How to Fix 500 Internal Server Error from Database Connection Failures

Table of contents
- Fixing the 500 Internal Server Error from Database Connection Failures
- Common Causes of Database Connection Failures
- How to Prevent Future Database Connection Errors
- APIs: A Simpler Way Forward
- Conclusion
- FAQ
- What causes a 500 Internal Server Error related to database connections?
- How can I quickly fix a database connection failure causing a 500 error?
- Can firewall settings block database connections and cause a 500 error?
- How does using pre-built APIs like ApyHub help reduce 500 errors?
- Can a misconfigured .env file trigger a 500 Internal Server Error?
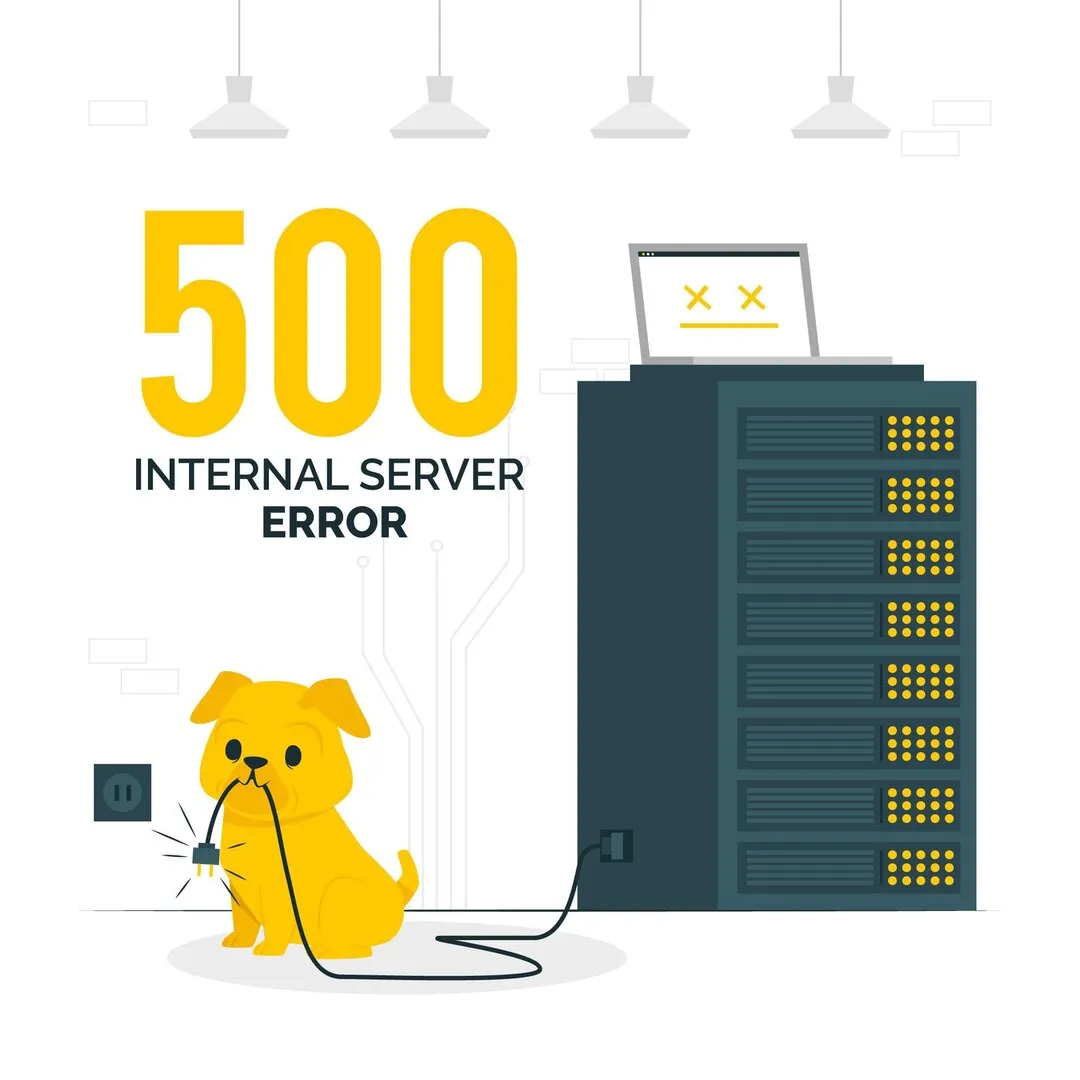
A normal and frequent scenario: You are a developer and you are deploying your latest web application, everything’s running smoothly, and then it hits — a "500 Internal Server Error." For developers, this HTTP status code is a red flag that something’s broken on the server. When it’s tied to a database connection failure, it means your app can’t reach its data lifeline.
ApyHub offers a smarter approach to API testing and automation, helping you save time and boost efficiency.
Fixing the 500 Internal Server Error from Database Connection Failures
The "500 Internal Server Error" signals a server-side breakdown, and when it’s due to a database connection failure, your application can’t communicate with the database. Here’s how experienced developers can resolve it fast, with precise steps and code where it counts.
1. Inspect Error Logs
Dive into your web server or application logs—Apache, Nginx, or framework-specific logs like Laravel’s. You’ll find detailed messages pinpointing the issue, such as "Access denied for user ‘root’@’localhost’" or "Connection refused." These clues direct your next move.
2. Validate Database Credentials
Incorrect credentials are a frequent culprit. Open your connection code and confirm the username, password, host, and database name are spot-on. Test it with this PHP snippet:
1<?php2
3$host = "localhost";4
5$username = "your_username";6
7$password = "your_password";8
9$dbname = "your_database";10
11 12
13$conn = new mysqli($host, $username, $password, $dbname);14
15 16
17if ($conn->connect_error) {18
19die("Connection failed: " . $conn->connect_error);20
21}22
23echo "Connected successfully!";24
25?>
If it fails, adjust the credentials and retest until it connects.
3. Ensure Database Server Availability
Verify the database server is running. For local setups, check with systemctl status mysql
(Linux) or equivalent. For remote or cloud-hosted databases, log into your provider’s dashboard to confirm uptime. Restart the server if it’s down.
4. Test Network Reachability
If the database is on a separate server, confirm connectivity with ping
or telnet
:
telnet your_database_host 3306
No response? Check for firewall blocks on port 3306 (MySQL default) or network restrictions. Whitelist your app server’s IP or open the port as needed.
5. Monitor Connection Limits
Exceeding the database’s max connections triggers failures. Query the limit:
1SHOW VARIABLES LIKE 'max_connections';
If it’s capped low (e.g., 100) and your app’s traffic is high, increase it via configuration (e.g., my.cnf
for MySQL) or optimize your app to close unused connections.
6. Audit Connection Code
Errors in your database code—typos, incorrect functions, or misconfigured ORMs—can break connections. Review your setup (e.g., Sequelize in Node.js, Django ORM) and test incrementally to isolate faults.
7. Confirm Database Drivers
Missing or misconfigured drivers halt communication. In PHP, run phpinfo()
to check for mysqli
or pdo_mysql
. In Node.js, ensure npm install mysql
or pg
is done. Install missing drivers, restart the server, and verify.
This approach resolves the error efficiently. Next, we’ll break it down for beginners with simpler terms and step-by-step guidance.
Common Causes of Database Connection Failures
- Incorrect Database Credentials
One of the most frequent reasons for database connection failures is misconfigured credentials. If the username, password, or hostname in your application's configuration is incorrect, the server cannot establish a database connection.
Example of a misconfigured .env
file:
DB_HOST=localhost
DB_USER=wrong_user
DB_PASS=incorrect_password
- Database Server Unavailability
If the database server is down, overloaded, or unreachable due to network issues, your application won’t be able to connect. Simple network tools like ping
or telnet
can help you check if the database server is accessible.
- Resource Exhaustion
Overloaded servers with too many concurrent connections or insufficient memory resources may refuse new database connections, resulting in 500 errors.
- Firewall or Security Restrictions
IP whitelisting, firewall rules, or database access permissions might block connection attempts, especially in cloud environments or shared hosting setups.
- Syntax Errors in Queries
Badly structured or malformed SQL queries can cause the database server to reject requests, leading to application-level failures and HTTP 500 errors.
- Application Code Bugs
Improper error handling or poorly written code can cause unhandled exceptions when interacting with the database, resulting in a 500 Internal Server Error.
How to Prevent Future Database Connection Errors
Once you’ve fixed the error, keep it gone with these best practices:
- Reuse Connections
Instead of sending a new waiter to the kitchen every time, keep a few on standby (connection pooling). Libraries like pg-pool
(Node.js) or HikariCP (Java) handle this automatically.
- Handle Slip-Ups Smoothly
Catch errors in your code so users see “We’re fixing this!” instead of a crash. Example in Node.js:
1const mysql = require('mysql');2
3const connection = mysql.createConnection({4
5host: 'localhost',6
7user: 'your_user',8
9password: 'your_pass',10
11database: 'your_db'12
13});14
15 16
17connection.connect(err => {18
19if (err) {20
21console.log('Trouble connecting:', err.message);22
23return;24
25}26
27console.log('All good!');28
29});
- Watch the Database
Use tools like MySQL Workbench or your host’s dashboard to track connection counts and performance. Spot issues before they escalate.
- Hide Credentials
Don’t write your kitchen key in the code. Store it safely with environment variables:
1export DB_HOST=localhost2
3export DB_USER=your_user4
5export DB_PASS=your_pass6
7export DB_NAME=your_db
Access them in code (e.g., process.env.DB_HOST
in Node.js).
- Test Before Launch
Run connection tests during development with scripts or tools like Postman. Fix problems early.
- Secure the Bridge
Encrypt connections with SSL/TLS to protect data. Check your database docs to enable it—most hosts support it.
APIs: A Simpler Way Forward
Managing database connections can feel like juggling flaming torches. Want an easier path? APIs offload the heavy lifting. ApyHub Catalog provides you with the tools to swap complex connection code for straightforward API calls. They manage the database, queries, and scaling—you just use the data. Fewer errors, less stress, more focus on building.
Conclusion
The "500 Internal Server Error" from database connection failures is a solvable puzzle. Devs can pinpoint and fix it with logs, credentials, and network checks. Beginners can follow the restaurant analogy to troubleshoot step-by-step. Add best practices, and you’ll keep your app running smoothly. Tired of the hassle? APIs like ApyHub’s catalog simplify data management, letting you skip the connection drama. Next time that 500 strikes, you’ve got this.
FAQ
What causes a 500 Internal Server Error related to database connections?
A 500 error often occurs when the server fails to connect to the database due to incorrect credentials, server downtime, network issues, or exhausted resources.
How can I quickly fix a database connection failure causing a 500 error?
Check your server logs, validate database credentials, ensure the database server is running, test network connectivity, and review your application’s connection code.
Can firewall settings block database connections and cause a 500 error?
Yes, strict firewall rules or IP whitelisting can block access to the database, leading to connection failures and triggering a 500 error.
How does using pre-built APIs like ApyHub help reduce 500 errors?
Pre-built APIs handle backend complexities, offer reliable infrastructure, and reduce coding errors, minimizing the risk of server-side failures.
Can a misconfigured .env file trigger a 500 Internal Server Error?
Yes, incorrect database credentials or configuration settings in the .env
file can prevent the application from connecting to the database, resulting in a 500 error.
Subscribe to my newsletter
Read articles from Nikolas Dimitroulakis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikolas Dimitroulakis
Nikolas Dimitroulakis
Life long learner, growth, entrepreneur, business dev. Currently helping organisations manage every aspect of their API lifecycle and collaborate effectively. Executive MBA degree holder with a background in operations and Industrial Engineering. Specialties: -Managing of Operations -Revenue Growth -Startup growth -Customer Success Management -Change Management and Strategic Management -Requirements engineering -Product Ownership -Continuous Improvement Capabilities and culture towards a first time right approach I write my thoughts here: https://nikolasdimitroulakis.substack.com/