Declarative Programming in React.js
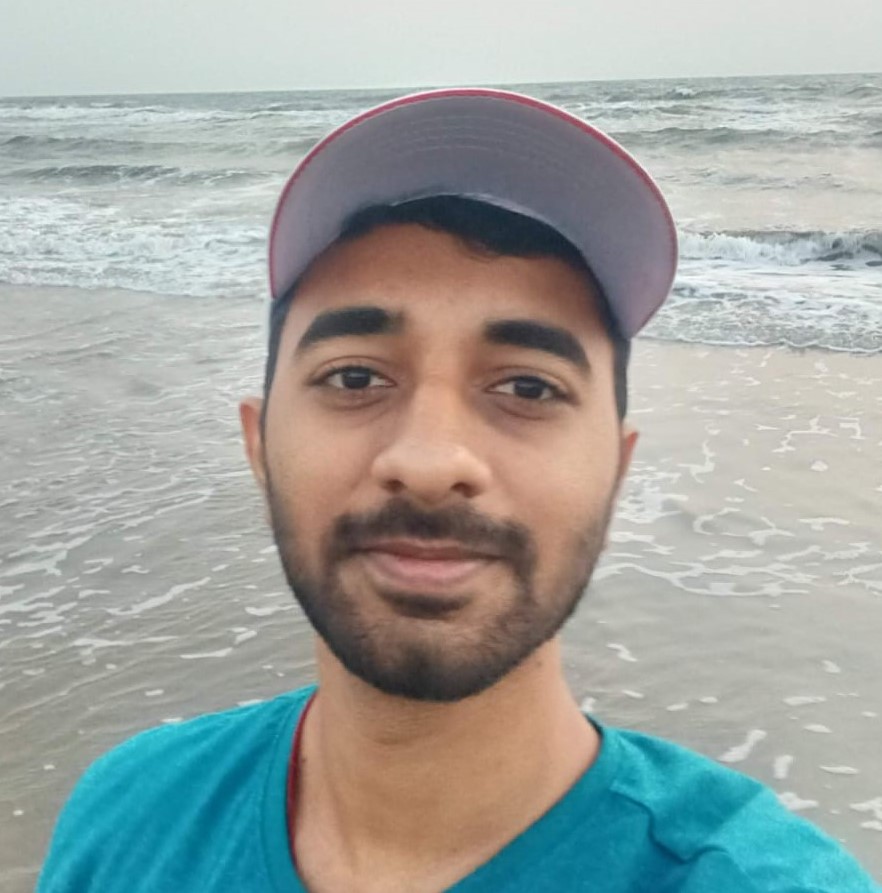
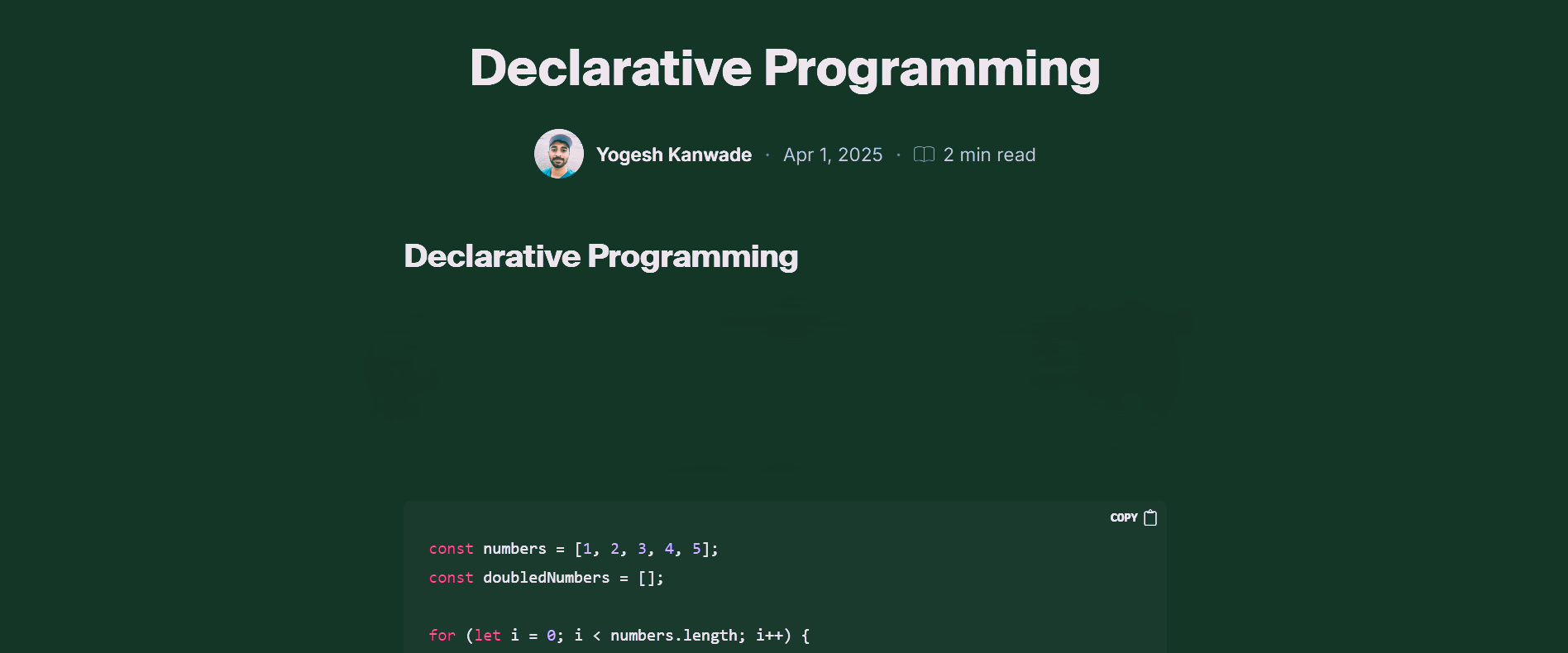
What is Declarative Programming
Declarative programming is a paradigm where you focus on what the program should accomplish rather than how it should do it.
Let’s start with a simple piece of code to double the numbers of an array. The below code shows imperative approach.
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = [];
for (let i = 0; i < numbers.length; i++) {
doubledNumbers.push(numbers[i] * 2);
}
Its declarative counterpart given below, we declare what we want (map
transforms the array) and let JavaScript handle the iteration.
const doubledNumbers = numbers.map(num => num * 2);
Another good example would be
const removeSelectedItem = (index: number) => {
const updatedItems = selectedItems.slice();
// create a copy of existing selected items using array method .slice
updatedItems.splice(index, 1);
// remove item (.splice returns removed item, which we arent storing anywhere here)
dispatch(setMultiSelectItem(updatedItems));
// now updatedItems is our required array
};
So here rather than specifying how to get the updatedItems
, we can simply use a more straight-forward, declarative approach that also aligns more with functional programming(pure functions that follow immutability).
const removeSelectedItem = (index: number) => {
const updatedItems = selectedItems.filter((_, i) => i !== index));
};
React.js (A Declarative Framework)
<button onClick={handleClick}>Click me</button>
Why is this declarative?
You describe what should happen when clicked (
onClick={handleClick}
).React handles how the event listener is attached behind the scenes.
Imperative Counterpart (Vanilla JavaScript)
const button = document.createElement("button");
button.textContent = "Click me";
button.addEventListener("click", handleClick);
document.body.appendChild(button);
Why is this imperative?
You manually create the button element.
You explicitly attach an event listener.
You specify how the button should be added to the DOM.
TL;DR (Declarative v/s Imperative)
Feature | Declarative | Imperative |
Focus | What to achieve | How to achieve it |
Control Flow | Hidden (handled by language) | Explicit (you define every step) |
Readability | More concise, easier to understand | Can be verbose and complex |
Examples | SQL, React, Functional Programming (map, filter, reduce) | Loops, conditionals, procedural code (C) |
Subscribe to my newsletter
Read articles from Yogesh Kanwade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
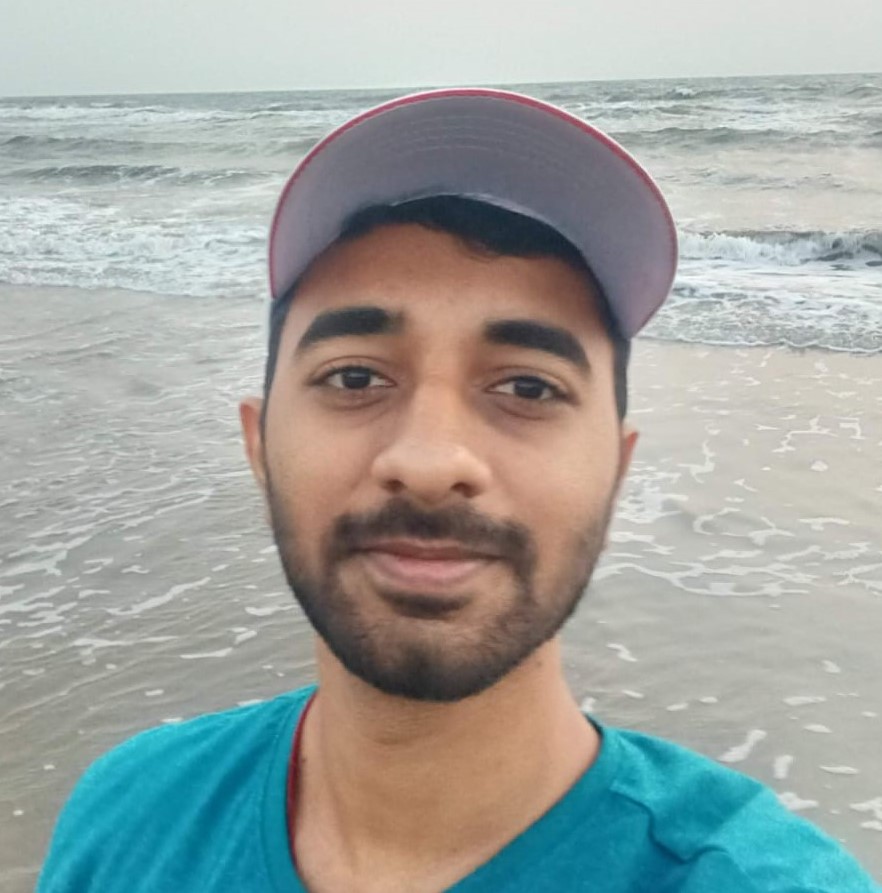
Yogesh Kanwade
Yogesh Kanwade
I'm Yogesh Kanwade, a final year Computer Engineering student with a deep passion for software development. I am a continuous learner, with hardworking and goal-driven mindset and strong leadership capabilities. I am actively exploring the vast possibilities of Web Development along with AWS and DevOps, fascinated by their impact on scalable and efficient web solutions.