How to Create RESTful APIs with Django REST Framework (DRF)
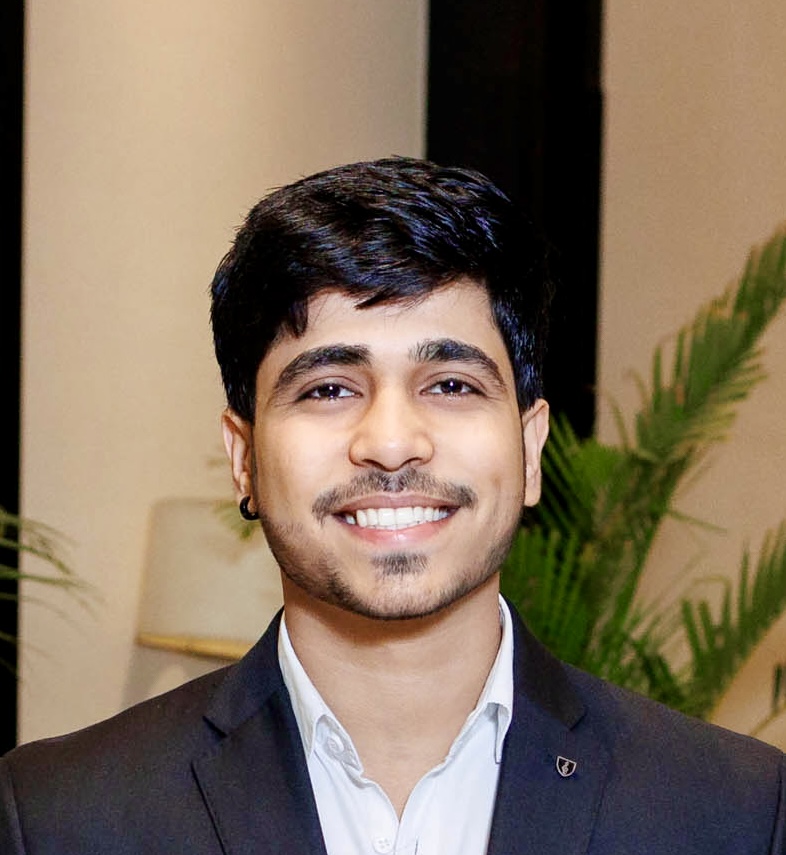
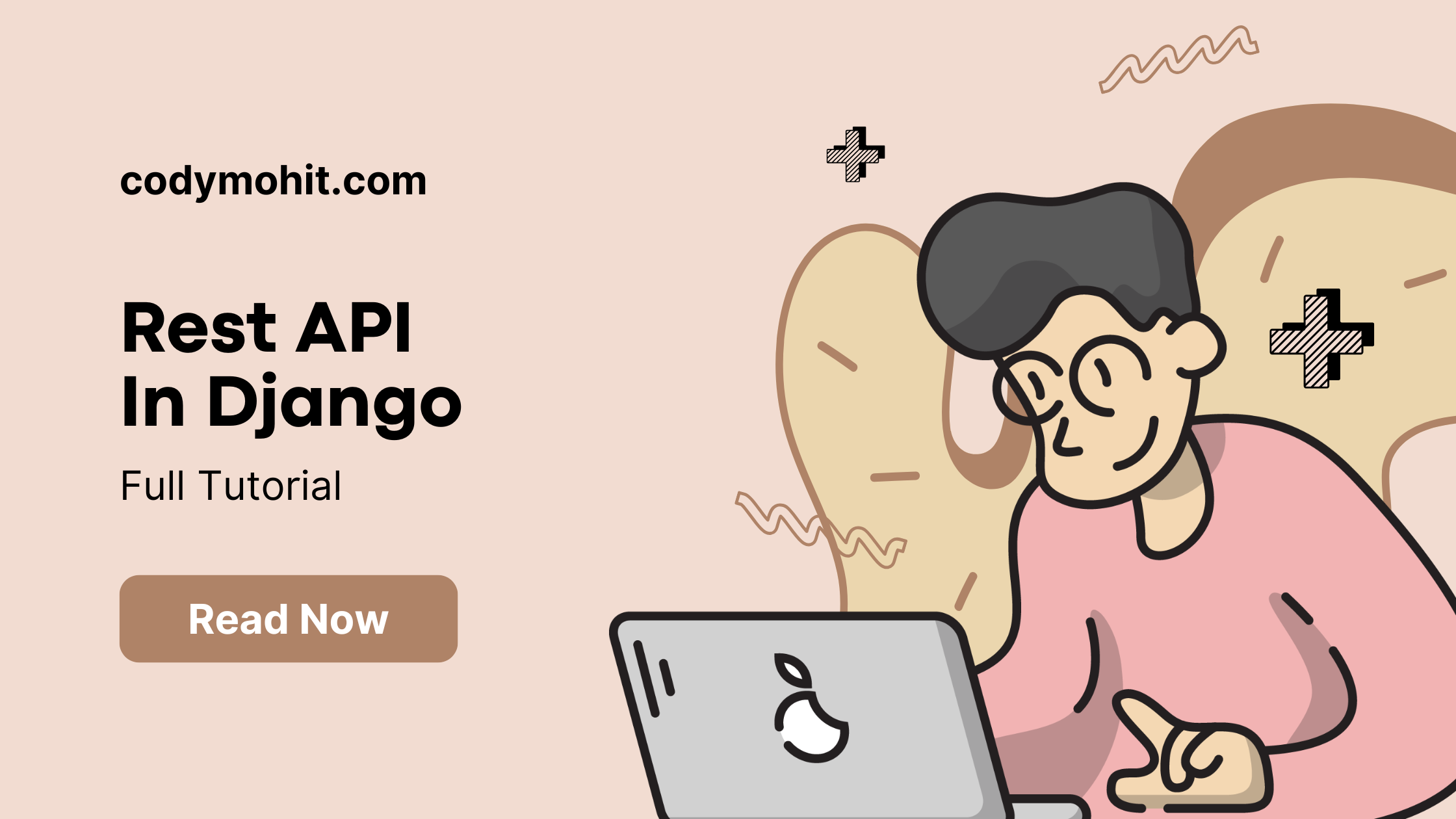
Why Choose Django REST Framework for API Development?
So, you're building an API, huh? Great! But with so many options out there, choosing the right framework can feel overwhelming. That's where Django REST Framework (DRF) steps in. It's a powerful and flexible toolkit that sits on top of the already amazing Django framework, making API development a breeze. Why is DRF so popular? It boils down to these key features: speed, scalability, and a ton of built-in features that save you time and headaches. Think automatic serialization, authentication, and browsable APIs—all without writing mountains of code. DRF lets you focus on the core logic of your application, not the boilerplate. Learn more about its amazing features here.
Understanding RESTful Principles: A Quick Recap
Before diving into Django REST Framework, it's helpful to understand the basics of RESTful APIs. REST, or Representational State Transfer, is an architectural style for building web services. It's all about using standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. Each method corresponds to a specific action: GET retrieves data, POST creates data, PUT updates data, and DELETE removes data. Understanding these principles is crucial for building well-structured and efficient APIs. Check out this excellent resource for a more in-depth understanding.
Django REST Framework and RESTful Principles
Django REST framework elegantly implements these RESTful principles. It provides tools to easily map HTTP methods to your Django models, creating clean and consistent APIs. You'll find that using DRF aligns perfectly with the best practices of RESTful design, making your APIs easier to understand and maintain.
Setting up Your Development Environment: A Step-by-Step Guide
Getting started with Django REST Framework is easier than you think! First, you'll need to have Python and pip installed. Then, create a new Django project and install DRF using pip: pip install djangorestframework
. Next, you'll need to add 'rest_framework' to your INSTALLED_APPS
setting in your settings.py
file. After that, its smooth sailing! Remember to refer to the official Django REST Framework tutorial for detailed instructions and examples. This guide will walk you through creating your first API view in no time!
Your First Django REST Framework API
Once your environment is set up, creating your first API is a breeze. DRF offers a simple, intuitive way to define serializers—objects that convert your Python models into JSON or other formats for your API responses. You will learn how to create views that handle different HTTP requests and interact with your database seamlessly. This is where the magic truly begins—building functional and powerful APIs using Django REST framework.
Troubleshooting Common Django REST Framework Issues
Even experienced developers encounter issues. Common problems include serialization errors, authentication problems, and database interactions. Don't worry! The Django REST Framework community is very active, and there are plenty of resources available online to help you troubleshoot. Remember to check the official documentation and search for solutions on Stack Overflow—you're not alone!
Project Setup and Configuration
Creating a New Django Project
So, you wanna build awesome APIs with Django? Great! The first step is creating your Django project. If you haven't already, you'll need to have Python and pip installed. Then, open your terminal and type: django-admin startproject myproject
(replace "myproject" with your project's name). This creates the basic structure for your project. Pretty simple, right? Next, navigate into your project directory using cd myproject
.
Installing Django REST Framework
Getting Started with Django REST Framework
Now for the fun part – installing the Django REST Framework! This is where the magic happens. Open your terminal (still inside your project directory) and run: pip install djangorestframework
. That's it! You've just added a powerful tool to your Django arsenal. You can find more info on installation and other details on the official Django REST Framework documentation. It's a really helpful resource.
Configuring Your Django Project for DRF
Integrating Django REST Framework into Your Project
Alright, we've got Django and Django REST Framework installed. Now let's tell Django to use it. Open your myproject/settings.py
file. You'll need to add 'rest_framework' to your INSTALLED_APPS
setting. It should look something like this:
INSTALLED_APPS = [
# ... other apps ...
'rest_framework',
]
That's it! You have successfully configured your Django project to work with DRF. You are now ready to start creating your APIs! Remember to run migrations using python manage.py migrate
after making changes to your settings.
For more advanced configuration options, check out this amazing Stack Overflow thread dedicated to Django REST Framework. It's a great place to find answers to your questions.
Building Your First Django REST Framework API
1. Defining Your Models: Structuring Your Data
Before you even think about APIs, you need to define your data structures. In Django, this means creating models. Think of models as blueprints for your data; they describe what kind of information you'll be storing (like users, products, or blog posts). Each model represents a table in your database. For example, a simple blog post model might have fields for title, content, and author. Defining these models clearly is crucial for a well-structured Django REST Framework application.
2. Creating Serializers: Transforming Data for APIs
Now, your data's in the database, but APIs don't speak database language. That's where serializers come in. A serializer in Django REST Framework translates your Python model instances into JSON or other formats that APIs understand, and vice versa. It's like a translator between your database and the outside world. You'll define how your model data should look when it's sent to or received from an API endpoint. This is essential for building robust and efficient APIs.
3. Building Your First ViewSet: Handling API Requests
ViewSets are the heart of your Django REST Framework API. They handle the logic of processing API requests (like GET, POST, PUT, and DELETE). Think of a ViewSet as a central hub that recieves requests, uses your serializers to process the data, and interacts with your database. They simplify things considerably compared to writing individual views for each HTTP method, which you would do without Django REST Framework. They are really helpfull when building complex APIs.
4. Defining API Endpoints: GET, POST, PUT, DELETE
Now it's time to define the actual API endpoints. These are the URLs that clients will use to interact with your data. Each endpoint corresponds to a specific action. A GET request retrieves data, a POST request creates new data, a PUT request updates existing data, and a DELETE request removes data. You'll map these HTTP methods to the appropriate actions within your ViewSets using Django REST Framework's router system. Learn more about Django REST Framework Routers.
5. Testing Your API: Ensuring Functionality
Thorough testing is absolutely vital for any API. You'll want to test that your API endpoints are responding correctly to different requests, handling errors gracefully, and returning the expected data. Django REST Framework integrates well with testing frameworks like pytest, making it easier to write comprehensive tests for your API. Use tools like pytest to make testing easier.
6. Handling Different HTTP Methods with Django REST Framework
Django REST Framework makes handling different HTTP methods a breeze. Each method (GET, POST, PUT, DELETE) maps to a specific method within your ViewSet (list
, create
, update
, destroy
, respectively). This clean separation of concerns makes your code more readable and maintainable. This makes the process of building robust and scalable APIs easier compared to writing everything from scratch.
Advanced Django REST Framework Techniques
Implementing Authentication and Authorization with Django REST Framework
Securing your API is paramount. Django REST Framework (DRF) offers robust tools for authentication and authorization. You can easily integrate with existing Django authentication backends, like those provided by the django.contrib.auth
app. Beyond simple authentication, DRF allows for granular authorization using permission classes. These classes determine whether a user has the necessary rights to access specific resources. For example, you might create a permission class to only allow staff users to modify data. Mastering this is crucial for building secure and reliable APIs. Check out the official DRF documentation for a deep dive into these features: Django REST Framework Permissions. Remember to consider different authentication methods like token-based authentication (JWTs are popular) to fit your application needs.
Working with Relationships: Handling Foreign Keys and Many-to-Many Fields in Django REST Framework
Dealing with database relationships is a core aspect of any web application. Django REST Framework handles foreign keys and many-to-many relationships gracefully. You can define serializers to represent these relationships effectively. For example, a foreign key can be represented by nested serializers, showing related data directly in the response. Many-to-many relationships might require more sophisticated handling, potentially using hyperlinked relationships. Understanding how DRF manages these relationships is essential for building complex APIs. Here's a great resource to help you understand this process better: Django REST Framework Relations. Properly handling these relationships can significantly impact API performance and data consistency.
Pagination and Filtering: Optimizing Django REST Framework API Responses
Large datasets can overwhelm your API. Pagination breaks down large responses into smaller, manageable chunks, improving performance and user experience. DRF provides various pagination classes to choose from, like page number, cursor-based, and limit-offset pagination. Filtering allows clients to request only the data they need, further enhancing performance and reducing bandwidth usage. DRF's filter backend framework makes implementing filters a breeze. Combining pagination and filtering can significantly improve the efficiency of your API. To lern more, check out: Django REST Framework Filtering and Django REST Framework Pagination. You should carefully consider which pagination style best suits your application.
Utilizing Django REST Framework's Built-in Features: Throttling, Rate Limiting, and Caching
DRF offers built-in mechanisms for managing API usage. Throttling helps prevent abuse by limiting the number of requests a user can make within a specific time frame. Rate limiting is a related concept that often works with IP addresses to prevent denial-of-service attacks. Caching can dramatically improve performance by storing frequently accessed data. Implementing these features is a vital step in building a robust and scalable API. Remember to configure these features carefully to avoid unintentionally blocking legitimate users. This blog post also covers some advanced concepts in DRF: [Link to your internal blog post about advanced DRF concepts]
Customizing the Django REST Framework Response: Tailoring the API Output
DRF provides considerable flexibility in customizing the API response. You can modify the structure, fields, and even the overall format (like JSON or XML). Using serializers allows you to map your Django models to the desired API response structure. Creating custom serializers allows you to tailor responses precisely to your needs. You can also use renderers to control the final output format if needed. This is incredibly important for API clients expecting particular data formats. For example, you could create custom serializers to only include specific fields for certain API endpoints.
Deploying Your Django REST Framework API
Choosing a Deployment Platform for Your Django REST Framework Application
So, you've built an awesome Django REST Framework (DRF) API. Congrats! Now comes the fun part—getting it live and accessible to the world. But where do you deploy it? The choice depends on several factors: your budget, technical expertise, expected traffic, and scalability needs. Let's explore some popular options.
Cloud Platforms: Giants like AWS, Google Cloud Platform (GCP), and Microsoft Azure offer managed services that simplify deployment and scaling. They handle server management, allowing you to focus on your code. AWS offers services like Elastic Beanstalk and Elastic Kubernetes Service (EKS), GCP provides Google App Engine and Kubernetes Engine, and Azure has Azure App Service and Azure Kubernetes Service (AKS). These are all great options if your budget allows it!
Managed Hosting Providers: Services like Heroku and PythonAnywhere abstract away much of the server management, making deployment easier for beginners. They often come with simpler pricing models, perfect for smaller projects or those just starting out. However, they may lack some of the granular control offered by cloud platforms.
VPS (Virtual Private Server): For more control and customization, a VPS provider like DigitalOcean or Linode offers a virtual server that you manage entirely. This gives you maximum flexibility but requires more technical expertise. Remember that you'll be responsible for security updates and server maintenance. This is great for those who want complete control.
Deployment Strategies for Django REST Framework
Once you've chosen a platform, you need a deployment strategy. Here are a few common approaches:
1. Gunicorn + Nginx (or Apache): This is a classic and very reliable setup. Gunicorn is a WSGI HTTP server that handles requests to your Django application, while Nginx (or Apache) acts as a reverse proxy, handling static files, load balancing, and SSL encryption. This is a robust setup used for many projects.
2. Docker: Docker containers package your application and its dependencies into a single, portable unit. This ensures consistency across different environments, simplifying deployment and scaling. Check out our article on Using Docker with Django REST Framework for a more detailed explanation.
3. Kubernetes: For large-scale deployments and complex architectures, Kubernetes is a powerful container orchestration platform. It automates the deployment, scaling, and management of containerized applications. This is only really needed for very large scale applications.
Optimizing Your DRF API for Performance and Scalability
A well-optimized API is crucial for a good user experience and cost-effectiveness. Here are some key optimization strategies:
Database Optimization: Use efficient database queries, indexing, and caching to minimize database load. Consider using database connection pooling to improve performance. This can speed up your application significantly!
Caching: Implement caching mechanisms like Redis or Memcached to store frequently accessed data in memory. This reduces the load on your database and speeds up response times. This is key to keeping your api responsive even with heavy traffic.
Asynchronous Tasks: For long-running tasks, use asynchronous task queues like Celery to avoid blocking the main thread. This keeps your API responsive even while processing background jobs.
Load Balancing: Distribute traffic across multiple servers to handle increased load and improve availability. This is essential for scalability.
Code Optimization: Write efficient and clean code. Profile your application to identify bottlenecks and optimize performance-critical sections. This is an ongoing process of refinement.
Using Docker with Django REST Framework
Docker simplifies deployment by packaging your application and its dependencies into a container. This ensures consistent behavior across different environments. You can find excellent tutorials on integrating Docker with Django REST Framework online. Search for "Dockerizing Django REST Framework" to find helpful resources.
Troubleshooting and Debugging Your Django REST Framework API
Common Errors and Solutions in Django REST Framework
Building a robust Django REST Framework API is a rewarding experience, but inevitably, you'll encounter errors. Let's tackle some common issues and their solutions. One frequent headache is dealing with 400 Bad Request
errors. These often stem from issues with your request data—maybe a missing field, incorrect data types, or exceeding field length limits. Carefully examine your API request payload and the serializer fields in your views to identify the mismatch. The Django REST Framework documentation offers excellent guidance on serializers and their validation here. Remember to check your browser's developer tools (Network tab) for detailed error messages and the exact server response. This often pinpoints the problem area.
Another common problem is 500 Internal Server Errors
. These are less specific, indicating a server-side problem. Thorough logging is essential. Make sure your Django project is configured to log errors comprehensively. Check your logs (typically found in your project's logs/
directory) for clues about the exception that caused the error. Debugging tools, such as Python's built-in pdb
(Python Debugger), can be incredibly valuable in tracking down where the error occurs within your code. Learning to effectively use a debugger is a crucial skill for any Django developer. You can find a helpful introduction to pdb
here.
Debugging Techniques for Django REST Framework
Effective debugging is vital for Django REST Framework development. Beyond logging and pdb
, consider these powerful techniques. Firstly, utilize the Django REST Framework's built-in browsable API. This interface allows for interactive testing of your endpoints, providing immediate feedback on requests and responses. Its easy to use and it is great to understand your API.
Using Django's Debug Toolbar
The Django Debug Toolbar (check it out here) provides detailed insights into your application's performance and requests. It's invaluable for identifying slow database queries or other performance bottlenecks that might contribute to errors or unexpected behavior. Its a must have for every Django project.
Leveraging Request Logging in Django REST Framework
Implementing detailed request logging in your views enables you to meticulously track API calls and responses. This comprehensive record is crucial for debugging complex issues or identifying patterns in errors. Add this to your settings file for more information. Add custom logging to your viewsets to improve logging capablities, if your basic logging is not giving you the required detail.
Testing Your Django REST Framework API
Writing comprehensive tests is an often overlooked but incredibly effective debugging strategy. Unit tests verify the correct functionality of individual components, while integration tests ensure that different parts of your API work together seamlessly. A robust test suite can prevent many issues from ever reaching production, catching errors early in the development cycle. Learn more about testing in Django REST Framework here. It is very usefull to start using this now.
Best Practices for Secure and Efficient Django REST Framework APIs
Security Considerations for Django REST Framework APIs
Building rock-solid APIs with Django REST Framework (DRF) requires a multi-layered security approach. Lets start with authentication. Don't even THINK about skipping this! DRF offers several built-in authentication classes, including SessionAuthentication, BasicAuthentication, and TokenAuthentication. For public APIs, TokenAuthentication or JSON Web Tokens (JWTs) are often preferred for statelessness and scalability. You can learn more about JWTs and their implementation with DRF here.
Next up: authorization. Just because a user is authenticated doesn't mean they should have access to everything! Use DRF's permission classes (like IsAuthenticated, IsAdminUser, or custom permissions) to control access to specific resources. Think carefully about the permissions your application needs – granular control is key. For example, you might only allow users to update their own profiles, not everyone else's.
Input validation is another crucial aspect. Never trust user input! DRF's serializers provide powerful validation tools. Define fields with appropriate data types and validators to prevent unexpected data from entering your system. This prevents vulnerabilities and improves data integrity. Properly handling exceptions is also important. Always log errors effectively to track down issues and improve your application's resilience. Consider using a robust logging system like Sentry https://sentry.io/ to monitor for potential security breaches.
Efficiency Tips for Django REST Framework APIs
For a snappy API, optimization is vital. Database queries are often the bottleneck. Use Django's ORM effectively, leveraging features like select_related()
and prefetch_related()
to reduce the number of database hits. These methods can significantly speed up your API responses. Remember to profile your code to identify performance bottlenecks. Tools like Django Debug Toolbar can help pinpoint slow queries or other areas needing optimization.
Caching is your friend. Implement caching strategies using tools like Redis or Memcached to store frequently accessed data. DRF provides excellent support for caching mechanisms. Caching reduces the load on your database and improves the response time of your API. Consider using appropriate cache headers in your API responses to leverage browser and CDN caching. This will further optimize the speed of your application.
Pagination is essential for handling large datasets. DRF offers built-in pagination classes to break down large responses into manageable chunks. This prevents the API from returning massive datasets that could overwhelm clients. You can learn more about pagination in DRF here.
Documentation for Django REST Framework API
Good documentation is as important as good code. DRF makes it easy to generate API documentation using tools like Swagger/OpenAPI. These tools automatically create interactive documentation based on your serializer and view definitions. Properly documenting your endpoints ensures that developers using your API can easily understand how to use it and prevents misunderstandings. A well-documented API is a happy API!
Consider using a documentation generator like DRF's browsable API, which provides a user interface for interacting with your API and viewing its documentation directly in your browser. You can customize its appearance to match the style of your application. You can even integrate this with tools that allow you to automatically generate documentation from code comments. Read more on how to effectively document your Django REST framework API here. This link provides detailed information on setting up and customizing the browsable API.
Example: Building a CRUD API with Django REST Framework
Let's build a simple CRUD (Create, Read, Update, Delete) API for a blog post model using DRF. First, define your models:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
# ... other fields ...
Next, create a serializer:
from rest_framework import serializers
class BlogPostSerializer(serializers.ModelSerializer):
class Meta:
model = BlogPost
fields = ['id', 'title', 'content']
Finally, build your viewset:
from rest_framework import viewsets
class BlogPostViewSet(viewsets.ModelViewSet):
queryset = BlogPost.objects.all()
serializer_class = BlogPostSerializer
This provides the basic structure. You'll need to include appropriate URLs and settings in your urls.py
and settings.py
files. This simple example demonstrates how easily you can build a fully functional API with Django REST Framework. For more detailed examples and tutorials, refer to the official DRF documentation here.
Extending Django REST Framework Functionality with Third-Party Packages
Integrating with Popular Libraries for Django REST Framework
Django REST Framework (DRF) is awesome, but sometimes you need more. That's where third-party packages come in! They add superpowers to your DRF APIs, letting you do things like handle complex authentication, add robust search functionality, or even integrate with specific databases more seamlessly. Think of them as power-ups for your API. For example, you might use a package like djangorestframework-simplejwt for super-easy JSON Web Token (JWT) authentication – way simpler than rolling your own solution. This saves you tons of time and effort, and ensures you're using a well-tested, secure method.
Another great example is integrating with a powerful search library like django-elasticsearch-dsl. If you're building an API for a large dataset, this can dramatically improve search performance and make your API much more responsive. Imagine trying to search through millions of records without it – a nightmare! These packages significantly improve the overall user experience of your API. Properly integrating them into your Django REST Framework application requires careful planning and consideration of your project's specific needs.
Customizing Your DRF API Behavior
Advanced Django REST Framework Techniques
Beyond just adding libraries, you can also extensively customize DRF's core behavior. Need to change how serialization works? DRF provides extensibility points to tweak almost every aspect of its functionality. You can create custom serializers, viewsets, and even override existing functionality to perfectly match your project's needs. This level of control is what makes DRF so powerful and adaptable.
Creating Custom Django REST Framework Serializers
Serializers are the heart of DRF, converting Python objects into JSON (and vice versa). Creating custom serializers lets you tailor the data transformation to exactly what your API needs to expose. You can add custom fields, modify existing ones, and even implement complex logic within the serialization process. Check out the official DRF documentation on serializers for more details. Learning to build these is a core skill for any serious DRF developer.
Extending Django REST Framework ViewSets
ViewSets provide a clean, organized way to build your API endpoints. But sometimes, you need something more. You can create custom ViewSets that inherit from the base DRF classes, adding or overriding methods to handle specific logic. This lets you create truly unique API behaviors without rewriting everything from scratch. For example, you might create a custom ViewSet to handle batch operations or implement complex permission systems.
More Advanced Django REST Framework Capabilities
Mastering DRF's Throttling Mechanisms
DRF offers built-in throttling to prevent abuse of your API. You can customize this further by creating your own throttling classes to enforce specific rate limits based on factors like IP address or user authentication. This is critical for maintaining API stability and performance, especially when dealing with high-traffic situations.
The Future of Django REST Framework and API Development
Emerging Trends in API Development
The world of APIs is constantly evolving, right? We're seeing a huge push towards microservices architectures. This means breaking down large applications into smaller, independent services that communicate with each other through APIs. This approach offers increased flexibility and scalability, but it also increases the complexity of managing those interactions. GraphQL, a query language for APIs, is gaining major traction. Unlike REST, which often returns more data than needed, GraphQL lets clients request exactly what they need, improving efficiency. Another important trend is the rise of serverless architectures – think AWS Lambda or Google Cloud Functions. These let you run code without managing servers, which is awesome for cost savings and scalability. But, you still need a robust framework like Django REST Framework to manage your API interactions, even in a serverless environment. Learn more about microservices architecture at Microservices.io.
The Role of Django REST Framework in Modern Applications
Django REST Framework (DRF) remains a top choice for building APIs in Python. Its mature ecosystem, excellent documentation, and strong community support make it a reliable and powerful tool. DRF simplifies the process of creating RESTful APIs, handling tasks like serialization, authentication, and permissions with elegance. It integrates seamlessly with Django, allowing you to leverage Django's robust features like its ORM (Object-Relational Mapper) for database interactions. This means less boilerplate code and faster development times. DRF is particularly well-suited for building complex, data-rich APIs commonly found in modern applications. Check out the official Django REST Framework documentation for a deeper dive: Django REST Framework Docs.
Django REST Framework: Authentication and Authorization
Security is paramount in API development. DRF offers several built-in authentication mechanisms, including token-based authentication, session authentication, and OAuth2 support. These features help ensure that only authorized clients can access your API resources. Moreover, DRF simplifies the implementation of authorization, allowing you to define fine-grained permissions to control access to specific data or functionalities. Understanding these security aspects is critical for building robust and secure APIs. Read more about DRF's authentication options here: DRF Authentication.
Future Directions for Django REST Framework
The future looks bright for DRF! We can anticipate continued improvements in performance, enhanced security features, and better integration with other cutting-edge technologies. The DRF community is active and responsive, so expect regular updates and new capabilities. Further exploration into asynchronous task handling and improved support for GraphQL might also be on the horizon. Keeping up-to-date with the latest releases and community discussions is key for any DRF developer. This blog post will be updated with those changes as they emerge, so bookmark us!
Leveraging Django REST Framework for Scalability
Building scalable APIs is crucial for applications that experience high traffic. Django REST Framework helps achieve this through its efficient design and its ability to integrate with various caching and database optimization techniques. Properly using DRF's features like pagination and throttling will greatly improve performance under load. Consider learning about database optimization strategies to further enhance scalability for your Django REST Framework projects. For more on scaling your Django applications, see Django Database Optimization.
Frequently Asked Questions
FAQ 1: How do I handle authentication in Django REST Framework?
Django REST Framework offers a flexible authentication system. You can choose from several built-in authentication classes, such as SessionAuthentication (using Django's built-in sessions), BasicAuthentication (HTTP Basic Auth), TokenAuthentication (using API tokens), or OAuth2 authentication. You typically configure authentication in your settings.py
file by setting the DEFAULT_AUTHENTICATION_CLASSES
within REST_FRAMEWORK
. For example, to use TokenAuthentication, you would add rest_framework.authentication.TokenAuthentication
to that setting. Then you'd need to generate and manage tokens for your users. For more complex authentication needs, you might create custom authentication classes inheriting from BaseAuthentication
to integrate with your existing authentication system or a third-party provider. Remember to choose an authentication method that suits your security needs and application's complexity. Consider factors like scalability, ease of implementation, and the security risks associated with each approach.
FAQ 2: What are the different serializer types in Django REST Framework?
Django REST Framework primarily uses two serializer types: ModelSerializers and Serializers. ModelSerializers are designed to work directly with Django models. They automatically generate fields based on your model's fields, simplifying the process significantly. You define which fields to include or exclude, and they handle the data transformation between your model instances and their JSON representation. Serializers are more general-purpose and are used when you're not directly dealing with Django models, perhaps when working with complex data structures or external APIs. You explicitly define all fields within the serializer class, giving you more control. Choosing between them depends on your data source and how much control you need over the serialization process. Both types offer features for field validation, nested serialization, and data transformations to customize the output.
FAQ 3: How do I implement pagination in my Django REST Framework API?
Pagination is crucial for handling large datasets efficiently. Django REST Framework offers several built-in pagination classes, such as PageNumberPagination
, LimitOffsetPagination
, and CursorPagination
. You select one by setting the DEFAULT_PAGINATION_CLASS
within REST_FRAMEWORK
in your settings.py
. For example, to use PageNumberPagination
, you would add rest_framework.pagination.PageNumberPagination
. This allows users to retrieve data in pages defined by a page number and page size. Each pagination class has its own advantages and disadvantages. PageNumberPagination
is simple and intuitive; LimitOffsetPagination
is suitable for APIs that rely on limits and offsets; and CursorPagination
is best for APIs needing efficient navigation through ordered data. You can customize the page size and other pagination settings as needed. It's highly recommended to implement pagination for a better user experience and to prevent overwhelming the client with massive datasets.
FAQ 4: What are the best practices for securing my Django REST Framework API?
Securing your DRF API is paramount. Start with robust authentication (as discussed in FAQ 1). Implement HTTPS to encrypt communication between the client and server. Use appropriate HTTP methods (GET for reading, POST for creating, PUT for updating, DELETE for deleting) and ensure that your views enforce these properly. Validate all incoming data rigorously using serializers to prevent injection attacks. Implement input sanitization to prevent cross-site scripting (XSS) attacks. Regularly update your Django, DRF, and other dependencies to patch security vulnerabilities. Consider rate limiting to mitigate denial-of-service attacks. Implement proper authorization (permissions) to restrict access based on user roles or other criteria. Regularly audit your API logs for suspicious activity. Use a web application firewall (WAF) to further protect your API from common attacks. Remember that security is an ongoing process and requires vigilance.
FAQ 5: How can I test my Django REST Framework API?
Testing is crucial for reliable APIs. You can use Django's built-in testing framework combined with DRF's test client to make requests to your API endpoints and verify the responses. Write unit tests to test individual components (serializers, views, etc.), and integration tests to test the interaction between multiple components. Use tools like pytest for improved test organization and reporting. Focus on testing different scenarios, including successful requests, error handling, and edge cases. Employ mocking and test doubles to isolate units under test and increase speed and reliability. Consider using tools that allow for testing API responses (e.g., checking status codes, response content). Well-structured tests make it easier to identify and fix bugs, leading to a more robust and reliable API.
FAQ 6: How do I deploy a Django REST Framework application?
Deployment options vary depending on your application's scale and requirements. Simple deployments might involve using a WSGI server like Gunicorn or uWSGI behind a reverse proxy such as Nginx or Apache. For larger applications, consider using containerization technologies like Docker for easier deployment and portability across different environments. Cloud platforms like AWS, Google Cloud, or Azure offer managed services like Elastic Beanstalk or Kubernetes for more robust and scalable deployments. Remember to configure your environment variables, database connections, and other settings appropriately for your deployment environment. Use a process manager like Supervisor or systemd to manage your application processes and ensure they restart automatically if they crash. Consider load balancing to distribute traffic across multiple instances for improved scalability and availability.
FAQ 7: What are some common errors when using Django REST Framework?
Common errors include incorrect serializer field definitions leading to validation errors or unexpected data transformations. Authentication and authorization issues are also frequent, often caused by misconfiguration or incorrect implementation of permissions. Problems with database queries can lead to performance bottlenecks or unexpected behavior. Improper handling of exceptions can result in unhelpful error messages for clients. Incorrect use of pagination can lead to unexpected results or inefficient data retrieval. Using the wrong HTTP methods or neglecting to handle them properly can create security risks. Careless error handling can expose sensitive information and make debugging difficult. Always carefully examine error messages, logs, and your code for clues to resolve these issues.
FAQ 8: How can I optimize my Django REST Framework API for performance?
Optimizing DRF APIs involves several strategies. Database optimization is key; use efficient queries, indexing, and database caching. Implement caching mechanisms (like Redis or Memcached) to reduce database load. Use serializers effectively to avoid unnecessary data transformations. Employ pagination to limit the amount of data returned in each response. Optimize your views to reduce redundant calculations and database calls. Use asynchronous tasks (Celery) for long-running operations to prevent blocking the main thread. Profile your application to identify performance bottlenecks and prioritize optimization efforts based on the results. Consider using a load balancer to distribute traffic across multiple instances and use CDNs to serve static assets closer to users.
FAQ 9: What are some alternatives to Django REST Framework for API development?
Alternatives to DRF include FastAPI (a modern, high-performance framework emphasizing speed and ease of use), Flask-RESTful (a lightweight extension for Flask), and Falcon (a minimalist framework focusing on performance). The best choice depends on your project's needs and preferences. DRF excels in its maturity, extensive documentation, and integration with Django's ecosystem. FastAPI is gaining popularity for its speed and modern features. Flask-RESTful provides a simpler alternative when working within a Flask application. Falcon offers high performance but with less built-in functionality. Consider the trade-offs between ease of use, performance, and community support when making your selection.
FAQ 10: How do I handle errors gracefully in my Django REST Framework API?
Graceful error handling provides informative and user-friendly responses to clients. Use Django's exception handling mechanisms to catch and process errors appropriately. Create custom exception classes that provide context-specific error messages. Use DRF's APIView
or GenericAPIView
classes to handle exceptions centrally and return standardized error responses. Consider using HTTP status codes accurately (e.g., 400 Bad Request, 404 Not Found, 500 Internal Server Error) to indicate the type of error. Include clear and concise error messages in the response payload, avoiding technical details that might confuse the client. In development, provide more verbose error messages for debugging, but keep production error messages concise and informative to prevent revealing sensitive information. Logging errors is crucial for debugging and monitoring your API's health.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
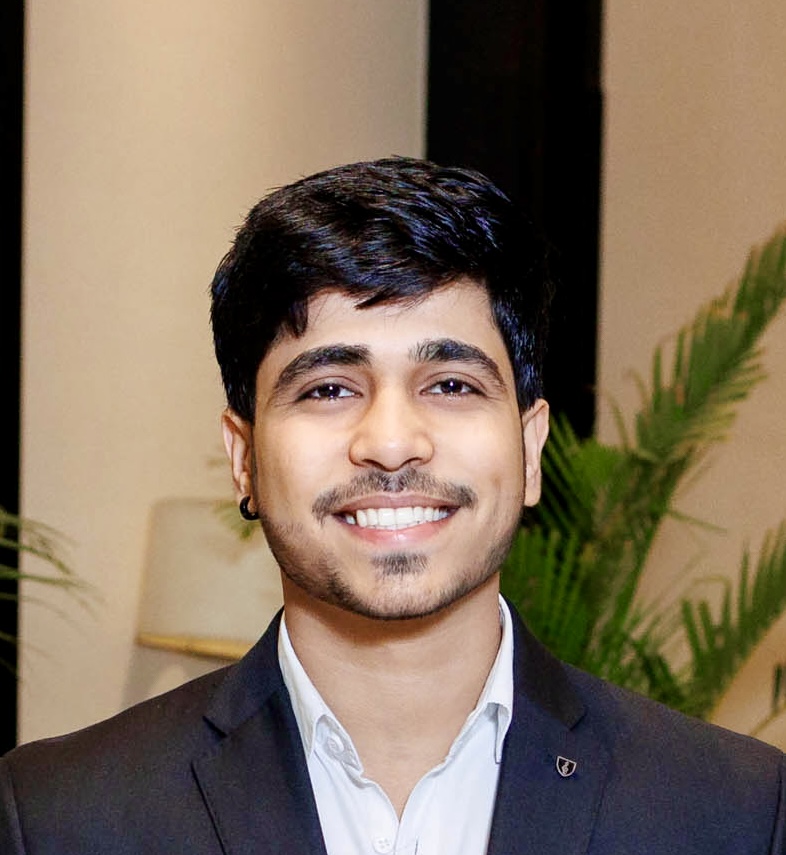
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.