Arrays v/s Sets

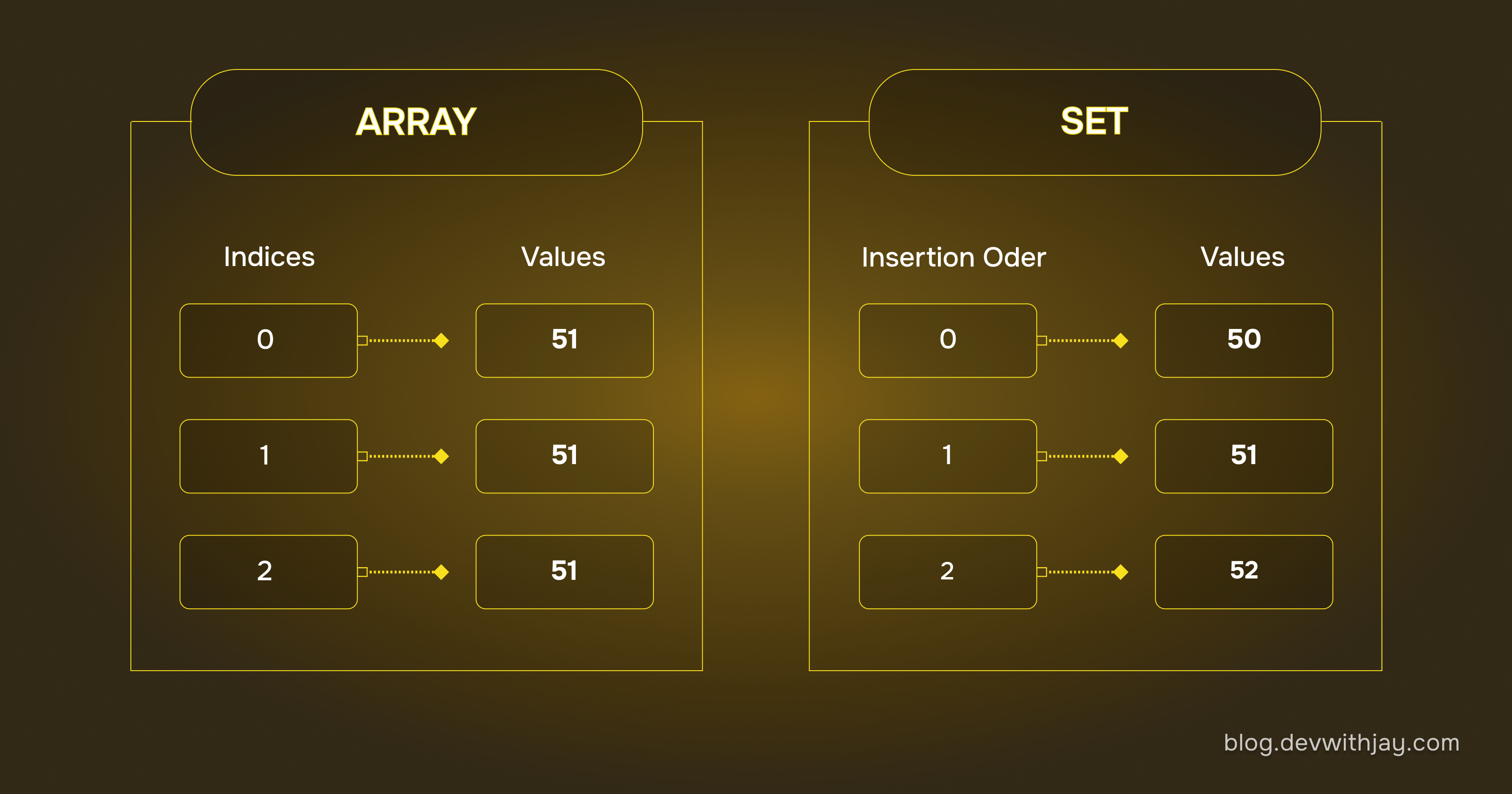
Introduction
In JavaScript, Arrays and Sets are both used to store collections of values, but they serve different purposes and have distinct characteristics. While Arrays allow you to store ordered collections and can contain duplicate values, Sets are designed to store unique values and automatically remove duplicates.
In this article, we’ll understand the differences between Arrays and Sets, their performance characteristics, and practical use cases where each is most effective.
What is a Set?
A Set is a special type of collection in JavaScript that stores unique values only. Unlike arrays or objects, a Set doesn’t use keys, and it doesn’t allow duplicate values.
Sets can store values of any type: strings, numbers, objects, or even other Sets. They’re useful when you want to keep a list of items without duplicates, such as a list of unique user IDs, selected tags, or visited pages.
Creating A Set
In JavaScript, a Set can be created using the Set constructor.
Creating an Empty Set
let mySet = new Set();
This creates a Set that doesn’t contain any values.
Creating a Set with Initial Values
You can also create a Set and initialize it with values by passing an array or another iterable to the constructor:
let mySet = new Set([1, 2, 3, 4, 5]);
This creates a Set with the values 1, 2, 3, 4, 5.
Adding Values to a Set
You can add individual values to a Set using the
.add()
method:let mySet = new Set(); mySet.add(1); mySet.add(2); mySet.add(3);
Methods & Properties
new Set([iterable]) – Creates a Set. If an iterable object (usually an array) is provided, it copies the values from it into the Set.
set.add(value) – Adds a value to the Set. It returns the Set itself. If the value already exists in the Set, it does nothing (i.e., Sets only store unique values).
set.delete(value) – Removes the specified value from the Set. It returns true if the value existed at the moment of the call, and false otherwise.
set.has(value) – Returns true if the specified value exists in the Set, and false otherwise.
set.clear() – Removes all elements from the Set.
set.size – Returns the number of elements in the Set.
The main feature of a Set is that repeated calls to set.add(value) with the same value don’t add the value again. This ensures that each value appears in a Set only once.
Example:
const set = new Set([1, 2, 2, 3]);
console.log([...set]); // [1, 2, 3]
Chaining Methods
Just like with Maps, you can chain multiple method calls together for cleaner and more concise code when working with Sets.
let userSet = new Set()
.add('Jay')
.add(20)
.add('developer');
Iteration Over Set
You can loop over a Set using either the for...of loop or the forEach() method.
Using for...of Loop
let set = new Set(["oranges", "apples", "bananas"]); for (let value of set) { alert(value); }
Using forEach() Method
You can also use set.forEach() to iterate over the values in a Set. The callback function passed to forEach() takes three arguments:
set.forEach((value, valueAgain, set) => { alert(value); });
value: The current value of the Set.
valueAgain: This is the same value as value. It is included for compatibility with Map, where the callback also receives three arguments.
set: The Set object itself.
This might seem odd because the value appears twice, but it’s there for consistency with the Map object, which uses three arguments in its forEach() method.
Additional Methods for Iterating
Like Map, the Set object also supports the following iterator methods:
set.keys() – Returns an iterable for the values in the Set (same as set.values()).
set.values() – Same as set.keys() for compatibility with Map.
set.entries() – Returns an iterable for entries [value, value]. This exists for compatibility with Map.
These methods are useful if you want to work with specific parts of a Set, similar to how they are used in a Map.
Array to Sets
Normally, when you create an array, it can contain duplicate values. But sometimes, you may want to remove duplicates from an array, and this is where Sets come in handy, as they only store unique values.
To convert an array into a Set, you can pass the array directly into the Set constructor.
let array = [1, 2, 3, 3, 4, 5, 5];
let set = new Set(array);
console.log(set); // Set { 1, 2, 3, 4, 5 }
Set to Array
Converting a Set back to an Array is just as easy. You can use the Array.from() method or the spread operator (...) to convert a Set into an array.
Using Array.from():
let set = new Set([1, 2, 3, 4, 5]);
let array = Array.from(set);
console.log(array); // [1, 2, 3, 4, 5]
Using Spread Operator:
let array = [...set];
console.log(array); // [1, 2, 3, 4, 5]
Why Convert Set to Array?
You might want to convert a Set back to an Array in cases where:
You need to work with array-specific methods like .map(), .filter(), or .reduce().
You need to pass data to a function that expects an array.
Performance Benefits of Sets
Fast Lookups: Sets allow quick checks to see if a value exists. The time to check if an item is in a Set is constant (O(1)), making it faster than arrays or objects, especially when dealing with a large number of values.
Unique Values: Sets automatically ensure that all values are unique. You don’t need to manually check for duplicates, which saves time and reduces the complexity of your code.
Efficient Memory Usage: Since Sets only store unique values and don’t store duplicate entries, they use memory more efficiently than arrays when managing large collections of data.
No Need for Indexing: Unlike arrays, Sets don’t rely on indexing. This can make operations like checking for the presence of an item faster and easier, without the overhead of index management.
Order Preservation: Just like Maps, Sets maintain the order of items as they are added. This allows for predictable iteration when looping through the Set.
Practical Applications of Sets
Removing Duplicates: Automatically remove duplicates from a list, like user IDs or tags.
Tracking Visited Pages: Store URLs of visited pages without repeats.
Managing Permissions: Track unique user permissions or actions, like ‘edit’ or ‘view’.
Unique Items Collection: Keep a collection of unique items, like feedback or tags.
Real-World Examples
Unique Tags on a Blog: On a blog, you might want to track tags that have been used across posts. A Set can store each tag as a unique value, automatically removing duplicates, so you don’t repeat tags.
Tracking Visited Websites: If you’re building a browser extension that tracks visited websites, a Set can be used to store each URL only once. When the user visits a new site, you can check if it’s already in the Set before adding it.
Membership List: A Set can be used to store unique membership IDs of users in a club or organization. This ensures no duplicate memberships and allows fast checks to see if someone is a member.
Differences Between Arrays and Sets
Feature | Array | Set |
Duplicates | Allows duplicates (values can repeat). | Does not allow duplicate values (each value is unique). |
Order | Elements are ordered by index (0, 1, 2, …). | Elements are ordered by the insertion order of values. |
Data Types | Can store values of any type, including mixed types. | Can store values of any type, but each value is unique. |
Access | Elements can be accessed by their index (e.g., array[0]). | Cannot be accessed by index; only through iteration. |
Methods | Methods like .push(), .pop(), .shift(), .map(), .filter(), etc. | Methods like .add(), .delete(), .has(), .clear(), etc. |
Performance | Slower for membership checking and removal due to the need to scan the array. | Faster lookups for membership (constant time O(1) complexity). |
Use Case | Ideal for ordered collections where index-based access is important, and duplicates are allowed. | Ideal for collections where uniqueness is required and fast membership checks are needed. |
Iteration | Iterates over elements by index (e.g., for...of, .forEach() on values). | Iterates over values in insertion order (e.g., for...of, .forEach() on values). |
Size Property | .length gives the number of elements. | .size gives the number of unique values. |
Methods for Filtering | Can use .filter() and .map() to manipulate or filter values. | Cannot directly use .map() or .filter() (as it’s designed for unique values). |
This comparison highlights the differences between arrays and sets, making it easier to choose the appropriate data structure based on the requirements of your project.
When To Use Sets
Choose Sets when you need:
A collection of unique values with no duplicates.
Automatic removal of duplicates when adding new values.
Fast checks to see if a value exists in the collection.
Conclusion
In conclusion, Arrays are great when you need to keep things in a specific order or when duplicates are okay. On the other hand, Sets are perfect for storing unique items and checking quickly if something is already there, without worrying about duplicates.
By understanding these differences, you can choose the right data structure for your needs and write cleaner, more efficient code.
Want More…?
I write articles on blog.devwithjay.com and also post development-related content on the following platforms:
Subscribe to my newsletter
Read articles from Jay Kadlag directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
