RabbitMQ: Dead Letter Exchanges (DLX)

Table of contents
- π· Default Behavior of RabbitMQ to Handle Failed Messages
- π§βπ» What is a Dead Letter Exchange (DLX)?
- π Flowchart: How Dead Letter Exchanges (DLX) Work
- π’ Why is DLX Important?
- π Implementing Dead Letter Exchanges (DLX) in RabbitMQ
- π§βπ» Consumer Code for Handling DLX Messages
- π§ͺ Key Takeaways
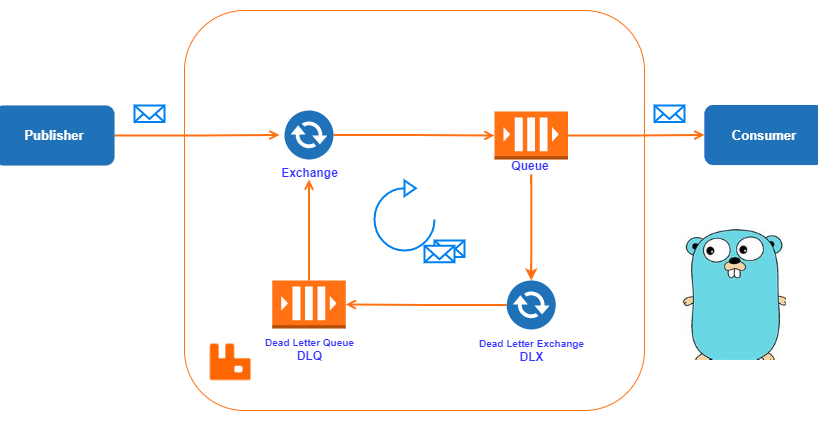
In this article, weβll explore the concept of Dead Letter Exchanges (DLX) in RabbitMQ. DLX is an essential feature for handling message failures or processing issues, allowing you to capture messages that cannot be delivered or processed as expected.
By the end of this article, you should understand:
What is a Dead Letter Exchange (DLX)?
Why is it important?
How to implement DLX in RabbitMQ.
A practical example to demonstrate the concept.
A flowchart to visualize how DLX works.
π· Default Behavior of RabbitMQ to Handle Failed Messages
By default, RabbitMQ does not have a built-in mechanism to handle failed messages. If a message cannot be delivered to a consumer or is rejected, it is simply discarded or lost. This behavior can lead to potential message loss, especially in cases of system failures or if there are consumer issues.
Default Behavior:
Message Rejection (NACK): If a consumer rejects a message (using
msg.Nack()
ormsg.Reject()
), RabbitMQ does not automatically requeue the message unless explicitly told to do so.Unroutable Messages: If a message cannot be routed to any queue (e.g., due to an incorrect routing key or a queue that no longer exists), RabbitMQ will drop the message by default.
Queue Overflow: If a queue is full (i.e., reaches its length or memory limit), RabbitMQ drops the excess messages.
Message Expiration: If a message expires (using TTL settings), RabbitMQ drops the expired message.
This default behavior can result in the loss of important messages, especially if RabbitMQ is unable to deliver or process a message. This is where mechanisms like Dead Letter Exchanges (DLX) come into play, providing a way to handle failed or undelivered messages.
π§βπ» What is a Dead Letter Exchange (DLX)?
A Dead Letter Exchange (DLX) is a special type of exchange in RabbitMQ where messages that cannot be delivered or processed are routed. These messages are often referred to as "dead letters" because they cannot be handled by the original destination queue.
Dead letters can arise for several reasons, such as:
Message Expiration: When a message expires (based on TTL settings).
Queue Length Limit: If a queue reaches its maximum length.
Message Rejection: If a consumer rejects the message without requeuing it.
Unroutable Messages: If the message cannot be routed to any queue due to routing issues.
When any of the above conditions occur, RabbitMQ sends the message to the DLX for further handling. This provides a way to monitor or log messages that couldn't be processed, and they can be retried or investigated later.
π Flowchart: How Dead Letter Exchanges (DLX) Work
+---------------------+
| Producer |
+---------------------+
|
| Publish Message
β
+--------------+
| Exchange |
+--------------+
|
| Route to Queue
β
+-----------------+
| Queue |
+-----------------+
|
| Consumer Consumes Message
β
+-----------------------+
| Message Processed |
| (ACK or NACK) |
+-----------------------+
|
(If message can't be processed)
|
+----------------------+
| Dead Letter Queue |
+----------------------+
|
| Further Handling / Retry
β
+-----------------+
| DLX Exchange |
+-----------------+
Producer publishes a message.
The message is routed through the Exchange to the Queue.
The Consumer processes the message.
If processing fails or the message canβt be delivered, it is routed to a Dead Letter Exchange (DLX).
The DLX can be further used to handle these dead-lettered messages, either by retrying, logging, or investigating issues.
π’ Why is DLX Important?
Dead Letter Exchanges are important for several reasons:
Message Handling: They provide a mechanism to handle messages that canβt be delivered or processed, preventing message loss.
Error Management: They help with managing failed messages (e.g., due to consumer errors or expired messages) by providing an isolated place for troubleshooting.
Retry Mechanism: Dead Letter Exchanges allow for retry mechanisms. You can move failed messages back to a normal queue after investigating the issues.
Queue Overflow Protection: They protect from queue overflow by rerouting undelivered or rejected messages to a designated exchange/queue.
Audit and Monitoring: DLXs allow you to inspect why messages were dead-lettered and help with system monitoring.
π Implementing Dead Letter Exchanges (DLX) in RabbitMQ
To configure Dead Letter Exchanges, you need to define two key things:
The DLX exchange to send the dead-lettered messages.
The DLX routing key to control how messages are routed.
Hereβs a step-by-step guide on how to implement DLX in RabbitMQ using Golang.
Step 1: Define the Queue with DLX
You need to configure a queue that will send dead-lettered messages to a specified exchange. Here's an example of how to set this up:
package main
import (
"log"
"github.com/streadway/amqp"
)
func main() {
conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/")
if err != nil {
log.Fatal(err)
}
defer conn.Close()
ch, err := conn.Channel()
if err != nil {
log.Fatal(err)
}
defer ch.Close()
// Declare a normal queue that will use DLX
normalQueue, err := ch.QueueDeclare(
"normal_queue", // Queue name
false, // Durable
false, // Delete when unused
false, // Exclusive
false, // No-wait
amqp.Table{
"x-dead-letter-exchange": amqp.String("dlx_exchange"), // Specify DLX exchange
},
)
if err != nil {
log.Fatal(err)
}
// Declare a DLX queue to handle dead-lettered messages
dlxQueue, err := ch.QueueDeclare(
"dlx_queue", // DLX Queue name
false, // Durable
false, // Delete when unused
false, // Exclusive
false, // No-wait
nil, // No arguments
)
if err != nil {
log.Fatal(err)
}
// Bind the DLX queue to a DLX exchange
ch.QueueBind(
dlxQueue.Name, // Queue name
"", // Routing key
"dlx_exchange", // DLX exchange
false,
nil,
)
log.Println("Queues declared successfully")
}
π Explanation of the Code
Normal Queue with DLX:
- The
normal_queue
is set to use the DLX feature. This is done by setting the argumentx-dead-letter-exchange
to"dlx_exchange"
. Any undeliverable or rejected messages from this queue will be sent to thedlx_exchange
.
- The
DLX Queue:
- The
dlx_queue
is created to handle messages that are dead-lettered from thenormal_queue
. TheQueueBind
method binds the DLX queue to thedlx_exchange
where dead-lettered messages will be routed.
- The
Step 2: Configure the Producer
You can now configure a producer to send messages to the normal_queue
. If the message cannot be processed or delivered, it will be sent to the dlx_queue
.
package main
import (
"log"
"github.com/streadway/amqp"
)
func main() {
conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/")
if err != nil {
log.Fatal(err)
}
defer conn.Close()
ch, err := conn.Channel()
if err != nil {
log.Fatal(err)
}
defer ch.Close()
// Declare the normal queue
normalQueue, err := ch.QueueDeclare(
"normal_queue",
false,
false,
false,
false,
nil,
)
if err != nil {
log.Fatal(err)
}
// Send a message to the normal queue
err = ch.Publish(
"",
normalQueue.Name,
false,
false,
amqp.Publishing{
ContentType: "text/plain",
Body: []byte("This is a message"),
},
)
if err != nil {
log.Fatal(err)
}
log.Println(" [x] Sent message to normal queue")
}
π§βπ» Consumer Code for Handling DLX Messages
Now, let's configure a consumer to consume messages from the dlx_queue
(dead-letter queue).
package main
import (
"log"
"github.com/streadway/amqp"
)
func main() {
conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/")
if err != nil {
log.Fatal(err)
}
defer conn.Close()
ch, err := conn.Channel()
if err != nil {
log.Fatal(err)
}
defer ch.Close()
// Declare the DLX queue
dlxQueue, err := ch.QueueDeclare(
"dlx_queue",
false,
false,
false,
false,
nil,
)
if err != nil {
log.Fatal(err)
}
// Consume messages from the DLX queue
msgs, err := ch.Consume(
dlxQueue.Name, // Queue name
"", // Consumer name
true, // Auto-ack
false, // Exclusive
false, // No local
false, // No-wait
nil, // Arguments
)
if err != nil {
log.Fatal(err)
}
// Handle dead-lettered messages
for msg := range msgs {
log.Printf("Received Dead Letter Message: %s", msg.Body)
}
}
π§ͺ Key Takeaways
Dead Letter Exchanges (DLX) help manage and troubleshoot undeliverable or failed messages.
By configuring DLX, you can route messages to a secondary queue for further investigation, logging, or retrying.
RabbitMQ uses x-dead-letter-exchange argument to configure the DLX behavior for a queue.
DLX can be extremely helpful for building reliable and fault-tolerant messaging systems.
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
