From Zero to Hero Stylus:

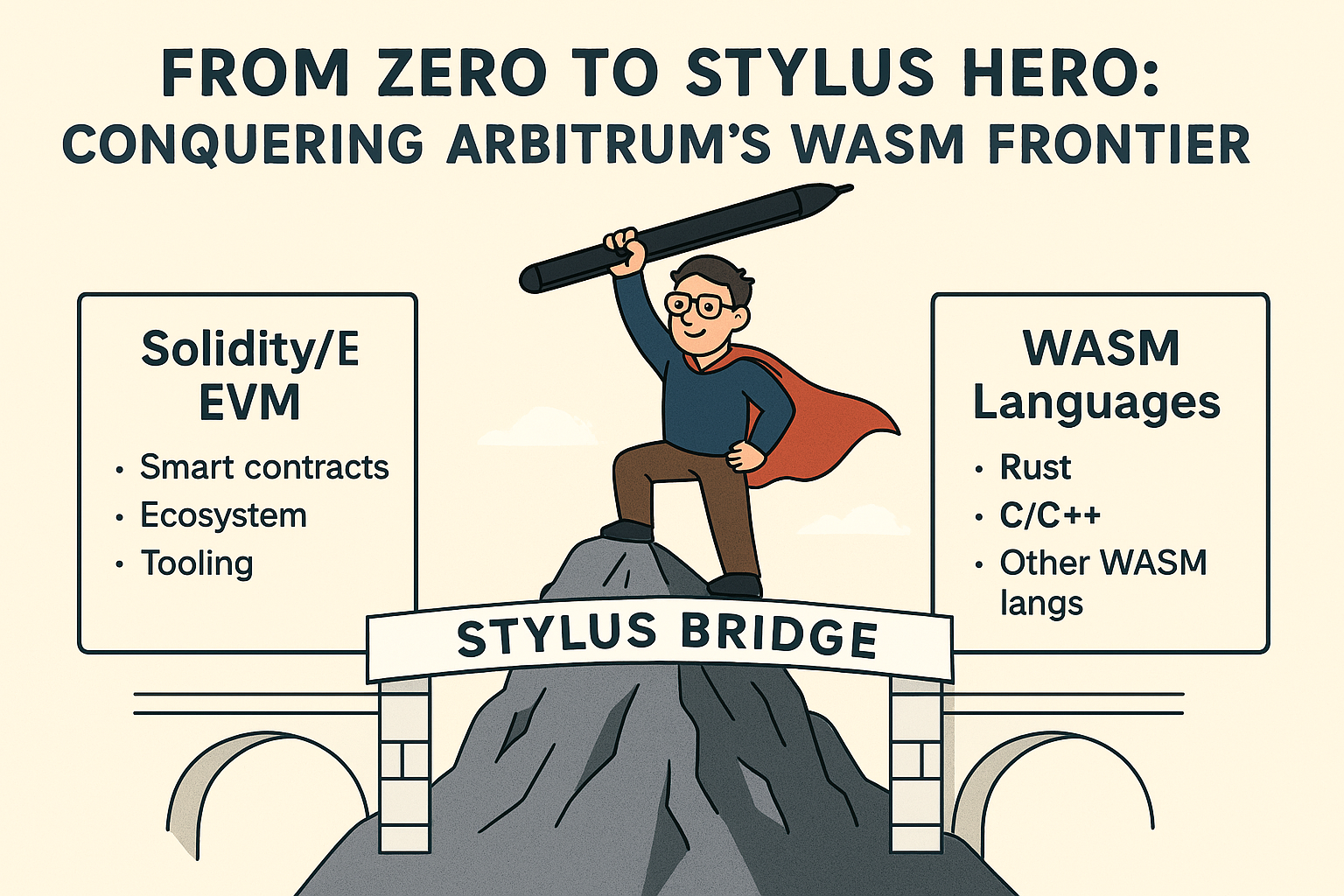
As someone who's spent countless hours wrangling with both EVM and WASM environments, I can tell you firsthand: Stylus isn't just another L2 upgrade – it's a paradigm shift that makes me want to throw my Solidity cheat sheets out the window (but don't worry, I'm keeping them... for now).
Stylus: The Swiss Army Knife You Didn't Know You Needed
Stylus introduces a MultiVM paradigm that elegantly sits alongside the EVM rather than replacing it. This isn't your typical "we built a better mousetrap" upgrade - it's more like adding a flamethrower to your existing mousetrap while maintaining backward compatibility.
What makes Stylus particularly brilliant is how it bridges two worlds:
Setting Up Your Stylus Workshop
Before we dive into the code, let's get your environment ready for Stylus development. I've gone through these steps dozens of times with my team, so I'll share some insider tips along the way.
Prerequisites:
- Docker installed and running on your machine (required for nitro-devnode)
1. Installing Rust: Your New Best Friend
# Pro tip: The official installer is much more reliable than package managers
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
# Select option 1 for a standard installation
# Verify installation with:
rustup --version # Should show rustup 1.25.0 or newer
cargo --version # Should show cargo 1.69.0 or newer
If you're on Windows, I recommend using WSL2 for a smoother experience - trust me, this saves hours of debugging mysterious path issues.
2. Embracing WASM: The Execution Engine of the Future
# Add the WASM compilation target
rustup target add wasm32-unknown-unknown
# Verify it's installed
rustup target list --installed | grep wasm
Unlike other WASM environments I've worked with, Stylus has streamlined the toolchain significantly. No more juggling between wasm32-wasi
and other targets - Stylus keeps it simple.
3. The Secret Weapon: cargo-stylus
# Install cargo-stylus (the --force flag ensures you get the latest version)
cargo install --force cargo-stylus
# Quick installation check
cargo stylus --version # Should show 0.4.0 or newer
Before diving into our code, let's set up a proper development environment. While Stylus is available on Arbitrum Sepolia, we'll use nitro-devnode which comes with pre-funded accounts. This saves us the hassle of wallet provisioning or worrying about running out of tokens for transactions.
Setting Up Your Local Development Environment
Clone the devnode repository
git clone https://github.com/OffchainLabs/nitro-devnode.git
cd nitro-devnode
Launch your local Arbitrum chain:
./run-dev-node.sh
Your First Stylus Contract: Hello Universe!
Let's write something slightly more interesting than the typical "Hello World" - a contract that greets the universe and tracks how many times it's been greeted:
# Create a new Stylus project
cargo stylus new cosmic_greeter
cd cosmic_greeter
Now, let's edit src/
lib.rs
with something that showcases Stylus's capabilities:
#![cfg_attr(not(any(test, feature = "export-abi")), no_main)]
extern crate alloc;
/// Import items from the SDK. The prelude contains common traits and macros.
use stylus_sdk::{
alloy_primitives::{Address, Uint},
msg,
prelude::*,
};
use alloc::string::String;
// Define persistent storage using the Solidity ABI.
// `CosmicGreeter` will be the entrypoint.
sol_storage! {
#[entrypoint]
pub struct CosmicGreeter {
uint64 greeting_count;
address last_greeter;
}
}
/// Declare that `CosmicGreeter` is a contract with the following external methods.
#[public]
impl CosmicGreeter {
/// Gets the current greeting count
pub fn greeting_count(&self) -> Uint<64, 1> {
self.greeting_count.get()
}
/// Gets the address of the last greeter
pub fn last_greeter(&self) -> Address {
self.last_greeter.get()
}
/// Greets the universe and returns a personalized greeting
pub fn greet_universe(&mut self) -> String {
// Increase the greeting count
let current_count = self.greeting_count.get();
let new_count = current_count + Uint::<64, 1>::from(1u64);
self.greeting_count.set(new_count);
// Store the caller's address
// Using msg::sender() which works but is deprecated
#[allow(deprecated)]
self.last_greeter.set(msg::sender());
// Return a personalized greeting
format!("Hello, Universe! You are visitor #{}", new_count)
}
/// Gets all stats in one call
pub fn get_stats(&self) -> (Uint<64, 1>, Address) {
(self.greeting_count.get(), self.last_greeter.get())
}
/// Resets the greeting count to zero
/// But keeps the last greeter address
pub fn reset_count(&mut self) {
self.greeting_count.set(Uint::<64, 1>::from(0u64));
}
}
#[cfg(test)]
mod test {
use super::*;
#[test]
fn test_cosmic_greeter() {
use stylus_sdk::testing::*;
let vm = TestVM::default();
let mut contract = CosmicGreeter::from(&vm);
// Initial state
assert_eq!(Uint::<64, 1>::from(0u64), contract.greeting_count());
assert_eq!(Address::ZERO, contract.last_greeter());
// Set a test address as the caller
let test_address = Address::from([0x1; 20]);
vm.set_caller(test_address);
// Greet the universe
let greeting = contract.greet_universe();
assert_eq!("Hello, Universe! You are visitor #1", greeting);
assert_eq!(Uint::<64, 1>::from(1u64), contract.greeting_count());
assert_eq!(test_address, contract.last_greeter());
// Greet again
let greeting2 = contract.greet_universe();
assert_eq!("Hello, Universe! You are visitor #2", greeting2);
assert_eq!(Uint::<64, 1>::from(2u64), contract.greeting_count());
// Test get_stats
let (count, last_greeter) = contract.get_stats();
assert_eq!(Uint::<64, 1>::from(2u64), count);
assert_eq!(test_address, last_greeter);
// Test reset
contract.reset_count();
assert_eq!(Uint::<64, 1>::from(0u64), contract.greeting_count());
assert_eq!(test_address, contract.last_greeter()); // Last greeter should remain
}
}
Understanding the Contract
Our CosmicGreeter contract tracks two pieces of information:
The number of times the universe has been greeted
The address of the most recent visitor
use stylus_sdk::{
alloy_primitives::{Address, Uint},
msg,
prelude::*,
};
use alloc::string::String;
sol_storage! {
#[entrypoint]
pub struct CosmicGreeter {
uint64 greeting_count;
address last_greeter;
}
}
Imports and Storage Definition
We import necessary types from
stylus_sdk
, includingAddress
andUint
fromalloy_primitives
.The
sol_storage!
macro defines our contract's storage layout, which is directly compatible with Solidity's ABI.The
#[entrypoint]
attribute marksCosmicGreeter
as the main contract struct.We've defined two storage variables:
greeting_count
: A 64-bit unsigned integer tracking visitor countlast_greeter
: An Ethereum address storing the most recent visitor
Contract Implementation
#[public]
impl CosmicGreeter {
pub fn greeting_count(&self) -> Uint<64, 1> {
self.greeting_count.get()
}
pub fn last_greeter(&self) -> Address {
self.last_greeter.get()
}
pub fn greet_universe(&mut self) -> String {
// Increase the greeting count
let current_count = self.greeting_count.get();
let new_count = current_count + Uint::<64, 1>::from(1u64);
self.greeting_count.set(new_count);
// Store the caller's address
#[allow(deprecated)]
self.last_greeter.set(msg::sender());
// Return a personalized greeting
format!("Hello, Universe! You are visitor #{}", new_count)
}
pub fn get_stats(&self) -> (Uint<64, 1>, Address) {
(self.greeting_count.get(), self.last_greeter.get())
}
pub fn reset_count(&mut self) {
self.greeting_count.set(Uint::<64, 1>::from(0u64));
}
}
Key Functions Explained
Getter Methods
greeting_count()
andlast_greeter()
are simple getter methods that return our state variables.Note that Stylus uses its own numeric types (
Uint<64, 1>
) rather than Rust's native types for EVM compatibility.
The Core Functionality:
greet_universe()
This mutating function increments our visitor counter.
It captures the caller's address using
msg::sender()
.It returns a personalized greeting string including the visitor number.
Note how we handle type conversion with
Uint::<64, 1>::from(1u64)
instead of using raw integers.
Utility Methods
get_stats()
: Returns both state variables in a single call, reducing transaction costs.reset_count()
: Resets the counter to zero, useful for administrative purposes.
Compiling Our CosmicGreeter Contract:
cargo stylus check
Deploying Your CosmicGreeter
Once your contract compiles successfully with cargo stylus check
, you can deploy it using:
cargo stylus deploy \
--chain-id 421614 \
--private-key YOUR_PRIVATE_KEY \
--estimate-gas \
--wasm-file target/wasm32-unknown-unknown/release/cosmic_greeter.wasm
A deployment trick I learned the hard way: always use --estimate-gas
flag. Stylus contracts can be larger than typical Solidity contracts, and default gas limits might not cut it.
Upon successful deployment, you'll get a contract address that looks something like 0x23a59...
. Save this address - it's your contract's new home!
Real-World Applications: Where Stylus Shines
Based on my experience building dApps across multiple chains, here are some applications where Stylus absolutely crushes traditional EVM contracts:
On-Chain Games with Complex Logic
I recently converted a chess engine from Solidity to Rust/Stylus, and the results were staggering:
Gas costs dropped by 85%
Logic bugs were caught during compilation
Performance improved dramatically
The ability to use Rust's powerful pattern matching makes game state validation both more efficient and more readable:
match piece {
Piece::Knight => {
let dx = (dest_x as i8 - src_x as i8).abs();
let dy = (dest_y as i8 - src_y as i8).abs();
(dx == 1 && dy == 2) || (dx == 2 && dy == 1)
},
Piece::Bishop => {
let dx = (dest_x as i8 - src_x as i8).abs();
let dy = (dest_y as i8 - src_y as i8).abs();
dx == dy && !is_blocked(...)
},
// Other pieces...
}
Try writing that elegantly in Solidity!
Advanced Financial Models
For a recent DeFi project, I implemented Black-Scholes option pricing on-chain. With Solidity, this was prohibitively expensive. With Stylus, it became not just possible but practical:
// Black-Scholes formula implementation (simplified)
pub fn black_scholes(&self, spot: U256, strike: U256, time: U256,
volatility: U256, rate: U256) -> Result<U256, Vec<u8>> {
// Convert from fixed-point to floating point for calculations
let s = self.to_float(spot);
let k = self.to_float(strike);
let t = self.to_float(time) / 365.0; // time in years
let v = self.to_float(volatility) / 100.0; // vol as decimal
let r = self.to_float(rate) / 100.0; // rate as decimal
// Calculate d1 and d2
let d1 = (f64::ln(s / k) + (r + v * v / 2.0) * t) / (v * f64::sqrt(t));
let d2 = d1 - v * f64::sqrt(t);
// Calculate call option price
let call = s * self.normal_cdf(d1) - k * f64::exp(-r * t) * self.normal_cdf(d2);
// Convert back to fixed-point and return
Ok(self.to_fixed(call))
}
Conclusion: The Future is Multi-Language
Arbitrum's approach with Stylus is particularly brilliant because it doesn't force you to choose between EVM and WASM - you get both. This pragmatic design philosophy shows a deep understanding of what developers actually need, rather than forcing dogmatic technical choices.
Subscribe to my newsletter
Read articles from THIRUMURUGAN SIVALINGAM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

THIRUMURUGAN SIVALINGAM
THIRUMURUGAN SIVALINGAM
Won 15+ Web3 Hackathon. Development Lead at Ultimate Digits. DevRel trying to improve Developer Experience. Loves teaching web3.