How I Patched a NPM Library and Used It in Production
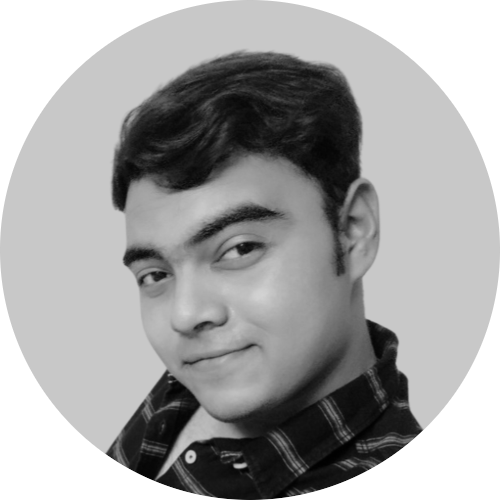
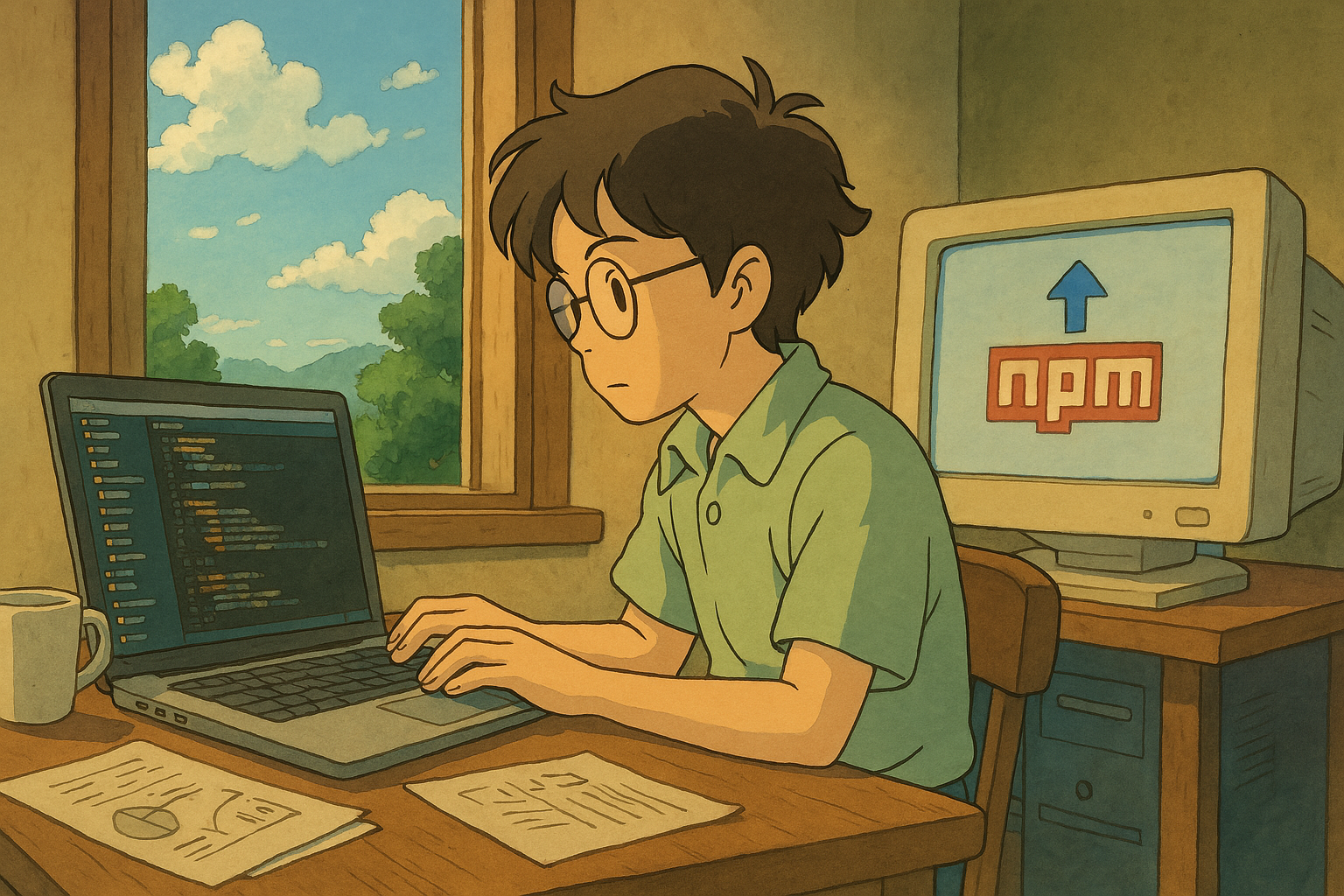
The Problem: A PR That Wasn't Merged
In our project, we were using react-arborist
for managing a tree view component. However, we needed a feature that wasn’t available in the official package. After searching, I found an open pull request (PR) that added exactly what I needed, but it hadn’t been merged yet.
Instead of waiting indefinitely, I decided to fork the repository, apply the PR myself, and use the patched version in our project. Here’s how I did it step by step.
Step 1: Forking the Repository and Checking Out the PR Branch
The first step was to fork the original repository on GitHub and check out the branch from the existing PR.
# Clone the forked repo
git clone https://github.com/yourusername/react-arborist.git
cd react-arborist
# Fetch the PR branch (assuming the PR branch is named `feature-branch`)
git fetch origin pull/238/head:feature-branch
# Switch to the PR branch
git checkout feature-branch
This gave me a local copy of the unmerged PR to work with.
Step 2: Testing the Feature Locally with npm link
Before using it in my main project, I needed to test the feature locally. To do this, I used npm link
:
Build the package (if necessary)
Many npm packages require a build step before they can be used. I checked the
package.json
for build scripts and ran:npm install npm run build # If there's a build script
Create a local npm link
In the root of
react-arborist
, I ran:npm link
This made the package globally available on my system.
Use the linked package in my main project
In my actual project directory, I ran:
npm link react-arborist
Now, my project was using my locally modified version of
react-arborist
instead of the one from npm.Test the feature
After linking, I tested the changes in my project. Once I confirmed that everything was working, I moved to productionizing the package.
Step 3: Creating a Tarball (.tgz
) and Hosting It for Free
To use the package in production, I needed to package it and host it somewhere accessible. Since I wanted a CDN-backed solution, I used GitHub and JSDelivr.
Create an npm tarball
npm pack
This generated a file like
react-arborist-1.0.0.tgz
.Upload the Tarball to GitHub
I created a new GitHub repository (or used an existing one) and uploaded the tarball as a release asset or a raw file.
Use JSDelivr to Serve It from a CDN
Once the
.tgz
file was uploaded to GitHub, I could serve it using JSDelivr’s GitHub CDN. The URL follows this pattern:https://cdn.jsdelivr.net/gh/yourusername/yourrepo@main/react-arborist-1.0.0.tgz
To find the exact URL for your file, navigate to the file on GitHub and copy its raw URL. Then, replace
raw.githubusercontent.com
withcdn.jsdelivr.net
.
Step 4: Installing the Custom Package in My Project
Now that the package was available via a CDN, I could install it in my project by updating package.json
:
"dependencies": {
"react-arborist": "https://cdn.jsdelivr.net/gh/yourusername/yourrepo@main/react-arborist-1.0.0.tgz"
}
Then, I ran:
npm install
Now my project was using the patched version of react-arborist
, served from a free CDN!
Conclusion
This approach allowed me to:
Use an unmerged PR in production without waiting for the official package to update.
Host a patched npm package for free using GitHub and JSDelivr.
Seamlessly integrate the package into my project.
If you ever find yourself needing a feature that’s stuck in an unmerged PR, you can follow this process to fork, patch, and use it in your own projects!
Subscribe to my newsletter
Read articles from Milind Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
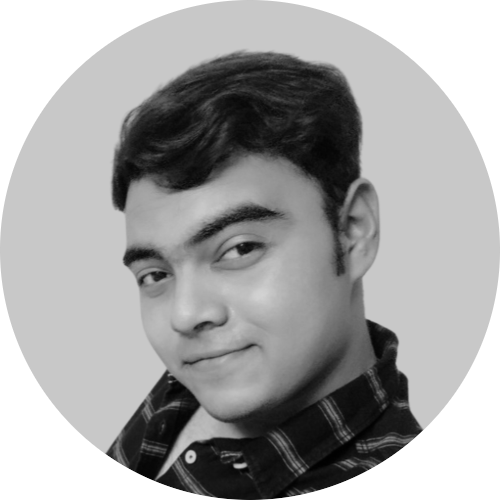
Milind Mishra
Milind Mishra
I'm a Developer from Bangalore, India, and I've recently been falling in love with Design and Development. I really enjoy learning languages and Web Development frameworks like React.