"></p>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<p class="w-full font-semibold text-slate-300">Price per unit</p>
<p th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.product.price, 0, 'POINT', 0, 'COMMA')}}" class="w-full font-semibold text-end"></p>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<p class="w-full font-semibold text-slate-300">Shipment Cost</p>
<p th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.shipmentPrice, 0, 'POINT', 0, 'COMMA')}}" class="w-full font-semibold text-end"></p>
</div>
<div class="w-full pt-2 flex items-center justify-between gap-2">
<p class="w-full font-semibold text-slate-300">Total Cost</p>
<p th:text="@{'Rp ' + ${#numbers.formatDecimal(shipment.totalPrice, 0, 'POINT', 0, 'COMMA')}}" class="w-full font-semibold text-end"></p>
</div>
</div>
</div>
</div>
</div>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.umd.js"></script>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
<script>
$(document).ready(function() {
Fancybox.bind('[data-fancybox="product-img"]', {
// Your custom options for a specific gallery
});
$(".delete-btn").on("click", function (e) {
e.preventDefault();
Swal.fire({
title: "Are you sure?",
text: "This action can't be reverted!",
icon: "warning",
showCancelButton: true,
confirmButtonColor: "#3085d6",
cancelButtonColor: "#d33",
confirmButtonText: "Yes, delete it!"
}).then((result) => {
if (result.isConfirmed) {
$(this).closest("form").submit();
}
});
});
});
</script>
</body>
</html>
Shipment - Update.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta
name="description"
content="Shipment App."
/>
<link rel="shortcut icon" href="/logo.ico" type="image/x-icon">
<link rel="icon" href="/logo.ico" type="image/x-icon">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Quicksand:wght@300..700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.css"/>
<script src="https://kit.fontawesome.com/5b8fa639bb.js" crossorigin="anonymous"></script>
<script src="https://unpkg.com/@tailwindcss/browser@4"></script>
<style type="text/tailwindcss">
@theme {
--font-quicksand: "Quicksand", "sans-serif";
}
a, button {
outline: none;
cursor: pointer;
}
th, td { white-space: nowrap; background-color: #ffffff; }
</style>
<title>Update Shipment | Shipment App</title>
</head>
<body>
<div class="w-full min-h-screen font-quicksand bg-linear-to-br from-[#A0C878] to-[#DDEB9D]">
<header class="w-full p-4 flex items-center justify-between border-b border-white">
<div class="w-full">
<h1 class="text-[3rem] font-bold drop-shadow-md text-white">
Shipments
<i class="fa-solid fa-truck-fast text-orange-500"></i>
</h1>
</div>
<nav class="w-full">
<ul class="w-full flex items-center justify-center gap-8">
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500 rounded-sm bg-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/shipments">
Shipments
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/products">
Products
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/merchants">
Merchant
</a></li>
<li><a class="px-4 py-2 text-white font-semibold border-y border-orange-500
hover:bg-orange-500 hover:rounded-sm focus:bg-orange-500 focus:rounded-sm active:bg-orange-700 transition-all"
href="/customers">
Customers
</a></li>
</ul>
</nav>
<div class="w-full"></div>
</header>
<section class="w-full p-8">
<div class="w-full max-w-[1200px] mx-auto rounded-lg bg-slate-100/20 shadow-lg">
<div class="w-full py-2 px-4">
<a class="block w-fit px-4 py-2 border border-orange-500 text-orange-500 font-medium rounded-md
hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
href="/shipments">
<i class="fa-solid fa-arrow-left"></i>
Back to Shipments
</a>
</div>
<form method="post" th:object="${shipmentDTO}" th:action="@{'/shipments/update/' + ${shipment.id}}">
<div class="w-full px-4">
<div class="w-full p-4 flex items-start justify-normal gap-4 bg-white rounded-t-lg">
<!-- PRODUCT -->
<div class="w-full max-w-[400px]">
<a th:href="@{'/images/'+${shipment.product.image}}" data-fancybox="product-img" th:data-caption="${shipment.product.name}" id="zoom-pic">
<img class="w-full aspect-square p-1 mb-2 object-contain bg-slate-300 rounded-lg"
th:src="@{'/images/'+${shipment.product.image}}" th:alt="${shipment.product.name}" id="preview_product_image">
</a>
<p th:text="@{'ID - ' + ${shipment.id}}" class="font-semibold text-[1.3em]"></p>
</div>
<!-- DETAIL -->
<div class="w-full flex flex-col items-end justify-normal">
<!-- INFO -->
<div class="w-full">
<!-- PRODUCT -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="product_id">Product</label>
<select name="product_id" id="product_id" th:field="${shipmentDTO.productId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Product --</option>
<option th:each="product : ${products}"
th:value="${product.id}"
th:data-image="${product.image}"
th:data-price="${product.price}"
th:text="@{'(' + ${product.merchant.name} + ') - (ID-' + ${product.id} + ') -' + ${product.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('productId')}" th:errors="${shipmentDTO.productId}"></p>
</div>
<!-- RECIEVER -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="customer_id">Deliver To</label>
<select name="customer_id" id="customer_id" th:field="${shipmentDTO.customerId}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="">-- Select a Customer --</option>
<option th:each="customer : ${customers}"
th:value="${customer.id}"
th:text="@{'(ID-' + ${customer.id} + ') - ' + ${customer.name}}"></option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('customerId')}" th:errors="${shipmentDTO.customerId}"></p>
</div>
<!-- DATES -->
<div class="w-full flex items-start justify-center gap-4">
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="created_at">Deliver At</label>
<input type="datetime-local" name="created_at" id="created_at" th:field="${shipmentDTO.createdAt}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('createdAt')}" th:errors="${shipmentDTO.createdAt}"></p>
</div>
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="arrived_at">Estimated Arrival At</label>
<input type="datetime-local" name="arrived_at" id="arrived_at" th:field="${shipmentDTO.arrivedAt}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('arrivedAt')}" th:errors="${shipmentDTO.arrivedAt}"></p>
</div>
</div>
<!-- STATUS -->
<div class="w-full mb-4">
<label class="w-full font-semibold text-slate-300" for="status">Status</label>
<select name="status" id="status" th:field="${shipmentDTO.status}" class="w-full p-2 border border-slate-300 rounded-lg" required>
<option value="Shipped">Shipped</option>
<option value="Delivered">Delivered</option>
</select>
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('status')}" th:errors="${shipmentDTO.status}"></p>
</div>
</div>
<div class="w-full h-[2px] mb-2 rounded-full bg-slate-300"></div>
<!-- PRICES -->
<div class="w-2/3">
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="product_quantity">Quantity</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="product_quantity" id="product_quantity" placeholder="product quantity" value="1" min="1" max="100" required th:field="${shipmentDTO.productQuantity}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('productQuantity')}" th:errors="${shipmentDTO.productQuantity}"></p>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="price_per_unit">Price per unit</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="price_per_unit" id="price_per_unit" placeholder="price per unit" th:value="${shipment.product.price}" readonly>
</div>
<div class="w-full py-2 flex items-center justify-between gap-2 border-b border-slate-500">
<label class="w-full font-semibold text-slate-300" for="shipment_price">Shipment Cost</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="shipment_price" id="shipment_price" placeholder="shipment price" value="0" min="0" required th:field="${shipmentDTO.shipmentPrice}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('shipmentPrice')}" th:errors="${shipmentDTO.shipmentPrice}"></p>
</div>
<div class="w-full pt-2 flex items-center justify-between gap-2">
<label class="w-full font-semibold text-slate-300" for="total_price">Total Cost</label>
<input class="w-full p-2 rounded-lg border border-slate-300 text-end" type="number" name="total_price" id="total_price" placeholder="total price" min="0" readonly required th:value="${shipmentDTO.totalPrice}">
<p class="mt-2 text-sm text-red-500 font-light" th:if="${#fields.hasErrors('totalPrice')}" th:errors="${shipmentDTO.totalPrice}"></p>
</div>
</div>
</div>
</div>
<div class="w-full p-2 flex items-center justify-end border-t border-slate-300 bg-white rounded-b-lg">
<button class="w-fit px-4 py-2 flex items-center justify-center gap-1 border border-orange-500 text-orange-500 font-medium rounded-md hover:bg-orange-500 hover:text-white focus:bg-orange-500 focus:text-white active:bg-orange-700 transition-colors"
type="submit">
Update Shipment
<i class="fa-solid fa-truck-arrow-right"></i>
</button>
</div>
</div>
</form>
<div class="w-full h-4"></div>
</div>
</section>
</div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/@fancyapps/ui@5.0/dist/fancybox/fancybox.umd.js"></script>
<script>
$(document).ready(function () {
Fancybox.bind('[data-fancybox="product-img"]', {
// Your custom options for a specific gallery
});
$('input[type="datetime-local"]')
.attr('min', new Date().toISOString().slice(0, 16))
.val(new Date().toISOString().slice(0, 16));
function updateTotalPrice() {
let pricePerUnit = parseFloat($("#price_per_unit").val()) || 0;
let quantity = parseInt($("#product_quantity").val()) || 1;
let shipmentPrice = parseFloat($("#shipment_price").val()) || 0;
let totalPrice = (pricePerUnit * quantity) + shipmentPrice;
$("#total_price").val(totalPrice.toFixed(2));
}
$("#product_id").on("change", function () {
let selectedOption = $(this).find("option:selected");
let imageUrl = selectedOption.data("image");
let price = selectedOption.data("price");
$("#preview_product_image").attr("src", imageUrl ? '/images/'+imageUrl : "#");
$("#preview_product_image").closest("a").attr("href", imageUrl ? '/images/'+imageUrl : "#").attr("data-caption", (selectedOption.text()).split('-')[3]);
$("#price_per_unit").val(price || 0);
updateTotalPrice();
});
$("#product_quantity").on("input", updateTotalPrice);
$("#shipment_price").on("input", updateTotalPrice);
updateTotalPrice();
});
</script>
</body>
</html>
Welp, I guess that’s all I can share for now. I pretty exhausting writing down an article even when using a platform like this XD. But I’m quite happy tho’ to be able to share this here.
Any comment on this post will be appreciated, but don’t expect to much if you’re gonna ask anything related to Java, since I’m quite new here :v
Subscribe to my newsletter
Read articles from Benedict Kenjiro Lehot directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Benedict Kenjiro Lehot
Benedict Kenjiro Lehot
Full-Stack Expert Wannabe