Mastering Comparator in Java – Sorting Made Fun!

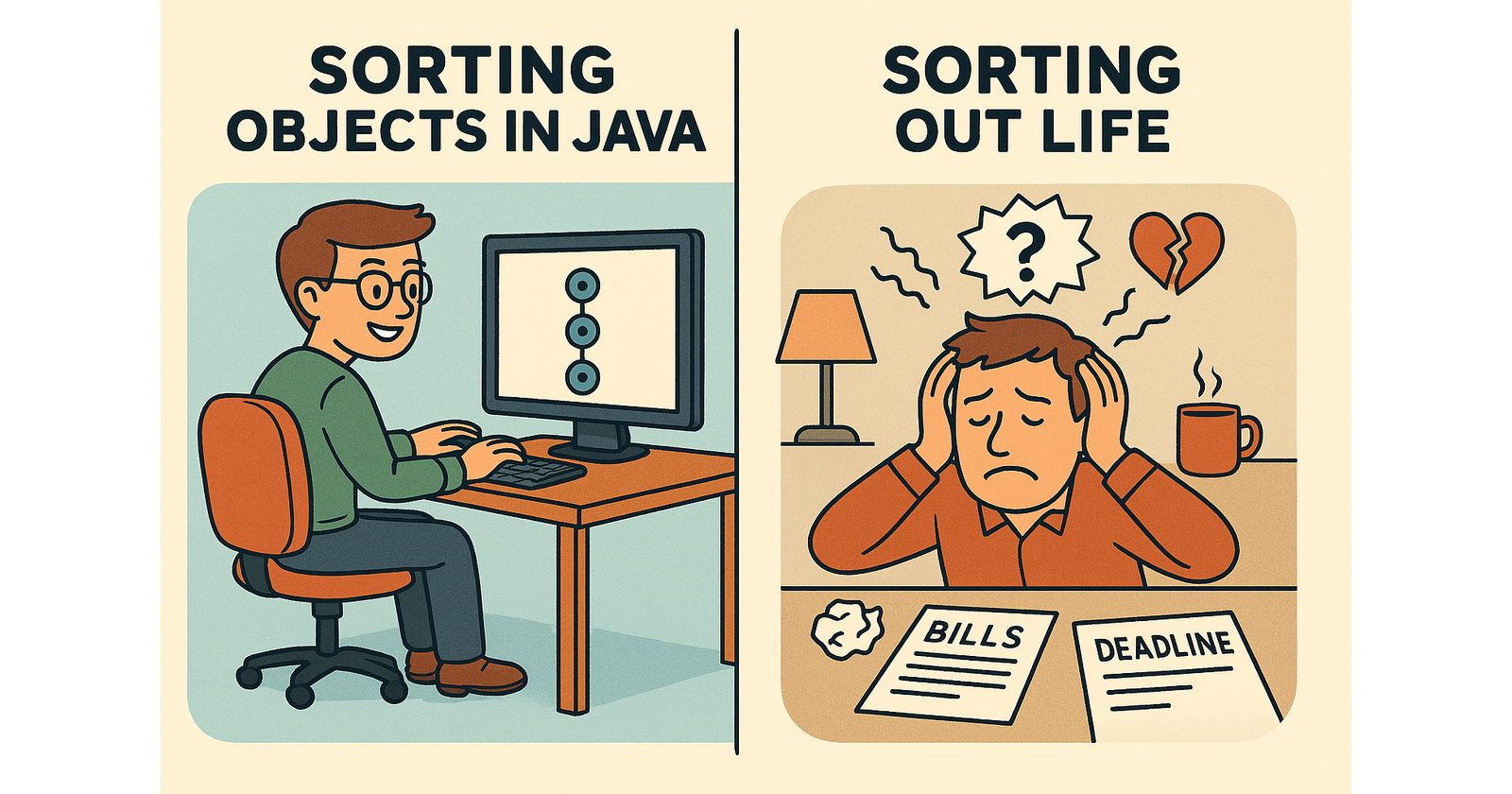
Sorting is like cleaning our room something we know we should do but often put off. Thankfully, Java’s Comparator
makes sorting so effortless that we can stop procrastinating and actually get it done (or at least, pretend to).
The Basics – Comparator 101
Before we dive into the deep waters, let’s wade through the basics. The Comparator
interface in Java helps sort objects in a custom manner, beyond the natural ordering defined by Comparable
.
Integer Comparison – The Simple Way
Need to compare two numbers? Java’s Integer.compare(a, b)
method has our back:
int result = Integer.compare(10, 20);
System.out.println(result); // Output: -1 (because 10 < 20)
This method returns:
-1
ifa
is less thanb
0
ifa
is equal tob
1
ifa
is greater thanb
Easy, right? Now let’s level up.
Lambda Expressions – Comparators Made Elegant
Before Java 8, writing a comparator meant creating an anonymous class, which felt like writing a novel just to compare two numbers. With lambdas, we can write comparators concisely:
Comparator<Integer> comparator = (a, b) -> Integer.compare(a, b);
Or even shorter:
Comparator<Integer> comparator = Integer::compare;
Now that’s what I call elegant!
Custom Comparator – Because Life is More Than Just Numbers (I know that’s an arguable topic, but let’s just move on)
Comparing numbers is fun, but what if we have a list of people and want to sort them by age?
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
}
Let’s create a comparator for sorting people by age:
Comparator<Person> ageComparator = (p1, p2) -> Integer.compare(p1.age, p2.age);
Or, using a method reference:
Comparator<Person> ageComparator = Comparator.comparingInt(Person::age);
Simple and powerful!
Deep Dive – Unlocking Comparator’s Full Potential
comparing()
– The Foundation of Great Sorting
The comparing
method lets us create comparators based on key extractors. For example, sorting people by name:
Comparator<Person> nameComparator = Comparator.comparing(p -> p.name);
Or even shorter with a method reference:
Comparator<Person> nameComparator = Comparator.comparing(Person::name);
thenComparing()
– Because Tiebreakers Matter
Let’s be real, when was the last time we sorted a list using just one field? Never. That’s why thenComparing()
exists to keep our sorting as fair as possible.
Comparator<Person> personComparator = Comparator.comparing(Person::name)
.thenComparingInt(Person::age);
Now, if names match, we compare by age.
reversed()
– Because Sometimes We Want to Go Backwards
Sorting in reverse? Just add .reversed()
and watch the world burn (or at least, your list go backward).
Comparator<Person> reverseAgeComparator = Comparator.comparingInt(Person::age).reversed();
It’s like sorting, but in reverse because sometimes chaos is fun.
Wrapping Up
Java’s Comparator
interface is a powerful tool for sorting objects with elegance and flexibility. Whether we’re comparing numbers, strings, or custom objects, we now have a Swiss Army knife for sorting.
So go forth and sort! And remember—if sorting objects ever frustrates you, just be glad it's easier than sorting out life’s mess. (No human is intentionally offended in this article. If this offends you, just call ExceptionHandler.handle()
and move on 😜)
What’s That ::
Thing We Used?
the ::
operator in Java is called the method reference operator. It provides a shorthand way to refer to methods and constructors without explicitly invoking them. It's a powerful tool that deserves a separate article of its own.
Stay tuned we'll be diving into method references soon! 🚀
Subscribe to my newsletter
Read articles from Santhosh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santhosh
Santhosh
I’m a software developer passionate about exploring new technologies and continuously learning. On my blog, I share what I discover—whether it’s cool tricks, coding solutions, or interesting tools. My goal is to document my journey and help others by sharing insights that I find useful along the way. Join me as I write about: Programming tips & tricks Lessons from everyday coding challenges Interesting tools & frameworks I come across Always curious, always learning—let’s grow together! 🚀