#NoStupidQuestions: What is an API in simple terms? How is it useful? Why was it needed? How does it work? And a bit of its history.
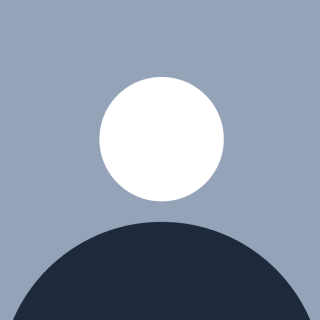
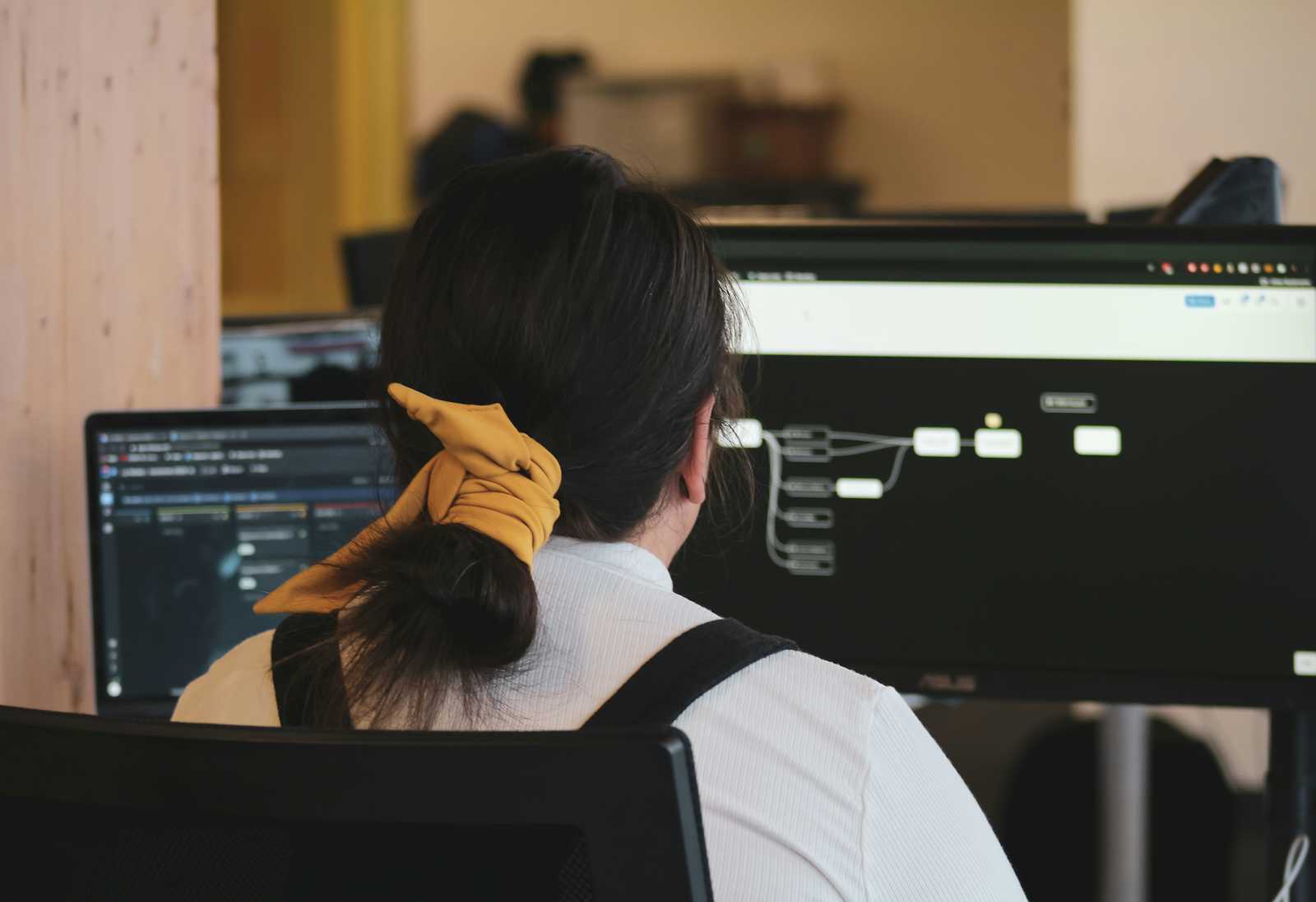
Back in the early days, computers worked alone. Each program did its job without talking to other programs or machines. Imagine having a phone but not being able to text or call anyone—that’s how computers were back then.
As technology improved, people wanted computers and programs to share information. For example, a weather app needed to get data from a weather station, or a shopping website needed to check your bank balance. Likewise, banks needed a secure way to share only your balance—and nothing else—with services that were allowed to see it.
The solution? APIs (Application Programming Interfaces).
The Birth of APIs
Let’s go back to the late 1960s when the idea of making computers “talk” to one another and the core concept of APIs started taking shape. Computers were big, expensive, and not interconnected. At that time, the focus was on making these machines more powerful.
As software developers built new programs, they realized that one of the best ways to enhance their creations was to create standardized interfaces that would allow different programs to talk to each other. They needed a way for different software systems to interact without needing to “understand” every detail of one another.
The Need for APIs
But the concept of APIs, as we know them today, didn’t take shape until the rise of the internet in the 1990s. The internet revolutionized everything, and businesses began to realize the power of connecting with one another. But instead of reinventing the wheel every time a new piece of software was needed, they realized that they could simply “ask” other programs for the services or data they required. And this is where the true power of APIs came in.
Think of APIs as waiters in a restaurant. You (the user) have a menu of options (services or data you need), and the waiter (API) takes your order and brings back exactly what you requested from the kitchen (the server or service). Just like a waiter doesn’t need to know the details of how the food is cooked, APIs don’t need to understand the internal workings of the programs they connect—they just need to know how to request and return data.
The reason APIs became necessary was clear: businesses and developers didn’t want to reinvent the wheel every time they built something. Instead, they wanted to leverage existing technology and data from other sources, like payment processors (iDeal or Stripe, for instance), mapping services (like Google Maps), or even weather data providers. Without APIs, each app would need to build every service from scratch, which would be both inefficient and impractical.
APIs helped solve several problems:
1. Modularity: Instead of reinventing the wheel, developers could reuse existing solutions.
2. Interoperability: Different systems and applications could now work together, even if they were built on different technologies.
3. Efficiency: APIs help businesses work faster and use fewer resources by letting specialized services handle specific tasks. For example, a travel website doesn’t need to collect flight data itself—it uses an airline API to fetch up-to-date flight schedules.
Key Concepts to Understand APIs
To truly understand APIs, there are a few important concepts:
1. Endpoints: The specific URLs where an API can be accessed. Each endpoint represents a different function (like fetching user data).
An API have a URL. Something like https://api.website.com
. Generally speaking, it's still kind of a website. It still runs on a server. It's still built with technologies that are used for websites. The difference is you don't use a web browser (and click a button) to directly get any information. And, that's the most important part.
You use a program to send a request to the API’s URL. The API then responds with data.
For example, a food delivery app.
When you order food, the app needs to:
Find nearby restaurants
Check if the food is available
Calculate delivery time
Process your payment
Each of these steps uses different APIs:
A restaurant API to list available places (https://api.foodapp.com/restaurants?location=NewYork)
A menu API to show food options (https://api.foodapp.com/menu?restaurant_id=123)
A maps API to estimate delivery time (https://api.maps.com/delivery?from=Restaurant&to=YourHome)
A payment API to charge your card (https://api.payment.com/pay?amount=20&card=xxxx)
Your app then displays this information in a user-friendly way.
2. Methods: The types of requests that can be made to the API, typically GET (retrieve data), POST (send data), PUT (update data), and DELETE (remove data).
3. Authentication: Ensuring that only authorized users or systems can access the API, often through API keys or OAuth tokens.
4. Rate Limiting: Limiting the number of requests that can be made to an API in a certain period to avoid overwhelming the system.
How APIs Work
An API is like an agreement between two people—let’s call them Person A and Person B. This agreement outlines what Person A can ask Person B to do, such as raising a hand, taking a step forward, stepping back, adding two numbers, or subtracting two numbers.
But the agreement doesn’t just say what Person A can ask—it also defines how to ask. It specifies the exact way Person A should approach Person B and the correct words to use for each request. Whenever A follows these rules and asks B for something, B must respond in the expected way.
Now, replace Person A and Person B with Program A and Program B, and that’s exactly what an API does! The I in API stands for Interface, meaning a way for systems to communicate. APIs allow programs to send requests, retrieve data, or perform actions—just like an agreement ensures smooth communication between two people.
In technical terms, an API is a set of protocols and tools that allow different software systems to communicate with each other. Again, it defines the rules for how one program can interact with another.
Here’s a simple breakdown of how it works:
Imagine a library that keeps all its books behind a counter. If you want a book, you need to ask the librarian for it. The librarian (the API) knows which books are available and will bring you the book you requested.
1. Request: The client (your app, website, or service) sends a request to an API. (like asking the librarian for a specific book).
The client is making an HTTP request to the API to ask for specific data (a book in this case). The request include parameters such as the book title.
import requests
# Client sends a request (like asking the librarian for a specific book)
url = "https://example-library-api.com/books" # URL of the API
params = {'book_name': 'Python for Beginners'} # Book details (like asking for a specific book)
# Send the request to the API
response = requests.get(url, params=params)
# Check if the request was successful
if response.status_code == 200:
print("Request sent successfully.")
else:
print(f"Error: Unable to send request. Status code: {response.status_code}")
2. Processing: The API takes the request and passes it to the relevant backend service (for example, a database or server)
Below’s a simplified version of the API that handles the request and communicates with a backend service (a mock database). The API processes the client’s request, checks if the book is available in the mock database, and prepares a response.
from flask import Flask, request, jsonify
app = Flask(__name__)
# Mock "database" of books
books_database = {
'Python for Beginners': {'author': 'Grace Doe', 'year': 2020},
'Advanced Python': {'author': 'Jane Smith', 'year': 2021}
}
@app.route('/books', methods=['GET'])
def get_book():
book_name = request.args.get('book_name') # Extracting the book name from the request
if book_name in books_database:
return jsonify(books_database[book_name]) # Return book details if found
else:
return jsonify({'error': 'Book not found'}), 404
if __name__ == "__main__":
app.run(debug=True) # Do not set debug to True on production
3. Response: The backend sends the data or service back through the API to the requester.
In the example above, if the book “Python for Beginners” is found in the database, the API sends back a JSON response containing the book’s author and year of publication.
import requests
# Client sends a request (like asking the librarian for a specific book)
url = "http://127.0.0.1:5000/books" # URL of the local API
params = {'book_name': 'Python for Beginners'} # Book name to search for
# Send the request to the API
response = requests.get(url, params=params)
# Step 3: Processing the response
if response.status_code == 200:
# Successfully received a response, now processing the JSON data
book_data = response.json() # Convert the JSON response to a Python dictionary
print("Book found!")
print(f"Title: Python for Beginners")
print(f"Author: {book_data['author']}")
print(f"Year: {book_data['year']}")
else:
print(f"Error: {response.json()['error']}") # Display error if the book was not found
APIs typically use HTTP/HTTPS for communication and return data in standardized formats like JSON (JavaScript Object Notation) or XML (eXtensible Markup Language).
# Example response from the API:
{
"author": "Grace Doe",
"year": 2020
}
4. Action: The API then returns this result to the client, which processes it and displays it to the user.
Example Output (if book is found):
Book found!
Title: Python for Beginners
Author: Grace Doe
Year: 2020
What do you think the output will be if the book is not found?
The Technologies Around APIs
The world of APIs has evolved alongside the growth of the internet. A few key technologies and standards that shape the modern API ecosystem include:
JSON & XML: Data formats for transferring information.
HTTP/HTTPS: The foundation of communication over the web.
Websites that start with https:// are safer than those with http://. Think of it like sending a letter:
✉️ HTTP = A postcard (anyone can read it).
🔒 HTTPS = A sealed envelope (only the right person can see inside).
When you use HTTPS, you’re just telling the web to keep your data private and secure!
HTTP (HyperText Transfer Protocol) is a protocol that websites follow to send and receive information over the internet. It sets the rules for how information is sent and received between your device (like a phone or computer) and a website (or the app you built).
For example, when you click a link or type a website address, your browser sends a request following these rules. The website then replies using the same rules, making sure your browser understands and displays the page correctly.
HTTPS is the same thing but with extra security (SSL/TLS encryption). This means:
It scrambles your data while it’s being sent, so hackers can’t read it.
It protects sensitive info like passwords and credit card numbers.
REST (Representational State Transfer): One of the most common architectural styles used for creating APIs today. REST APIs use HTTP methods like GET, POST, PUT, and DELETE to interact with resources on a server. They are simple, stateless, and lightweight, making them highly scalable.
SOAP (Simple Object Access Protocol): A more formal, older approach compared to REST. SOAP is highly structured and uses XML for message formatting, but it can be more rigid and complex to work with.
GraphQL: A newer API standard that allows clients to request exactly the data they need. Instead of having fixed endpoints, you can ask for specific data within a single query, offering more flexibility compared to REST.
OAuth: A security protocol often used with APIs to allow third-party services to access your data without giving them your password. For example, when you use your Google account to log into a new app, OAuth is handling the authentication.
SDKs & Libraries: These help developers interact with APIs easily by providing tools and pre-written code.
In essence, APIs are the unsung heroes of the modern digital world. They allow everything from your favorite mobile apps to large-scale enterprise systems to interact with one another seamlessly. They were born out of the need for efficiency, integration, and innovation——and they still play a huge role in technology today. Whether you’re ordering a ride, making a payment, or or integrating an AI model into your app, APIs are the behind-the-scenes links that make it all happen.
Subscribe to my newsletter
Read articles from anj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by