Learning Shopify Liquid (part 1)

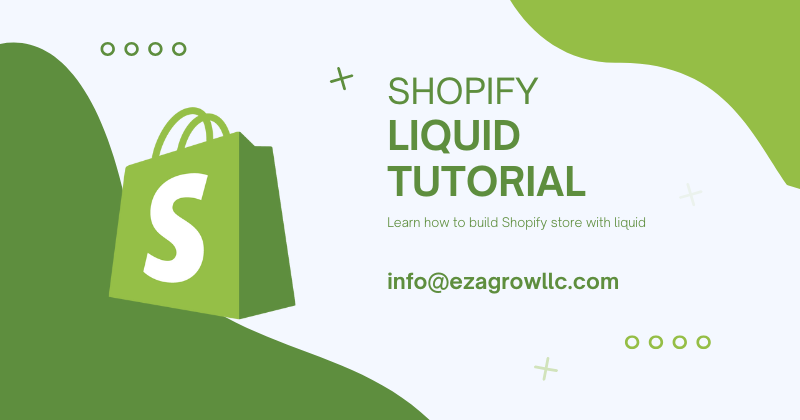
Welcome to our comprehensive series, where we will dive deep into the world of Liquid, the versatile language that plays a crucial role in building powerful and dynamic online stores within the Shopify ecosystem. This series aims to provide you with a thorough understanding of how Liquid works and how you can leverage it to enhance your Shopify store.
What is Liquid?
Liquid is a robust templating language specifically designed for creating dynamic and interactive websites. But what exactly is a templating language, you might ask? Allow me to break it down for you. A templating language is essentially a tool that enables developers to craft web pages or documents using templates with placeholders. These placeholders are then populated with actual data, allowing for the seamless integration of dynamic content into your HTML structure. This means that the content displayed on your website can be updated automatically based on the data retrieved from your server, providing a personalized and up-to-date experience for your users.
To help you grasp the concept more clearly, let's explore some simple examples. These examples will illustrate how Liquid can be used to dynamically fetch and display data, making your Shopify store more engaging and efficient. Throughout this series, we will cover various aspects of Liquid, from basic syntax to advanced techniques, ensuring you have the knowledge to fully utilize this powerful tool in your development projects.
HTML example:
<title>My website</>
In the example above, we are using a basic HTML title tag, which is a fundamental part of web page creation. This tag is used to set the title of the web page that appears in the browser's title bar or tab. However, in this case, the title is hardcoded, meaning it is static and unchanging. As a result, every page that uses this code will display the same title, "My website," regardless of the content or context of the page. This approach is straightforward but lacks flexibility because it doesn't allow for dynamic content. In contrast, using a templating language like Liquid, you can replace static text with dynamic placeholders. These placeholders can be filled with different data for each page, allowing for a more personalized and adaptable user experience. This is particularly useful in scenarios where you want to display unique titles for different pages, such as product names on an e-commerce site or article titles on a blog.
Liquid example:
<title>
{{ page_title }}
</title>
In the Liquid example, you can observe that we used double curly braces “{{ }}” to insert dynamic data into our HTML. Specifically, we used the variable “page_title,” which is a built-in variable provided by Shopify, to dynamically display the title on each page. This means that if I have 20 different pages, each with its own unique title, the titles will be displayed automatically and dynamically without requiring me to manually set each one. This is the advantage of using a templating language like Liquid. It allows for a more efficient and flexible approach to web development by enabling the automatic generation of content based on variables. This not only saves time but also reduces the potential for errors that can occur when manually updating each page. Additionally, it enhances the user experience by ensuring that each page is accurately and consistently labeled, which is particularly beneficial for websites with a large number of pages, such as e-commerce sites or blogs.
Before we begin, it's essential to set up a Shopify Partner account. This account will allow you to create a Shopify development store, which is necessary for testing and development purposes. Once you have your Shopify Partner account, the next step is to create a development store. This store acts as a sandbox environment where you can experiment with different themes and functionalities without affecting a live store.
After setting up your development store, you need to clone it onto your local computer. Cloning the store locally allows you to make changes and test them in a controlled environment before deploying them to your development store. This process involves using tools like Git to manage your theme files and ensure that any changes you make are tracked and version-controlled.
Before proceeding with these steps, I recommend reading this comprehensive article. It provides detailed instructions on how to clone a theme, such as the Dawn theme, or any other theme you choose, onto your local machine. This guide will walk you through the necessary steps, ensuring that you have a smooth setup process. By following these instructions, you'll be well-prepared to start customizing your Shopify store and experimenting with different design and functionality options.
The comment
When we begin learning a new coding, programming, or templating language, one of the initial aspects we typically concentrate on is understanding how to write comments. Comments play a vital role in any codebase because they serve multiple purposes. Firstly, they provide explanations about the purpose and functionality of the code, which is incredibly helpful for anyone who might read or work with the code in the future. This includes team members, collaborators, or even your future self when you return to the code after some time.
Moreover, comments are essential for debugging purposes. By temporarily commenting out sections of code, you can isolate and identify issues without permanently altering the codebase. This makes it easier to test different parts of the code and understand how changes affect the overall functionality.
Additionally, comments are crucial for documenting your code. They ensure that the code is easier to maintain and update over time. As projects grow in complexity, well-documented code helps developers quickly grasp the logic and structure, facilitating smoother transitions during updates or when new features are added.
When working with Liquid code in Shopify, there are two primary methods for adding comments to your code. Understanding these methods is essential for maintaining clarity and organization within your codebase.
The first method involves using the {% comment %}
and {% endcomment %}
tags. This approach allows you to create multi-line comments, which are particularly useful when you need to provide detailed explanations or notes about sections of your code. By enclosing your comments within these tags, you can ensure that they are ignored during the code execution process, allowing you to document your code without affecting its functionality.
The second method is to use the {# #}
syntax for single-line comments. This is a more concise way to add brief notes or reminders directly within your code. Single-line comments are ideal for quick annotations or for marking specific lines that require attention or further development.
Both methods are integral to writing clear and maintainable Liquid code in Shopify. By effectively using comments, you can enhance the readability of your code, making it easier for yourself and others to understand and work with it in the future.
The Html comment
You can also use HTML comment syntax to comment on your code. The main difference is that when you use Liquid tags, your comments won't be visible when someone inspects your code. However, with HTML comments, even though the code isn't executed, it will still be visible during inspection. We recommend always using Liquid comment tags, but ultimately, it's up to you.
Creating logic {% %} and displaying data {{ }} in liquid.
Before we dive into the details, it's important to understand how to write logic and display data in Liquid. This might seem a bit confusing at first, but stick with me, and it will become clearer.
Let's start with logic. Suppose you want to create a variable, use an if statement to make decisions, or loop through an array or a collection of objects. These actions are all part of what we call logic in programming. In Liquid, you need to wrap these logical operations within specific syntax tags: {% %}
. These tags tell Liquid that you are performing a logical operation, allowing you to control the flow of your code effectively.
Now, when it comes to displaying data, Liquid uses a different approach. If you want to output or render data on the page, you should use double curly braces like this: {{ }}
. This syntax is used to display the value of a variable or the result of an expression directly in your HTML. For example, if you have a variable that holds a product name, you would use {{ product_name }}
to show it on the page.
Whitespace control
When you create logic tags or display data using Liquid, it automatically adds spaces around the elements. While these spaces do not directly impact your user interface design, they become noticeable when you inspect your code. You will see extra spaces on both the left and right sides of your elements. To remove these spaces from both sides of your tags, you can use hyphens. Here's how you can do it: {%- Here goes your logic -%} or {{- Here is displayed data -}}. Using these formats will strip the whitespace from both the left and right sides.
If you want to remove whitespace only from the left or the right side, you can do that too.
Here are some examples:
to strip whitespace from the left side, you would write {%- your logic goes here %} and {{- your displayed data }}.
To strip whitespace from the right side, you would use {% your logic goes here -%} and {{ your displayed data -}}.
That concludes the first part of our discussion. In the next section, we will delve into the process of creating variables in more detail. We will explore how to define these variables and effectively display their values within your HTML using Liquid syntax. Additionally, we will cover various techniques and best practices for managing and manipulating these variables to enhance your web development projects. Stay tuned for more insights and practical examples that will expand your understanding and skills.
Subscribe to my newsletter
Read articles from Kamba Tukebele directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kamba Tukebele
Kamba Tukebele
I'm a Full-stack developer specializing in Laravel, React.js, and Next.js, with a strong focus on eCommerce development. My expertise lies in building Shopify stores and crafting headless commerce solutions using Shopify Hydrogen. I love creating scalable, high-performance online stores, whether through custom Shopify themes, apps, or headless storefronts. My goal is to help businesses leverage modern web technologies for a seamless and engaging shopping experience. Follow along as I share insights, tutorials, and best practices on Shopify development, Liquid, Hydrogen, and more!