How to Get Started with .NET: A Beginner’s Roadmap

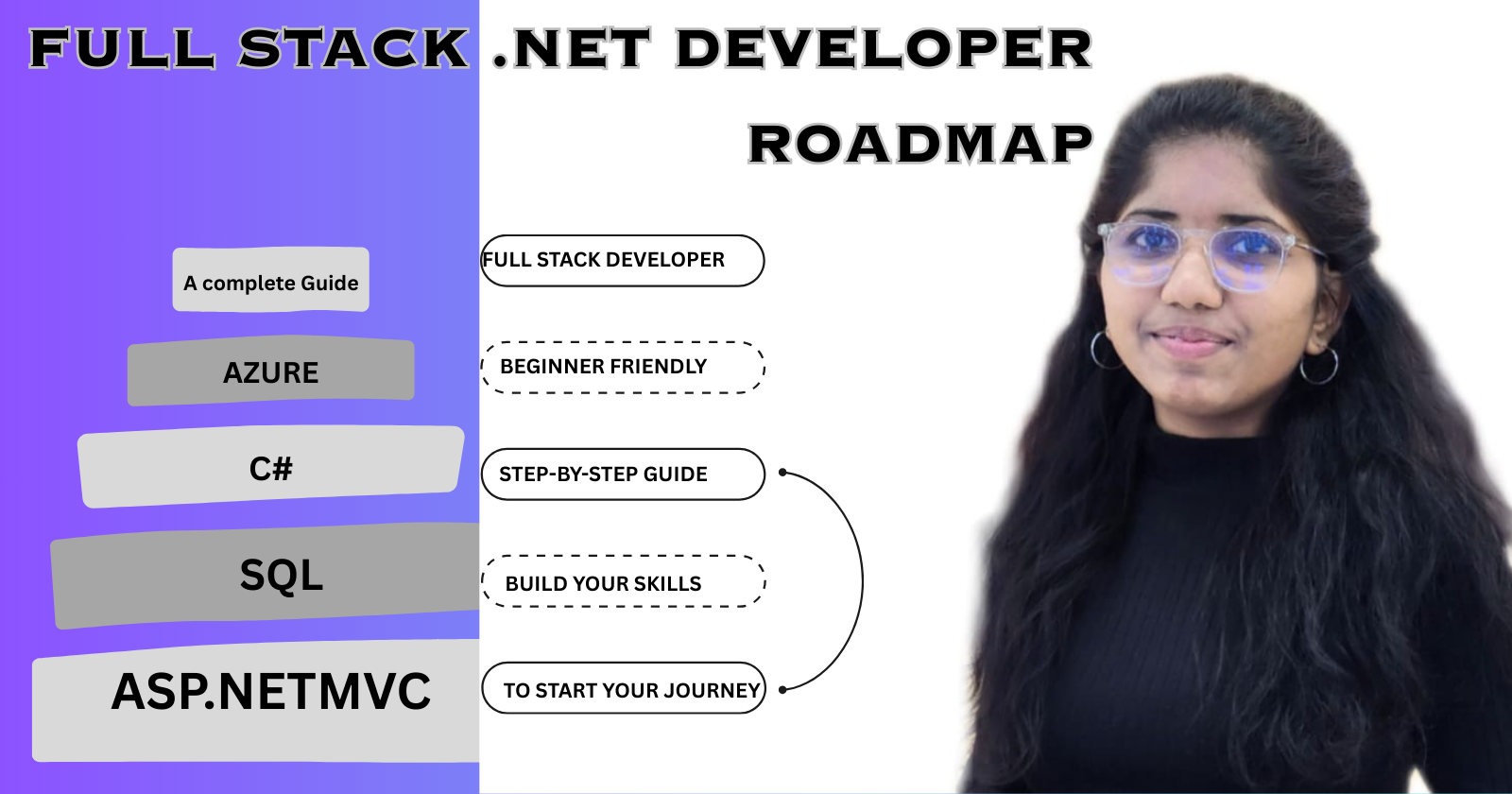
Step 1: Install the Right Tools
🔹 Download & Install .NET SDK (Download .NET SDK)
🔹 Install Visual Studio (Download Visual Studio 2022 )
Pro Tip: Use the dotnet --version command in CMD to check if .NET is installed.
Step 2: Learn the Basics of C#
.NET applications are mostly written in C#, so start with:
Variables and Data types
Loops and conditions
Functions and OOPs
Recommended Resources:
Step 3: Build Your First .NET App
// C# Console Application
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
Step 5: Learn SQL for .NET Development
Why SQL?
Most .NET applications store data in a database, and SQL helps you manage it!
SQL Basics You Need to Know
CRUD Operations – SELECT, INSERT, UPDATE, DELETE
Joins – INNER JOIN, LEFT JOIN, RIGHT JOIN
Stored Procedures & Functions
Indexes & Performance Optimization
Next Step: Install SQL Server and connect it with .NET using Entity Framework Core!
Step 6: Learn Entity Framework (EF) Core
What is EF Core?
EF Core is an ORM (Object-Relational Mapper) that lets you interact with the database using C# objects instead of writing SQL queries.
Key Concepts to Learn:
DbContext & DbSet (How to define & manage databases)
Migrations (How to update the database without losing data)
LINQ Queries (Write queries using C# instead of SQL)
CRUD Operations (Create, Read, Update, Delete)
Step 7: Learn ASP.NET Core (Building a Web API)
What is ASP.NET Core?
ASP.NET Core is a cross-platform framework for building web applications & APIs.
Key Concepts to Learn:
Routing & Controllers
Dependency Injection
Middleware
Authentication & Authorization (JWT, Identity)
Step 8: Learn ASP.NET Core MVC
What is MVC?
MVC (Model-View-Controller) is a design pattern that separates logic, UI, and data for better structure and scalability.
Key Concepts to Learn:
Models (Data & EF Core)
Views (UI with Razor Syntax & HTML)
Controllers (Handling Requests & Responses)
Creating an ASP.NET Core MVC Project
Step 9: Learn Angular (Frontend Framework)
Why Angular?
Angular is a powerful TypeScript-based frontend framework for building single-page applications (SPAs).
Key Concepts to Learn:
Components & Modules
Services & HTTP Requests
Routing & Navigation
Data Binding & Forms
Getting Started with Angular
Step 10: Learn Docker Basics
What is Docker?
Docker allows you to package your .NET app + dependencies into a container, so it runs the same everywhere (local, server, cloud).
Key Docker Concepts to Learn
Docker Images & Containers
Dockerfile (How to create a container)
Docker Compose (Multiple containers in one app)
Volume & Networking
Create a Simple Docker Container for .NET
Step 11: Learn Azure Fundamentals
What is Azure?
Azure is a cloud platform where you can deploy, manage, and scale applications globally.
Key Azure Services to Learn
Azure App Service → Deploy .NET apps easily
Azure Container Registry (ACR) → Store Docker images
Azure Kubernetes Service (AKS) → Manage Docker containers at scale
Azure SQL Database → Cloud-hosted SQL database
Azure Virtual Machines (VMs) → Host applications in the cloud
Step 12: Create an ASP.NET Core MVC Project
Student Attendance System
Tech Stack: ASP.NET Core MVC, EF Core, SQL Server
Features:
Register students
Mark attendance
View daily/weekly reports
Subscribe to my newsletter
Read articles from Damini Akunapuram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
