Maven and Gradle: Understanding Their Purpose and Differences
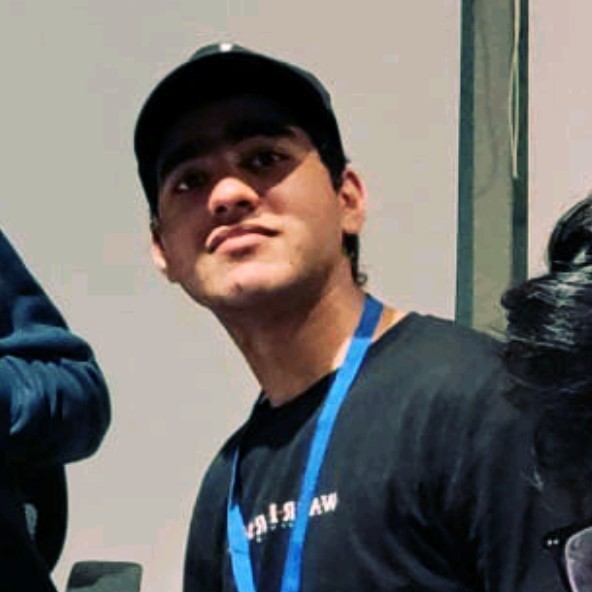
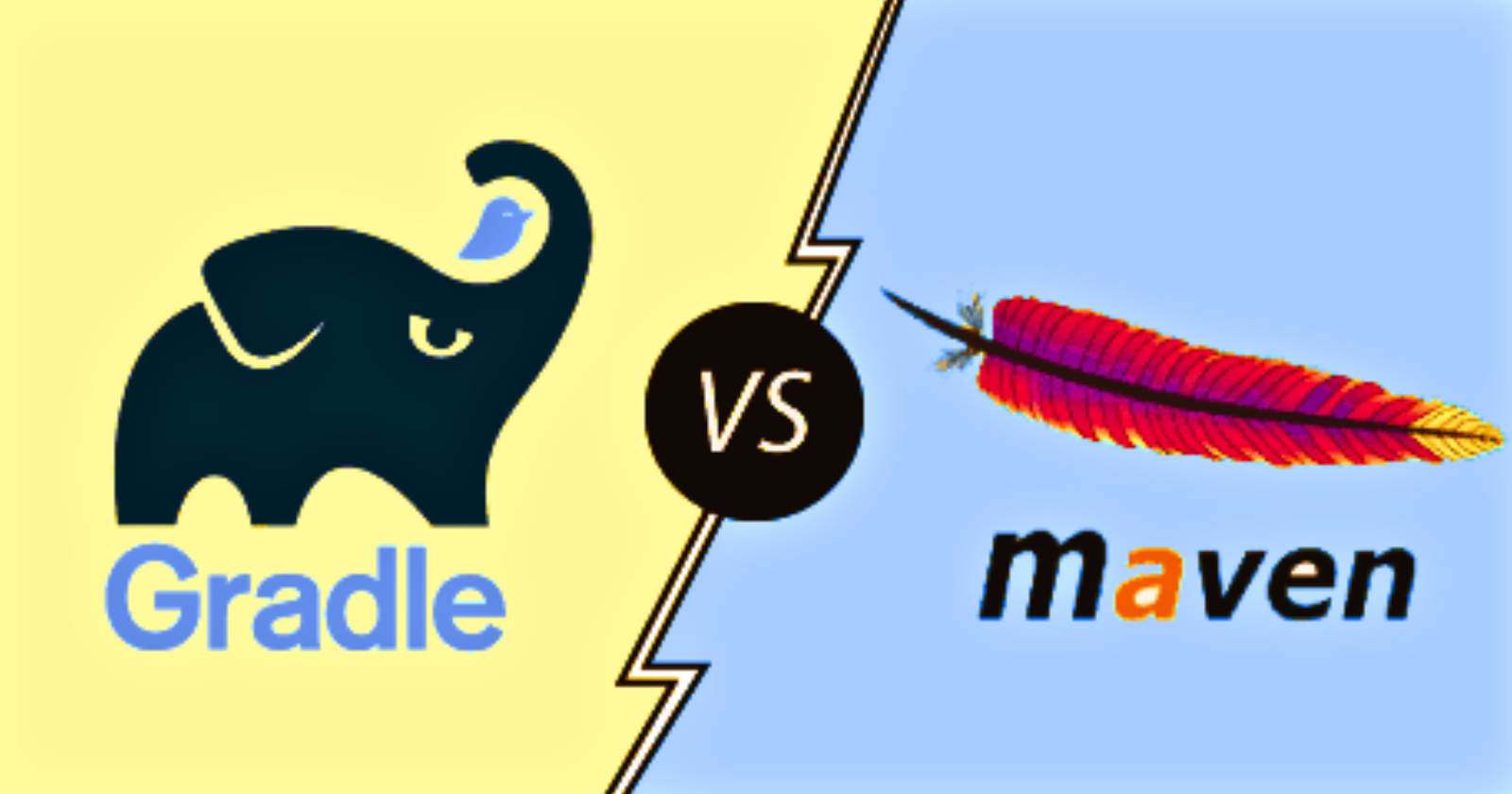
Maven and Gradle are build automation tools primarily used in Java-based projects. They help manage dependencies, compile source code, package applications, and automate deployment processes.
1. Why Were Maven and Gradle Developed?
The Need for Build Tools
Before Maven and Gradle, Java projects were built using Ant (Apache Ant) or even manual scripts like Makefile
. These approaches had problems:
Complex Build Scripts: Developers had to write detailed build scripts specifying every step.
Dependency Management Issues: Adding external libraries was manual, requiring developers to download
.jar
files and configure them properly.Inconsistent Builds: Without standardized tools, teams used different methods, leading to conflicts and errors.
To address these issues, Maven was introduced first, followed by Gradle as an alternative to improve performance and flexibility.
2. What is Maven?
Maven (released in 2004) is a dependency management and build tool developed by the Apache Software Foundation.
How Maven Solves the Problem
Introduced a standardized project structure (using a
pom.xml
file).Automated dependency management using Maven Central Repository.
Simplified build processes with predefined goals (e.g.,
mvn compile
,mvn package
).
Example Maven Usage
Install Maven.
Create a
pom.xml
file:<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> <version>3.2.1</version> </dependency> </dependencies> </project>
Run build command:
mvn clean package
3. What is Gradle?
Gradle (released in 2010) is a more flexible and efficient alternative to Maven.
How Gradle Solves the Problem
Uses Groovy/Kotlin DSL instead of XML for configuration (less verbose).
Faster build times due to incremental builds and caching.
More flexibility and better support for multi-project builds.
Example Gradle Usage
Install Gradle.
Create a
build.gradle
file:/*gradle*/ plugins { id 'java' id 'org.springframework.boot' version '3.2.1' } dependencies { implementation 'org.springframework.boot:spring-boot-starter' }
Run build command:
gradle build
4. Difference Between Maven and Gradle
Feature | Maven | Gradle |
Configuration | Uses XML (pom.xml ) | Uses Groovy/Kotlin (build.gradle ) |
Performance | Slower (full builds) | Faster (incremental builds, caching) |
Flexibility | Predefined lifecycle | More customizable |
Readability | Verbose XML | Concise Groovy/Kotlin |
Learning Curve | Easier for beginners | Requires learning Groovy/Kotlin |
5. Potential of Maven and Gradle
Use Case | Preferred Tool |
Standard Java applications | Maven |
Large, modular projects | Gradle |
Faster builds (CI/CD pipelines) | Gradle |
Legacy projects | Maven (widely adopted) |
6. How Can You Use Maven or Gradle?
Installing
Install Java JDK.
Install Maven (
sudo apt install maven
on Linux,brew install maven
on macOS).Install Gradle (
sdk install gradle
using SDKMAN or manually).
Creating a Project
Use Maven:
mvn archetype:generate
Use Gradle:
gradle init
Adding Dependencies
In
pom.xml
for MavenIn
build.gradle
for Gradle
Building & Running
Maven:
mvn package
Gradle:
gradle build
Conclusion
Maven is simple, reliable, and widely used.
Gradle is more powerful and optimized for speed.
If you're using Spring Boot, both tools work well, but Gradle is becoming more popular.
thank you.
Subscribe to my newsletter
Read articles from Eshaan Walia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
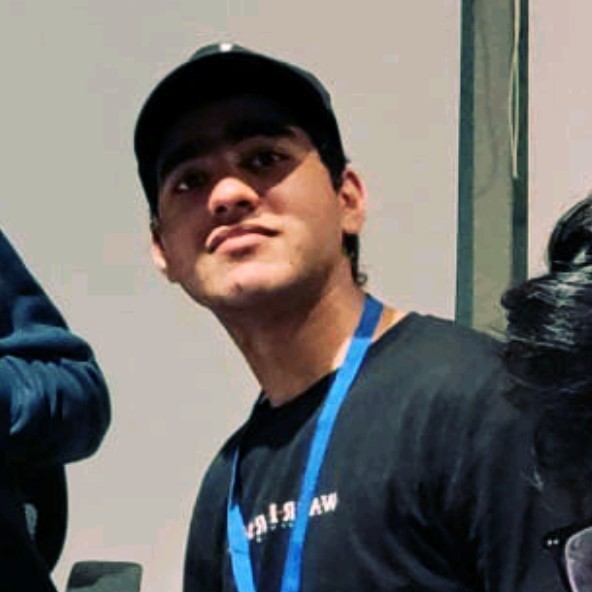
Eshaan Walia
Eshaan Walia
Hi, sometimes I write