How to Set Up AWS Lambda in a Spring Boot Scheduler Project
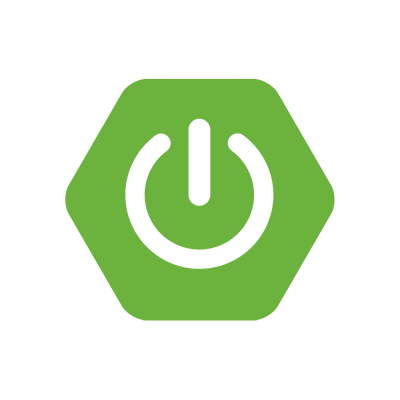
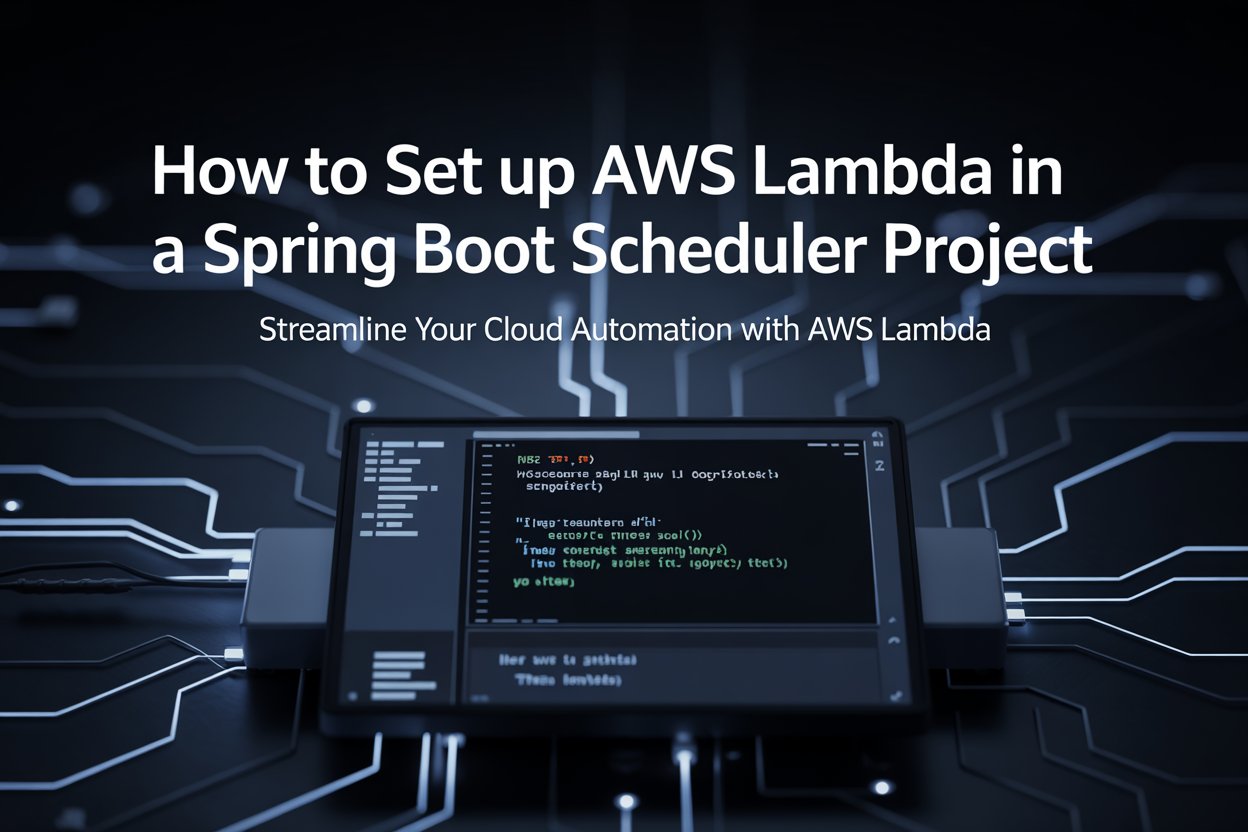
Introduction
Imagine you have a Spring Boot application that runs scheduled tasks using @Scheduled
, but now you want to offload some of these tasks to AWS Lambda for better scalability, cost-efficiency, and reliability. How do you integrate AWS Lambda with your Spring Boot scheduler? Let's explore step by step!
Why Use AWS Lambda for Scheduled Tasks?
Before diving into the setup, let’s understand why moving scheduled tasks to AWS Lambda can be beneficial:
Scalability: AWS Lambda automatically scales as per the workload.
Cost-Efficiency: You only pay for execution time, unlike a constantly running server.
Reliability: No need to manage infrastructure—AWS handles failovers.
Reduced Server Load: Offloading tasks to Lambda frees up your Spring Boot application resources.
Now, let’s set it up!
Step 1: Create an AWS Lambda Function
AWS Lambda is a serverless compute service that runs code in response to triggers. To integrate it with a Spring Boot scheduler, we’ll create a Lambda function that performs a scheduled task, such as clearing expired cache entries.
Login to AWS Console and navigate to Lambda.
Click Create function → Choose Author from scratch.
Enter function name (e.g.,
ClearCacheLambda
).Select runtime Java 17 (or latest supported Java version).
Choose an execution role with sufficient permissions (e.g., AWS Lambda Basic Execution Role).
Click Create function.
Now, let’s write the Lambda handler.
Step 2: Write the AWS Lambda Handler
Create a new Maven or Gradle Spring Boot project (or add it to an existing project) and add the AWS SDK dependencies:
Add Dependencies (Maven)
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-lambda-java-core</artifactId>
<version>1.2.1</version>
</dependency>
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-lambda-java-events</artifactId>
<version>3.11.1</version>
</dependency>
Lambda Handler in Java
Create a Java class that serves as the Lambda entry point:
package com.example.lambda;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class ClearCacheLambda implements RequestHandler<Object, String> {
@Override
public String handleRequest(Object input, Context context) {
context.getLogger().log("Clearing expired cache entries...");
// Your cache clearing logic here
return "Cache cleared successfully";
}
}
Step 3: Deploy the Lambda Function
1. Package and Upload the JAR
Use Maven to build a fat JAR:
mvn clean package
Upload the target/*.jar
file to AWS Lambda through the AWS Console or using AWS CLI:
aws lambda update-function-code --function-name ClearCacheLambda --zip-file fileb://target/your-lambda.jar
Step 4: Trigger Lambda on a Schedule
Instead of using @Scheduled
in Spring Boot, we'll configure Amazon EventBridge to invoke the Lambda function at specified intervals.
1. Create a Rule in Amazon EventBridge
Go to Amazon EventBridge → Rules → Create Rule.
Set a name (e.g.,
DailyCacheClearRule
).Select Schedule as the event source.
Define a cron expression (e.g.,
cron(0 0 * * ? *)
for midnight daily execution).Choose Lambda Function as the target and select your
ClearCacheLambda
function.Click Create.
Now, AWS will invoke the Lambda function based on the defined schedule.
Step 5: Invoke Lambda from a Spring Boot Application (Optional)
If you want to trigger the AWS Lambda function manually from your Spring Boot app, use the AWS SDK:
Add AWS SDK Dependency
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>lambda</artifactId>
<version>2.20.50</version>
</dependency>
Invoke Lambda from Spring Boot
package com.example.scheduler;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
import software.amazon.awssdk.services.lambda.LambdaClient;
import software.amazon.awssdk.services.lambda.model.InvokeRequest;
import software.amazon.awssdk.services.lambda.model.InvokeResponse;
import java.nio.charset.StandardCharsets;
@Service
public class LambdaScheduler {
private final LambdaClient lambdaClient = LambdaClient.create();
@Scheduled(cron = "0 0 * * * ?") // Runs daily at midnight
public void triggerLambda() {
InvokeRequest request = InvokeRequest.builder()
.functionName("ClearCacheLambda")
.build();
InvokeResponse response = lambdaClient.invoke(request);
String result = StandardCharsets.UTF_8.decode(response.payload()).toString();
System.out.println("Lambda Response: " + result);
}
}
Conclusion
By offloading scheduled tasks to AWS Lambda, you make your Spring Boot application lighter, more scalable, and cost-effective. AWS Lambda, combined with EventBridge scheduling, allows you to run tasks without maintaining servers, improving performance and reducing costs.
🚀 Next Steps: Try extending this setup with SNS notifications, S3 event triggers, or database cleanup tasks!
Got questions or insights? Share them in the comments! Let’s discuss how we can further optimize serverless scheduling in Spring Boot. 🚀
Subscribe to my newsletter
Read articles from Nikhil Soman Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
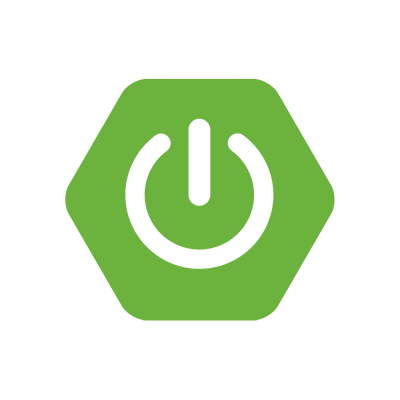
Nikhil Soman Sahu
Nikhil Soman Sahu
Software Developer