Write Cleaner Express.js Code: The Ultimate Guide to Absolute Imports with TypeScript

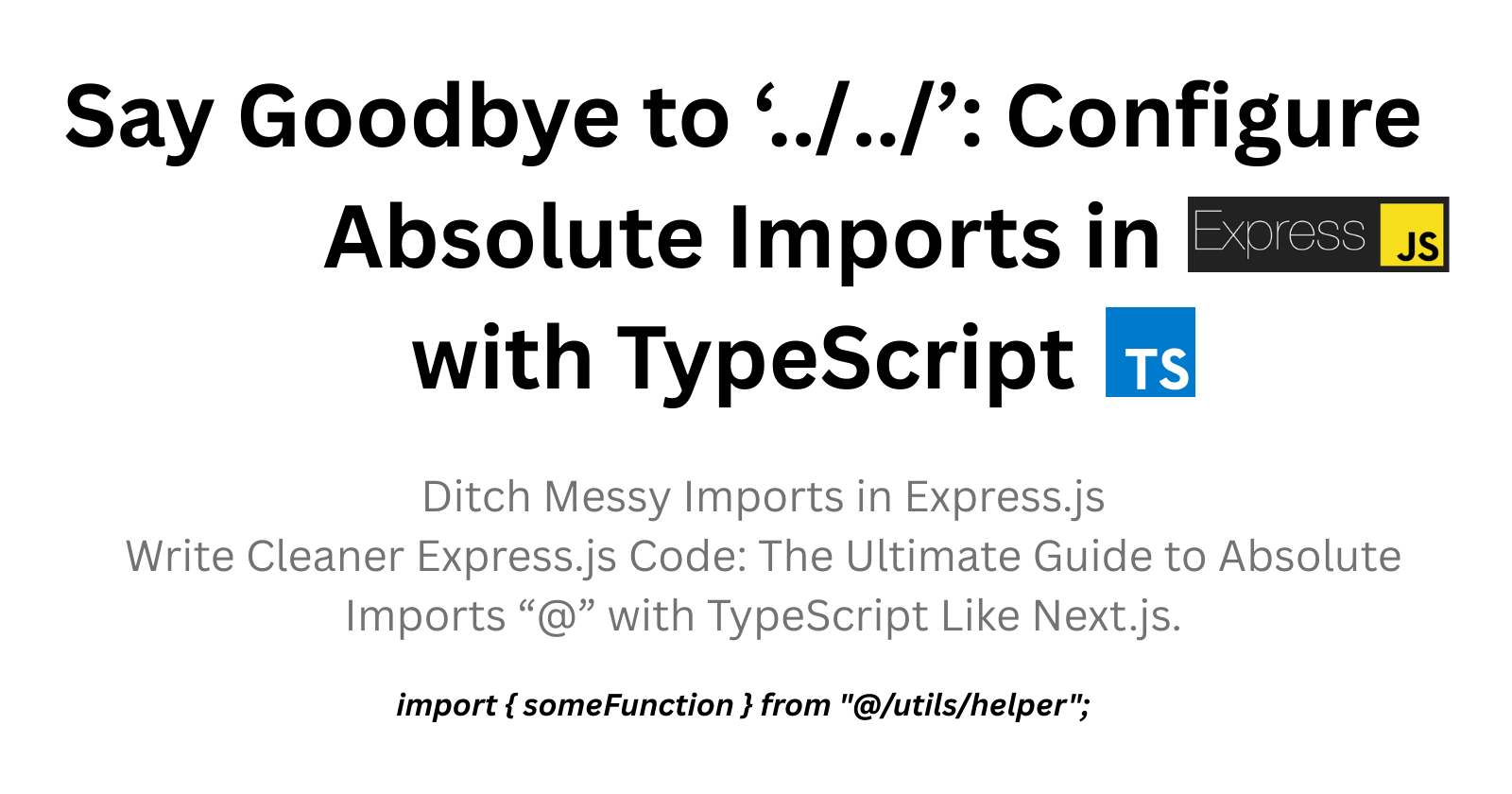
Introduction
When working on an Express.js project with TypeScript, managing file imports can become a headache. As projects grow, deep folder structures lead to confusing relative paths like:
import { someFunction } from "../../../../utils/helper";
This makes the code harder to read, navigate, and maintain. Next.js solves this issue with absolute imports, where you can write:
import { someFunction } from "@/utils/helper";
Wouldn’t it be great if we could do the same in an Express.js app? Let’s break down the problem and implement a simple solution step by step.
The Problem: Messy and Hard-to-Maintain Imports
The Typical Developer Experience
Imagine you're working on a backend system with this structure:
project-root/
│-- src/
│ ├── controllers/
│ │ ├── userController.ts
│ ├── models/
│ │ ├── userModel.ts
│ ├── utils/
│ │ ├── helper.ts
│ ├── server.ts
Now, in server.ts
, you need to import helper.ts
. Without absolute imports, you’d write:
import { someFunction } from "../utils/helper";
It’s manageable for now, but what happens when your project grows? Deeply nested folders make paths even worse:
import { someFunction } from "../../../../utils/helper";
This is hard to read and fragile—moving a file breaks all related imports.
How Next.js Solves This Problem
In Next.js, we use an alias like @/
to point to the src/
folder:
import { someFunction } from "@/utils/helper";
This keeps imports clean and prevents path-related issues. Let’s apply the same solution to an Express.js + TypeScript project.
The Solution: Configuring Absolute Imports
To achieve Next.js-style absolute imports in Express.js, we will:
Set up a TypeScript project (if not already done).
Configure TypeScript to recognize
@/
assrc/
.Adjust scripts and dependencies to support absolute imports.
Implement and verify the changes.
Implementing the Solution
Step 1: Setting Up a TypeScript Project
If you don’t have a TypeScript-based Express.js project, create one:
mkdir express-ts-app && cd express-ts-app
npm init -y
npm install express
npm install -D typescript @types/node @types/express
Initialize TypeScript:
npx tsc --init
This creates a tsconfig.json
file.
Step 2: Configuring tsconfig.json
Modify tsconfig.json
to define the @
alias:
{
"compilerOptions": {
"baseUrl": "./",
"paths": {
"@/*": ["src/*"]
},
"outDir": "./dist",
"moduleResolution": "node",
"module": "CommonJS",
"target": "ES6",
"esModuleInterop": true
},
"ts-node": {
"require": ["tsconfig-paths/register"]
},
"exclude": ["node_modules", "**/*.spec.ts"],
"include": ["src/**/*"]
}
Now, TypeScript understands that @/
refers to src/
.
Step 3: Using Absolute Imports in Code
Now you can update your imports from:
import { someFunction } from "../../utils/helper";
to:
import { someFunction } from "@/utils/helper";
Much cleaner and easier to manage!
Step 4: Updating Scripts for Compilation and Running
Modify the scripts
section of package.json
:
{
"scripts": {
"build": "rm -rf dist && tsc && tsc-alias",
"dev": "nodemon src/server.ts",
"start": "node dist/server.js"
}
}
Install nodemon
, tsconfig-paths
, and tsc-alias
:
npm install -D nodemon tsconfig-paths tsc-alias
Why These Changes?
build
: Cleans thedist/
folder, compiles TypeScript, and updates paths usingtsc-alias
.dev
: Runs the app in development mode withnodemon
.start
: Runs the compiled JavaScript code fromdist/
.
Step 5: Running the Application
Start the development server:
npm run dev
For production:
npm run build
npm start
If everything is set up correctly, your Express.js app should now support absolute imports! 🎉
Impact of the Solution
✅ Pros
Cleaner Imports: No more
../../../
headaches.Easier Refactoring: Moving files doesn’t break imports.
Improved Readability: Faster to understand where files come from.
Next.js Consistency: Works like Next.js, reducing context switching.
❌ Cons
Requires Configuration: Needs extra setup in
tsconfig.json
.Only for TypeScript: Pure JavaScript projects don’t benefit without additional tools.
Conclusion
Absolute imports significantly improve code clarity and maintainability in an Express.js + TypeScript project. By configuring TypeScript and setting up tsconfig-paths
, we can enjoy Next.js-style imports, making backend development smoother and more scalable.
Now, go ahead and clean up your imports—your future self will thank you! 🚀
Subscribe to my newsletter
Read articles from J.A. Shezan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

J.A. Shezan
J.A. Shezan
Shezan loves technology who is currently studying Computer Science and Engineering. He codes frontend & backend of a website. He also does penetration testing on web apps.