Week 4 – Simple Math & String Magic

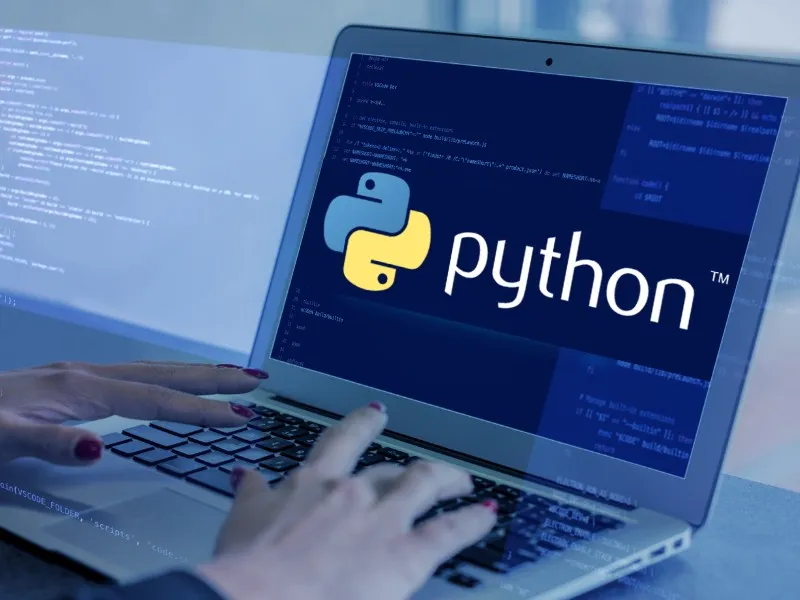
Hello Future Coders!
Welcome to Week 4 of our Python Adventures! This week, we’re going to have fun with two cool topics: Simple Math (Arithmetic) and Mixing Words (String Concatenation). By the end of today’s lesson, you'll be able to do basic math with Python and join words together to create awesome sentences!
What You'll Learn
Arithmetic: Learn how to add, subtract, multiply, and divide numbers.
String Concatenation: Discover how to join words and sentences using the
+
operator.Make your programs interactive and fun by combining math and text!
1. Simple Arithmetic in Python
What is Arithmetic?
Arithmetic is just another word for math. In Python, we use special symbols (operators) to do math with numbers:
Addition (
+
): Adds numbers together.Subtraction (
-
): Takes one number away from another.Multiplication (
*
): Multiplies numbers.Division (
/
): Divides one number by another.
Examples
# Addition
print(2 + 3) # This prints: 5
# Subtraction
print(10 - 4) # This prints: 6
# Multiplication
print(4 * 5) # This prints: 20
# Division
print(20 / 4) # This prints: 5.0
Kid Tip:
Think of addition like stacking your toy blocks to make a taller tower, and subtraction like taking some blocks away!
2. String Concatenation in Python
What is String Concatenation?
String concatenation means joining words together. In Python, we use the +
operator to stick words together.
Example
greeting = "Hello"
name = "Emma"
print(greeting + ", " + name + "!")
Output:
Hello, Emma!
Kid Tip:
Imagine you have two pieces of a sticker: one says "Hello" and the other says "Emma." When you stick them together, you make a cool greeting sticker!
3. Interactive Coding Challenges
Challenge 1: Math Helper
Write a simple program that asks for two numbers and then prints their sum, difference, and product.
Example Code:
num1 = 8
num2 = 5
print("Sum:", num1 + num2)
print("Difference:", num1 - num2)
print("Product:", num1 * num2)
Try changing the numbers to see what happens!
Challenge 2: The Talking Calculator
Make a program that asks for your favorite number, adds 10 to it, and prints a fun message.
Example Code:
favorite_number = int(input("What is your favorite number? "))
print("Your favorite number plus 10 is:", favorite_number + 10)
Remember: We use int()
to turn your text into a number so Python can do the math!
Challenge 3: Mix and Match Words
Create a program that joins different pieces of text to make a sentence.
Example Code:
part1 = "Python is"
part2 = "awesome!"
print(part1 + " " + part2)
Now, try making your own sentence with your favorite words!
4. Quiz Time!
Let's check what you've learned:
What does the
+
operator do when used with numbers?A) Joins them together
B) Adds them together
C) Divides them
What is string concatenation?
A) Adding numbers
B) Joining words together
C) Multiplying letters
Which code correctly converts a user’s input into a number for math?
A)
favorite = input("Enter your favorite number: ")
B)
favorite = int(input("Enter your favorite number: "))
C)
print("Enter your favorite number: ")
What will this code do?
name = input("What is your name? ") print(f"Hello {name}, nice to meet you!")
A) It will print "Hello name, nice to meet you!"
B) It will greet you using your name.
C) It will show an error.
5. Recap & Reflection
Key Takeaways:
Arithmetic:
- Python helps you do math using symbols like
+
,-
,*
, and/
.
- Python helps you do math using symbols like
String Concatenation:
- The
+
operator lets you join words together.
- The
Interactive Coding:
- By combining
input()
andprint()
, you can create fun programs that both do math and mix words to make cool messages.
- By combining
Discussion Prompt:
Imagine you’re creating a magic calculator. What fun message would you print when you add two numbers together? Write your idea down and share it with the class next time!
What’s Next?
In our next lesson, we’ll dive into Conditionals (if/else). Get ready to learn how to make decisions in your programs—just like a smart robot that knows what to do in different situations!
Happy Coding, Future Coders!
Keep experimenting, have fun, and see you next week for more Python Adventures!
Subscribe to my newsletter
Read articles from Ridwan Ibidunni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ridwan Ibidunni
Ridwan Ibidunni
I'm a mathematician that loves its applications in all spheres of life, especially in the field of machine learning. I write Java, Python and Android applications