Mastering Shell Scripting: A Beginner's Guide with Code Examples

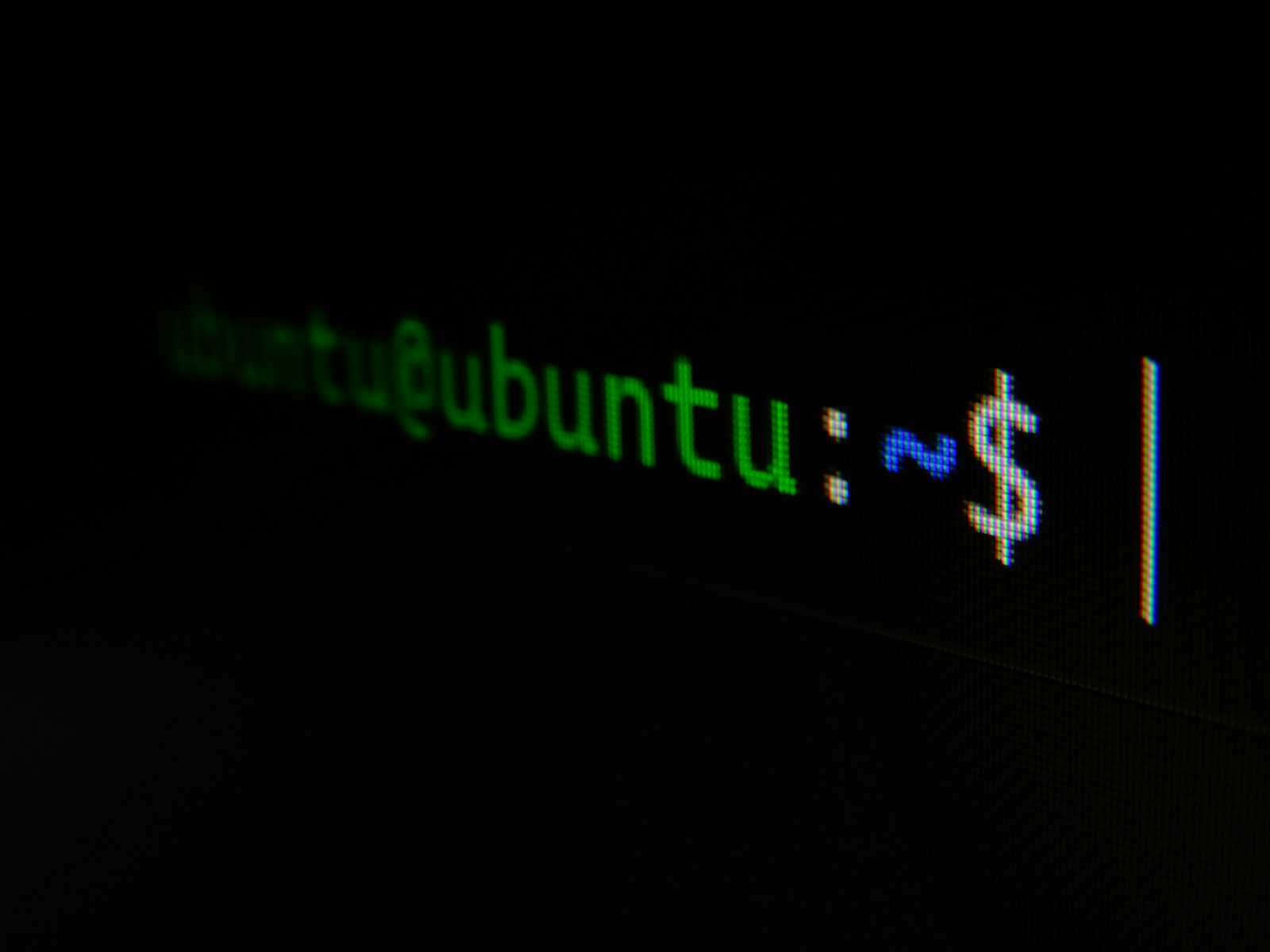
Introduction
Shell scripting is a powerful way to automate repetitive tasks in Linux. Whether you're a beginner or an aspiring DevOps engineer, understanding shell scripting is essential. In this blog, we’ll explore the fundamentals with practical examples and their outputs.
1. Creating and Executing a Shell Script
A shell script is a file containing multiple commands that execute sequentially.
Example:
vim myfile.sh
Inside myfile.sh
, add:
date
cal
ls
Save the file and execute it using:
bash myfile.sh
Output:
Mon Mar 31 12:30:00 UTC 2025
March 2025
Su Mo Tu We Th Fr Sa
1
2 3 4 5 6 7 8
9 10 11 12 13 14 15
...
file1.txt file2.sh myfile.sh
2. Variables in Shell
Declaring and Using Variables
x=5
echo $x
Output:
5
Unsetting Variables
unset x
echo $x
Output: (Nothing prints as x is removed)
String Variables and Expansion
x=pop
echo "I am piyush, hi $x how are you"
Output:
I am piyush hi pop how are you
Using {}
for expansion:
echo "I am piyush am in ${x}th class"
Output:
I am piyush am in popth class
3. Command Substitution
Command substitution allows capturing command output in a variable.
x=$(date)
echo $x
Alternative:
y=`date`
echo $y
Both methods store the current date inside x
or y
.
Output:
Mon Mar 31 12:30:00 UTC 2025
4. Using read
to Take User Input
read p
echo $p
Example with prompt:
read -p "Enter your name: " n
echo $n
Limiting Input Length:
read -p "Enter your name: " -n 2 myname
echo $myname
5. Creating a Script That Creates a File
vim my.sh
Inside my.sh
:
read -p "Enter your file name : " myfile
touch $myfile
Execute:
bash my.sh
Output:
Enter your file name: hello.txt
This creates hello.txt
in the current directory.
6. Exit Status ($?
)
Exit status helps determine if a command ran successfully.
date
echo $?
Output:
0 # (Success)
For an invalid command:
date123
echo $?
Output:
127 # (Command not found)
7. test
Command for Comparisons
String Comparison
test "hi" == "hi"
echo $?
Output:
0 # (True)
test "hi" == "hello"
echo $?
Output:
1 # (False)
Integer Comparison
test 5 -eq 5
echo $?
Output:
0
Other integer operators:
-ne
→ Not equal to-lt
→ Less than-le
→ Less than or equal to-gt
→ Greater than-ge
→ Greater than or equal to
8. Running Multiple Commands
date; cal; ls
Output:
Mon Mar 31 12:30:00 UTC 2025
March 2025
Su Mo Tu We Th Fr Sa
...
file1.txt file2.sh myfile.sh
If a command fails, subsequent commands still execute:
date1; cal; ls
Output:
bash: date1: command not found
March 2025
file1.txt file2.sh myfile.sh
Key Takeaways
✅ Scripts execute commands sequentially.
✅ Variables store values (use $var
to retrieve).
✅ {}
is necessary when appending text to variables.
✅ Use $(command)
for command substitution.
✅ Use read
for user input.
✅ $?
shows exit status (0 = success, non-zero = failure).
✅ test
command checks conditions (strings/numbers).
✅ ;
separates commands (executes all regardless of failure).
Conclusion
Shell scripting is an essential skill for any Linux user. Mastering the basics will help you automate tasks efficiently. Try modifying these scripts and experiment further to deepen your understanding!
Subscribe to my newsletter
Read articles from Piyush Kabra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
