Stateless Bottom Navigation in Flutter: Using GetX, Riverpod, and Bloc Effectively

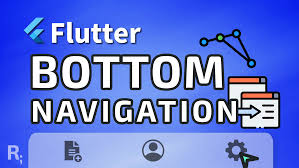
Flutter’s declarative UI paradigm encourages stateless navigation, where navigation logic is detached from widget state. Unlike traditional imperative navigation, which relies on managing routes through widget lifecycle changes, stateless navigation leverages the declarative approach to improve app maintainability, scalability, and testability.
Why Use Stateless Navigation?
Predictability: Eliminates hidden state dependencies in navigation.
Performance Optimization: Prevents unnecessary widget rebuilds.
Better Testability: Simplifies unit and UI testing.
Improved Code Maintainability: Keeps navigation logic independent from UI state.
Implementing Stateless Navigation with Different State Management Tools
1. GetX: Simple and Reactive Navigation
GetX offers a lightweight approach to navigation, with dependency injection and reactive state management.
Implementation
import 'package:flutter/material.dart';
import 'package:get/get.dart'; // GetX package for state management
import 'package:iconsax/iconsax.dart'; // Icons package for better UI icons
// Controller class to manage navigation state using GetX
class NavigationController extends GetxController {
// Observable integer to track the selected index of the bottom navigation bar
final Rx<int> selectedIndex = 0.obs;
// List of screens corresponding to each navigation destination
final screens = [
const HomeScreen(), // Home screen
const StoreScreen(), // Store screen
const WishlistScreen(), // Wishlist screen
const ProfileScreen() // Profile screen
];
}
// Stateless widget that builds the bottom navigation menu
class NavigationMenu extends StatelessWidget {
const NavigationMenu({super.key});
@override
Widget build(BuildContext context) {
// Initialize the navigation controller using GetX dependency injection
final controller = Get.put(NavigationController());
return Scaffold(
// Bottom navigation bar with reactive state management using Obx
bottomNavigationBar: Obx(() => NavigationBar(
// Set the selected index dynamically from the controller
selectedIndex: controller.selectedIndex.value,
// Update the selected index when a navigation item is tapped
onDestinationSelected: (index) =>
controller.selectedIndex.value = index,
// Navigation bar items with icons and labels
destinations: const [
NavigationDestination(icon: Icon(Iconsax.home), label: 'Home'),
NavigationDestination(icon: Icon(Iconsax.message), label: 'Chat'),
NavigationDestination(icon: Icon(Iconsax.location), label: 'Favorite'),
NavigationDestination(icon: Icon(Iconsax.calendar), label: 'Profile'),
])),
// Display the currently selected screen dynamically
body: Obx(() => controller.screens[controller.selectedIndex.value]),
);
}
}
Best Practices
Use
Get.put()
for dependency injection.Implement
Obx
to manage reactive state efficiently.Encapsulate navigation logic within a controller.
2. Riverpod: State-Driven Navigation
Riverpod provides provider-based state management, allowing navigation to be purely state-driven.
Implementation
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart'; // Riverpod for state management
import 'package:iconsax/iconsax.dart'; // Icons package for a modern UI
// NavigationController manages the state of the selected navigation index
class NavigationController extends StateNotifier<int> {
// List of screens corresponding to each navigation item
final screens = [
const HomeScreen(), // Home screen
const MessageScreen(), // Chat screen
const MapScreen(), // Find screen (Map feature)
const ScheduleScreen(), // Schedule screen
];
// Constructor initializes the selected index to 0 (first screen)
NavigationController() : super(0);
// Getter to retrieve the current selected index
int get index => state;
// Method to update the selected index when a navigation item is tapped
void updateState(int index) => state = index;
}
// Riverpod provider to manage the navigation state globally
final navigationController = StateNotifierProvider<NavigationController, int>(
(ref) => NavigationController());
// ConsumerWidget to build the navigation UI with Riverpod state management
class NavigationMenu extends ConsumerWidget {
const NavigationMenu({super.key});
@override
Widget build(BuildContext context, WidgetRef ref) {
// Watching the navigation controller provider to update UI on state change
final controller = ref.watch(navigationController.notifier);
return Scaffold(
// Bottom navigation bar with interactive navigation items
bottomNavigationBar: NavigationBar(
// Update navigation state when an item is selected
onDestinationSelected: (index) => controller.updateState(index),
// Navigation items with corresponding icons and labels
destinations: const [
NavigationDestination(icon: Icon(Iconsax.home), label: 'Home'),
NavigationDestination(icon: Icon(Iconsax.message), label: 'Chat'),
NavigationDestination(icon: Icon(Iconsax.location), label: 'Find'),
NavigationDestination(icon: Icon(Iconsax.calendar), label: 'Schedule'),
],
),
// Display the currently selected screen dynamically
body: controller.screens[ref.watch(navigationController)],
);
}
}
Best Practices
Use
StateNotifierProvider
to manage navigation state.Avoid direct state mutation; use
updateState()
instead.Optimize widget rebuilds with
ConsumerWidget
.
3. Bloc: Event-Driven Navigation with GetIt
Bloc ensures event-driven state changes, and GetIt simplifies dependency injection.
Implementation
import 'package:beamer/beamer.dart'; // Beamer for navigation
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart'; // Bloc for state management
import 'package:iconsax/iconsax.dart'; // Iconsax for modern icons
import 'package:get_it/get_it.dart'; // GetIt for service locator pattern
// Service locator for dependency injection using GetIt
final serviceLocator = GetIt.instance;
// Registering NavigationCubit as a lazy singleton to ensure a single instance is used across the app
serviceLocator.registerLazySingleton(() => NavigationCubit());
// Bloc-based state management for handling navigation index
class NavigationCubit extends Cubit<int> {
NavigationCubit() : super(0); // Initial index set to 0 (Home screen)
// Method to update the navigation index
void updateIndex(int index) {
emit(index); // Emits a new state (index) to trigger UI updates
}
}
// Stateless widget representing the navigation menu
class NavigationMenu extends StatelessWidget {
const NavigationMenu({super.key});
// List of navigation icons (default and selected states) and labels
List<List> get screenIcons {
return [
[Icons.home_outlined, Icons.home_rounded, 'Home'], // Home tab
[Iconsax.search_normal, Iconsax.search_normal, 'Search'], // Search tab
[Iconsax.add_circle, Iconsax.add_circle5, 'Sell'], // Sell tab
[Iconsax.heart, Iconsax.heart5, 'Wishlist'], // Wishlist tab
[Iconsax.user, Icons.person_rounded, 'Account'], // Account tab
];
}
// List of route names corresponding to each navigation item
List<String> get labels {
return [
RouteNames.home, // Home route
RouteNames.discover, // Discover/Search route
RouteNames.sell, // Sell route
RouteNames.favourites, // Wishlist route
RouteNames.personalization, // Profile/Account route
];
}
// List of screens corresponding to each navigation tab
List<Widget> get screens {
return [
HomeScreen(), // Home screen widget
const DiscoverScreen(), // Discover/Search screen
const UploadProductScreen(), // Upload product screen (Sell tab)
const SupportScreen(), // Support/Wishlist screen
ProfileScreen(), // Profile screen
];
}
@override
Widget build(BuildContext context) {
final isDark = PHelperFunctions.isDarkMode(context); // Check if dark mode is enabled
return Scaffold(
// Bottom Navigation Bar with Bloc state management
bottomNavigationBar: BlocBuilder<NavigationCubit, int>(
builder: (context, state) {
return NavigationBar(
backgroundColor: isDark ? PColors.dark : PColors.white, // Dynamic background color based on theme
surfaceTintColor: PColors.transparent, // Transparent tint color
indicatorColor: PColors.transparent, // Transparent indicator for a clean UI
labelTextStyle: WidgetStateProperty.all(
const TextStyle(fontWeight: FontWeight.w500), // Styling for labels
),
selectedIndex: serviceLocator<NavigationCubit>().state, // Get current navigation index from NavigationCubit
onDestinationSelected: (index) {
serviceLocator<NavigationCubit>().updateIndex(index); // Update index when a tab is selected
},
destinations: screenIcons
.map(
(e) => NavigationDestination(
icon: Icon(
screenIcons[state].first == e.first ? e[1] : e[0], // Toggle between default and selected icons
color: screenIcons[state] == e ? PColors.primary : Colors.black, // Change icon color when selected
),
label: e[2], // Set label for the navigation item
),
)
.toList(),
);
},
),
// Display the currently selected screen
body: BlocBuilder<NavigationCubit, int>(
builder: (context, state) => screens[state],
),
);
}
}
Best Practices
Use
BlocBuilder
for efficient UI updates.Inject
NavigationCubit
usingGetIt
to decouple dependencies.Keep navigation state separate from UI components.
Conclusion
Stateless navigation improves the scalability of Flutter applications by separating navigation logic from UI state. Whether using GetX, Riverpod, or Bloc with GetIt, each approach offers a structured way to implement seamless, reactive navigation.
Which approach do you prefer?
Subscribe to my newsletter
Read articles from Diwe innocent directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
