🚀How to create the EC2 instance in AWS Using boto3 python
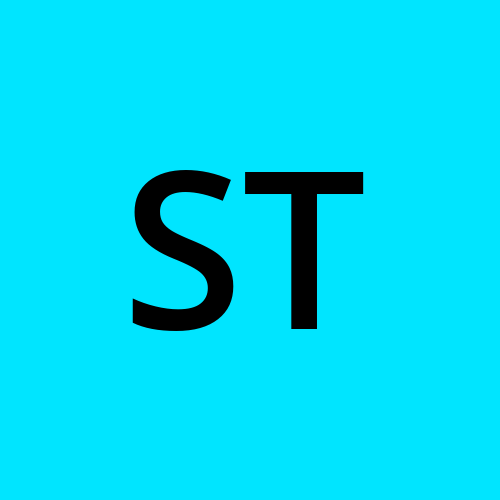
1 min read

Here’s a Python script using boto3
to create an EC2 instance in AWS. Make sure you have AWS credentials configured using aws configure
or environment variables.
Configure AWS CLI credentials:
aws configure
Prerequisites:
Install
boto3
:pip install boto3
Create Ec2 Instance
import boto3
def create_ec2_instance():
ec2 = boto3.resource('ec2')
instance = ec2.create_instances(
ImageId='ami-0c55b159cbfafe1f0', # Replace with your AMI ID
MinCount=1,
MaxCount=1,
InstanceType='t2.micro', # Change as needed
KeyName='your-key-pair-name', # Replace with your key pair name
SecurityGroupIds=['sg-0123456789abcdef0'], # Replace with your security group ID
SubnetId='subnet-0123456789abcdef0', # Replace with your subnet ID
TagSpecifications=[
{
'ResourceType': 'instance',
'Tags': [{'Key': 'Name', 'Value': 'MyEC2Instance'}]
}
]
)
print(f'EC2 instance {instance[0].id} created successfully!')
if __name__ == "__main__":
create_ec2_instance()
Explanation:
Replace
ami-0c55b159cbfafe1f0
with an appropriate AMI ID for your region.Set the
KeyName
to your existing key pair.Use the correct
SecurityGroupIds
andSubnetId
.
0
Subscribe to my newsletter
Read articles from SRINIVAS TIRUNAHARI directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
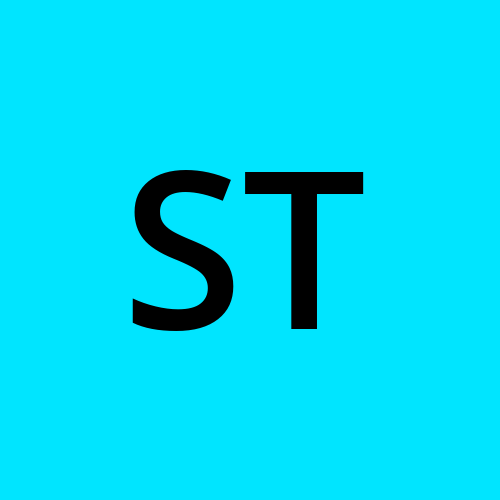