What is an MCP Server? Ultimate Guide to Building Your Own AI Tools (2025)

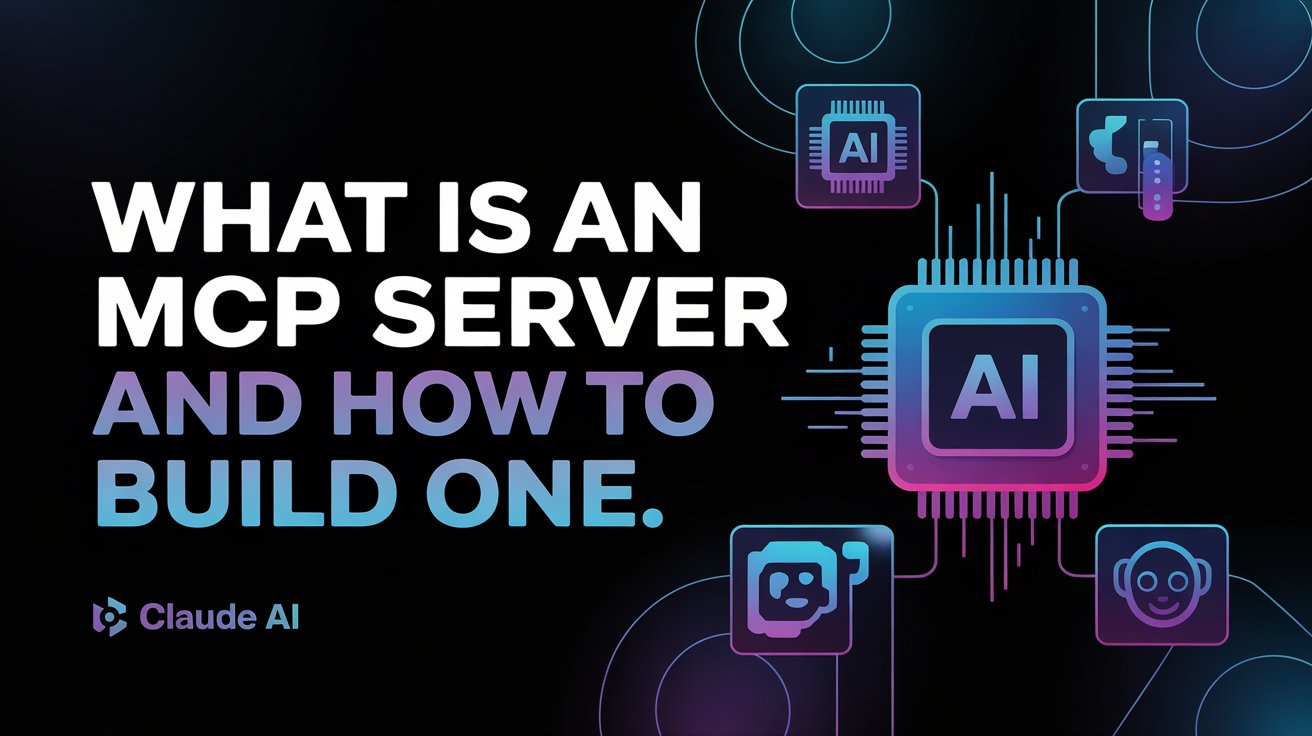
In today's rapidly evolving AI landscape, Model Context Protocol (MCP) servers are becoming increasingly important. They represent the next big thing in AI development, particularly for those working with Large Language Models (LLMs) and agent-based workflows. But what exactly are MCP servers, and why should you care about them? Let's dive in.
Understanding MCP Servers
MCP stands for Model Context Protocol, not Multi-Channel Protocol as some might assume. It was introduced by Anthropic, the company behind Claude. At its core, MCP is an open protocol that standardizes how applications provide context to Large Language Models (LLMs).
Think of MCP like a USB for AI applications. Just as USB provides a standardized way to connect your devices to various peripherals and accessories, MCP provides a standard way to connect AI models to different data sources and tools.
What Problem Does MCP Solve?
LLMs face two significant limitations:
Outdated Information: LLMs are pre-trained on data that becomes outdated. Even if you train a model daily (which is rare), its information would still be 24 hours old. Most models are trained much less frequently, perhaps once a year.
Limited Context Window: LLMs have a finite context window. If you want to ask about your city's news, you'd need to scrape and feed all relevant news into the LLM first, which is inefficient and costly.
MCP addresses these issues by defining how to efficiently provide context to a model in a structured way.
How MCP Works
The MCP ecosystem consists of three main components:
MCP Host: Programs like Claude Desktop, Claude AI, or any AI tool that needs access through MCP.
MCP Client: Software that maintains connections with MCP servers.
MCP Server: Lightweight programs that expose specific capabilities through standardized MCP.
Here's a simplified flow:
You have a conversation with an AI like Claude
When you ask something requiring external data (like weather)
The AI, through MCP, asks available servers if they can provide this information
The appropriate MCP server fetches only the needed data
The data is fed back into the AI's context
The AI provides a human-like response based on this fresh, specific data
Types of Context in MCP
MCP servers can provide context in several ways:
1. Tools
Tools are functions that LLMs can call. For example, a weather tool might take a city name as input and return current weather data. These are perhaps the most commonly used feature in MCP servers.
// Example of a weather tool in an MCP server
server.registerTool({
name: "getWeatherDataByCityName",
description: "Get current weather data for a specific city",
parameters: z.object({
city: z.string().describe("Name of the city")
}),
handler: async ({ city }) => {
// Function to fetch weather data
const weatherData = await getWeather(city);
return JSON.stringify(weatherData);
}
});
2. Resources
Resources allow you to expose file contents, database records, or API responses to the LLM. For instance, you might attach a CSV file or provide access to your JavaScript/TypeScript files.
3. Prompts
MCP servers can provide pre-built prompts or enhance user prompts. This is similar to the "Enhance this prompt" feature you might have seen in Claude, where the AI improves upon the user's initial instructions.
4. Sampling
Though less commonly used, sampling allows different models to provide context to each other. For example, you might use Claude for code generation but Gemini for test cases.
Building Your Own MCP Server
Now that we understand what MCP servers are, let's build a simple one. We'll create a weather data MCP server using TypeScript.
Step 1: Set Up Your Project
First, create a new directory and initialize your project:
mkdir my-mcp
cd my-mcp
npm init -y
Step 2: Install Dependencies
Install the MCP SDK and Zod for validation:
npm install @anthropic-ai/sdk @model-context-protocol/mcp.js zod
Step 3: Create Your Server
Create an index.js
file with the following code:
import { MCPServer } from "@model-context-protocol/mcp.js";
import { z } from "zod";
// Create an MCP server
const server = new MCPServer({
name: "weather-data",
version: "1.0.0"
});
// Create an async function to get weather data
async function getWeatherByCity(city) {
// In a real application, you would call a weather API here
// This is a simplified example with hardcoded responses
if (city.toLowerCase() === "patiala") {
return {
temperature: "30 degree Celsius",
forecast: "Chances of high rain"
};
} else if (city.toLowerCase() === "delhi") {
return {
temperature: "40 degree Celsius",
forecast: "Chances of warm winds"
};
} else {
return {
temperature: null,
forecast: "Unable to get the data"
};
}
}
// Register a tool for getting weather data
server.registerTool({
name: "getWeatherDataByCityName",
description: "Get current weather data for a specific city",
parameters: z.object({
city: z.string().describe("Name of the city")
}),
handler: async ({ city }) => {
const data = await getWeatherByCity(city);
return JSON.stringify(data);
}
});
// Initialize the server with standard input/output transport
import { StdioTransport } from "@model-context-protocol/mcp.js";
const transport = new StdioTransport();
server.connectToTransport(transport);
// Start the server
async function init() {
await server.initialize();
}
init();
Step 4: Configure Your Package.json
Make sure your package.json includes the type module:
{
"type": "module"
// other configurations...
}
Step 5: Run Your Server
You can run your server with:
node index.js
Step 6: Connect to an MCP Host
To use your MCP server with a host like Claude, you need to register it. In Claude Desktop or similar applications, you would go to settings and add a new MCP server with the path to your server script.
Transport Methods
MCP servers can communicate in two main ways:
1. Standard Input/Output (stdio)
This is the simplest method, where the server reads from and writes to the terminal. It's good for local integration but requires the code to run on the same machine as the client.
2. Server-Sent Events (SSE)
This allows remote access to your MCP server through HTTP. You can host your server on a domain and connect to it from anywhere.
import express from "express";
import { ServerSentEventsTransport } from "@model-context-protocol/mcp.js";
const app = express();
const sseTransport = new ServerSentEventsTransport();
// Connect your server to the SSE transport
server.connectToTransport(sseTransport);
// Set up the endpoint
app.post("/mcp", (req, res) => {
sseTransport.handlePostMessage(req, res);
});
app.listen(3000, () => {
console.log("MCP server running on port 3000");
});
Real-World Applications
MCP servers open up countless possibilities:
Database Interactions: Create MCP servers for MongoDB, PostgreSQL, or any database to let AI interact with your data.
Design Tools: Imagine controlling Figma through natural language.
Video Editing: Control Premier Pro or other editing software via prompts.
Corporate Tools: Access Slack, Teams, or GitHub directly through AI interfaces.
Real-time Data: Get weather, stock prices, or any API data seamlessly integrated into AI responses.
Best Practices for MCP Server Development
Security First: Be careful about what capabilities you expose. Implement proper authentication and authorization.
Efficient Context: Only provide the context that's needed. Sending too much data is inefficient and costly.
Error Handling: Implement robust error handling in your tools and resources.
Documentation: Clearly document what your MCP server does and how to use it.
Versioning: Use semantic versioning for your MCP servers to manage changes.
The Future of MCP
As AI continues to evolve, MCP servers will likely become a standard part of the ecosystem. Major companies like GitHub, Slack, Teams, and others will likely host their own MCP servers, creating a rich library of capabilities that can be plugged into any AI system.
For developers, this represents an exciting opportunity to create tools that extend AI capabilities into specific domains and applications. Whether you're interested in freelancing in the AI world or building the next big AI-powered application, understanding MCP servers is becoming increasingly crucial.
Conclusion
MCP servers represent a significant advancement in how we interact with AI models. By standardizing the way context is provided to LLMs, they make AI more capable, more current, and more useful for specific tasks.
Building your own MCP server is relatively straightforward and opens up a world of possibilities for creating AI-powered tools and applications. As this ecosystem grows, we can expect to see more standardization, more capabilities, and more innovative uses of AI in our daily workflows.
Whether you're a developer looking to extend AI capabilities or a business looking to leverage AI more effectively, MCP servers are definitely worth exploring.
Subscribe to my newsletter
Read articles from Aman Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aman Raj
Aman Raj
I’m a full-stack developer and open-source contributor pursuing B.Tech in CSE at SKIT, Jaipur. I specialize in building scalable web apps and AI-driven tools. With internship experience and a strong portfolio, I’m actively open to freelance projects and remote job opportunities. Let’s build something impactful!