Mastering MVC Architecture in Spring Boot: A Complete Guide
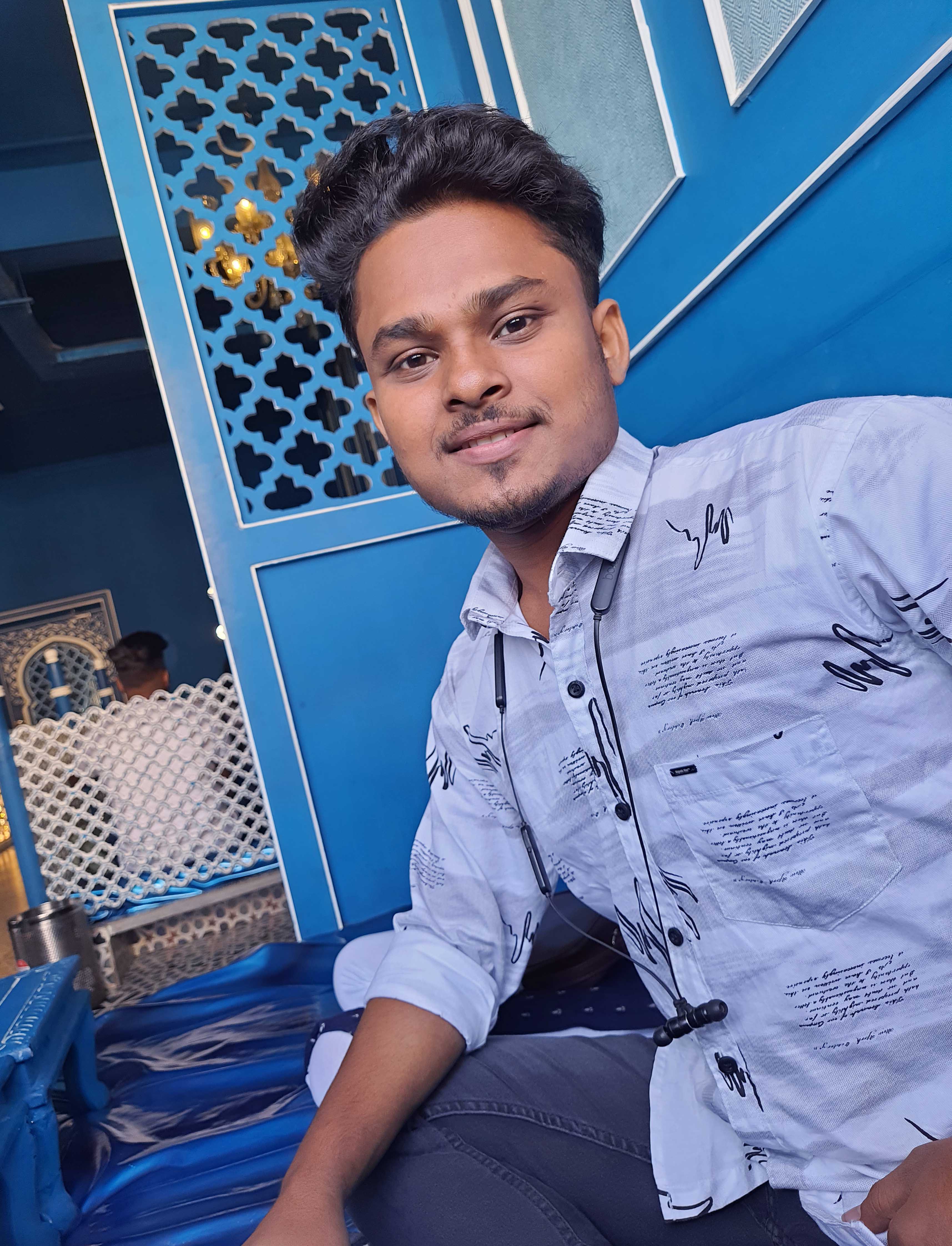
What is MVC architecture ?
MVC (Model-View-Controller) is a software architectural pattern used for developing user interfaces that divides an application into three interconnected components. This thing helps developer and future developer to organize their codes, making it more maintainable and scalable.
Three Parts of MVC(Model-View-Controller)
Model:
Definition: The model represents the data and the business logic of the application. It directly manages the data, logic, and rules of the application.
Responsibilities:
Data Management: It retrieves data from the database and processes it.
Business Logic: It handles business rules and operations like validating inputs, processing data, etc.
View:
Definition: The view represents the UI (User Interface) of the application. It displays the data from the model to the user.
Responsibilities:
- Data Presentation: It takes data from the model and presents it in a format suitable for the user (like HTML, JSON, etc.).
Controller:
Definition: The controller acts as an intermediary between the Model and the View. It processes incoming requests, manipulates the data (via the model), and returns the appropriate view.
Responsibilities:
Handling User Input: It listens for user inputs (e.g., HTTP requests) and processes them.
Updating the Model: It interacts with the model to perform the necessary operations (e.g., save data, fetch records).
Returning the View: It returns the view that will display the appropriate data (in the case of a web application, this is typically an HTML page or JSON).
How MVC Works in Spring Boot:
Request Handling:
The user sends an HTTP request (
like GET,POST,PUT,PATCH
).The Spring controller receives the request and processes it using its corresponding method (
@GetMapping
or@PostMapping
).
Business Logic:
- The controller delegates the task to the model (e.g., a service class) to handle business logic or interact with the database.
Return Data to View:
After processing, the controller returns a view (for a web application) or data (for an API).
For REST APIs, the controller returns data (often in JSON format).
Benefits of MVC in Spring Boot:
Separation of Concerns:
- MVC divides an application into three distinct sections, making it easier to manage and maintain each component independently.
Code Reusability:
- Since the model and view are separated, the same model can be used by different views (e.g., a web page and a mobile app). The controller can also handle multiple requests for different views.
Testability:
- Each component in MVC can be tested individually. For example, you can unit test business logic in the model, without worrying about the view or controller.
Maintainability:
- Changes in the view (UI) don’t affect the business logic or data layer. Similarly, changes in business logic don’t affect the view, making the system more maintainable.
Scalability:
- As your application grows, MVC allows easy scaling by updating or adding new views or controllers while keeping the model logic intact.
Real-World Example in Spring Boot:
Imagine you’re building an e-commerce application. The MVC structure would look something like this:
Model: Represents products, orders, and customers. These are your entities (
Product
,Order
,Customer
) that interact with the database.View: The user interface displaying products, order details, and customer information. This would be your react component that represent the UI.
Controller: The
ProductController
,OrderController
, andCustomerController
handle incoming HTTP requests for viewing, creating, or modifying products, orders, and customer data.
Conclusion:
MVC architecture plays a crucial role in organizing and structuring web applications. By dividing the application into distinct components (Model, View, Controller), it promotes maintainability, scalability, and flexibility. In Spring Boot, MVC makes development easier, allowing developers to focus on their domain logic, user experience, and request handling without the complexity of tightly coupled code.
Subscribe to my newsletter
Read articles from RAJESH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
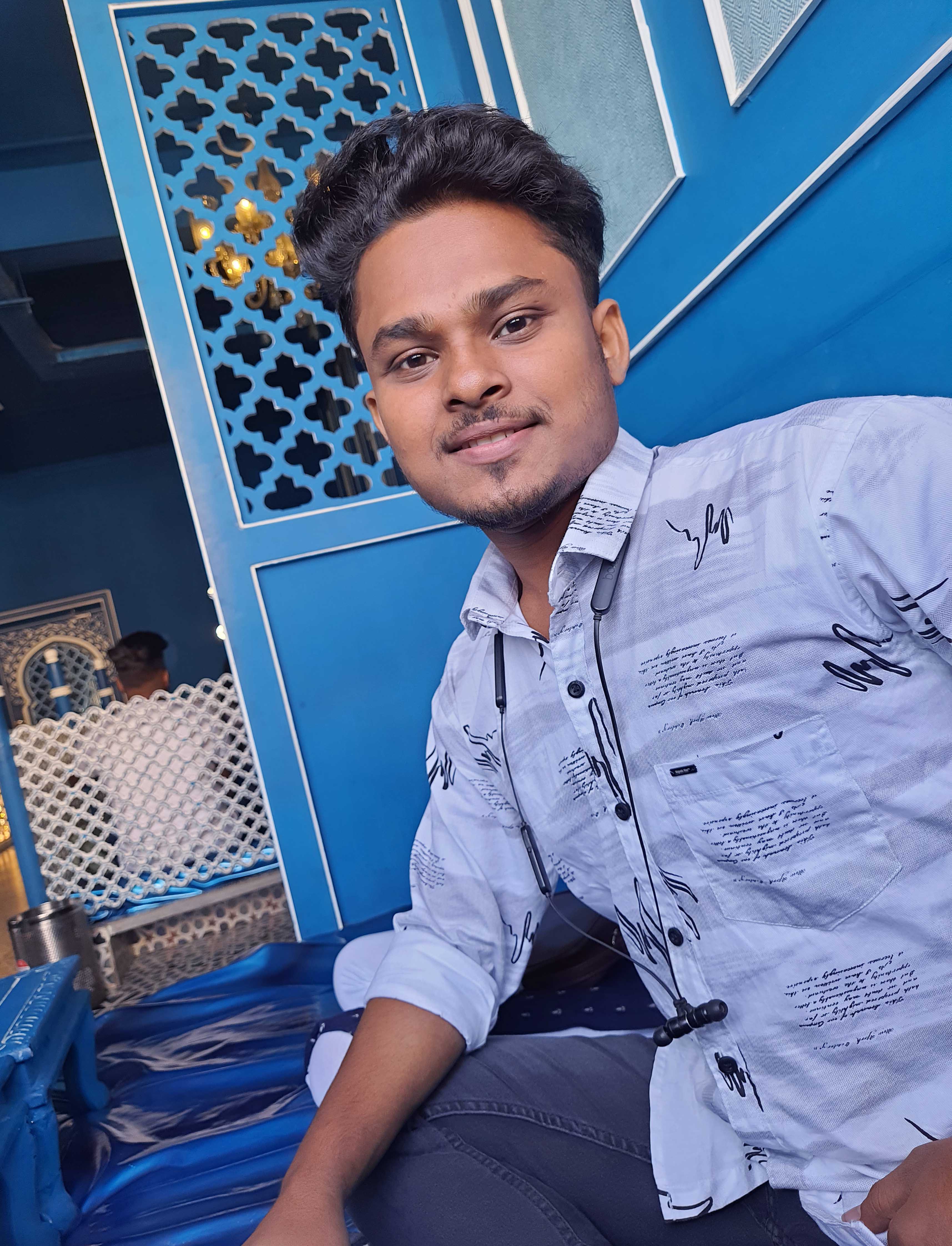