Python: Errors, Exceptions and the Raise Keyword
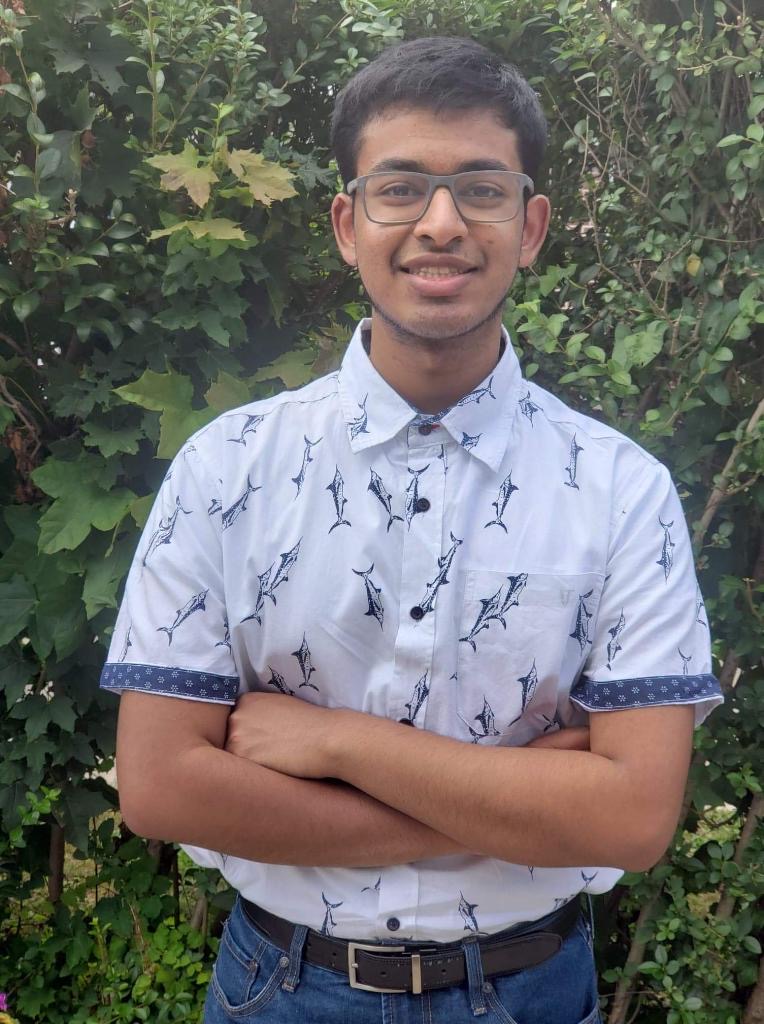
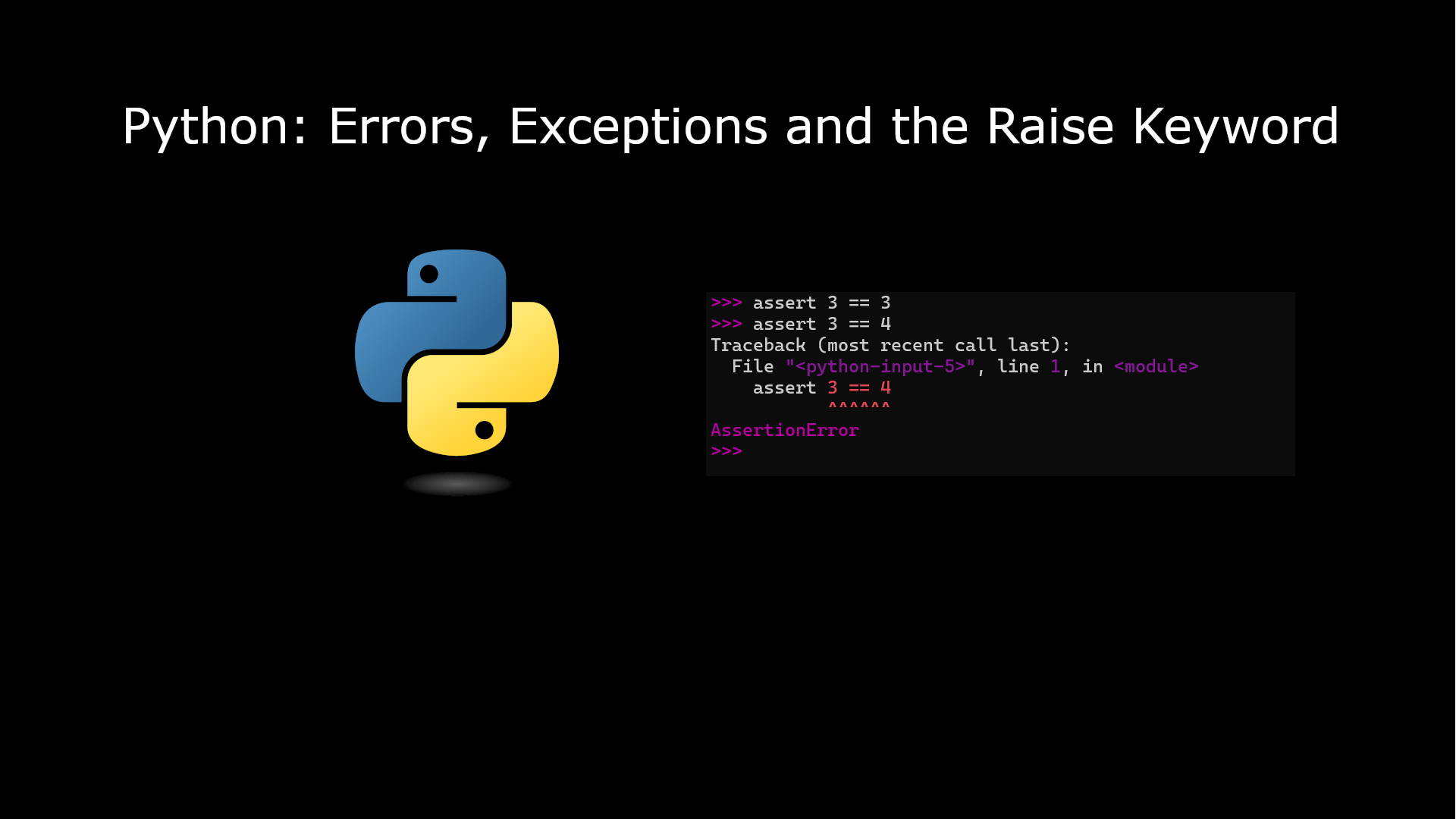
Overview: What an Error and Exception is
This article will explain in the Python Programming Language, how a few different errors can be triggered when there are certain Python syntax mistakes when a Python script or code is run. Exceptions (1) can be implemented to catch different errors by using try and except statements to catch and even bypass an error and continue running the remainder of the Python script. An exception can also be triggered by using the “raise” keyword, which will also be demonstrated.
Materials
Computer
Python (2)
Different Errors
Below are examples of different errors that can happen.
Assertion Error
The keyword “assert” validates if a statement is True. If the statement is False, then an assertion error occurs. The statement 3 == 3 is True but not the statement 3 == 4, therefore, an assertion error would occur.
Arithmetic Error
An arithmetic error occurs when there is a problem with a mathematical statement. An arithmetic error is the most general error that occurs when there is a mathematical problem in the code. Common arithmetic errors are called type error, value error, or zero-division error.
Type Error
A type error occurs when a different data type is incorrectly used with another data type. Below shows an example, where a string data type is trying to be added to an integer. Of course, it is not possible to add a number with a string and receive a numerical result.
Value Error
A value error occurs when there is a statement that is incorrectly casted, or a value cannot be displayed due to certain conditions.
Zero-Division Error
A zero-division error is when division occurs where the denominator number is a zero. Dividing any number by zero does not exist.
KeyBoard Interrupt
A keyboard interrupt error can occur if a variable asks the user for an input, but CTRL + C is pressed instead to exit out and cancel the running script.
Import Error
An import error occurs when a module is imported but a function that does not exist is trying to be used.
Module Not Found Error
A module not found error occurs when a file or a module is trying to be imported and there seems to be an error for one of the possible reasons: the file that is being read is in the wrong directory or does not exist, the package is not installed on the system to use the module or the name that is written after “import” is incorrectly spelt.
Lookup Error
A lookup error occurs when searching for an element from a certain data type is not found. Common lookup errors are index errors and key errors.
Index Error
An Index Error occurs when an element is trying to be accessed from a data type that exceeds or is below its index. Remember, that elements from a list can be accessed using negative numbers too.
Key Error
A key error occurs when trying to access a key from a dictionary that does not exist.
Memory Error
A memory error occurs when a Python script takes too long to run a command or commands because it requires a lot of memory to store the result in real-time, therefore, it will crash because there is no more memory left to complete the script’s output.
Name Error
A name error occurs when trying to print out a variable that does not exist.
Overflow Error
An Overflow Error occurs when the result of a mathematical operation outputs too many digits. The terminal will not output the result but instead stay blank unless the user manually exits out of the process, or it throws an overflow error. Below shows an image of 10 being raised by a large number, and the output will not be displayed on the terminal.
Syntax Error
A syntax error occurs when a statement is entered in Python, but it is written incorrectly, or something is missing.
Errors Script
Below is the script that was used to receive the many errors shown above. Before running, uncomment the code to replicate the errors.
# Different Exceptions
# Assertion Error
# assert == 4
# Arithmetic Errors:
# Type Error
# print(3 + "hi")
# Value Error
# print(int("hi"))
# Zero-Division Error
# print(3 / 0)
# KeyBoard Interrupt
# write_something = input("Enter a word: ")
# When running the write_something variable, press CTRL + C to exit out when asked of an input
# Import Error
# from math import s
# Module Not Found Error
# import insert_module_name
# Lookup Errors
# Index Error
# a_list = [1,2,3]
# print(a_list[3])
# Key Error
# a_dictionary = {"one": 1, "two": 2}
# print(a_dictionary["three"])
# Name Error
# print(Hello)
# Overflow Error
# 10 * 100000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000
# Syntax Error
# print(3 + )
How to use Exceptions in a Python Script or Code
Exceptions are often used with try and except statements to catch specified errors by the user. It is optional for a try and except statement to include an else or finally block, and it can be written in the following format:
try:
# Write code for the Python script to run
except:
# This block of code runs only if an error occurs in the try block
else:
# This block of code runs only if no errors were detected in the try block
finally:
# This block of code always runs
Examples of using try and except Blocks
Below shows how to write a try and except statement:
try:
print(3 / 0)
except:
print("An error occurred")
Usually, running three divided by zero would result in a Zero-Division Error, but the most general code to catch any exception would be is to specify the word “except:” or “except Exception:”. To run this code optimally, the except statement can specify a particular error:
try:
print(3 / 0)
except ZeroDivisionError:
print("An error occurred")
It is possible to include more than one except statement and the order matters. Since specifying except will catch any error, it is not possible to write “except” and then include another except statement that particularly specifies one error statement. If more than one except statement is in the try and except block, it has to be written as shown below.
try and block Script
try:
num1 = int(input("Enter a number: "))
num2 = int(input("Enter another number: "))
print(num1/num2)
except ZeroDivisionError:
print("Can't have a denominator number by 0")
except:
print("An error occurred")
else:
print("No errors detected")
finally:
print("Code is finished running")
If the zero division error is not triggered, then the other except statement will catch any other errors if any has occurred. If not except statements has occurred, then the else statement will occur after the try statement. The finally block will always run after the try and except blocks, or the try and else blocks.
Different Scenarios of using try and except:
Dividing a number by Zero:
Detecting a Different Error:
No Errors Were Detected:
Giving Exceptions Different Names and How to Use the Raise Keyword
Exception name blocks can be renamed in a different way by using the keyword as and then state a word in order to give it another name.
try:
print(3/0)
except Exception as e:
e
To print the details of the error to the terminal, the keyword “raise” is needed
try:
print(3/0)
except Exception as e:
raise e
Sources
Subscribe to my newsletter
Read articles from Andrew Dass directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
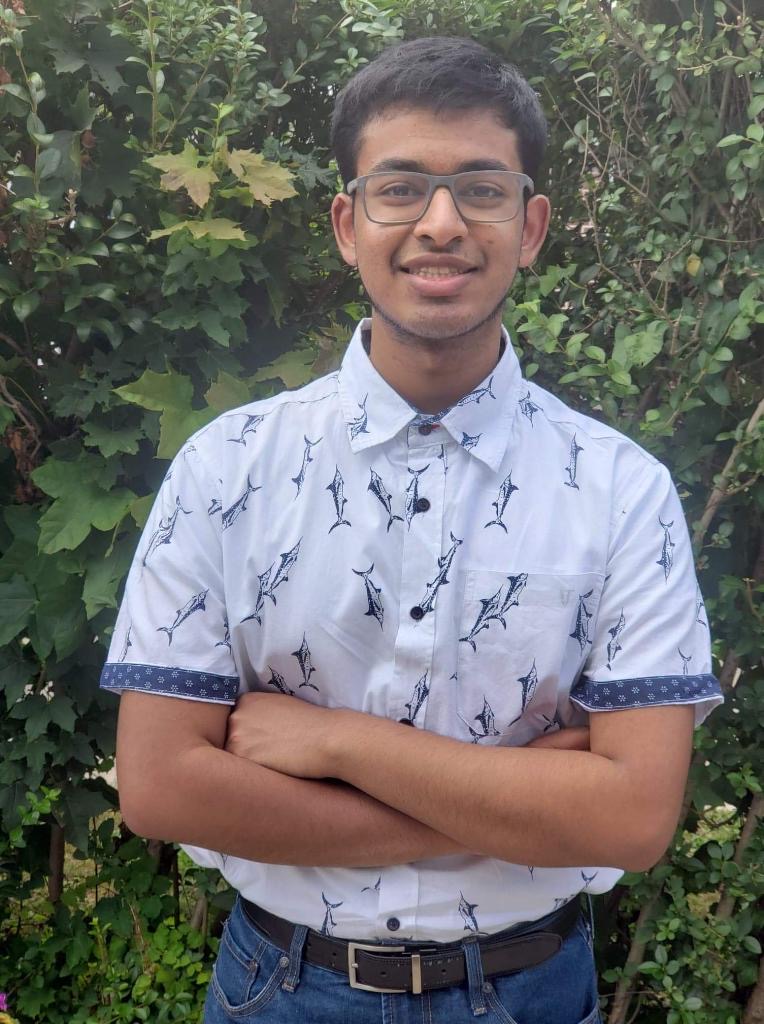
Andrew Dass
Andrew Dass
On my free time, I like to learn more about hardware, software and different technologies. I publish articles about my recent learnings or the projects I have done.