Python Type Hints
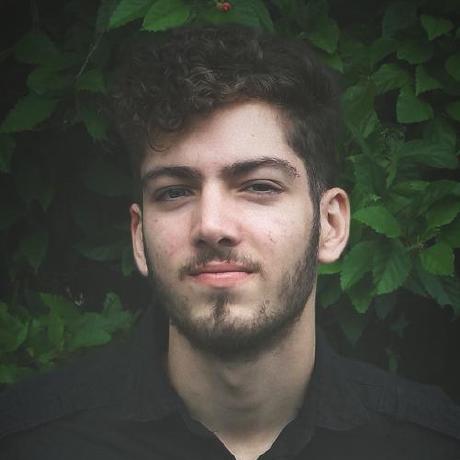
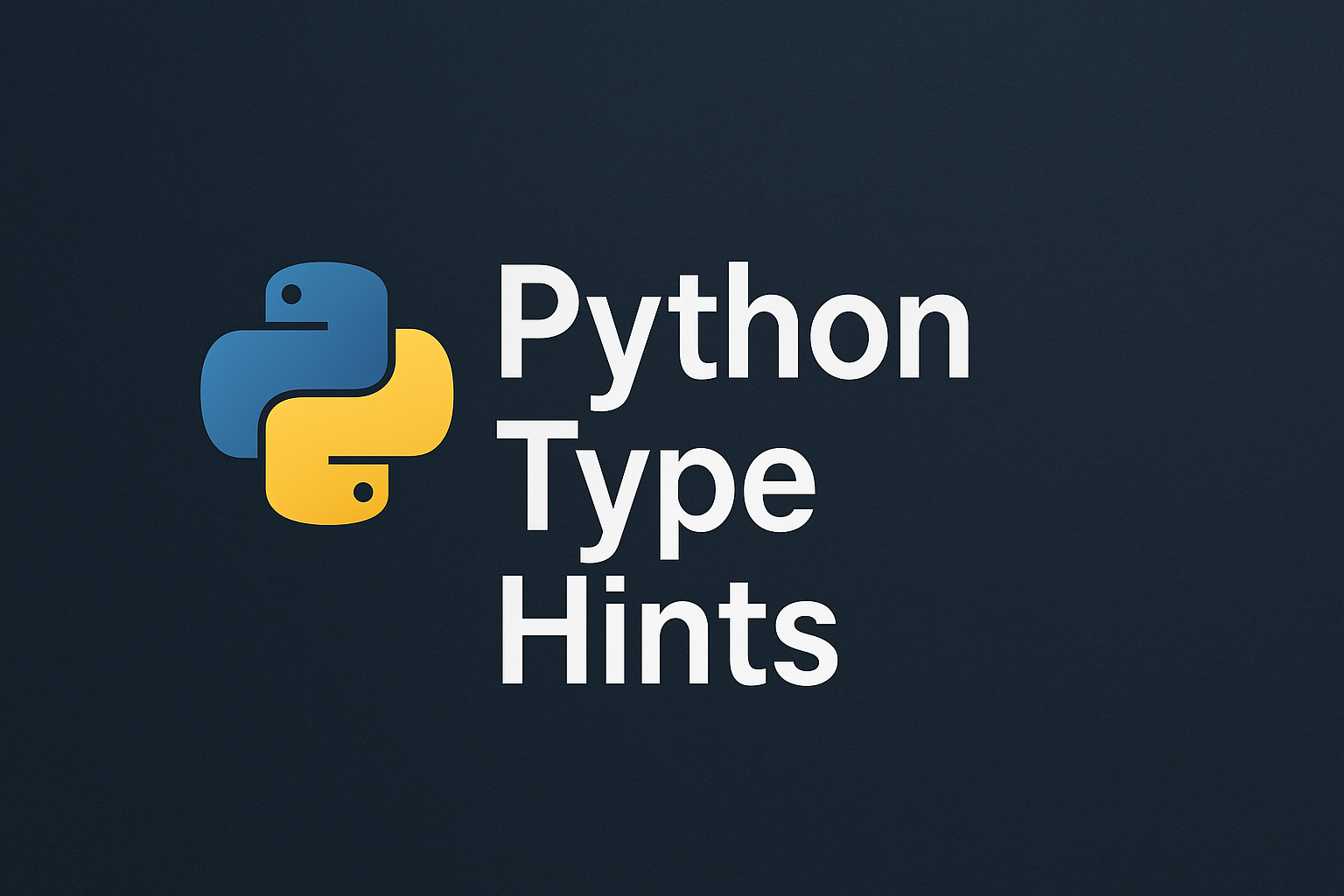
Unlike C, Python is a dynamically typed programming language, which means you do not need to specify variable types in your code. The type of a variable is determined at runtime and can change while the program is running.
However, this flexibility can sometimes make code and logic less obvious. To address this, Python introduced Type Hints in version 3.5. Type hints allow us to specify the expected data type of variables, making the code more readable and understandable.
It’s important to note that I wrote "expected data type" rather than saying it's mandatory—because type hints are optional, as we’ll see later.
Example
def calc_triangle_area(base, height):
return 0.5 * base * height
This simple function calculates the area of a triangle given a base and height. If we ask people to provide values, some might say 1, 2, 9, or 10, while others might say 3.5 or 10.5. All these answers are correct since triangles can have a wide range of base and height values.
However, we cannot accept values like strings (e.g., "3"
and "8.5"
), as they are not valid numerical inputs.
This is where Type Hints come in handy.
def calc_triangle_area(base: float, height: float) -> Float:
return 0.5 * base * height
Even in a simple function like this, type hints improve readability by clearly indicating what types the variables (base
and height
) should have. Additionally, the -> float
syntax specifies the expected return type.
One of the best things about Type Hints is that modern code editors (IDEs) will show expected types as you write the code. This makes debugging easier, improves logic validation, and helps ensure data quality across your project.
Subscribe to my newsletter
Read articles from Luiz Gustavo Erthal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
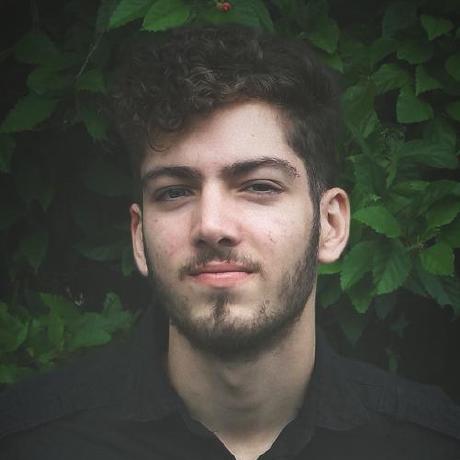