Next.js Authentication Best Practices in 2025
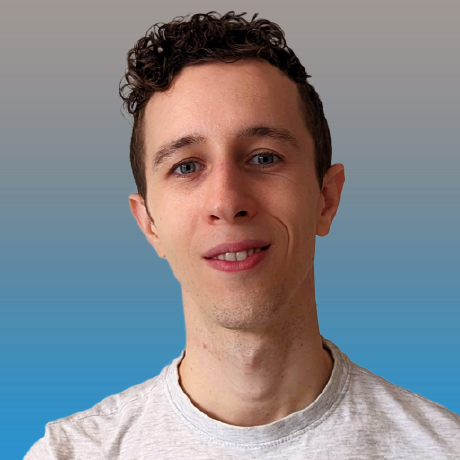
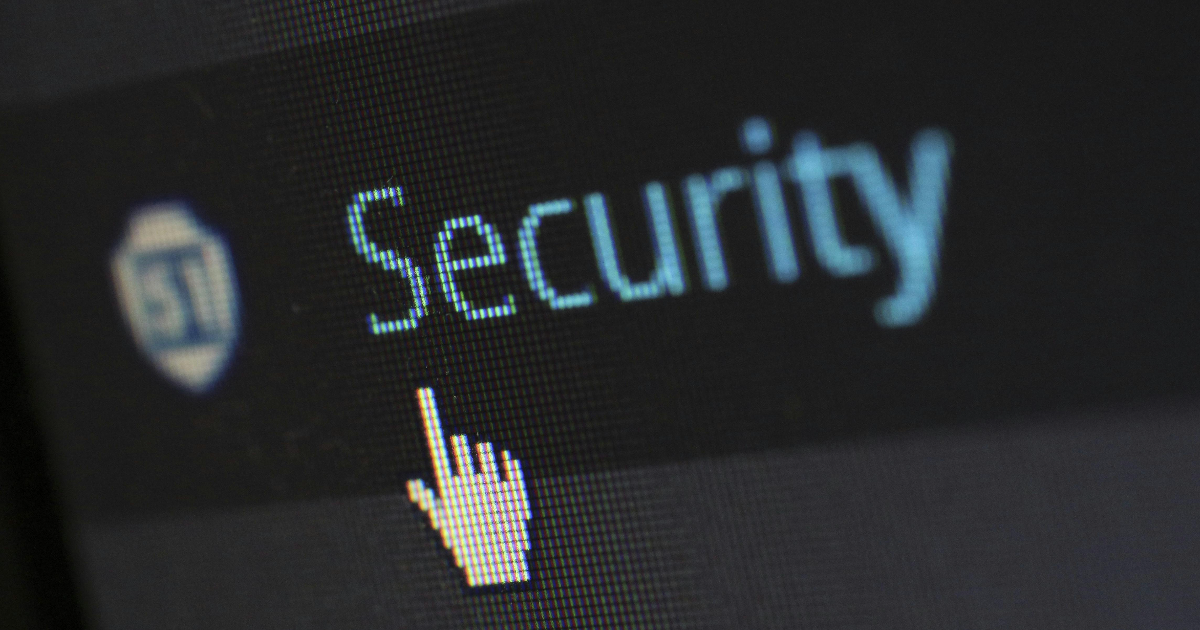
Note: This article updates previous authentication guide, which contained outdated recommendations. Next.js has since changed its authentication guidance, particularly in light of the CVE-2025-29927 security vulnerability.
This articles containes a simplified version of the official Next.js authentication guide.
Why Next.js Authentication Guidance Has Changed
The Next.js team has significantly updated their authentication recommendations. The most important change: middleware is no longer considered safe for authentication. Instead, the new approach focuses on:
- Using Data Access Layers (DAL)
- Centralizing authentication logic
- Keeping auth checks close to data access
- Implementing proper role-based access in Server Components
Let's explore these updated best practices in detail.
Data Access Layers: The New Best Practice
A Data Access Layer centralizes all data access logic, including authentication checks. This approach provides:
- Consistent security across your application
- Protection against unauthorized data access
- Clear separation between authorization and data fetching
Here's a simple example of a DAL implementation:
// app/lib/dal.ts
import { cache } from 'react'
import { cookies } from 'next/headers'
import { redirect } from 'next/navigation'
export const verifySession = cache(async () => {
const cookieStore = cookies()
const sessionToken = cookieStore.get('session-token')?.value
if (!sessionToken) {
return null
}
try {
// Verify token with your auth provider
const session = await validateToken(sessionToken)
return session
} catch (error) {
console.error('Invalid session')
return null
}
})
export const getUser = cache(async () => {
const session = await verifySession()
if (!session) {
return null
}
try {
// Get user data using the session
const data = await db.query.users.findMany({
where: eq(users.id, session.userId),
columns: {
id: true,
name: true,
email: true,
},
})
return data[0]
} catch (error) {
console.error('Failed to fetch user')
return null
}
})
The Problem with Middleware for Authentication
Middleware in Next.js executes before the application renders. While this appears ideal for authentication, there are significant limitations:
- Static Routes Vulnerability: Middleware doesn't completely protect statically generated routes, as the content is already built
- Limited Context: Middleware has limited access to the application's full context
- Complexities with Static Generation: Auth logic in middleware can conflict with Next.js's static optimization
Proximity Principle: Auth Checks Close to Data
The proximity principle is now more important than ever. Keep authentication checks as close as possible to where sensitive data is accessed:
// Direct data access with auth check
async function fetchSensitiveUserData(userId: string) {
const session = await verifySession()
if (!session || session.user.id !== userId) {
throw new Error('Unauthorized')
}
// Fetch and return data only after verifying
return await db.users.findUnique({ where: { id: userId } })
}
Server Components and Role-Based Authentication
Server Components provide a powerful way to implement role-based access control:
// app/admin/page.tsx
import { verifySession } from '@/app/lib/dal'
import { redirect } from 'next/navigation'
export default async function AdminDashboard() {
const session = await verifySession()
if (!session) {
redirect('/login')
}
if (session.user.role !== 'admin') {
// User is logged in but not an admin
redirect('/dashboard')
}
return (
<div>
<h1>Admin Dashboard</h1>
{/* Admin-only content */}
</div>
)
}
Authentication in Layouts: Proceed with Caution
Due to partial rendering in Next.js, authentication in layouts requires special care:
- Layouts don't re-render on navigation within their subtree
- Auth checks may not run on every route change
Instead of relying on layout-level checks, perform authentication checks:
- In your Data Access Layer
- In page components
- Directly in data fetching functions
Securing Data Access and Mutations
Server Actions and data transfer operations need special protection as they're entry points to your application's data. Implement these practices for robust security:
- Always authenticate in Server Actions: Server Actions can be called directly from clients, so validate sessions before performing operations
- Use DTOs to control data exposure: Never return complete data objects to clients - expose only what's necessary
- Add role-based permissions: Check not just authentication but authorization for specific operations
Here's how to secure a Server Action:
// app/lib/actions.ts
'use server'
import { verifySession } from '@/app/lib/dal'
export async function updateUserProfile(formData: FormData) {
// Auth check before any operation
const session = await verifySession()
if (!session) throw new Error('Unauthorized')
// Get only the fields you need
const userId = session.user.id
const name = formData.get('name')
// Validate input data
if (!name || typeof name !== 'string') {
throw new Error('Invalid input')
}
// Update only for authorized users
return await db.users.update({
where: { id: userId },
data: { name },
})
}
For data retrieval, use DTOs to limit exposure of sensitive data:
// app/lib/dto.ts
import 'server-only'
import { getUser } from '@/app/lib/dal'
export async function getUserProfileDTO(userId: string) {
// Authentication check
const currentUser = await getUser()
if (!currentUser) return null
// Authorization check
const isAdmin = currentUser.role === 'admin'
const isSelf = currentUser.id === userId
if (!isAdmin && !isSelf) return null
// Get the user data
const userProfile = await db.users.findUnique({
where: { id: userId },
select: { id: true, name: true, email: true, role: true },
})
// Return only appropriate fields
return {
id: userProfile.id,
name: userProfile.name,
email: isAdmin || isSelf ? userProfile.email : null,
role: isAdmin ? userProfile.role : null,
}
}
By consistently implementing these patterns at every data entry and exit point, you maintain a secure boundary around your application's data.
Context Providers: Client-Side Limitations
React Context providers work for auth state due to Next.js's component interleaving architecture. However, there's an important limitation: React Context is not supported in Server Components and only works with Client Components.
When using a Context provider for authentication:
// app/providers.tsx
'use client'
import { createContext, useContext, useState, useEffect } from 'react'
const AuthContext = createContext(null)
export function AuthProvider({ children }) {
const [user, setUser] = useState(null)
const [loading, setLoading] = useState(true)
useEffect(() => {
// Fetch user on client
async function loadUserFromAPI() {
try {
const res = await fetch('/api/user')
const userData = await res.json()
setUser(userData)
} catch (error) {
setUser(null)
} finally {
setLoading(false)
}
}
loadUserFromAPI()
}, [])
return <AuthContext.Provider value={{ user, loading }}>{children}</AuthContext.Provider>
}
export const useAuth = () => useContext(AuthContext)
This approach works with Client Components that need authentication state:
// app/components/profile.tsx
'use client'
import { useAuth } from '../providers'
export function Profile() {
const { user, loading } = useAuth()
if (loading) return <div>Loading...</div>
if (!user) return <div>Please log in</div>
return <div>Welcome, {user.name}!</div>
}
However, any Server Components inside your component tree will be rendered on the server first, before the client-side context is available. This means:
- Server Components cannot access context values
- They cannot conditionally render based on authentication state from context
- You'll need to use your DAL for any auth checks in Server Components
This is why a dual approach is recommended:
- Use DAL for Server Components and data access
- Use Context for Client Components that need real-time auth state
Note that sharing sensitive session information between server and client components requires careful handling to prevent security issues.
Security Best Practices Summary
Protect your Next.js app at multiple layers for maximum security:
- Data layer: Add auth checks directly in your Data Access Layer functions
- Route level: Check authentication in page components
- UI elements: Hide sensitive components when users aren't authenticated
- Server actions: Verify authentication in all mutation functions
For best results:
- Replace middleware with a Data Access Layer for auth checks
- Put auth checks as close as possible to where data is accessed
- Be careful with layout-level authentication
- Add proper auth verification in Server Components and Actions
- Use Data Transfer Objects to control exactly what data gets exposed
This multi-layered approach creates apps that stay secure and easy to maintain without adding unnecessary complexity.
References
Subscribe to my newsletter
Read articles from Francisco Moretti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
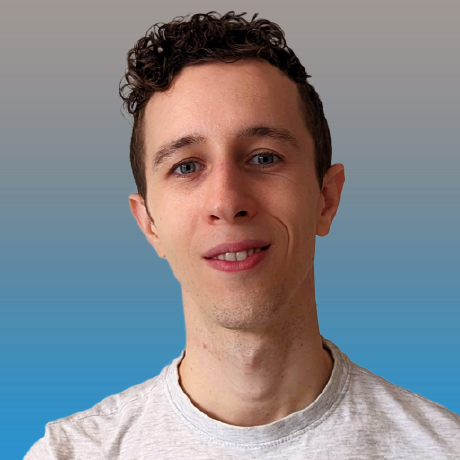
Francisco Moretti
Francisco Moretti
Full-Stack Engineer specializing in Web Development and AI.