Breaking Down the Database Dilemma: SQL and NoSQL Explained!

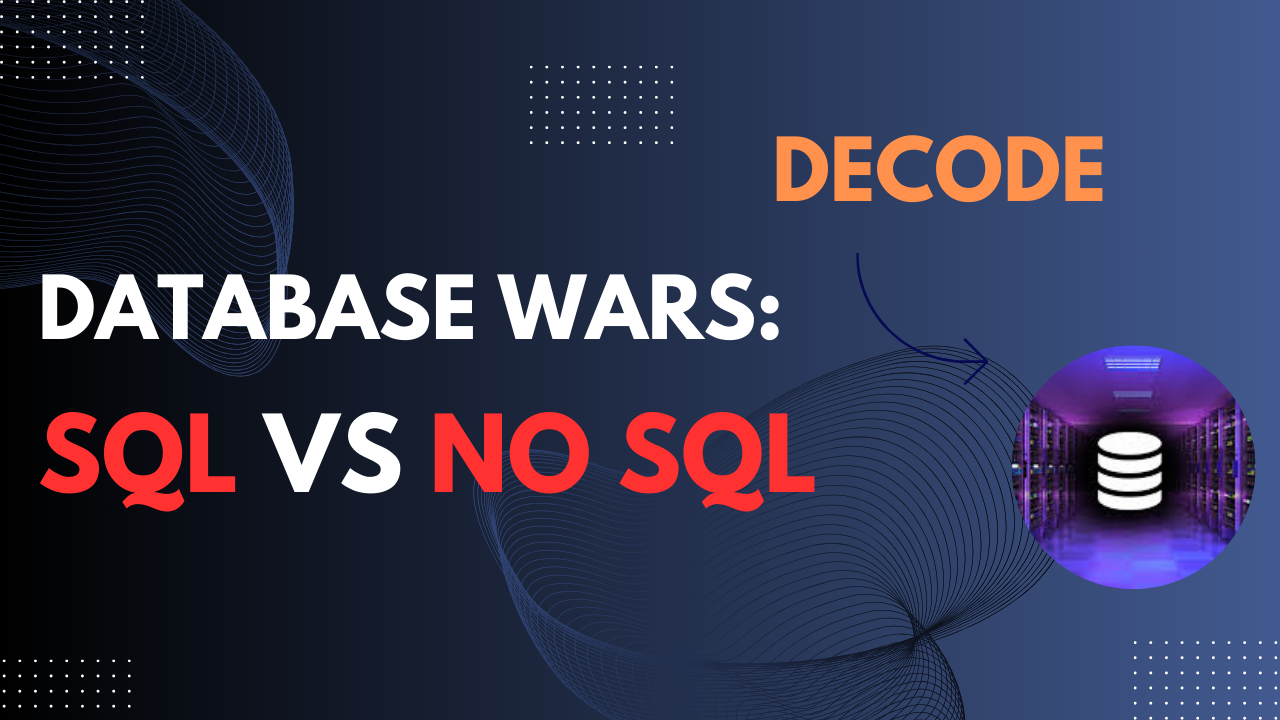
A database is an organized digital storage for data, making it easy to access, update, and manage. It's the backbone for apps and systems like Netflix profiles and Amazon product listings. Let’s break down the two main types—SQL and NoSQL—in plain language, with examples.
SQL Databases (Relational Databases)
What is SQL?
SQL (Structured Query Language) is the language used to communicate with relational databases. These databases store data in tables (like spreadsheets) with rows and columns. Each table represents a specific entity (e.g., "Users" or "Orders"), and tables can link to each other using relationships.
Key Features of SQL Databases:
Structured Data: Information is stored in tables with specific rules (like a "Users" table with columns for ID, Name, and Email), making it neat and organized.
ACID Compliance: Ensures transactions are reliable and safe:
Atomicity: Transactions are all-or-nothing.
Consistency: Data remains valid after transactions.
Isolation: Concurrent transactions don’t interfere.
Durability: Data is saved even if the system crashes.
Vertical Scalability: Handles more users or data by improving server hardware, like adding more RAM or a stronger CPU. SQL databases are designed to keep your data organized, safe, and ready to handle increased demand!
Examples of SQL Databases:
MySQL
PostgreSQL
Microsoft SQL Server
Neon DB (A serverless, scalable PostgreSQL-compatible database)
SQL Example (Structured Table):
Database: MySQL
-- Create a table for users
CREATE TABLE Users (
id INT PRIMARY KEY,
name VARCHAR(50),
email VARCHAR(100)
);
-- Insert data
INSERT INTO Users (id, name, email)
VALUES (1, 'iamuser', 'iamuser@example.com');
-- Query data
SELECT * FROM Users WHERE name = 'iamuser';
Result:
id | name | |
1 | Alice | alice@example.com |
SQL uses fixed tables (rows/columns).
NoSQL Databases (Non-Relational Databases)
What is NoSQL?
NoSQL databases are like free-spirited organizers for data! Unlike traditional databases, they don't need strict rules or fixed tables (schemas). They work great with messy or unpredictable data, such as user activity logs or social media posts. Instead of upgrading one big server when handling more data, NoSQL databases can grow by simply adding more servers—like expanding storage bins instead of making one bigger. This makes them fast, flexible, and ideal for handling large amounts of varied data!
Key Features of NoSQL Databases:
Flexible Schemas: Data formats can vary (e.g., a user profile with or without a phone number).
BASE Model: Focuses on availability over strict consistency:
Basically Available: System works even during failures.
Soft State: Data may change over time.
Eventually Consistent: Data becomes consistent eventually.
Horizontal Scalability: Distribute data across multiple servers.
Examples of NoSQL Databases:
MongoDB (Document)
Redis (Key-Value)
Cassandra (Column-Family)
Neo4j (Graph)
NoSQL Example (Flexible Document):
Database: MongoDB
// Insert a user document
{
"_id": "user123",
"name": "iamuser",
"email": "iamuser@example.com",
"address": {
"city": "Paris",
"country": "France"
}
}
// Query by name
db.users.find({ name: "iamuser" });
Result:
{
"_id": "user123",
"name": "Bob",
"email": "bob@example.com",
"address": { "city": "Paris", "country": "France" }
}
NoSQL uses flexible documents (nested data, no rigid schema).
SQL & NoSQL Together? (Hybrid Approach)
Many modern applications use both SQL & NoSQL. Example: A Netflix-like Platform
SQL: Stores user subscriptions & billing information (structured, consistent).
NoSQL: Stores watch history, recommendations, and logs (flexible, scalable).
This hybrid approach balances data integrity (SQL) with performance & flexibility (NoSQL).
SQL vs. NoSQL: When to Use What?
Use Case | SQL (Relational) | NoSQL (Non-Relational) |
Banking & Transactions | ✅ Strong consistency (ACID) | ❌ Not ideal due to BASE model |
E-commerce (Products, Orders) | ✅ Structured orders & payments | ✅ User behavior logs & recommendations |
Real-time Analytics (IoT, Logs) | ❌ Slower for big data | ✅ Optimized for fast processing |
Content Management (Blogs, Media) | ❌ Rigid schema limitations | ✅ Flexible storage for varied content |
Chat Applications | ❌ Might struggle with performance | ✅ Efficient for distributed storage |
Common Mistakes Students Make (and How to Avoid Them!)
SQL Pitfalls:
❌ Forgetting to index tables → Slow queries
❌ Overusing joins → Performance bottlenecks
❌ Not normalizing data → Wasted storage, redundant data
✅ Solution: Use indexes, normalize data, and optimize queries.
NoSQL Pitfalls:
❌ Not defining an efficient schema → Query performance issues
❌ Ignoring indexing → Slower reads
❌ Over-relying on flexible schemas → Data inconsistency
✅ Solution: Plan document structure ahead of time, use proper indexing, and balance flexibility with consistency.
Conclusion
Now that you have a solid understanding of SQL and NoSQL, experiment with both! Try setting up a simple MySQL database or building a small MongoDB project. Hands-on practice is the best way to master database management and find the right approach for your applications🚀.
Subscribe to my newsletter
Read articles from Akriti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akriti
Akriti
“Innovative developer with a passion for continuous learning and expertise in cutting-edge technologies. Proficient in frontend development, particularly ReactJS and Tailwind CSS, and currently expanding my skills in Node.js and backend development. Enthusiastic about creating seamless user experiences and optimizing performance. Always eager to explore new tools and frameworks to stay ahead in the tech world.”