Roman to Integer: LeetCode
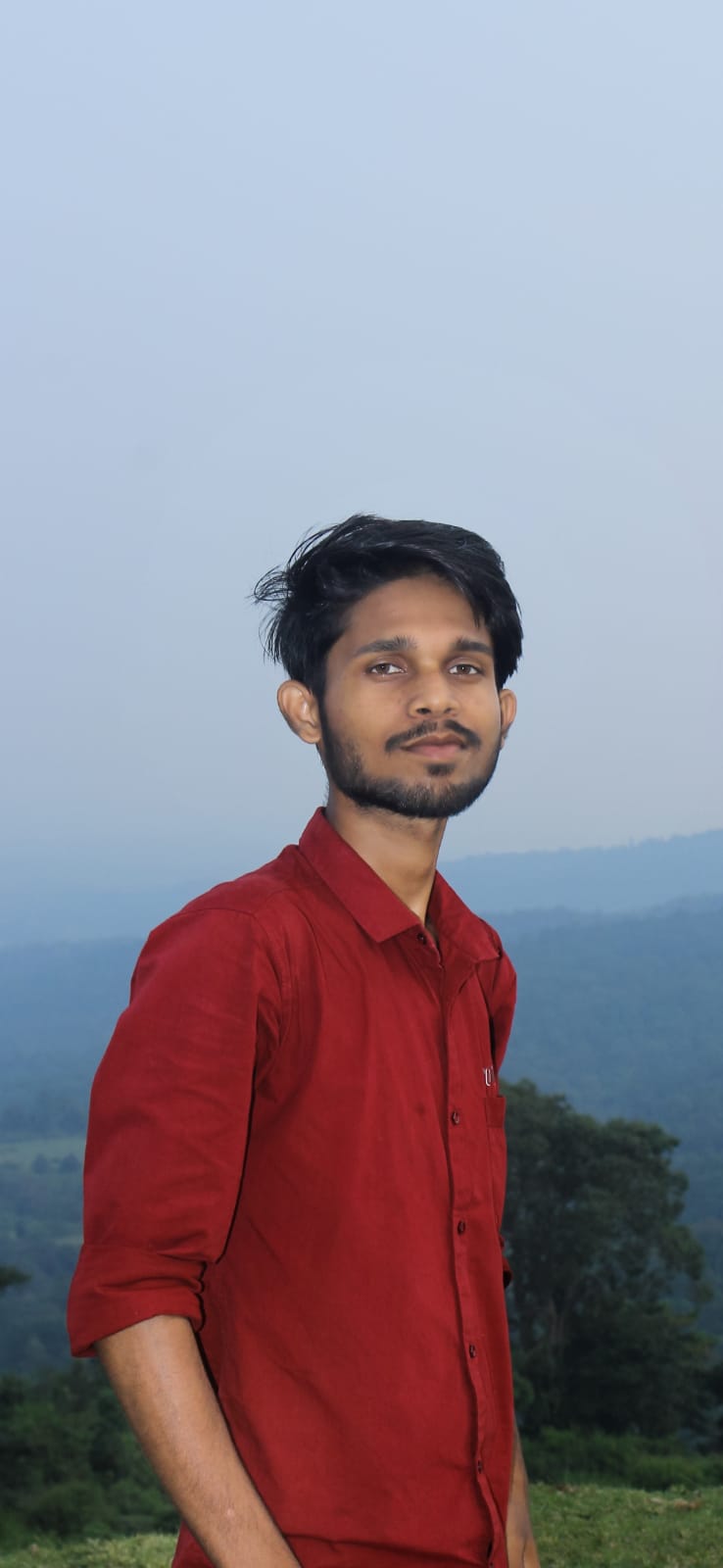
Have you ever wondered how Roman numerals like XIV or MCMXCIV translate into modern-day numbers? If you’re a Dart enthusiast, you’re in for a treat! In this blog, I’ll walk you through an efficient way to convert Roman numerals to integers using Dart.
Understanding Roman Numerals
Before diving into the code, let’s take a quick refresher on Roman numeral rules:
Symbols:
- I = 1, V = 5, X = 10, L = 50, C = 100, D = 500, M = 1000
Basic Rules:
Numerals are usually written from largest to smallest (e.g., VI = 6).
If a smaller numeral appears before a larger numeral, it must be subtracted (e.g., IV = 4, IX = 9).
With this in mind, let’s code a Dart function that can convert any valid Roman numeral into an integer.
Writing the Dart Function
Let’s jump straight into the implementation:
class Solution {
int romanToInt(String s) {
// Mapping Roman numerals to their respective values
Map<String, int> lettersMap = {
'I': 1, 'V': 5, 'X': 10, 'L': 50, 'C': 100, 'D': 500, 'M': 1000
};
int res = 0;
// Iterating through each character of the string
for (int i = 0; i < s.length; i++) {
// If a smaller numeral precedes a larger one, subtract it
if (i + 1 < s.length && lettersMap[s[i]]! < lettersMap[s[i + 1]]!) {
res -= lettersMap[s[i]]!;
} else {
// Otherwise, add its value to the result
res += lettersMap[s[i]]!;
}
}
return res;
}
}
Breaking Down the Logic
Here’s how the function works step by step:
Create a Map: We define a
lettersMap
to store Roman numeral values.Initialize a Result Variable:
res = 0
will store our final number.Loop Through the String: We iterate through each character of the input string.
Handle Special Cases:
If a numeral is smaller than the next one, we subtract its value.
Otherwise, we simply add its value.
Return the Result: Once the loop completes, we return
res
, which contains the converted integer.
Testing Our Function
A function is only as good as its test cases! Let’s run our solution with a few examples:
tvoid main() {
Solution solution = Solution();
print(solution.romanToInt("III")); // Output: 3
print(solution.romanToInt("IV")); // Output: 4
print(solution.romanToInt("IX")); // Output: 9
print(solution.romanToInt("LVIII")); // Output: 58
print(solution.romanToInt("MCMXCIV")); // Output: 1994
}
Why This Solution Works Efficiently
Time Complexity: O(n) → We iterate through the string once.
Space Complexity: O(1) → We use a fixed-size map and a few variables, regardless of input size.
Final Thoughts
Do you have other ways to tackle this problem? Let’s discuss in the comments!
Subscribe to my newsletter
Read articles from Ishu Prabhakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
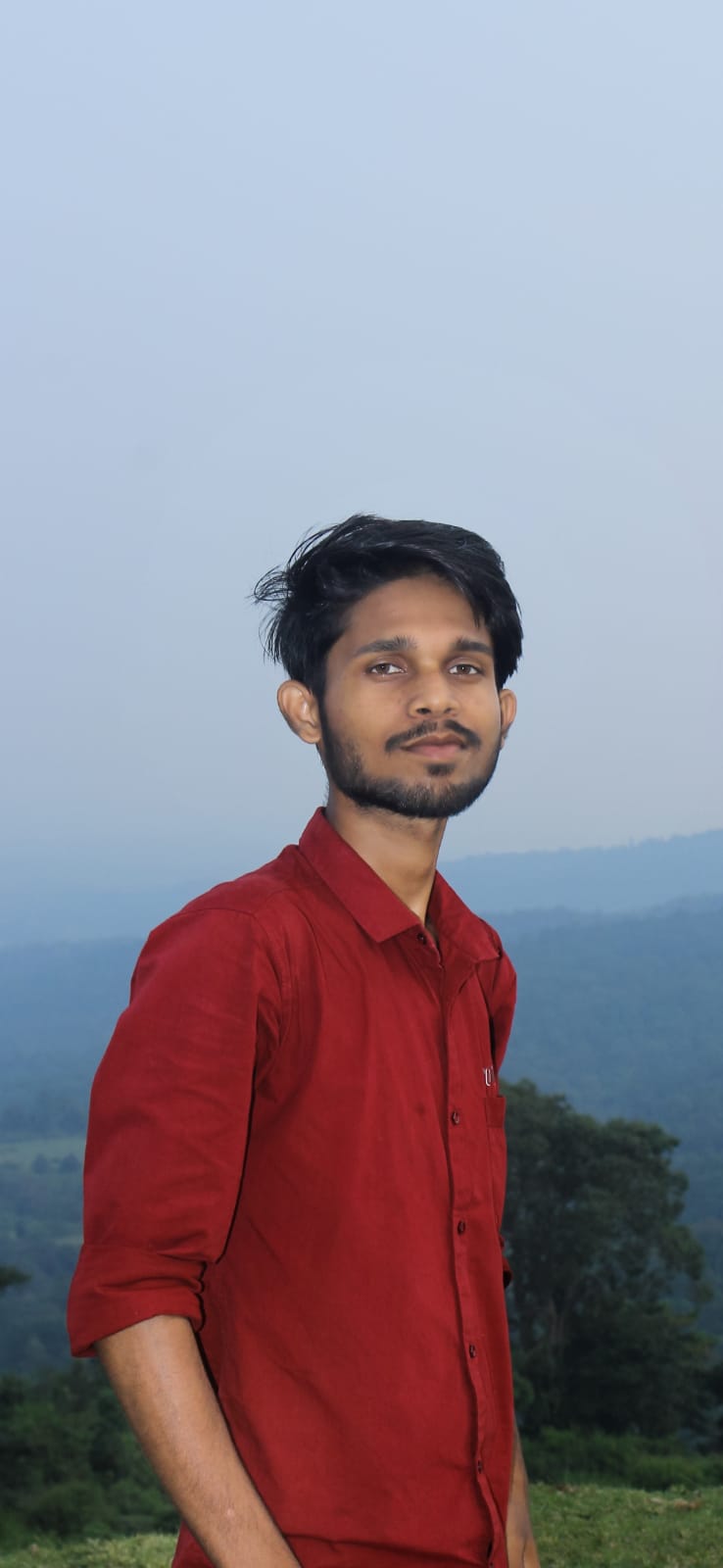